使用Python生成JSON文件非常简单,可以通过Python内置的json
模块来完成。、首先,要将数据结构(如字典或列表)转换为JSON格式的字符串,然后将其写入文件中。、此外,还可以使用json.dump()
方法将数据直接写入文件,而不需要先转换为字符串。
让我们详细探讨如何使用Python生成JSON文件。
一、导入json模块
在开始之前,需要先导入Python的json
模块,这是一个内置模块,因此无需额外安装。
import json
二、准备数据
在生成JSON文件之前,需要准备好要写入的数据。通常,这些数据是以字典(dict)或列表(list)的形式存在。
data = {
"name": "John Doe",
"age": 30,
"is_student": False,
"courses": ["Math", "Science", "Literature"],
"address": {
"street": "123 Main St",
"city": "Anytown",
"country": "USA"
}
}
三、将数据转换为JSON字符串
使用json.dumps()
函数可以将Python数据结构转换为JSON字符串。
json_string = json.dumps(data, indent=4)
print(json_string)
这里的indent=4
参数用于格式化输出,使JSON字符串更易读。
四、将JSON字符串写入文件
将JSON字符串写入文件可以使用Python的标准文件操作方法。
with open('data.json', 'w') as json_file:
json_file.write(json_string)
五、直接将数据写入JSON文件
使用json.dump()
函数可以直接将Python数据结构写入文件,而不需要先转换为字符串。
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
六、处理复杂数据类型
在处理复杂数据类型时,如日期和时间,需要进行序列化处理。可以通过自定义序列化函数来实现。
from datetime import datetime
data_with_date = {
"name": "John Doe",
"timestamp": datetime.now()
}
def datetime_serializer(obj):
if isinstance(obj, datetime):
return obj.isoformat()
raise TypeError("Type not serializable")
json_string_with_date = json.dumps(data_with_date, default=datetime_serializer, indent=4)
print(json_string_with_date)
七、读取JSON文件
生成JSON文件后,可以通过json.load()
函数来读取文件中的数据。
with open('data.json', 'r') as json_file:
loaded_data = json.load(json_file)
print(loaded_data)
八、使用try-except处理异常
在处理文件操作时,最好使用try-except
块来捕获潜在的异常。
try:
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
except IOError as e:
print(f"Error writing to file: {e}")
九、使用上下文管理器
使用上下文管理器(with
语句)可以确保文件在操作完成后被正确关闭。
with open('data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
十、优化大数据处理
在处理大型数据集时,可以使用生成器或分块写入文件的方法来优化性能。
def data_generator():
for i in range(1000000):
yield {"index": i, "value": f"Item {i}"}
with open('large_data.json', 'w') as json_file:
json_file.write('[')
for i, item in enumerate(data_generator()):
if i > 0:
json_file.write(',')
json.dump(item, json_file)
json_file.write(']')
十一、使用第三方库
在一些复杂的应用场景中,可以使用第三方库如ujson
或simplejson
来提高性能和功能。
import ujson
with open('data.json', 'w') as json_file:
ujson.dump(data, json_file, indent=4)
十二、应用案例
1、配置文件
JSON文件常用于存储配置文件。以下是一个简单的示例:
config = {
"database": {
"host": "localhost",
"port": 5432,
"username": "user",
"password": "password"
},
"features": {
"enable_feature_x": True,
"max_retries": 5
}
}
with open('config.json', 'w') as config_file:
json.dump(config, config_file, indent=4)
2、数据传输
JSON文件广泛用于数据传输,特别是在Web应用程序中。以下是一个示例:
import requests
url = "https://api.example.com/data"
response = requests.get(url)
data = response.json()
with open('api_data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
3、数据备份
可以使用JSON文件进行数据备份和恢复。以下是一个示例:
import sqlite3
conn = sqlite3.connect('example.db')
cursor = conn.cursor()
cursor.execute("SELECT * FROM users")
users = cursor.fetchall()
users_data = [{"id": user[0], "name": user[1], "email": user[2]} for user in users]
with open('backup.json', 'w') as backup_file:
json.dump(users_data, backup_file, indent=4)
恢复数据
with open('backup.json', 'r') as backup_file:
restored_data = json.load(backup_file)
for user in restored_data:
cursor.execute("INSERT INTO users (id, name, email) VALUES (?, ?, ?)",
(user['id'], user['name'], user['email']))
conn.commit()
conn.close()
十三、注意事项
1、编码问题
确保在处理文件时使用合适的编码,特别是在处理非ASCII字符时。
with open('data.json', 'w', encoding='utf-8') as json_file:
json.dump(data, json_file, indent=4, ensure_ascii=False)
2、数据验证
在生成JSON文件之前,最好先验证数据的正确性,以避免潜在的错误。
def validate_data(data):
if not isinstance(data, dict):
raise ValueError("Data must be a dictionary")
validate_data(data)
3、版本控制
在一些应用场景中,可能需要对JSON文件进行版本控制,以便在数据格式发生变化时进行兼容性处理。
data = {
"version": 1.0,
"data": {
"name": "John Doe",
"age": 30
}
}
with open('versioned_data.json', 'w') as json_file:
json.dump(data, json_file, indent=4)
十四、结论
生成JSON文件是Python编程中的常见任务,通过使用内置的json
模块,可以轻松实现这一操作。无论是处理简单的数据结构,还是复杂的数据类型,都可以通过适当的编码和处理方法来生成和管理JSON文件。希望通过本文的详细介绍,您能够更好地理解和应用Python生成JSON文件的技巧和方法。
相关问答FAQs:
如何在Python中创建一个新的JSON文件?
在Python中,可以使用内置的json
模块来创建JSON文件。首先,需要准备一个Python字典或列表,然后使用json.dump()
函数将其写入文件中。以下是一个简单的示例:
import json
data = {
"name": "Alice",
"age": 30,
"city": "New York"
}
with open('data.json', 'w') as json_file:
json.dump(data, json_file)
上述代码将创建一个名为data.json
的文件,并将字典data
的内容写入该文件中。
如何读取JSON文件中的数据?
读取JSON文件同样简单,可以使用json.load()
函数。通过打开文件并将其内容加载到Python对象中,用户可以很方便地访问数据。示例如下:
import json
with open('data.json', 'r') as json_file:
data = json.load(json_file)
print(data)
此代码将读取之前创建的data.json
文件,并打印出其内容。
在Python中如何处理嵌套的JSON数据?
处理嵌套的JSON数据同样可以使用json
模块。嵌套的JSON对象通常是以字典或列表的形式存在。以下是一个示例:
import json
nested_data = {
"employees": [
{
"name": "John",
"age": 28
},
{
"name": "Jane",
"age": 32
}
]
}
with open('nested_data.json', 'w') as json_file:
json.dump(nested_data, json_file)
# 读取嵌套数据
with open('nested_data.json', 'r') as json_file:
data = json.load(json_file)
print(data['employees'][0]['name']) # 输出: John
通过这种方式,用户能够轻松地创建和读取嵌套的JSON数据结构。
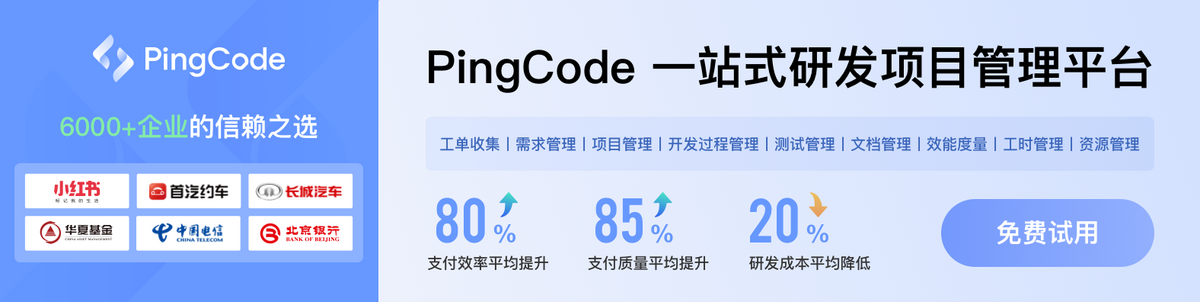