要用Python代码发送图片,可以使用多种方法,下面列举几种常见的方法:使用SMTP发送电子邮件、使用HTTP协议发送图片、使用FTP协议上传图片。其中,使用SMTP发送电子邮件是一种常见且简单的方法,我们将详细介绍如何使用这种方法发送图片。
一、使用SMTP发送电子邮件
SMTP(Simple Mail Transfer Protocol,简单邮件传输协议)是发送电子邮件的标准协议。使用Python的smtplib
库可以轻松实现发送电子邮件的功能,包括发送带有附件的电子邮件。以下是一个详细的示例,展示如何使用Python通过SMTP发送带有图片附件的电子邮件。
1、准备工作
首先,确保已经安装了smtplib
库,这个库是Python的标准库,通常不需要额外安装。如果需要发送带有附件的邮件,还需要使用email
库,它也是Python的标准库。
2、编写代码
下面是一个完整的示例代码,展示如何通过SMTP发送带有图片附件的电子邮件:
import smtplib
from email.mime.multipart import MIMEMultipart
from email.mime.text import MIMEText
from email.mime.base import MIMEBase
from email import encoders
def send_email_with_attachment(subject, body, to_email, from_email, smtp_server, smtp_port, login, password, attachment_file_path):
# 创建一个MIMEMultipart对象
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
# 添加邮件正文
msg.attach(MIMEText(body, 'plain'))
# 打开文件并将其作为附件添加到邮件中
with open(attachment_file_path, 'rb') as attachment:
part = MIMEBase('application', 'octet-stream')
part.set_payload(attachment.read())
encoders.encode_base64(part)
part.add_header('Content-Disposition', f'attachment; filename={attachment_file_path}')
msg.attach(part)
# 连接到SMTP服务器并发送邮件
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls() # 启用TLS
server.login(login, password)
server.send_message(msg)
server.quit()
示例用法
subject = "Test Email with Attachment"
body = "This is a test email with an image attachment."
to_email = "recipient@example.com"
from_email = "sender@example.com"
smtp_server = "smtp.example.com"
smtp_port = 587
login = "your_email@example.com"
password = "your_password"
attachment_file_path = "path/to/your/image.jpg"
send_email_with_attachment(subject, body, to_email, from_email, smtp_server, smtp_port, login, password, attachment_file_path)
3、解释代码
MIMEMultipart
:用于创建包含多个部分的电子邮件,如文本和附件。MIMEText
:用于创建电子邮件正文。MIMEBase
:用于创建电子邮件附件。encoders.encode_base64
:用于对附件进行Base64编码。smtplib.SMTP
:用于连接到SMTP服务器。server.starttls
:启用TLS(传输层安全性),确保连接是加密的。server.login
:登录到SMTP服务器。server.send_message
:发送电子邮件。
二、使用HTTP协议发送图片
HTTP协议是互联网上传输数据的基础协议,可以使用HTTP POST请求将图片上传到服务器。这种方法通常用于与Web服务器交互,例如上传图片到一个RESTful API。
1、准备工作
首先,确保已经安装了requests
库,这是一个流行的HTTP库,可以简化HTTP请求的发送。
pip install requests
2、编写代码
下面是一个示例代码,展示如何使用HTTP POST请求将图片上传到服务器:
import requests
def upload_image(url, image_path):
with open(image_path, 'rb') as image_file:
files = {'file': image_file}
response = requests.post(url, files=files)
return response
示例用法
url = "http://example.com/upload"
image_path = "path/to/your/image.jpg"
response = upload_image(url, image_path)
print(response.status_code)
print(response.text)
3、解释代码
requests.post
:用于发送HTTP POST请求。files
:包含要上传的文件,键名通常是服务器端期望的字段名。response.status_code
:返回HTTP响应状态码。response.text
:返回HTTP响应正文。
三、使用FTP协议上传图片
FTP(File Transfer Protocol,文件传输协议)是一种用于在网络上进行文件传输的标准协议。使用Python的ftplib
库可以轻松实现通过FTP上传图片的功能。
1、准备工作
首先,确保已经安装了ftplib
库,这个库是Python的标准库,通常不需要额外安装。
2、编写代码
下面是一个示例代码,展示如何通过FTP上传图片:
import ftplib
def upload_image_ftp(server, port, username, password, image_path, remote_path):
# 连接到FTP服务器
ftp = ftplib.FTP()
ftp.connect(server, port)
ftp.login(username, password)
# 打开要上传的文件
with open(image_path, 'rb') as image_file:
# 使用STOR命令上传文件
ftp.storbinary(f'STOR {remote_path}', image_file)
# 关闭FTP连接
ftp.quit()
示例用法
server = "ftp.example.com"
port = 21
username = "your_username"
password = "your_password"
image_path = "path/to/your/image.jpg"
remote_path = "remote/directory/image.jpg"
upload_image_ftp(server, port, username, password, image_path, remote_path)
3、解释代码
ftplib.FTP
:用于创建FTP连接。ftp.connect
:连接到FTP服务器。ftp.login
:登录到FTP服务器。ftp.storbinary
:上传二进制文件。ftp.quit
:关闭FTP连接。
总结
通过以上三种方法,我们可以使用Python代码发送图片。使用SMTP发送电子邮件、使用HTTP协议发送图片、使用FTP协议上传图片,这些方法各有优缺点,具体选择哪种方法取决于实际需求和应用场景。
使用SMTP发送电子邮件:适用于发送带有图片附件的电子邮件,简单易用,但需要配置邮件服务器。
使用HTTP协议发送图片:适用于与Web服务器交互,上传图片到RESTful API,灵活性高,但需要服务器端的支持。
使用FTP协议上传图片:适用于通过FTP协议上传文件,适用于需要批量上传文件的场景,但需要FTP服务器的配置。
无论选择哪种方法,都可以通过Python代码实现图片的发送和上传功能。根据具体需求和应用场景,选择最合适的方法进行实现。
相关问答FAQs:
如何用Python发送图片到社交媒体平台?
使用Python发送图片到社交媒体平台通常需要借助API。以Twitter为例,您可以使用tweepy
库来上传图片。首先,安装tweepy
,然后使用Twitter API的认证信息来创建一个API对象,接着使用api.update_with_media()
方法上传图片。确保遵循社交媒体平台的API文档,以便正确处理身份验证和上传过程。
Python中有哪些库可以用来发送图片?
在Python中,有多个库可以实现发送图片的功能。requests
库是一个流行的选择,适用于HTTP请求,可以轻松上传文件。smtplib
库可用于通过电子邮件发送图片,而pygame
和PIL
(Pillow)等库则可以用于图像处理和展示。选择合适的库取决于您的具体需求和使用场景。
在发送图片时需要注意哪些事项?
发送图片时需要注意文件格式和大小限制。确保图片格式(如JPEG、PNG等)符合接收方的要求,同时注意文件大小,避免超出上传限制。此外,处理图片时要考虑到隐私和版权问题,确保您有权发送该图片,并遵循相关法律法规。在编写代码时,处理异常情况也是很重要的,以确保程序的健壮性。
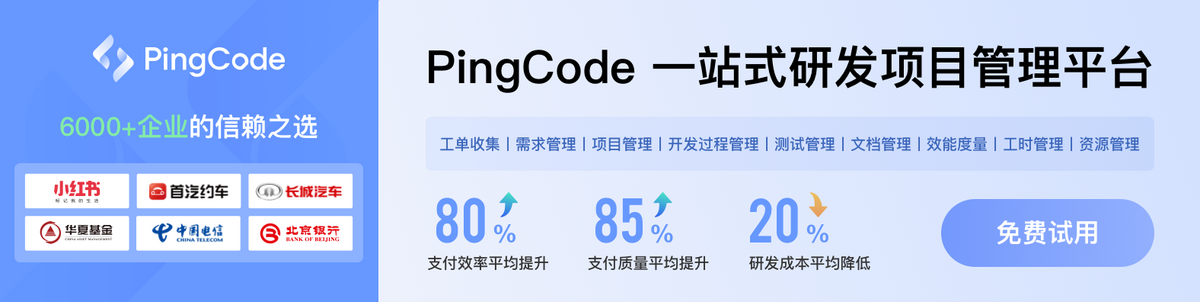