如何抽取关键字python:使用TF-IDF算法、使用TextRank算法、使用分词工具、使用主题模型、使用机器学习
在本文的开头,我们将直接回答标题所提问题。关键字抽取在文本分析中是一个非常重要的步骤,Python提供了多种方法来实现这一目标。主要方法包括:使用TF-IDF算法、使用TextRank算法、使用分词工具、使用主题模型、使用机器学习。其中,使用TF-IDF算法是一种常见且有效的关键字抽取方法。TF-IDF算法通过计算词频和逆文档频率,衡量一个词在文档中的重要性。具体来说,TF-IDF值越高,表示该词在文档中越重要。下面我们将详细介绍如何在Python中实现这些关键字抽取方法。
一、使用TF-IDF算法
TF-IDF(Term Frequency-Inverse Document Frequency)是一种常用的文本分析技术,用于衡量一个词在文档中的重要性。它结合了词频(TF)和逆文档频率(IDF)两个指标,来评价一个词在文档集中的代表性。TF-IDF算法的公式如下:
[ \text{TF-IDF}(t,d) = \text{TF}(t,d) \times \text{IDF}(t) ]
其中:
- (\text{TF}(t,d)) 是词 (t) 在文档 (d) 中出现的次数。
- (\text{IDF}(t)) 是词 (t) 在文档集中的逆文档频率,计算公式为:[ \text{IDF}(t) = \log \left( \frac{N}{1 + \text{DF}(t)} \right) ],其中 (N) 是文档总数,(\text{DF}(t)) 是包含词 (t) 的文档数量。
1.1、导入必要的库
from sklearn.feature_extraction.text import TfidfVectorizer
import pandas as pd
1.2、准备文本数据
documents = [
"Python is a high-level programming language.",
"Machine learning and data science are applications of Python.",
"Python is popular for web development.",
"Data analysis and machine learning are key applications of Python."
]
1.3、计算TF-IDF值
vectorizer = TfidfVectorizer()
tfidf_matrix = vectorizer.fit_transform(documents)
1.4、提取关键字
feature_names = vectorizer.get_feature_names_out()
for doc in range(len(documents)):
df = pd.DataFrame(tfidf_matrix[doc].T.todense(), index=feature_names, columns=["TF-IDF"])
df = df.sort_values(by=["TF-IDF"], ascending=False)
print(f"Document {doc+1} top keywords:\n", df.head(5))
二、使用TextRank算法
TextRank是一种基于图的排序算法,用于抽取文本中的重要信息。它类似于PageRank算法,最初用于网页排名。TextRank通过构建词语之间的关系图,利用图的结构来确定每个词的重要性。
2.1、导入必要的库
import jieba.analyse
2.2、准备文本数据
text = "Python is a high-level programming language. Machine learning and data science are applications of Python. Python is popular for web development. Data analysis and machine learning are key applications of Python."
2.3、使用TextRank算法抽取关键字
keywords = jieba.analyse.textrank(text, topK=5, withWeight=True)
print("Top keywords using TextRank:\n", keywords)
三、使用分词工具
分词是文本处理中的基础步骤,通过将文本切分成一个个的词语,可以更好地进行后续的文本分析。在Python中,有许多分词工具可供使用,如Jieba、NLTK、SpaCy等。
3.1、使用Jieba分词
3.1.1、导入必要的库
import jieba
3.1.2、准备文本数据
text = "Python is a high-level programming language. Machine learning and data science are applications of Python."
3.1.3、进行分词
words = jieba.cut(text)
print("Words using Jieba:\n", "/".join(words))
3.2、使用NLTK分词
3.2.1、导入必要的库
import nltk
nltk.download('punkt')
3.2.2、准备文本数据
text = "Python is a high-level programming language. Machine learning and data science are applications of Python."
3.2.3、进行分词
words = nltk.word_tokenize(text)
print("Words using NLTK:\n", words)
四、使用主题模型
主题模型是一种无监督的机器学习技术,用于从大量文档中发现潜在的主题。常见的主题模型有LDA(Latent Dirichlet Allocation)等。
4.1、导入必要的库
from sklearn.decomposition import LatentDirichletAllocation
from sklearn.feature_extraction.text import CountVectorizer
4.2、准备文本数据
documents = [
"Python is a high-level programming language.",
"Machine learning and data science are applications of Python.",
"Python is popular for web development.",
"Data analysis and machine learning are key applications of Python."
]
4.3、转换文本数据
vectorizer = CountVectorizer()
data_vectorized = vectorizer.fit_transform(documents)
4.4、训练LDA模型
lda_model = LatentDirichletAllocation(n_components=2, random_state=42)
lda_model.fit(data_vectorized)
4.5、显示主题关键词
def print_top_words(model, feature_names, n_top_words):
for topic_idx, topic in enumerate(model.components_):
print("Topic #%d:" % topic_idx)
print(" ".join([feature_names[i] for i in topic.argsort()[:-n_top_words - 1:-1]]))
print()
tf_feature_names = vectorizer.get_feature_names_out()
print_top_words(lda_model, tf_feature_names, 5)
五、使用机器学习
机器学习方法可以通过训练模型来自动抽取文本中的关键字。常见的机器学习方法包括监督学习和无监督学习等。
5.1、导入必要的库
from sklearn.feature_extraction.text import TfidfVectorizer
from sklearn.cluster import KMeans
5.2、准备文本数据
documents = [
"Python is a high-level programming language.",
"Machine learning and data science are applications of Python.",
"Python is popular for web development.",
"Data analysis and machine learning are key applications of Python."
]
5.3、转换文本数据
vectorizer = TfidfVectorizer()
X = vectorizer.fit_transform(documents)
5.4、训练KMeans模型
kmeans = KMeans(n_clusters=2, random_state=42)
kmeans.fit(X)
5.5、显示聚类结果
order_centroids = kmeans.cluster_centers_.argsort()[:, ::-1]
terms = vectorizer.get_feature_names_out()
for i in range(2):
print("Cluster %d:" % i),
for ind in order_centroids[i, :5]:
print(' %s' % terms[ind])
通过上述方法,可以有效地抽取文本中的关键字。不同的方法有各自的优缺点,具体选择哪种方法取决于具体的应用场景和数据特点。无论是使用TF-IDF算法、TextRank算法、分词工具、主题模型还是机器学习方法,都需要根据实际情况进行适当的调整和优化。
相关问答FAQs:
如何选择合适的Python库来进行关键字抽取?
在Python中,有多种库可以用来进行关键字抽取,如NLTK、spaCy和Gensim等。选择合适的库主要取决于你的需求。例如,NLTK适合基础的自然语言处理任务,spaCy则更适合处理大型文本数据并具有更高的性能,而Gensim在主题建模和词向量方面表现突出。了解各个库的特性和应用场景,可以帮助你做出更明智的选择。
关键字抽取的常用算法有哪些?
在进行关键字抽取时,可以使用多种算法,其中包括TF-IDF、TextRank和LDA等。TF-IDF是一种常用的统计方法,可以衡量一个词在文档中的重要性;TextRank是一种基于图的算法,适合提取文本中的关键词和短语;而LDA则是一种主题模型,可以帮助识别文档中的主题及其关键词。根据具体需求选择合适的算法可以提高抽取的准确性和效率。
关键字抽取的结果如何进行评估?
评估关键字抽取的效果可以通过多种方法进行,包括人工评审和自动化评估指标。人工评审可以通过专业人员对抽取结果的相关性和准确性进行打分,而自动化评估则可以使用Precision、Recall和F1 Score等指标来量化抽取效果。此外,结合领域知识和用户反馈也能有效提升评估的全面性和准确性。
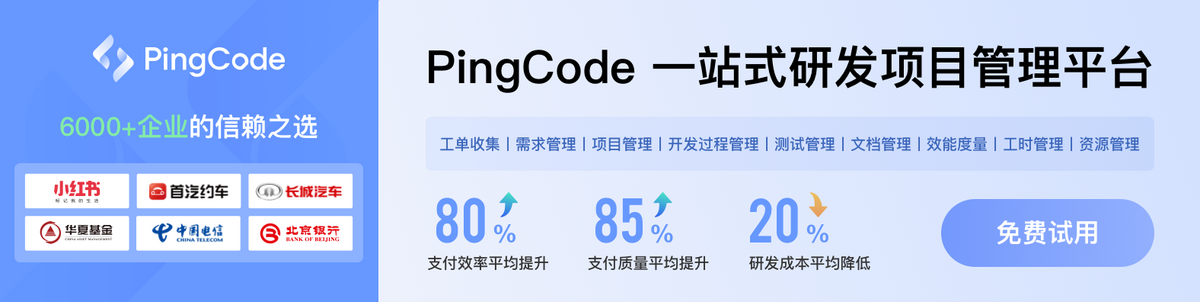