在VB中使用Python可以通过调用Python脚本、使用COM接口、使用IronPython、嵌入Python解释器等方法。 其中,使用COM接口是一个较为直接且常用的方法。通过创建一个COM对象,你可以在VB中调用Python函数并获取其返回值。以下是详细的描述和实现步骤。
一、调用Python脚本
-
编写Python脚本:首先,在Python中编写你需要执行的脚本,并保存为一个.py文件。例如,创建一个简单的Python脚本
example.py
,内容如下:def add(a, b):
return a + b
if __name__ == "__main__":
import sys
a = int(sys.argv[1])
b = int(sys.argv[2])
print(add(a, b))
-
在VB中调用Python脚本:在VB中,通过Shell命令调用Python脚本,并捕获其输出。例如:
Dim pythonScript As String
pythonScript = "C:\path\to\example.py"
Dim a As Integer
Dim b As Integer
a = 10
b = 20
Dim command As String
command = "python " & pythonScript & " " & a & " " & b
Dim shell As Object
Set shell = CreateObject("WScript.Shell")
Dim exec As Object
Set exec = shell.Exec(command)
Dim output As String
output = exec.StdOut.ReadAll()
MsgBox "The result is: " & output
二、使用COM接口
-
安装Python for Windows Extensions:确保安装了
pywin32
库,这是Python的Windows扩展库,提供了对COM接口的支持。可以通过以下命令安装:pip install pywin32
-
编写Python脚本:编写一个Python脚本,注册一个COM对象。例如,创建
com_example.py
,内容如下:import win32com.server.register
class PythonCOMServer:
_reg_clsid_ = "{00000000-0000-0000-0000-000000000000}"
_reg_desc_ = "Python COM Server"
_reg_progid_ = "PythonCOMServer.Application"
_public_methods_ = ['Add']
def Add(self, a, b):
return a + b
if __name__ == "__main__":
win32com.server.register.UseCommandLine(PythonCOMServer)
运行此脚本以注册COM服务器:
python com_example.py --register
-
在VB中使用COM对象:在VB中创建COM对象并调用其方法。例如:
Dim pythonCom As Object
Set pythonCom = CreateObject("PythonCOMServer.Application")
Dim result As Integer
result = pythonCom.Add(10, 20)
MsgBox "The result is: " & result
三、使用IronPython
-
安装IronPython:首先,需要安装IronPython,这是一个运行在.NET框架上的Python实现。可以从IronPython官网下载并安装。
-
编写Python脚本:编写一个Python脚本,并保存为
.py
文件。例如,创建example.py
,内容如下:def add(a, b):
return a + b
-
在VB中调用IronPython:在VB中使用IronPython的
Microsoft.Scripting.Hosting
库来执行Python脚本。例如:Imports Microsoft.Scripting.Hosting
Imports IronPython.Hosting
Module Module1
Sub Main()
Dim pyEngine As ScriptEngine
Dim pyScope As ScriptScope
pyEngine = Python.CreateEngine()
pyScope = pyEngine.CreateScope()
pyEngine.ExecuteFile("C:\path\to\example.py", pyScope)
Dim addFunction As Object
addFunction = pyScope.GetVariable("add")
Dim result As Integer
result = addFunction(10, 20)
MsgBox("The result is: " & result)
End Sub
End Module
四、嵌入Python解释器
-
安装Python:确保安装了Python,并且Python的路径已添加到系统的环境变量中。
-
使用Python C API:可以通过Python的C API在VB中嵌入Python解释器。这种方法较为复杂,需要使用C/C++编写一个动态链接库(DLL),并在VB中调用该DLL。以下是一个简化的示例。
2.1 编写C/C++代码:编写一个C/C++代码,嵌入Python解释器。例如,创建
embed_python.cpp
:#include <Python.h>
extern "C" __declspec(dllexport) int Add(int a, int b) {
Py_Initialize();
PyObject* pName, * pModule, * pFunc;
PyObject* pArgs, * pValue;
int result = 0;
pName = PyUnicode_DecodeFSDefault("example");
pModule = PyImport_Import(pName);
Py_DECREF(pName);
if (pModule != NULL) {
pFunc = PyObject_GetAttrString(pModule, "add");
if (PyCallable_Check(pFunc)) {
pArgs = PyTuple_Pack(2, PyLong_FromLong(a), PyLong_FromLong(b));
pValue = PyObject_CallObject(pFunc, pArgs);
Py_DECREF(pArgs);
if (pValue != NULL) {
result = PyLong_AsLong(pValue);
Py_DECREF(pValue);
}
}
Py_XDECREF(pFunc);
Py_DECREF(pModule);
}
Py_Finalize();
return result;
}
2.2 编译为DLL:将上述代码编译为DLL。例如,使用Visual Studio创建一个DLL项目,并将代码添加到项目中。
2.3 在VB中调用DLL:在VB中声明并调用DLL中的函数。例如:
Declare Function Add Lib "C:\path\to\embed_python.dll" (ByVal a As Integer, ByVal b As Integer) As Integer
Sub Main()
Dim result As Integer
result = Add(10, 20)
MsgBox("The result is: " & result)
End Sub
通过以上方法,你可以在VB中使用Python,实现跨语言调用和数据处理。每种方法都有其优缺点和适用场景,可以根据实际需求选择合适的方法。
相关问答FAQs:
如何在VB中调用Python脚本?
在VB中调用Python脚本,可以使用Process类来启动一个新的进程,运行Python解释器并传入脚本路径。首先,需要确保Python已安装并且在系统的环境变量中设置了路径。然后,可以通过以下代码示例来实现:
Dim psi As New ProcessStartInfo("python.exe", "C:\path\to\your_script.py")
psi.UseShellExecute = False
psi.RedirectStandardOutput = True
Dim process As Process = Process.Start(psi)
Dim output As String = process.StandardOutput.ReadToEnd()
process.WaitForExit()
Console.WriteLine(output)
这样,就能在VB中执行Python脚本并获取其输出。
在VB中如何处理Python返回的结果?
处理Python脚本返回的结果可以通过标准输出进行捕捉。上述代码示例中使用RedirectStandardOutput
属性来获取Python脚本的输出。可以将输出存储在变量中,进一步进行解析或显示。根据需要,可以使用字符串分割或正则表达式来提取信息。
如何在VB项目中管理Python库和依赖?
在VB项目中使用Python时,确保所需的Python库和依赖已经安装。可以使用Python的包管理工具pip来安装所需的库。例如,在命令提示符中输入pip install package_name
来安装库。在VB中调用Python脚本时,确保这些库在Python环境中可用。为了避免版本冲突,可以考虑使用虚拟环境来隔离项目依赖。
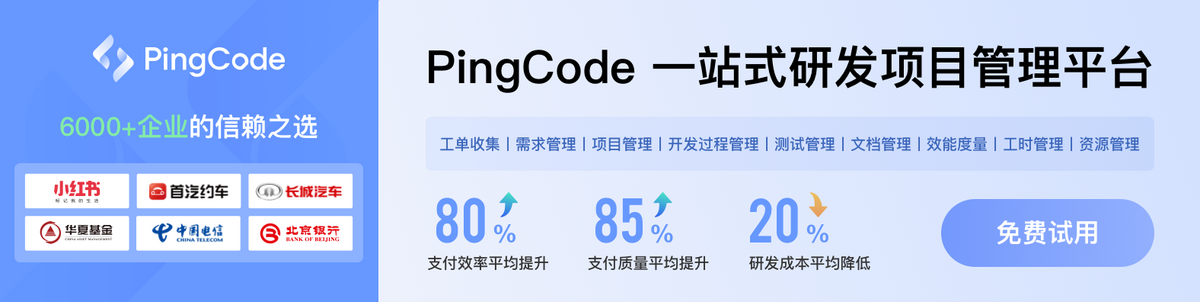