Python3中可以通过多种方法对字符串进行空格切片,如使用split()方法、正则表达式、列表解析等。最常用的是split()方法,它可以将字符串按空格分割成一个列表。接下来我们详细介绍一下如何使用split()方法进行空格切片。
使用split()方法进行空格切片非常简单,它会将字符串按空格分割成一个列表。如果不传递参数给split()方法,它会默认按任意数量的空格进行分割。示例如下:
text = "Python 3 is great for beginners and experts alike"
words = text.split()
print(words)
在上面的代码中,字符串text
被按空格分割成单词列表words
,并输出结果。接下来,我们将从多个方面详细介绍Python3中空格切片的各种方法。
一、SPLIT()方法
1、基本用法
split()方法是Python字符串方法之一,它可以将字符串按指定的分隔符进行分割。如果不传递参数,默认按任意数量的空格进行分割。以下是基本用法示例:
text = "Python 3 is great for beginners and experts alike"
words = text.split()
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
上述代码中,字符串text
被按空格分割成单词列表words
。
2、指定分隔符
split()方法还可以通过传递参数来指定分隔符,例如逗号、分号等。以下是示例:
text = "Python,3,is,great,for,beginners,and,experts,alike"
words = text.split(',')
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,字符串text
被按逗号分割成单词列表words
。
3、限制分割次数
split()方法还可以通过传递第二个参数来限制分割次数。例如,以下代码将字符串text
分割成最多三个部分:
text = "Python 3 is great for beginners and experts alike"
words = text.split(' ', 3)
print(words) # ['Python', '3', 'is', 'great for beginners and experts alike']
在此示例中,字符串text
被按空格分割成最多三个部分。
二、正则表达式
1、使用re.split()
正则表达式(regular expression,简称regex)是用来匹配字符串中字符组合的模式。在Python中,可以使用re
模块来处理正则表达式。re.split()
方法可以按正则表达式分隔字符串。以下是示例:
import re
text = "Python 3 is great for beginners and experts alike"
words = re.split(r'\s+', text)
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,使用正则表达式\s+
匹配一个或多个空格,将字符串text
分割成单词列表words
。
2、复杂的分隔模式
正则表达式还可以用于更复杂的分隔模式。例如,要按逗号或分号分隔字符串,可以使用以下代码:
import re
text = "Python;3,is;great,for;beginners,and,experts;alike"
words = re.split(r'[;,]', text)
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,使用正则表达式[;,]
匹配逗号或分号,将字符串text
分割成单词列表words
。
三、列表解析
1、基本用法
列表解析是一种简洁的创建列表的方式,它可以与split()方法结合使用来实现空格切片。以下是示例:
text = "Python 3 is great for beginners and experts alike"
words = [word for word in text.split()]
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,使用列表解析将字符串text
按空格分割成单词列表words
。
2、过滤空字符串
在某些情况下,字符串中可能包含多个连续的空格,导致split()方法返回空字符串。可以使用列表解析过滤掉这些空字符串。例如:
text = "Python 3 is great for beginners and experts alike"
words = [word for word in text.split() if word]
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,使用列表解析过滤掉了由多个连续空格导致的空字符串。
四、字符串切片
1、基本用法
字符串切片是一种访问字符串中子字符串的方法。虽然不直接用于空格切片,但它可以用来处理字符串中的特定部分。例如:
text = "Python 3 is great for beginners and experts alike"
first_word = text[:6]
print(first_word) # 'Python'
在此示例中,使用字符串切片获取字符串text
的第一个单词。
2、结合find()方法
字符串切片可以与find()方法结合使用,按空格分割字符串。例如:
text = "Python 3 is great for beginners and experts alike"
first_space = text.find(' ')
first_word = text[:first_space]
print(first_word) # 'Python'
在此示例中,使用find()方法找到第一个空格的位置,然后使用字符串切片获取第一个单词。
五、使用生成器
1、基本用法
生成器是一种迭代器,可以在需要时生成值。生成器可以与split()方法结合使用来按空格分割字符串。例如:
def word_generator(text):
for word in text.split():
yield word
text = "Python 3 is great for beginners and experts alike"
words = list(word_generator(text))
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,使用生成器函数word_generator
按空格分割字符串text
,并生成单词列表words
。
2、处理大字符串
生成器特别适合处理大字符串,因为它们不会一次性将所有单词加载到内存中。例如:
def word_generator(text):
for word in text.split():
yield word
large_text = "Python " * 1000000 # 大字符串
words = word_generator(large_text)
仅打印前10个单词
for i, word in enumerate(words):
if i >= 10:
break
print(word)
在此示例中,使用生成器处理大字符串large_text
,并仅打印前10个单词。
六、使用itertools模块
1、基本用法
itertools模块提供了一组用于操作迭代器的工具。可以使用itertools.chain()方法将多个迭代器组合成一个。例如:
import itertools
text1 = "Python 3 is great"
text2 = "for beginners and experts alike"
words = list(itertools.chain(text1.split(), text2.split()))
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,使用itertools.chain()方法将两个字符串按空格分割后的单词列表组合成一个。
2、组合多个字符串
itertools模块还可以用于组合多个字符串的单词列表。例如:
import itertools
texts = ["Python 3 is great", "for beginners and experts alike", "Enjoy coding!"]
words = list(itertools.chain(*[text.split() for text in texts]))
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike', 'Enjoy', 'coding!']
在此示例中,使用itertools.chain()方法将多个字符串按空格分割后的单词列表组合成一个。
七、使用collections模块
1、基本用法
collections模块提供了一组容器数据类型。可以使用collections.deque()方法来存储按空格分割的单词。例如:
from collections import deque
text = "Python 3 is great for beginners and experts alike"
words = deque(text.split())
print(words) # deque(['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike'])
在此示例中,使用collections.deque()方法将字符串text
按空格分割成单词队列words
。
2、操作双端队列
collections.deque()方法创建的双端队列可以高效地在两端添加或删除元素。例如:
from collections import deque
text = "Python 3 is great for beginners and experts alike"
words = deque(text.split())
words.appendleft("Hello")
words.append("World")
print(words) # deque(['Hello', 'Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike', 'World'])
在此示例中,使用collections.deque()方法将字符串text
按空格分割成单词队列words
,并在两端添加元素。
八、使用numpy模块
1、基本用法
numpy模块是一个强大的数值计算库,虽然不直接用于字符串操作,但可以结合numpy数组进行操作。例如:
import numpy as np
text = "Python 3 is great for beginners and experts alike"
words = np.array(text.split())
print(words) # ['Python' '3' 'is' 'great' 'for' 'beginners' 'and' 'experts' 'alike']
在此示例中,使用numpy.array()方法将字符串text
按空格分割成单词数组words
。
2、数组操作
numpy数组可以进行高效的数组操作。例如:
import numpy as np
text = "Python 3 is great for beginners and experts alike"
words = np.array(text.split())
print(words[0]) # 'Python'
print(words[-1]) # 'alike'
在此示例中,使用numpy数组操作获取字符串text
按空格分割后的第一个和最后一个单词。
九、使用pandas模块
1、基本用法
pandas模块是一个强大的数据分析库,可以用于处理表格数据。虽然不直接用于字符串操作,但可以结合pandas数据框进行操作。例如:
import pandas as pd
text = "Python 3 is great for beginners and experts alike"
words = pd.Series(text.split())
print(words)
在此示例中,使用pandas.Series()方法将字符串text
按空格分割成单词系列words
。
2、数据分析
pandas数据框可以用于高效的数据分析。例如:
import pandas as pd
text = "Python 3 is great for beginners and experts alike"
words = pd.Series(text.split())
print(words.value_counts())
在此示例中,使用pandas.Series()方法将字符串text
按空格分割成单词系列words
,并统计每个单词的出现次数。
十、使用string模块
1、基本用法
string模块提供了一些常用的字符串操作工具。例如,可以使用string.whitespace来去除字符串中的空格。例如:
import string
text = "Python 3 is great for beginners and experts alike"
words = text.split()
print([word.strip(string.whitespace) for word in words])
在此示例中,使用string.whitespace去除字符串text
按空格分割后的单词中的空格。
2、去除多种空白字符
string模块还可以用于去除多种空白字符。例如:
import string
text = "Python\t3\nis great for beginners and experts alike"
words = text.split()
print([word.strip(string.whitespace) for word in words])
在此示例中,使用string.whitespace去除字符串text
按空格分割后的单词中的制表符和换行符。
十一、使用自定义函数
1、基本用法
可以编写自定义函数来按空格分割字符串。例如:
def split_by_space(text):
return text.split()
text = "Python 3 is great for beginners and experts alike"
words = split_by_space(text)
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,定义了自定义函数split_by_space
来按空格分割字符串text
。
2、处理特殊情况
自定义函数可以用于处理特殊情况。例如:
def split_by_space(text):
return [word for word in text.split() if word]
text = "Python 3 is great for beginners and experts alike"
words = split_by_space(text)
print(words) # ['Python', '3', 'is', 'great', 'for', 'beginners', 'and', 'experts', 'alike']
在此示例中,自定义函数split_by_space
过滤掉了由多个连续空格导致的空字符串。
十二、总结
在Python3中,空格切片可以通过多种方法实现,包括split()方法、正则表达式、列表解析、字符串切片、生成器、itertools模块、collections模块、numpy模块、pandas模块、string模块、自定义函数等。每种方法都有其独特的优点和适用场景。通过灵活运用这些方法,可以高效地处理各种字符串操作需求。
相关问答FAQs:
如何在Python3中实现空格切片?
在Python3中,可以使用字符串的split()
方法来实现空格切片。这种方法会根据空格将字符串拆分成多个部分,并返回一个列表。例如,"hello world".split()
会返回['hello', 'world']
。如果你希望保留多个空格之间的空白内容,可以使用re
模块中的正则表达式。
空格切片的结果是什么样的?
使用空格切片后,字符串将被分割为由空格分开的多个子字符串。这些子字符串将组成一个列表。例如,输入"Python is fun"
,经过空格切片后,输出将是['Python', 'is', 'fun']
。如果你需要获取某个特定位置的切片内容,可以直接通过索引访问。
除了空格切片,还有哪些其他切片方法?
除了空格切片,Python还支持多种切片操作。例如,可以使用split()
方法按其他字符分割字符串,如逗号或分号。另一个常用的切片方法是利用切片语法string[start:end]
来提取字符串的一部分。通过结合这些方法,用户可以灵活处理各种字符串需求。
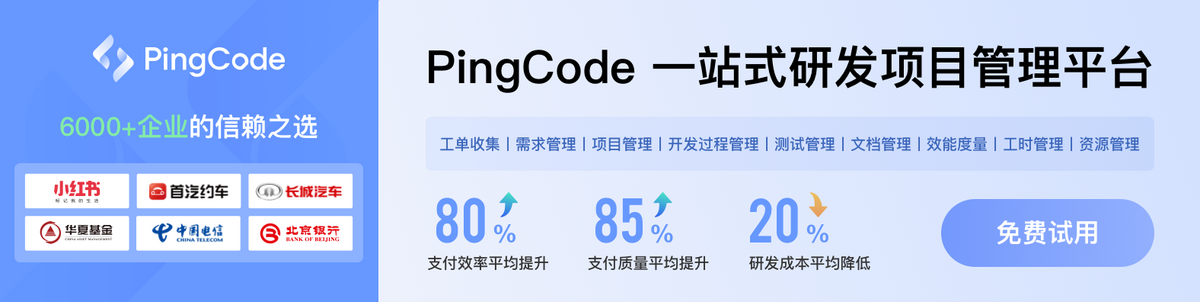