Python 通过使用库生成词云的过程包括:安装相关库、准备文本数据、创建并自定义词云、显示和保存词云。 本文将详细介绍如何使用Python创建和自定义词云,以及一些常见问题的解决方案。
一、安装和导入相关库
在开始创建词云之前,首先需要安装一些必要的Python库。这些库包括wordcloud
、matplotlib
和Pillow
。安装这些库可以使用pip命令:
pip install wordcloud matplotlib pillow
安装完成后,导入这些库:
from wordcloud import WordCloud
import matplotlib.pyplot as plt
from PIL import Image
import numpy as np
二、准备文本数据
词云的生成需要输入文本数据。文本数据可以来自文件、字符串或网络资源。下面是一些示例代码,用来从一个文本文件读取数据:
# 从文本文件读取数据
with open('your_text_file.txt', 'r', encoding='utf-8') as file:
text = file.read()
你也可以直接使用字符串:
text = "Python is an amazing programming language. It is widely used for data science, web development, automation, and more."
三、创建和自定义词云
使用WordCloud类来创建词云,并自定义它的外观和行为。以下是一些常用的自定义选项:
wordcloud = WordCloud(
width=800,
height=400,
background_color='white',
max_words=200,
colormap='viridis',
contour_width=1,
contour_color='steelblue'
).generate(text)
在上面的代码中,我们指定了词云的宽度、高度、背景颜色、最大词数、颜色映射、轮廓宽度和轮廓颜色。你可以根据需要调整这些参数。
四、显示和保存词云
生成词云后,我们可以使用matplotlib
库来显示它,并使用Pillow
库来保存图像。
# 显示词云
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
保存词云到文件
wordcloud.to_file('wordcloud.png')
五、使用掩膜图像创建词云
我们还可以使用掩膜图像来创建特定形状的词云。掩膜图像是一幅黑白图像,其中白色部分表示词云可以填充的位置。
# 导入掩膜图像
mask = np.array(Image.open('mask_image.png'))
创建词云并应用掩膜
wordcloud = WordCloud(
width=800,
height=400,
background_color='white',
mask=mask,
contour_width=1,
contour_color='black'
).generate(text)
显示词云
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
六、处理中文词云
处理中文词云时,需要进行分词。我们可以使用jieba
库来实现分词,并创建中文词云。
import jieba
进行分词
text = "Python是一种非常流行的编程语言。它广泛用于数据科学、Web开发、自动化等领域。"
cut_text = " ".join(jieba.cut(text))
创建词云
wordcloud = WordCloud(
font_path='path_to_chinese_font.ttf',
width=800,
height=400,
background_color='white',
max_words=200,
colormap='viridis',
contour_width=1,
contour_color='steelblue'
).generate(cut_text)
显示词云
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
在上面的代码中,我们使用jieba.cut
函数对中文文本进行分词,并将分词结果连接成一个字符串。然后,我们使用指定的中文字体文件生成词云。
七、分析和解决常见问题
-
词云生成速度慢:如果词云生成速度较慢,可以尝试减少最大词数、降低图像分辨率或优化文本预处理。例如,删除停用词和低频词可以提高词云生成效率。
-
词频统计不准确:确保输入文本经过适当的预处理,例如删除标点符号、转换为小写、删除停用词等。可以使用
nltk
库来进行文本预处理。 -
图像颜色和效果不佳:尝试使用不同的颜色映射、背景颜色和轮廓颜色来调整词云的外观。还可以使用自定义颜色函数来设置每个词的颜色。
-
中文词云乱码:确保指定了正确的中文字体文件,并在生成词云时使用该字体文件。可以下载常用的中文字体文件并将其路径指定给
font_path
参数。
八、扩展词云的应用
除了生成基本的词云外,我们还可以将词云应用到更多场景中。例如,结合自然语言处理技术进行情感分析、主题建模等高级分析任务。
- 情感分析:可以对文本进行情感分析,将正面和负面词汇分别生成不同颜色的词云,以直观展示文本的情感倾向。
from textblob import TextBlob
进行情感分析
text = "Python is an amazing programming language. It is widely used for data science, web development, automation, and more."
blob = TextBlob(text)
positive_words = [word for word in blob.words if TextBlob(word).sentiment.polarity > 0]
negative_words = [word for word in blob.words if TextBlob(word).sentiment.polarity < 0]
创建正面词云
positive_wordcloud = WordCloud(
width=800,
height=400,
background_color='white',
colormap='cool'
).generate(" ".join(positive_words))
创建负面词云
negative_wordcloud = WordCloud(
width=800,
height=400,
background_color='white',
colormap='hot'
).generate(" ".join(negative_words))
显示词云
plt.figure(figsize=(10, 5))
plt.subplot(1, 2, 1)
plt.imshow(positive_wordcloud, interpolation='bilinear')
plt.axis('off')
plt.title('Positive Words')
plt.subplot(1, 2, 2)
plt.imshow(negative_wordcloud, interpolation='bilinear')
plt.axis('off')
plt.title('Negative Words')
plt.show()
- 主题建模:可以使用主题建模技术(如LDA)从文本中提取主题词汇,并生成每个主题的词云。
from sklearn.feature_extraction.text import CountVectorizer
from sklearn.decomposition import LatentDirichletAllocation
准备文本数据
texts = [
"Python is great for data science.",
"JavaScript is popular for web development.",
"Machine learning is a key skill for data scientists.",
"Web development involves HTML, CSS, and JavaScript."
]
向量化文本数据
vectorizer = CountVectorizer(stop_words='english')
text_matrix = vectorizer.fit_transform(texts)
进行LDA主题建模
lda = LatentDirichletAllocation(n_components=2, random_state=42)
lda.fit(text_matrix)
提取主题词汇
terms = vectorizer.get_feature_names_out()
for topic_idx, topic in enumerate(lda.components_):
print(f"Topic {topic_idx}:")
print(" ".join([terms[i] for i in topic.argsort()[:-11:-1]]))
生成主题词云
for topic_idx, topic in enumerate(lda.components_):
topic_words = " ".join([terms[i] for i in topic.argsort()[:-11:-1]])
wordcloud = WordCloud(
width=800,
height=400,
background_color='white'
).generate(topic_words)
plt.figure()
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.title(f'Topic {topic_idx}')
plt.show()
- 词云动画:可以创建词云动画,展示文本随时间变化的词频。例如,分析社交媒体数据中的热点话题,生成随时间变化的词云动画。
import matplotlib.animation as animation
准备文本数据
texts = [
"Python is great for data science.",
"JavaScript is popular for web development.",
"Machine learning is a key skill for data scientists.",
"Web development involves HTML, CSS, and JavaScript."
]
创建动画函数
def update_wordcloud(i, wordcloud, ax):
ax.clear()
wordcloud.generate(texts[i])
ax.imshow(wordcloud, interpolation='bilinear')
ax.axis('off')
创建词云对象
wordcloud = WordCloud(
width=800,
height=400,
background_color='white'
)
创建动画
fig, ax = plt.subplots()
ani = animation.FuncAnimation(fig, update_wordcloud, frames=len(texts), fargs=(wordcloud, ax), interval=2000)
plt.show()
通过以上示例,我们可以看到词云在不同场景中的应用。掌握这些技术可以帮助我们更好地分析和可视化文本数据,挖掘有价值的信息。
九、优化词云生成的技巧
-
使用高质量的掩膜图像:如果使用掩膜图像来创建特定形状的词云,确保掩膜图像的质量足够高,以避免生成的词云出现模糊或失真。
-
调整词云参数:根据文本数据的特点,调整词云参数(如最大词数、最小词频、颜色映射等),以生成更符合预期的词云。
-
预处理文本数据:对文本数据进行适当的预处理(如去除停用词、标点符号、低频词等),可以提高词云的可读性和准确性。
-
使用自定义颜色函数:自定义颜色函数可以使词云的颜色更加丰富多样,提升视觉效果。
import random
def random_color(word, font_size, position, orientation, random_state=None, kwargs):
return "hsl({}, 100%, 50%)".format(random.randint(0, 360))
wordcloud = WordCloud(
width=800,
height=400,
background_color='white',
color_func=random_color
).generate(text)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
十、总结
通过本文的介绍,我们详细讲解了如何使用Python创建和自定义词云,包括安装相关库、准备文本数据、创建和显示词云、处理中文词云、分析常见问题和扩展应用等内容。希望这些内容能帮助你更好地掌握词云的生成技巧,并将其应用到实际项目中。
相关问答FAQs:
如何使用Python生成词云?
要生成词云,您可以使用Python中的wordcloud
库。首先,确保安装了此库,可以通过命令pip install wordcloud
来安装。接下来,准备您的文本数据,并使用以下代码示例来生成词云:
from wordcloud import WordCloud
import matplotlib.pyplot as plt
text = "您的文本数据在这里"
wordcloud = WordCloud(width=800, height=400, background_color='white').generate(text)
plt.figure(figsize=(10, 5))
plt.imshow(wordcloud, interpolation='bilinear')
plt.axis('off')
plt.show()
通过此代码,您可以快速创建并可视化词云。
生成词云时需要注意哪些参数设置?
在生成词云时,您可以调整多个参数以获得最佳效果。例如,width
和height
参数可以控制图像的尺寸,background_color
用于设置背景颜色。此外,可以使用max_words
参数限制词云中显示的最多单词数量,并通过colormap
指定颜色方案,这样可以确保生成的词云既美观又有吸引力。
如何处理文本数据以提高词云的质量?
为了提高生成词云的质量,预处理文本数据非常重要。常见的处理方法包括:去除停用词(如“的”、“是”等无意义的词)、进行词干提取或词形还原以及清除标点符号和特殊字符。可以使用nltk
或re
库来实现这些文本处理操作。良好的文本预处理可以显著增强词云的可读性和视觉效果。
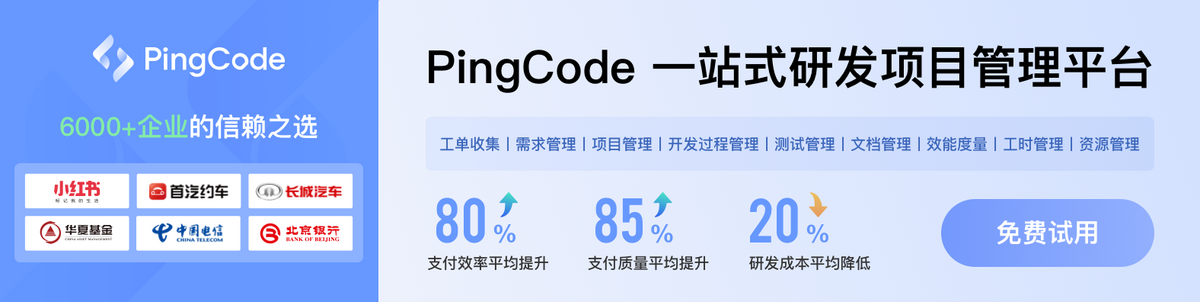