在Python中统计数字的方法有很多种,你可以使用内置函数、列表解析、循环、字典、Counter类等方式来统计数字。其中,使用Counter类是最简单和高效的方法之一。详细的方法如下:
使用Counter类统计数字:Python的collections模块提供了一个名为Counter的类,它是一个方便的工具,用于统计可迭代对象中的元素个数。使用Counter类不仅可以快速统计数字,还可以统计其他类型的元素,例如字符串、元组等。Counter类可以自动将元素作为键,并将其出现的次数作为值存储在字典中。例如,给定一个列表,可以使用Counter类来统计列表中每个数字出现的次数。
from collections import Counter
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
counter = Counter(numbers)
print(counter)
这段代码将输出Counter({4: 4, 3: 3, 2: 2, 1: 1})
,表示数字4出现了4次,数字3出现了3次,数字2出现了2次,数字1出现了1次。下面我们将详细介绍各种方法来统计数字。
一、使用内置函数统计数字
1. 使用count()方法
对于列表或字符串等可迭代对象,可以使用其自带的count()方法来统计某个特定数字出现的次数。
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
count_2 = numbers.count(2)
print(f"Number 2 appears {count_2} times.")
2. 使用sum()方法结合生成器表达式
你也可以使用sum()方法结合生成器表达式来统计数字出现的次数。
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
count_2 = sum(1 for number in numbers if number == 2)
print(f"Number 2 appears {count_2} times.")
二、使用字典统计数字
字典是Python中非常灵活的数据结构,用于存储键值对。可以使用字典来统计数字出现的次数。
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
number_count = {}
for number in numbers:
if number in number_count:
number_count[number] += 1
else:
number_count[number] = 1
print(number_count)
这段代码将输出{1: 1, 2: 2, 3: 3, 4: 4}
。
使用defaultdict简化代码
collections模块中的defaultdict可以简化上述代码。
from collections import defaultdict
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
number_count = defaultdict(int)
for number in numbers:
number_count[number] += 1
print(number_count)
三、使用Counter类统计数字
1. 统计列表中的数字
Counter类是统计数字最方便的方法。
from collections import Counter
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
counter = Counter(numbers)
print(counter)
2. 统计字符串中的字符
Counter类不仅可以统计数字,还可以统计字符串中的字符。
from collections import Counter
text = "hello world"
counter = Counter(text)
print(counter)
这段代码将输出Counter({'l': 3, 'o': 2, 'h': 1, 'e': 1, ' ': 1, 'w': 1, 'r': 1, 'd': 1})
。
四、使用循环统计数字
1. 使用for循环
你可以使用for循环来统计数字出现的次数。
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
count_2 = 0
for number in numbers:
if number == 2:
count_2 += 1
print(f"Number 2 appears {count_2} times.")
2. 使用while循环
也可以使用while循环来统计数字。
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
count_2 = 0
i = 0
while i < len(numbers):
if numbers[i] == 2:
count_2 += 1
i += 1
print(f"Number 2 appears {count_2} times.")
五、统计二维列表中的数字
对于二维列表(列表的列表),你可以使用嵌套循环来统计数字。
matrix = [
[1, 2, 2],
[3, 3, 3],
[4, 4, 4, 4]
]
number_count = {}
for row in matrix:
for number in row:
if number in number_count:
number_count[number] += 1
else:
number_count[number] = 1
print(number_count)
六、统计数字出现的位置
除了统计数字出现的次数,有时候你还需要统计数字出现的位置。
1. 使用列表解析统计位置
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
positions = [i for i, number in enumerate(numbers) if number == 2]
print(positions)
2. 使用循环统计位置
numbers = [1, 2, 2, 3, 3, 3, 4, 4, 4, 4]
positions = []
for i, number in enumerate(numbers):
if number == 2:
positions.append(i)
print(positions)
七、统计数组中的数字
对于使用Numpy的数组,可以使用Numpy的内置方法来统计数字。
import numpy as np
array = np.array([1, 2, 2, 3, 3, 3, 4, 4, 4, 4])
count_2 = np.sum(array == 2)
print(f"Number 2 appears {count_2} times.")
八、统计Pandas数据框中的数字
对于使用Pandas的数据框,可以使用Pandas的内置方法来统计数字。
import pandas as pd
df = pd.DataFrame({
'A': [1, 2, 2, 3, 3],
'B': [3, 3, 4, 4, 4]
})
count_2 = (df == 2).sum().sum()
print(f"Number 2 appears {count_2} times.")
九、总结
Python提供了多种统计数字的方法,包括使用内置函数、字典、Counter类、循环、列表解析、Numpy数组和Pandas数据框等。不同的方法适用于不同的场景和需求,选择合适的方法可以提高代码的可读性和效率。
在实际应用中,Counter类是最简洁和高效的方法之一,特别是在处理大量数据时。使用字典和defaultdict可以灵活地处理更复杂的统计需求。循环和列表解析提供了更多的控制和灵活性,适用于需要自定义统计逻辑的场景。Numpy和Pandas提供了高效的数组和数据框操作方法,适用于科学计算和数据分析领域。
总之,根据具体需求选择合适的方法,可以有效地统计数字并提高代码的效率和可读性。
相关问答FAQs:
如何在Python中统计列表中数字的出现频率?
在Python中,可以使用collections
模块中的Counter
类来统计列表中各个数字的出现频率。首先,将数字放入一个列表中,然后通过Counter
类生成一个字典,其中键为数字,值为出现的次数。例如:
from collections import Counter
numbers = [1, 2, 2, 3, 4, 4, 4, 5]
frequency = Counter(numbers)
print(frequency) # 输出: Counter({4: 3, 2: 2, 1: 1, 3: 1, 5: 1})
如何使用Python对文件中的数字进行统计?
如果你想从文件中读取数字并进行统计,可以先使用open()
函数读取文件内容,然后将其中的数字提取出来。接着,使用Counter
类进行统计。例如:
from collections import Counter
with open('numbers.txt', 'r') as file:
numbers = [int(num) for num in file.read().split()]
frequency = Counter(numbers)
print(frequency)
有哪些库可以帮助我在Python中统计数字?
除了collections
模块的Counter
类外,pandas
库也是一个强大的工具,可以轻松处理和统计数字。使用pandas
的value_counts()
方法,可以快速获取数字出现的频率。例如:
import pandas as pd
numbers = [1, 2, 2, 3, 4, 4, 4, 5]
series = pd.Series(numbers)
frequency = series.value_counts()
print(frequency)
这些方法都能有效地帮助你在Python中统计数字的出现次数。
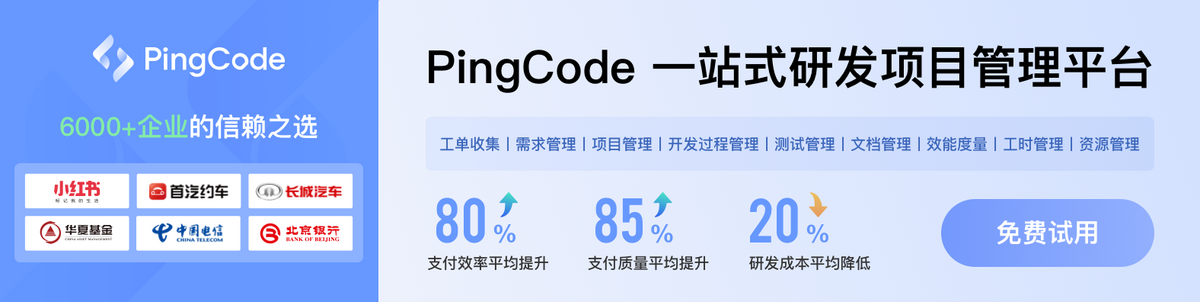