在Python中,可以通过使用多个库和方法来调用桌面的文件,如os、pathlib、shutil等。首先,定位桌面路径非常重要,其次,打开文件或者对文件进行操作是关键步骤。
首先,我们需要获取桌面的路径。可以使用os
模块或者pathlib
模块来获取桌面路径。我们可以使用os.path.expanduser
来获取用户的主目录路径,然后拼接桌面路径。接下来,我们可以使用os
模块中的方法来操作文件,比如打开、读取、写入等。
一、获取桌面路径
使用os
模块获取桌面路径是常见的方法之一。我们可以通过os.path.expanduser
获取用户主目录,然后拼接桌面路径。
import os
def get_desktop_path():
desktop_path = os.path.join(os.path.expanduser("~"), "Desktop")
return desktop_path
desktop_path = get_desktop_path()
print(f"Desktop Path: {desktop_path}")
另一种方法是使用pathlib
模块,它提供了更现代和面向对象的方式来操作文件路径。
from pathlib import Path
def get_desktop_path():
desktop_path = Path.home() / 'Desktop'
return desktop_path
desktop_path = get_desktop_path()
print(f"Desktop Path: {desktop_path}")
二、操作桌面文件
获取了桌面的路径之后,我们可以对桌面上的文件进行各种操作,比如打开文件、读取文件内容、写入文件等等。
1、检查文件是否存在
我们可以使用os.path.exists
或者pathlib.Path.exists
来检查文件是否存在。
file_name = "example.txt"
file_path = os.path.join(desktop_path, file_name)
if os.path.exists(file_path):
print(f"{file_name} exists on the desktop.")
else:
print(f"{file_name} does not exist on the desktop.")
file_path = desktop_path / "example.txt"
if file_path.exists():
print(f"{file_path.name} exists on the desktop.")
else:
print(f"{file_path.name} does not exist on the desktop.")
2、读取文件内容
我们可以使用open
函数来读取文件内容。
if os.path.exists(file_path):
with open(file_path, 'r') as file:
content = file.read()
print(content)
if file_path.exists():
with file_path.open('r') as file:
content = file.read()
print(content)
3、写入文件内容
我们可以使用open
函数并指定写入模式来写入文件内容。
file_path = os.path.join(desktop_path, "example.txt")
with open(file_path, 'w') as file:
file.write("Hello, Desktop!")
file_path = desktop_path / "example.txt"
with file_path.open('w') as file:
file.write("Hello, Desktop!")
三、移动、复制和删除文件
我们还可以使用shutil
模块来移动、复制和删除文件。
1、复制文件
import shutil
source_file = desktop_path / "example.txt"
destination_file = desktop_path / "example_copy.txt"
shutil.copy(source_file, destination_file)
print(f"Copied {source_file.name} to {destination_file.name}")
2、移动文件
destination_path = desktop_path / "example_moved.txt"
shutil.move(source_file, destination_path)
print(f"Moved {source_file.name} to {destination_path.name}")
3、删除文件
os.remove(destination_file)
print(f"Deleted {destination_file.name}")
destination_file.unlink()
print(f"Deleted {destination_file.name}")
四、列出桌面所有文件
我们可以使用os.listdir
或者pathlib.Path.iterdir
来列出桌面上的所有文件。
files = os.listdir(desktop_path)
print("Files on Desktop:")
for file in files:
print(file)
files = desktop_path.iterdir()
print("Files on Desktop:")
for file in files:
print(file.name)
五、示例代码汇总
以下是一个完整的示例,展示了如何获取桌面路径并进行各种文件操作。
import os
from pathlib import Path
import shutil
def get_desktop_path():
return Path.home() / 'Desktop'
desktop_path = get_desktop_path()
print(f"Desktop Path: {desktop_path}")
Check if file exists
file_path = desktop_path / "example.txt"
if file_path.exists():
print(f"{file_path.name} exists on the desktop.")
else:
print(f"{file_path.name} does not exist on the desktop.")
Read file content
if file_path.exists():
with file_path.open('r') as file:
content = file.read()
print(content)
Write file content
with file_path.open('w') as file:
file.write("Hello, Desktop!")
Copy file
source_file = file_path
destination_file = desktop_path / "example_copy.txt"
shutil.copy(source_file, destination_file)
print(f"Copied {source_file.name} to {destination_file.name}")
Move file
destination_path = desktop_path / "example_moved.txt"
shutil.move(source_file, destination_path)
print(f"Moved {source_file.name} to {destination_path.name}")
Delete file
destination_file.unlink()
print(f"Deleted {destination_file.name}")
List files on Desktop
files = desktop_path.iterdir()
print("Files on Desktop:")
for file in files:
print(file.name)
通过以上步骤和代码,您可以轻松地在Python中调用桌面的文件并进行各种操作。希望这些内容对您有所帮助。
相关问答FAQs:
如何在Python中访问桌面文件?
在Python中,可以使用os
库和pathlib
库来访问桌面文件。通过获取用户的桌面路径,可以轻松地读取或操作文件。例如,使用os.path.expanduser("~/Desktop/filename.txt")
可以获取桌面上某个文件的完整路径。
Python能否打开桌面上的文件?
是的,Python可以通过多种方式打开桌面上的文件。例如,可以使用os.startfile()
方法来直接打开桌面上的文件,或者使用subprocess
模块来调用其他应用程序打开该文件。这些方法可以帮助您在编程中实现对文件的快速访问。
如何在Python中列出桌面上的所有文件?
可以使用os.listdir()
函数或pathlib.Path
类来列出桌面上的所有文件。通过获取桌面的路径并传递给这些函数,您可以获取桌面上所有文件和文件夹的列表。示例代码如下:
import os
desktop_path = os.path.join(os.path.expanduser("~"), "Desktop")
files = os.listdir(desktop_path)
print(files)
Python如何处理桌面文件的路径问题?
处理文件路径时,应注意不同操作系统的路径分隔符问题。使用os.path.join()
可以确保路径在不同操作系统上的兼容性。此外,pathlib
库提供了面向对象的路径处理方式,使得路径操作更加直观和方便。
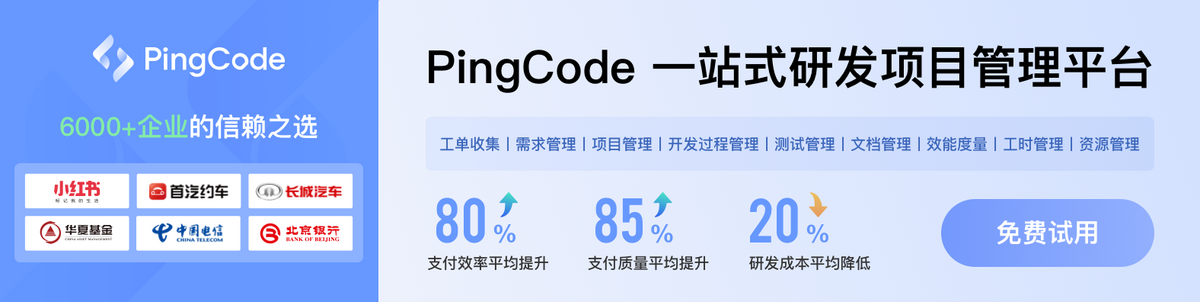