在Python中,表示字符串的方法有很多种,包括使用单引号、双引号、三重引号、转义字符等。单引号、双引号、三重引号、转义字符。其中,单引号和双引号是最常见的表示字符串的方法。单引号和双引号没有实际的区别,可以互换使用。三重引号则通常用于表示多行字符串或包含复杂内容的字符串。转义字符可以用于在字符串中包含一些特殊的字符。
一、单引号和双引号
单引号和双引号是最常见的字符串表示方法。使用单引号或双引号包围文本即可创建字符串。
str1 = 'Hello, World!'
str2 = "Hello, World!"
这两种方式创建的字符串是等价的。可以根据个人习惯或字符串内容选择使用单引号或双引号。
二、三重引号
三重引号用于表示多行字符串或需要包括特殊字符的字符串。可以使用三个单引号或三个双引号。
str3 = '''This is a
multi-line
string.'''
str4 = """This is another
multi-line string."""
三重引号的优势在于可以直接包含换行符和其他特殊字符,而不需要使用转义字符。
三、转义字符
转义字符用于在字符串中包含一些特殊字符,比如换行符(\n)、制表符(\t)、反斜杠(\)等。使用反斜杠(\)表示转义。
str5 = 'It\'s a beautiful day.'
str6 = "He said, \"Hello, World!\""
str7 = "First line.\nSecond line."
str8 = "Column1\tColumn2"
在这些示例中,反斜杠用于转义单引号、双引号、换行符和制表符。
四、字符串常用操作
1、字符串拼接
字符串可以通过 +
运算符进行拼接。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # 输出: Hello World
2、字符串重复
使用 *
运算符可以重复字符串。
str3 = "Ha"
result = str3 * 3
print(result) # 输出: HaHaHa
3、字符串切片
字符串可以通过切片操作获取子字符串。切片的语法为 str[start:end:step]
。
str4 = "Hello, World!"
print(str4[0:5]) # 输出: Hello
print(str4[7:12]) # 输出: World
print(str4[::2]) # 输出: Hlo ol!
4、字符串格式化
Python提供了多种字符串格式化方法,其中最常见的是使用 format()
方法和 f-strings(格式化字符串)。
name = "Alice"
age = 30
使用 format() 方法
str5 = "My name is {}. I am {} years old.".format(name, age)
print(str5) # 输出: My name is Alice. I am 30 years old.
使用 f-strings
str6 = f"My name is {name}. I am {age} years old."
print(str6) # 输出: My name is Alice. I am 30 years old.
5、字符串查找和替换
可以使用 find()
方法查找子字符串,使用 replace()
方法替换子字符串。
str7 = "Hello, World!"
index = str7.find("World")
print(index) # 输出: 7
str8 = str7.replace("World", "Python")
print(str8) # 输出: Hello, Python!
五、字符串内建方法
Python提供了许多字符串内建方法,这些方法可以帮助进行各种字符串操作。
1、大小写转换
字符串可以通过 upper()
、lower()
、capitalize()
等方法进行大小写转换。
str9 = "hello, world!"
upper_str = str9.upper()
print(upper_str) # 输出: HELLO, WORLD!
lower_str = upper_str.lower()
print(lower_str) # 输出: hello, world!
capitalized_str = str9.capitalize()
print(capitalized_str) # 输出: Hello, world!
2、去除空白字符
使用 strip()
、lstrip()
和 rstrip()
方法可以去除字符串两端或指定位置的空白字符。
str10 = " Hello, World! "
stripped_str = str10.strip()
print(stripped_str) # 输出: Hello, World!
left_stripped_str = str10.lstrip()
print(left_stripped_str) # 输出: Hello, World!
right_stripped_str = str10.rstrip()
print(right_stripped_str) # 输出: Hello, World!
3、分割和合并字符串
可以使用 split()
方法分割字符串,使用 join()
方法合并字符串。
str11 = "Hello, World!"
split_str = str11.split(", ")
print(split_str) # 输出: ['Hello', 'World!']
joined_str = ", ".join(split_str)
print(joined_str) # 输出: Hello, World!
六、字符串与编码
1、字符串与字节之间的转换
在处理字符串时,有时需要将字符串转换为字节,或者将字节转换为字符串。可以使用 encode()
和 decode()
方法进行转换。
str12 = "Hello, World!"
将字符串编码为字节
byte_str = str12.encode('utf-8')
print(byte_str) # 输出: b'Hello, World!'
将字节解码为字符串
decoded_str = byte_str.decode('utf-8')
print(decoded_str) # 输出: Hello, World!
2、处理不同编码的字符串
在全球化应用中,可能需要处理不同编码的字符串。Python内置支持多种编码格式,可以使用 encode()
和 decode()
方法指定编码格式。
str13 = "你好,世界!"
将字符串编码为字节(UTF-8)
utf8_byte_str = str13.encode('utf-8')
print(utf8_byte_str) # 输出: b'\xe4\xbd\xa0\xe5\xa5\xbd\xef\xbc\x8c\xe4\xb8\x96\xe7\x95\x8c\xef\xbc\x81'
将字节解码为字符串(UTF-8)
utf8_decoded_str = utf8_byte_str.decode('utf-8')
print(utf8_decoded_str) # 输出: 你好,世界!
将字符串编码为字节(GBK)
gbk_byte_str = str13.encode('gbk')
print(gbk_byte_str) # 输出: b'\xc4\xe3\xba\xc3\xa3\xac\xca\xc0\xbd\xe7\xa3\xac'
将字节解码为字符串(GBK)
gbk_decoded_str = gbk_byte_str.decode('gbk')
print(gbk_decoded_str) # 输出: 你好,世界!
七、字符串在数据处理中的应用
1、数据清洗
在数据处理中,字符串操作是数据清洗的重要工具。例如,去除数据中的多余空白字符、替换错误数据、拆分和合并字段等。
raw_data = " Alice, 30, Data Scientist ; Bob, 25, Software Engineer ; Carol, 35, Manager "
去除多余空白字符
clean_data = raw_data.replace(" ", "").strip()
print(clean_data) # 输出: Alice, 30, Data Scientist; Bob, 25, Software Engineer; Carol, 35, Manager
拆分数据
records = clean_data.split(";")
for record in records:
fields = record.split(",")
name = fields[0].strip()
age = fields[1].strip()
profession = fields[2].strip()
print(f"Name: {name}, Age: {age}, Profession: {profession}")
2、数据格式转换
在数据处理中,经常需要将数据从一种格式转换为另一种格式。例如,将CSV格式的数据转换为JSON格式。
import json
csv_data = "name,age,profession\nAlice,30,Data Scientist\nBob,25,Software Engineer\nCarol,35,Manager"
拆分CSV数据
lines = csv_data.split("\n")
header = lines[0].split(",")
data = []
for line in lines[1:]:
fields = line.split(",")
record = {header[i]: fields[i] for i in range(len(header))}
data.append(record)
转换为JSON格式
json_data = json.dumps(data, indent=4)
print(json_data)
八、字符串在自然语言处理中的应用
1、文本预处理
在自然语言处理中,文本预处理是非常重要的一步。包括去除标点符号、大小写转换、去除停用词等。
import string
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize
text = "Hello, world! This is a test sentence."
去除标点符号
text = text.translate(str.maketrans("", "", string.punctuation))
print(text) # 输出: Hello world This is a test sentence
大小写转换
text = text.lower()
print(text) # 输出: hello world this is a test sentence
去除停用词
stop_words = set(stopwords.words("english"))
words = word_tokenize(text)
filtered_words = [word for word in words if word not in stop_words]
print(filtered_words) # 输出: ['hello', 'world', 'test', 'sentence']
2、词频统计
词频统计是自然语言处理中常见的任务,可以帮助理解文本的主题和内容。
from collections import Counter
text = "hello world hello hello test test"
拆分文本
words = text.split()
统计词频
word_counts = Counter(words)
print(word_counts) # 输出: Counter({'hello': 3, 'test': 2, 'world': 1})
九、字符串在网络编程中的应用
1、HTTP请求
在网络编程中,字符串用于构建和解析HTTP请求和响应。例如,使用 requests
库发送HTTP请求。
import requests
url = "https://jsonplaceholder.typicode.com/posts"
response = requests.get(url)
解析响应
if response.status_code == 200:
data = response.json()
print(data)
else:
print(f"Request failed with status code {response.status_code}")
2、解析URL
可以使用 urllib.parse
模块解析和构建URL。
from urllib.parse import urlparse, urlunparse
url = "https://www.example.com/path?query=param#fragment"
parsed_url = urlparse(url)
print(parsed_url.scheme) # 输出: https
print(parsed_url.netloc) # 输出: www.example.com
print(parsed_url.path) # 输出: /path
print(parsed_url.query) # 输出: query=param
print(parsed_url.fragment) # 输出: fragment
构建URL
new_url = urlunparse(parsed_url)
print(new_url) # 输出: https://www.example.com/path?query=param#fragment
十、字符串在文件操作中的应用
1、读取和写入文件
在文件操作中,字符串用于读取和写入文本文件。
# 写入文件
with open("example.txt", "w") as file:
file.write("Hello, World!\nThis is a test file.")
读取文件
with open("example.txt", "r") as file:
content = file.read()
print(content)
2、处理CSV文件
可以使用 csv
模块处理CSV文件。
import csv
写入CSV文件
with open("example.csv", "w", newline="") as file:
writer = csv.writer(file)
writer.writerow(["name", "age", "profession"])
writer.writerow(["Alice", 30, "Data Scientist"])
writer.writerow(["Bob", 25, "Software Engineer"])
writer.writerow(["Carol", 35, "Manager"])
读取CSV文件
with open("example.csv", "r") as file:
reader = csv.reader(file)
for row in reader:
print(row)
十一、字符串在图形用户界面编程中的应用
1、Tkinter中的字符串操作
在使用 Tkinter
库进行图形用户界面编程时,字符串用于显示文本、获取用户输入等。
import tkinter as tk
def on_button_click():
user_input = entry.get()
label.config(text=f"Hello, {user_input}!")
root = tk.Tk()
root.title("String Example")
label = tk.Label(root, text="Enter your name:")
label.pack()
entry = tk.Entry(root)
entry.pack()
button = tk.Button(root, text="Submit", command=on_button_click)
button.pack()
root.mainloop()
在这个示例中,用户输入的字符串被获取并显示在标签中。
十二、字符串在Web开发中的应用
1、模板引擎
在Web开发中,字符串用于模板引擎渲染网页。例如,使用Jinja2模板引擎。
from jinja2 import Template
template_str = """
<!DOCTYPE html>
<html>
<head>
<title>{{ title }}</title>
</head>
<body>
<h1>{{ heading }}</h1>
<p>{{ content }}</p>
</body>
</html>
"""
template = Template(template_str)
rendered_html = template.render(title="Hello, World!", heading="Welcome", content="This is a sample page.")
print(rendered_html)
2、处理表单数据
在Web应用中,处理表单数据时会涉及到字符串操作。例如,使用Flask框架处理表单提交。
from flask import Flask, request
app = Flask(__name__)
@app.route("/", methods=["GET", "POST"])
def index():
if request.method == "POST":
name = request.form["name"]
return f"Hello, {name}!"
return """
<form method="post">
Name: <input type="text" name="name">
<input type="submit">
</form>
"""
if __name__ == "__main__":
app.run()
在这个示例中,表单提交的数据是字符串,并在服务器端进行处理。
十三、总结
在Python中,字符串是一种重要且常用的数据类型,广泛应用于各种编程场景。通过掌握字符串的表示方法和常用操作,可以有效地进行数据处理、自然语言处理、网络编程、文件操作、图形用户界面编程和Web开发等任务。希望本文对你理解和应用字符串有所帮助。
相关问答FAQs:
如何在Python中定义一个字符串?
在Python中,字符串可以通过使用单引号、双引号或三引号来定义。例如,'Hello'
、"World"
和'''这是一个多行字符串'''
都被视为有效字符串。使用单引号和双引号的字符串在功能上是等价的,而三引号通常用于需要换行的长字符串。
Python支持哪些字符串操作?
Python提供了丰富的字符串操作方法,包括拼接、分割、查找、替换等。可以使用+
运算符拼接字符串,使用.split()
方法分割字符串,使用.find()
和.replace()
来查找和替换特定的子字符串。此外,字符串对象还支持切片操作,允许您提取字符串的特定部分。
如何处理Python中的字符串编码问题?
在Python中,字符串默认使用Unicode编码,这使得处理多语言文本变得更加简单。如果需要处理不同编码格式的字符串,可以使用.encode()
和.decode()
方法来进行转换。了解不同编码之间的差异(如UTF-8和ASCII)对于确保程序的兼容性和正确性至关重要。
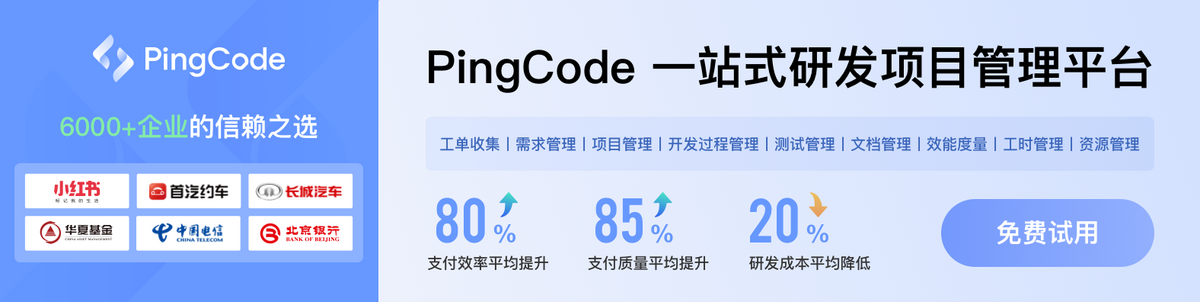