Python中识别数字的方法有多种,主要包括使用内置函数、正则表达式、类型转换、第三方库等。其中,常用的方法包括:使用内置函数isdigit()
、使用正则表达式、类型转换(如使用int()
或float()
函数)以及第三方库(如NumPy)。下面将详细介绍其中一种方法——使用正则表达式进行数字识别。
正则表达式是一个强大的工具,可以用来匹配复杂的字符串模式。Python中的re
模块提供了对正则表达式的支持。使用正则表达式可以精确地匹配各种格式的数字,包括整数、小数、负数等。具体来说,可以使用以下步骤来识别数字:
- 导入
re
模块。 - 编写匹配数字的正则表达式模式。
- 使用
re.match()
或re.search()
函数匹配字符串。
例如,以下代码段演示了如何使用正则表达式识别一个字符串是否为有效的数字:
import re
def is_number(string):
pattern = r'^-?\d+(\.\d+)?$'
return re.match(pattern, string) is not None
print(is_number("123")) # True
print(is_number("-123.45")) # True
print(is_number("abc123")) # False
下面将详细介绍Python中识别数字的其他方法。
一、使用内置函数
1. isdigit()
方法
isdigit()
是Python字符串对象的一个方法,用于判断字符串是否只包含数字字符。需要注意的是,它只能识别正整数,不包括负数、小数和其他数字格式。
num_str = "12345"
if num_str.isdigit():
print(f"{num_str} 是一个数字")
else:
print(f"{num_str} 不是一个数字")
2. isnumeric()
方法
isnumeric()
方法与isdigit()
类似,但它可以识别更多的数字形式,如罗马数字、分数等。
num_str = "12345"
if num_str.isnumeric():
print(f"{num_str} 是一个数字")
else:
print(f"{num_str} 不是一个数字")
3. isdecimal()
方法
isdecimal()
方法用于判断字符串是否只包含十进制字符。与isdigit()
和isnumeric()
相比,它的适用范围更窄。
num_str = "12345"
if num_str.isdecimal():
print(f"{num_str} 是一个数字")
else:
print(f"{num_str} 不是一个数字")
二、使用正则表达式
正则表达式是一种强大的工具,可以用来匹配复杂的字符串模式。Python中的re
模块提供了对正则表达式的支持。使用正则表达式可以精确地匹配各种格式的数字,包括整数、小数、负数等。
1. 匹配整数
要匹配整数,可以使用以下正则表达式模式:
import re
pattern = r'^-?\d+$'
2. 匹配小数
要匹配小数,可以使用以下正则表达式模式:
pattern = r'^-?\d+(\.\d+)?$'
3. 匹配科学计数法
要匹配科学计数法表示的数字,可以使用以下正则表达式模式:
pattern = r'^-?\d+(\.\d+)?([eE][-+]?\d+)?$'
示例代码
以下代码段演示了如何使用正则表达式识别一个字符串是否为有效的数字:
import re
def is_number(string):
pattern = r'^-?\d+(\.\d+)?([eE][-+]?\d+)?$'
return re.match(pattern, string) is not None
print(is_number("123")) # True
print(is_number("-123.45")) # True
print(is_number("1.23e10")) # True
print(is_number("abc123")) # False
三、使用类型转换
1. 使用 int()
函数
使用 int()
函数可以将字符串转换为整数。如果字符串不能转换为有效的整数,则会引发 ValueError
异常。
num_str = "12345"
try:
num = int(num_str)
print(f"{num_str} 是一个整数")
except ValueError:
print(f"{num_str} 不是一个整数")
2. 使用 float()
函数
使用 float()
函数可以将字符串转换为浮点数。如果字符串不能转换为有效的浮点数,则会引发 ValueError
异常。
num_str = "123.45"
try:
num = float(num_str)
print(f"{num_str} 是一个浮点数")
except ValueError:
print(f"{num_str} 不是一个浮点数")
3. 综合使用
可以综合使用 int()
和 float()
函数来判断一个字符串是否为有效的数字。
num_str = "123.45"
try:
num = int(num_str)
print(f"{num_str} 是一个整数")
except ValueError:
try:
num = float(num_str)
print(f"{num_str} 是一个浮点数")
except ValueError:
print(f"{num_str} 不是一个数字")
四、使用第三方库
1. NumPy库
NumPy是Python的一个科学计算库,提供了丰富的数值计算功能。可以使用NumPy库来判断一个字符串是否为有效的数字。
import numpy as np
def is_number(string):
try:
num = np.float64(string)
return True
except ValueError:
return False
print(is_number("123")) # True
print(is_number("-123.45")) # True
print(is_number("abc123")) # False
2. pandas库
Pandas是Python的一个数据分析库,也提供了数值识别的功能。可以使用Pandas库来判断一个字符串是否为有效的数字。
import pandas as pd
def is_number(string):
return pd.to_numeric(string, errors='coerce').notna()
print(is_number("123")) # True
print(is_number("-123.45")) # True
print(is_number("abc123")) # False
3. 使用 decimal
模块
decimal
模块提供了用于十进制浮点运算的 Decimal
数据类型。可以使用 decimal.Decimal()
方法来判断一个字符串是否为有效的数字。
from decimal import Decimal, InvalidOperation
def is_number(string):
try:
num = Decimal(string)
return True
except InvalidOperation:
return False
print(is_number("123")) # True
print(is_number("-123.45")) # True
print(is_number("abc123")) # False
五、更多正则表达式示例
1. 匹配整数和小数(包括正负数)
import re
pattern = r'^-?\d+(\.\d+)?$'
def is_number(string):
return re.match(pattern, string) is not None
print(is_number("123")) # True
print(is_number("-123.45")) # True
print(is_number("abc123")) # False
2. 匹配科学计数法表示的数字
import re
pattern = r'^-?\d+(\.\d+)?([eE][-+]?\d+)?$'
def is_number(string):
return re.match(pattern, string) is not None
print(is_number("1.23e10")) # True
print(is_number("-1.23E-10")) # True
print(is_number("123abc")) # False
3. 匹配带千位分隔符的数字
import re
pattern = r'^-?\d{1,3}(,\d{3})*(\.\d+)?$'
def is_number(string):
return re.match(pattern, string) is not None
print(is_number("1,234")) # True
print(is_number("-1,234.56")) # True
print(is_number("1234,567")) # False
六、综合示例
以下是一个综合示例,演示了如何使用多种方法来判断一个字符串是否为有效的数字。
import re
import numpy as np
import pandas as pd
from decimal import Decimal, InvalidOperation
def is_number(string):
# 使用正则表达式匹配整数和小数
pattern = r'^-?\d+(\.\d+)?([eE][-+]?\d+)?$'
if re.match(pattern, string):
return True
# 使用 int() 函数
try:
num = int(string)
return True
except ValueError:
pass
# 使用 float() 函数
try:
num = float(string)
return True
except ValueError:
pass
# 使用 NumPy 库
try:
num = np.float64(string)
return True
except ValueError:
pass
# 使用 pandas 库
if pd.to_numeric(string, errors='coerce').notna():
return True
# 使用 decimal 模块
try:
num = Decimal(string)
return True
except InvalidOperation:
pass
return False
print(is_number("123")) # True
print(is_number("-123.45")) # True
print(is_number("1.23e10")) # True
print(is_number("1,234.56")) # False
print(is_number("abc123")) # False
通过上述方法和示例,我们可以在Python中有效地识别各种格式的数字,包括整数、小数、负数、科学计数法等。不同的方法有不同的适用范围和特点,可以根据实际需求选择合适的方法来判断字符串是否为有效的数字。
相关问答FAQs:
如何在Python中检查一个字符串是否为数字?
在Python中,可以使用内置的str.isdigit()
方法来检查字符串是否仅包含数字字符。例如,'123'.isdigit()
会返回True
,而'123a'.isdigit()
则返回False
。此外,使用try
和except
结构结合int()
或float()
函数也能有效判断字符串是否可以转换为数字。
Python中有哪些库可以用于数字识别?
在Python中,常用的库如re
(正则表达式)和numpy
可以帮助识别和处理数字。使用re
库,可以通过编写模式匹配来识别字符串中的数字,例如通过re.findall(r'\d+', my_string)
可以提取所有数字。numpy
则提供了多种数学函数,适合处理和分析数值数据。
如何处理包含数字的混合字符串?
在Python中处理混合字符串(即同时包含字母和数字的字符串)时,可以使用正则表达式来提取数字部分。例如,通过re.findall(r'\d+', mixed_string)
可以获取所有数字,返回值会是一个列表。如果需要将提取的数字转换为整数或浮点数,可以使用map()
函数来处理列表中的每一个元素。
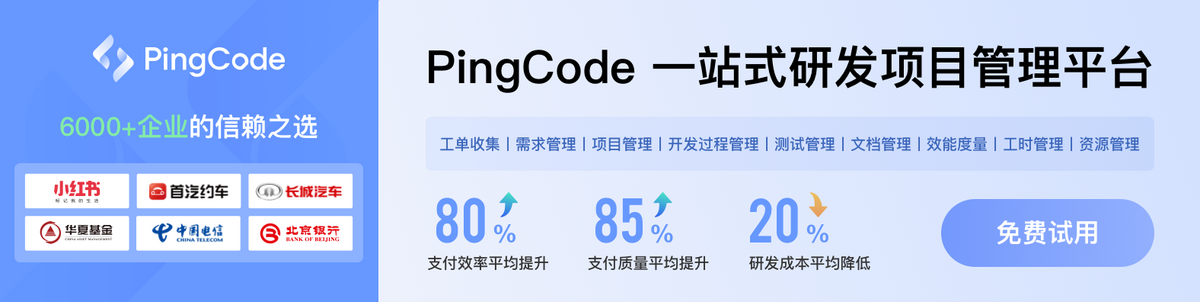