Caffe写Python层的方法有:编写Python代码文件、定义类并继承caffe.Layer、实现setup、reshape、forward、backward方法。具体步骤如下:
编写Python代码文件:创建一个Python文件,并在其中编写自定义层的代码。这个文件应包含一个定义自定义层的类。
定义类并继承caffe.Layer:在Python文件中定义一个类,该类应继承自caffe.Layer类。
实现setup方法:在类中实现setup方法,用于初始化层的参数和配置。
实现reshape方法:实现reshape方法,用于定义层的输出形状。
实现forward方法:实现forward方法,用于在前向传播时计算输出。
实现backward方法(可选):实现backward方法,用于在反向传播时计算梯度。
一、编写Python代码文件
首先,创建一个Python文件,并在其中编写自定义层的代码。假设这个文件名为my_layer.py
。在文件中导入必要的模块,例如caffe和numpy。
import caffe
import numpy as np
二、定义类并继承caffe.Layer
在Python文件中定义一个类,该类应继承自caffe.Layer
类。
class MyLayer(caffe.Layer):
def setup(self, bottom, top):
pass
def reshape(self, bottom, top):
pass
def forward(self, bottom, top):
pass
def backward(self, top, propagate_down, bottom):
pass
三、实现setup方法
setup
方法用于初始化层的参数和配置。它接收两个参数:bottom和top,分别代表输入和输出的Blob列表。在这个方法中,我们可以解析参数和配置。
class MyLayer(caffe.Layer):
def setup(self, bottom, top):
# 检查输入参数数量是否正确
if len(bottom) != 1:
raise Exception("Only one input is allowed.")
# 读取参数并进行初始化
params = eval(self.param_str)
self.param1 = params['param1']
self.param2 = params['param2']
四、实现reshape方法
reshape
方法用于定义层的输出形状。它接收两个参数:bottom和top,分别代表输入和输出的Blob列表。在这个方法中,我们可以设置输出Blob的形状。
class MyLayer(caffe.Layer):
def reshape(self, bottom, top):
# 设置输出Blob的形状
top[0].reshape(*bottom[0].shape)
五、实现forward方法
forward
方法用于在前向传播时计算输出。它接收两个参数:bottom和top,分别代表输入和输出的Blob列表。在这个方法中,我们可以实现前向传播的计算逻辑。
class MyLayer(caffe.Layer):
def forward(self, bottom, top):
# 实现前向传播的计算逻辑
top[0].data[...] = bottom[0].data + self.param1
六、实现backward方法(可选)
backward
方法用于在反向传播时计算梯度。它接收三个参数:top、propagate_down和bottom,分别代表输出、是否向下传播梯度和输入的Blob列表。在这个方法中,我们可以实现反向传播的梯度计算逻辑。
class MyLayer(caffe.Layer):
def backward(self, top, propagate_down, bottom):
# 实现反向传播的梯度计算逻辑
if propagate_down[0]:
bottom[0].diff[...] = top[0].diff
七、使用自定义Python层
在完成自定义Python层的实现后,可以在Caffe的prototxt文件中使用该层。首先,在prototxt文件中定义一个Python层,并指定layer参数和模块路径。
layer {
name: "my_layer"
type: "Python"
bottom: "input"
top: "output"
python_param {
module: "my_layer"
layer: "MyLayer"
param_str: "{'param1': 1.0, 'param2': 2.0}"
}
}
八、常见问题及解决方法
1、无法找到模块
在使用自定义Python层时,可能会遇到无法找到模块的问题。确保Python文件所在的目录在Python的搜索路径中,可以通过以下方式添加路径:
import sys
sys.path.append('/path/to/your/python/files')
2、参数解析错误
在解析参数时,确保参数字符串格式正确。例如,使用JSON格式的字符串,并在Python代码中使用eval
或json.loads
进行解析。
3、形状匹配错误
在实现reshape方法时,确保输入和输出Blob的形状匹配。如果形状不匹配,可能会导致运行时错误。
4、梯度计算错误
在实现backward方法时,确保梯度计算正确。如果梯度计算错误,可能会影响模型的训练效果。
九、实际应用中的案例
案例一:自定义归一化层
下面是一个自定义归一化层的实现示例,该层将输入数据归一化到指定范围内。
import caffe
import numpy as np
class NormalizeLayer(caffe.Layer):
def setup(self, bottom, top):
params = eval(self.param_str)
self.min_val = params['min_val']
self.max_val = params['max_val']
def reshape(self, bottom, top):
top[0].reshape(*bottom[0].shape)
def forward(self, bottom, top):
data = bottom[0].data
norm_data = (data - data.min()) / (data.max() - data.min())
top[0].data[...] = norm_data * (self.max_val - self.min_val) + self.min_val
def backward(self, top, propagate_down, bottom):
if propagate_down[0]:
bottom[0].diff[...] = top[0].diff
在prototxt文件中使用自定义归一化层:
layer {
name: "normalize_layer"
type: "Python"
bottom: "input"
top: "output"
python_param {
module: "normalize_layer"
layer: "NormalizeLayer"
param_str: "{'min_val': 0.0, 'max_val': 1.0}"
}
}
案例二:自定义激活函数层
下面是一个自定义激活函数层的实现示例,该层实现了ReLU激活函数。
import caffe
import numpy as np
class ReLULayer(caffe.Layer):
def setup(self, bottom, top):
pass
def reshape(self, bottom, top):
top[0].reshape(*bottom[0].shape)
def forward(self, bottom, top):
top[0].data[...] = np.maximum(bottom[0].data, 0)
def backward(self, top, propagate_down, bottom):
if propagate_down[0]:
bottom[0].diff[...] = top[0].diff * (bottom[0].data > 0)
在prototxt文件中使用自定义激活函数层:
layer {
name: "relu_layer"
type: "Python"
bottom: "input"
top: "output"
python_param {
module: "relu_layer"
layer: "ReLULayer"
}
}
十、调试和测试
在实现自定义Python层后,进行调试和测试非常重要。可以通过以下步骤进行调试和测试:
1、单元测试
编写单元测试代码,测试自定义层的各个方法。例如,使用unittest
模块编写测试代码。
import unittest
import numpy as np
from my_layer import MyLayer
class TestMyLayer(unittest.TestCase):
def test_forward(self):
layer = MyLayer()
bottom = [np.array([[1, 2], [3, 4]])]
top = [np.zeros((2, 2))]
layer.setup(bottom, top)
layer.reshape(bottom, top)
layer.forward(bottom, top)
self.assertTrue(np.array_equal(top[0], bottom[0] + 1.0))
if __name__ == '__main__':
unittest.main()
2、运行Caffe模型
在Caffe模型中使用自定义Python层,并运行模型进行验证。可以使用Caffe的命令行工具进行训练和测试,确保自定义层的功能和性能符合预期。
caffe train -solver solver.prototxt
3、调试信息输出
在自定义层的代码中添加调试信息输出,例如使用print
函数输出中间结果,以便检查和定位问题。
class MyLayer(caffe.Layer):
def forward(self, bottom, top):
print("Input data:", bottom[0].data)
top[0].data[...] = bottom[0].data + self.param1
print("Output data:", top[0].data)
十一、优化和改进
在完成自定义Python层的初步实现和测试后,可以进行优化和改进,以提高性能和功能。例如,使用NumPy的矢量化操作提高计算效率,添加更多的参数和配置选项,支持更多的输入和输出类型等。
1、使用NumPy的矢量化操作
在计算过程中,尽量使用NumPy的矢量化操作,以提高计算效率。例如,使用NumPy的内置函数进行批量操作,而不是使用循环。
class MyLayer(caffe.Layer):
def forward(self, bottom, top):
top[0].data[...] = np.add(bottom[0].data, self.param1)
2、添加更多的参数和配置选项
根据需求,添加更多的参数和配置选项,以提高自定义层的灵活性和适用性。例如,添加更多的激活函数选项,支持不同的归一化方式等。
class MyLayer(caffe.Layer):
def setup(self, bottom, top):
params = eval(self.param_str)
self.param1 = params.get('param1', 1.0)
self.activation = params.get('activation', 'relu')
def forward(self, bottom, top):
if self.activation == 'relu':
top[0].data[...] = np.maximum(bottom[0].data + self.param1, 0)
elif self.activation == 'sigmoid':
top[0].data[...] = 1 / (1 + np.exp(-(bottom[0].data + self.param1)))
3、支持更多的输入和输出类型
根据需求,支持更多的输入和输出类型,例如多输入多输出、多维度数据等。
class MyLayer(caffe.Layer):
def setup(self, bottom, top):
params = eval(self.param_str)
self.param1 = params.get('param1', 1.0)
self.param2 = params.get('param2', 2.0)
def reshape(self, bottom, top):
for i in range(len(top)):
top[i].reshape(*bottom[i].shape)
def forward(self, bottom, top):
for i in range(len(top)):
top[i].data[...] = bottom[i].data * self.param1 + self.param2
通过以上步骤,我们可以在Caffe中编写自定义的Python层,并在实际应用中使用、调试和优化。自定义Python层的实现可以满足特定的需求,提高模型的灵活性和适用性。
相关问答FAQs:
如何在Caffe中添加自定义的Python层?
在Caffe中添加自定义Python层的过程主要包括创建Python模块、定义层的前向和反向传播方法,以及在Caffe的配置文件中注册该层。首先,你需要安装Caffe并确保Python环境配置正确。接下来,创建一个Python脚本,继承caffe.Layer
类,并实现setup
, reshape
, forward
, 和backward
等方法。完成后,你可以在Caffe的网络配置文件中引入这个新层。
Caffe中的Python层与C++层有什么区别?
Python层与C++层的主要区别在于灵活性和性能。Python层通常用于快速原型开发和模型调试,它允许用户快速实现和修改算法,但在性能上可能不如C++层。C++层则更适合需要高效计算和优化的场景,通常用于生产环境中的模型部署。用户可以根据项目需求选择合适的层类型。
在使用Python层时,如何调试和测试?
调试和测试Python层的方法包括使用Caffe提供的测试工具和Python的调试工具。可以在Python脚本中添加打印语句来检查输入和输出数据,确保数据在预期的范围内。此外,还可以使用Python的pdb
库进行逐行调试,分析代码执行过程中的变量状态。这些方法将帮助用户快速定位问题并优化代码。
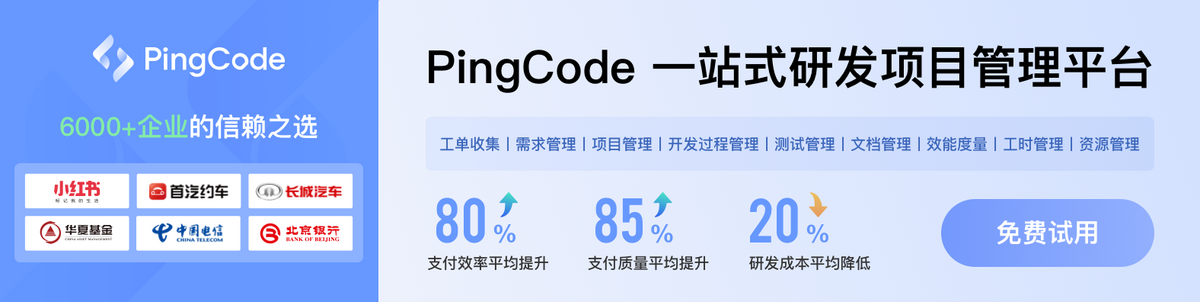