Python爬虫下载文件的方法包括使用requests库、urllib库、以及Scrapy框架等方式。 其中,使用requests库最为简单和常用,因此我们将详细介绍如何使用requests库下载文件。
使用requests库下载文件时,主要步骤包括:构建请求、获取响应、保存文件。通过requests库,我们可以方便地发送HTTP请求并处理响应。此外,requests库还支持流式下载大文件,避免内存占用过大。
一、使用requests库下载文件
1、构建请求并获取响应
首先,我们需要导入requests库,并发送HTTP请求来获取文件的响应。以下是一个基本的例子:
import requests
url = 'https://example.com/file.zip'
response = requests.get(url)
在这段代码中,我们使用requests.get()
方法发送GET请求,并将响应对象存储在response
变量中。
2、检查响应状态码
在处理响应之前,我们需要检查响应的状态码,以确保请求成功。通常,状态码为200表示请求成功:
if response.status_code == 200:
print('Request successful')
else:
print(f'Request failed with status code {response.status_code}')
3、保存文件
如果请求成功,我们可以将响应内容保存为文件。对于小文件,我们可以直接将响应内容写入文件:
with open('file.zip', 'wb') as file:
file.write(response.content)
对于大文件,我们可以使用流式下载,以避免内存占用过大:
import requests
url = 'https://example.com/largefile.zip'
response = requests.get(url, stream=True)
with open('largefile.zip', 'wb') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
在这段代码中,我们使用stream=True
参数启用流式下载,并通过迭代response.iter_content()
方法逐块读取响应内容。
二、使用urllib库下载文件
除了requests库,我们还可以使用Python标准库中的urllib来下载文件。以下是一个使用urllib下载文件的例子:
import urllib.request
url = 'https://example.com/file.zip'
file_path = 'file.zip'
urllib.request.urlretrieve(url, file_path)
urllib.request.urlretrieve()
方法可以直接下载文件并保存到指定路径。
三、使用Scrapy框架下载文件
Scrapy是一个用于爬取网站数据并提取结构化数据的应用框架,它也可以用于下载文件。以下是一个使用Scrapy下载文件的基本例子:
首先,安装Scrapy:
pip install scrapy
然后,创建一个Scrapy项目并生成一个爬虫:
scrapy startproject myproject
cd myproject
scrapy genspider myspider example.com
在生成的爬虫文件中,编写下载文件的代码:
import scrapy
class MySpider(scrapy.Spider):
name = 'myspider'
start_urls = ['https://example.com']
def parse(self, response):
file_url = 'https://example.com/file.zip'
yield scrapy.Request(file_url, callback=self.save_file)
def save_file(self, response):
file_path = 'file.zip'
with open(file_path, 'wb') as file:
file.write(response.body)
运行爬虫:
scrapy crawl myspider
四、处理文件下载中的常见问题
1、重定向问题
有些下载链接可能会遇到重定向问题,可以通过设置allow_redirects
参数来处理:
response = requests.get(url, allow_redirects=True)
2、身份验证问题
有些文件下载可能需要身份验证,可以通过提供用户名和密码来处理:
from requests.auth import HTTPBasicAuth
url = 'https://example.com/file.zip'
response = requests.get(url, auth=HTTPBasicAuth('username', 'password'))
if response.status_code == 200:
with open('file.zip', 'wb') as file:
file.write(response.content)
3、处理Cookies
有些网站需要处理Cookies,可以使用requests.Session
对象来保持会话:
import requests
session = requests.Session()
login_url = 'https://example.com/login'
data = {
'username': 'your_username',
'password': 'your_password'
}
session.post(login_url, data=data)
file_url = 'https://example.com/file.zip'
response = session.get(file_url)
if response.status_code == 200:
with open('file.zip', 'wb') as file:
file.write(response.content)
五、下载文件的高级技巧
1、处理大文件的断点续传
对于大文件下载,断点续传可以提高下载效率并减少资源浪费。可以通过设置HTTP请求头中的Range
字段来实现断点续传:
import os
import requests
url = 'https://example.com/largefile.zip'
file_path = 'largefile.zip'
file_size = os.path.getsize(file_path) if os.path.exists(file_path) else 0
headers = {
'Range': f'bytes={file_size}-'
}
response = requests.get(url, headers=headers, stream=True)
with open(file_path, 'ab') as file:
for chunk in response.iter_content(chunk_size=8192):
file.write(chunk)
2、多线程下载
多线程下载可以加快下载速度,以下是一个使用多线程下载文件的例子:
import threading
import requests
def download_chunk(url, start, end, file_path):
headers = {
'Range': f'bytes={start}-{end}'
}
response = requests.get(url, headers=headers, stream=True)
with open(file_path, 'r+b') as file:
file.seek(start)
file.write(response.content)
def multi_thread_download(url, file_path, num_threads=4):
response = requests.head(url)
file_size = int(response.headers['content-length'])
chunk_size = file_size // num_threads
with open(file_path, 'wb') as file:
file.truncate(file_size)
threads = []
for i in range(num_threads):
start = i * chunk_size
end = (i + 1) * chunk_size - 1 if i != num_threads - 1 else file_size - 1
thread = threading.Thread(target=download_chunk, args=(url, start, end, file_path))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
url = 'https://example.com/largefile.zip'
file_path = 'largefile.zip'
multi_thread_download(url, file_path)
在这段代码中,我们首先获取文件的大小,然后将文件分成若干块,每块由一个线程下载。下载完成后,将所有线程加入主线程,确保文件完整下载。
六、下载文件的安全性
在下载文件时,需要注意安全性问题,以下是一些常见的安全措施:
1、验证文件完整性
下载完成后,可以通过验证文件的哈希值(如MD5、SHA256等)来确保文件完整性:
import hashlib
def calculate_hash(file_path, hash_type='md5'):
hash_func = getattr(hashlib, hash_type)()
with open(file_path, 'rb') as file:
while chunk := file.read(8192):
hash_func.update(chunk)
return hash_func.hexdigest()
file_path = 'file.zip'
expected_hash = 'expected_hash_value'
if calculate_hash(file_path) == expected_hash:
print('File integrity verified')
else:
print('File integrity verification failed')
2、处理SSL证书
在下载文件时,可以通过设置verify
参数来处理SSL证书:
response = requests.get(url, verify=True)
如果使用自签名证书,可以提供证书路径:
response = requests.get(url, verify='/path/to/certfile')
七、总结
Python爬虫下载文件的方法包括使用requests库、urllib库、以及Scrapy框架等方式。requests库是最为简单和常用的工具,适用于大多数文件下载需求。urllib库和Scrapy框架也有各自的优势,适用于特定场景。处理文件下载中的常见问题,如重定向、身份验证和Cookies,可以提高下载的稳定性和成功率。高级技巧如断点续传和多线程下载可以显著提高下载效率。最后,下载文件时需注意安全性,通过验证文件完整性和处理SSL证书来确保文件的安全。
通过掌握这些方法和技巧,可以轻松应对各种文件下载需求,提高爬虫的效率和可靠性。希望这篇文章能对你有所帮助,祝你在Python爬虫的学习和实践中取得更大的进步!
相关问答FAQs:
如何使用Python爬虫下载特定类型的文件?
使用Python爬虫下载特定类型的文件,通常需要使用requests库来获取文件的内容,然后将其保存到本地。可以通过指定文件的URL,获取数据后,再通过二进制模式打开文件并写入内容。例如,对于PDF文件,可以使用以下代码:
import requests
url = 'http://example.com/file.pdf'
response = requests.get(url)
with open('file.pdf', 'wb') as file:
file.write(response.content)
确保在下载文件时处理好异常情况,避免因网络问题导致下载失败。
在下载文件时如何处理重定向和错误?
在下载文件时,可能会遇到重定向或下载错误的情况。使用requests库可以通过设置参数来自动处理重定向,同时可以检查响应状态码以确定下载是否成功。以下是示例代码:
response = requests.get(url, allow_redirects=True)
if response.status_code == 200:
with open('file.pdf', 'wb') as file:
file.write(response.content)
else:
print(f"下载失败,状态码:{response.status_code}")
这种方法能够确保即使在遇到重定向的情况下,文件也能顺利下载。
使用Python爬虫下载文件时如何提高下载效率?
提高下载效率可以通过多线程或异步请求来实现。使用Python的concurrent.futures模块可以轻松实现多线程下载。示例代码如下:
from concurrent.futures import ThreadPoolExecutor
import requests
def download_file(url):
response = requests.get(url)
filename = url.split('/')[-1]
with open(filename, 'wb') as file:
file.write(response.content)
urls = ['http://example.com/file1.pdf', 'http://example.com/file2.pdf']
with ThreadPoolExecutor(max_workers=5) as executor:
executor.map(download_file, urls)
通过这种方式,可以同时下载多个文件,从而显著提高下载速度。
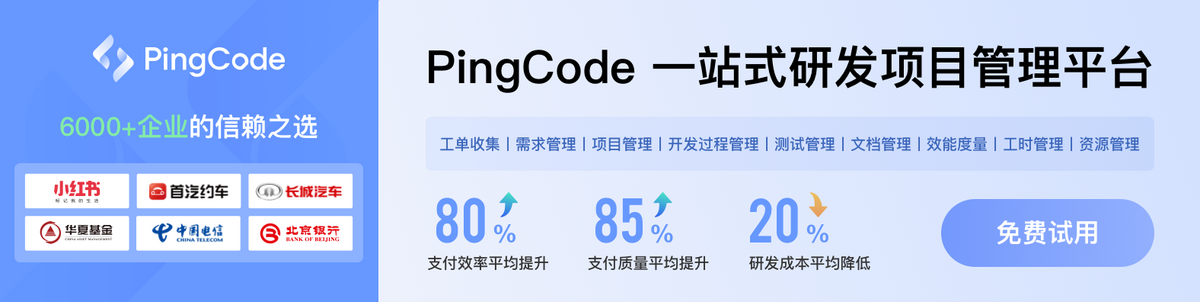