Python可以通过调用操作系统的命令行工具、使用第三方库如pyppeteer
、使用win32ras
模块自动连接宽带拨号。 这些方法各有优劣,具体选择可以根据实际需求和环境来确定。下面详细介绍其中一种方法,即使用win32ras
模块来进行宽带拨号。
win32ras
是Windows操作系统的一个API,用于管理远程访问服务(RAS)连接。Python可以通过pywin32
库中的win32ras
模块调用这些API,实现自动拨号连接。
一、安装和配置环境
要使用win32ras
模块,首先需要安装pywin32
库。可以通过以下命令安装:
pip install pywin32
安装完成后,您需要创建一个拨号连接的配置文件。这个配置文件包含了拨号连接所需的所有信息,如宽带连接的名称、用户名和密码等。
二、创建拨号连接
-
创建宽带连接
首先,在Windows操作系统中创建一个宽带连接。具体步骤如下:
- 打开“控制面板”
- 选择“网络和共享中心”
- 选择“设置新的连接或网络”
- 选择“连接到Internet”
- 选择“宽带(PPPoE)”
- 输入ISP提供的用户名和密码,完成连接设置。
-
保存拨号配置
保存该连接的配置文件,以便于使用Python脚本自动调用。通常情况下,配置文件会保存在系统的网络连接设置中。
三、使用win32ras
模块进行拨号
以下是一个示例脚本,展示了如何使用win32ras
模块来自动进行宽带拨号连接:
import win32ras
import time
def connect(name, username, password):
# 获取所有连接条目
entries = win32ras.EnumEntries(None, None)
# 查找指定的宽带连接
entry = None
for e in entries:
if e[0] == name:
entry = e
break
if not entry:
raise Exception(f"Dial-up connection '{name}' not found")
# 创建拨号连接参数
dial_params = (name, '', username, password, '', '')
# 进行拨号连接
handle, result = win32ras.Dial(None, None, dial_params, None)
if result != 0:
raise Exception(f"Failed to connect: {result}")
print("Successfully connected!")
return handle
def disconnect(handle):
win32ras.HangUp(handle)
print("Disconnected!")
if __name__ == "__main__":
connection_name = "Your Connection Name"
username = "Your ISP Username"
password = "Your ISP Password"
try:
handle = connect(connection_name, username, password)
# 保持连接一段时间
time.sleep(60)
finally:
disconnect(handle)
四、注意事项
-
权限问题
在执行拨号连接操作时,确保脚本具有足够的权限。通常情况下,需要以管理员权限运行Python脚本。
-
错误处理
在实际使用中,可能会遇到各种错误情况,例如网络不可用、用户名或密码错误等。因此,建议在脚本中添加更多的错误处理逻辑,以提高脚本的健壮性。
-
日志记录
为了更好地调试和监控拨号连接过程,建议在脚本中添加日志记录功能。可以使用Python的
logging
模块记录拨号连接的过程和结果。
import logging
配置日志记录
logging.basicConfig(level=logging.INFO, format='%(asctime)s - %(levelname)s - %(message)s')
def connect(name, username, password):
# 获取所有连接条目
entries = win32ras.EnumEntries(None, None)
# 查找指定的宽带连接
entry = None
for e in entries:
if e[0] == name:
entry = e
break
if not entry:
logging.error(f"Dial-up connection '{name}' not found")
raise Exception(f"Dial-up connection '{name}' not found")
# 创建拨号连接参数
dial_params = (name, '', username, password, '', '')
# 进行拨号连接
handle, result = win32ras.Dial(None, None, dial_params, None)
if result != 0:
logging.error(f"Failed to connect: {result}")
raise Exception(f"Failed to connect: {result}")
logging.info("Successfully connected!")
return handle
def disconnect(handle):
win32ras.HangUp(handle)
logging.info("Disconnected!")
if __name__ == "__main__":
connection_name = "Your Connection Name"
username = "Your ISP Username"
password = "Your ISP Password"
try:
handle = connect(connection_name, username, password)
# 保持连接一段时间
time.sleep(60)
finally:
disconnect(handle)
通过添加日志记录,可以更方便地监控拨号连接过程,及时发现和解决问题。
五、扩展应用
-
定时拨号
可以结合Python的定时任务库(如
schedule
或APScheduler
)实现定时拨号连接。例如,可以在每天的特定时间自动拨号连接:
import schedule
import time
def job():
logging.info("Starting dial-up connection job")
handle = None
try:
handle = connect(connection_name, username, password)
time.sleep(60)
finally:
if handle:
disconnect(handle)
schedule.every().day.at("08:00").do(job)
while True:
schedule.run_pending()
time.sleep(1)
-
检测网络状态
可以结合网络状态检测工具(如
ping
命令)实现自动重连功能。当检测到网络断开时,自动尝试重新拨号连接:
import os
def is_connected():
response = os.system("ping -c 1 google.com")
return response == 0
while True:
if not is_connected():
logging.warning("Network disconnected, attempting to reconnect")
handle = None
try:
handle = connect(connection_name, username, password)
time.sleep(60)
finally:
if handle:
disconnect(handle)
time.sleep(10)
通过这种方式,可以实现更加智能的拨号连接管理,确保网络连接的稳定性。
六、总结
本文介绍了如何使用Python的win32ras
模块自动进行宽带拨号连接。通过合理配置环境、创建拨号连接、编写拨号脚本以及添加日志记录和错误处理,可以实现自动化的宽带拨号连接。进一步,可以结合定时任务和网络状态检测工具,实现更加智能的拨号连接管理。
在实际应用中,建议根据具体需求和环境选择合适的方法,并进行充分的测试和调试,以确保脚本的稳定性和可靠性。
相关问答FAQs:
如何使用Python编写自动拨号脚本?
要实现自动连接宽带拨号,您可以使用Python的subprocess
模块来调用系统命令。通常,Windows系统使用rasdial
命令来连接拨号网络。您可以创建一个Python脚本,通过subprocess.run()
函数执行相应的命令,传入用户名和密码等参数,从而实现自动连接。
在Linux系统中,如何使用Python进行宽带拨号?
在Linux系统中,可以使用nmcli
命令来管理网络连接。编写Python脚本时,同样可以利用subprocess
模块来调用nmcli
命令,连接特定的宽带拨号网络。确保您的网络配置已存在,并通过脚本输入相应的连接名称来实现自动拨号。
Python自动拨号脚本需要哪些权限?
运行自动拨号脚本时,可能需要管理员权限,特别是在Windows系统中。确保您的脚本拥有相应的权限来执行网络连接命令,以防止因权限不足而导致拨号失败。可以通过右键点击Python脚本并选择“以管理员身份运行”来实现。
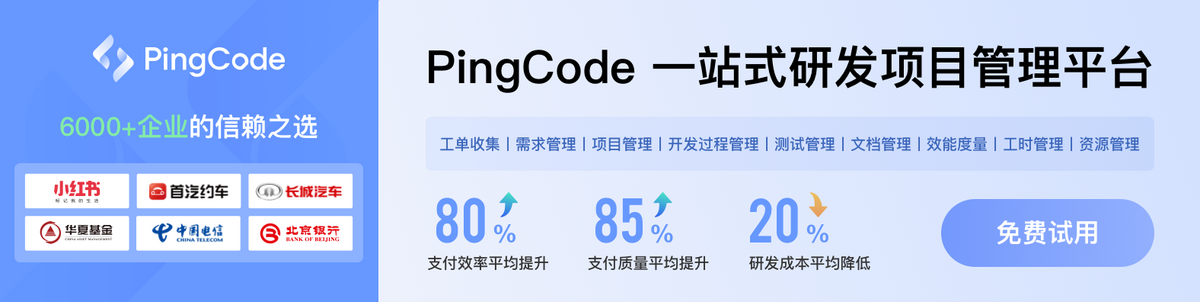