Python中要关闭特定线程,可以使用多种方法,包括设置线程标志、使用事件对象以及守护线程(Daemon Threads)等方法。
一、设置线程标志:
这种方法涉及在线程中创建一个标志变量,然后在需要停止线程时更改该标志的状态。线程在运行时会不断检查该标志的状态,如果标志被设置为停止状态,线程就会自行退出。
import threading
import time
class MyThread(threading.Thread):
def __init__(self):
threading.Thread.__init__(self)
self._stop_event = threading.Event()
def run(self):
while not self._stop_event.is_set():
print("Thread is running")
time.sleep(1)
def stop(self):
self._stop_event.set()
创建并启动线程
thread = MyThread()
thread.start()
让线程运行一段时间
time.sleep(5)
停止线程
thread.stop()
在这个例子中,MyThread
类继承自 threading.Thread
,并包含一个 _stop_event
标志。线程在运行时会不断检查 _stop_event
是否被设置,如果被设置则退出线程。
二、使用事件对象:
事件对象在多线程编程中非常有用,它允许线程在某个条件满足时停止运行。与标志变量类似,事件对象也可以被设置和清除。
import threading
import time
class MyThread(threading.Thread):
def __init__(self, event):
threading.Thread.__init__(self)
self._event = event
def run(self):
while not self._event.is_set():
print("Thread is running")
time.sleep(1)
创建事件对象
stop_event = threading.Event()
创建并启动线程
thread = MyThread(stop_event)
thread.start()
让线程运行一段时间
time.sleep(5)
设置事件对象,停止线程
stop_event.set()
在这个例子中,MyThread
类接受一个事件对象作为参数。线程在运行时会检查事件对象是否被设置,如果被设置则退出线程。
三、守护线程(Daemon Threads):
守护线程是指在主线程结束时自动退出的线程。可以通过将线程设置为守护线程来确保在主线程结束时,所有子线程也会自动退出。
import threading
import time
def my_thread():
while True:
print("Thread is running")
time.sleep(1)
创建并启动守护线程
thread = threading.Thread(target=my_thread)
thread.daemon = True
thread.start()
让主线程运行一段时间
time.sleep(5)
主线程结束,守护线程也会自动退出
print("Main thread is ending")
在这个例子中,线程被设置为守护线程,因此在主线程结束时,守护线程也会自动退出。
四、线程间通信:
使用队列可以实现线程间的通信和控制。通过将特定的消息放入队列,可以通知线程执行某些操作,例如停止运行。
import threading
import queue
import time
def my_thread(q):
while True:
try:
msg = q.get(block=False)
if msg == "STOP":
break
except queue.Empty:
print("Thread is running")
time.sleep(1)
创建队列对象
q = queue.Queue()
创建并启动线程
thread = threading.Thread(target=my_thread, args=(q,))
thread.start()
让线程运行一段时间
time.sleep(5)
向队列发送停止消息
q.put("STOP")
等待线程结束
thread.join()
在这个例子中,线程通过检查队列中的消息来判断是否需要停止运行。如果收到 "STOP" 消息,线程会退出。
五、使用线程池:
线程池可以更方便地管理线程的生命周期。在使用线程池时,可以提交任务并使用 concurrent.futures
模块来管理线程。
import concurrent.futures
import time
def my_task():
while True:
print("Task is running")
time.sleep(1)
创建线程池
with concurrent.futures.ThreadPoolExecutor(max_workers=1) as executor:
future = executor.submit(my_task)
# 让任务运行一段时间
time.sleep(5)
# 取消任务
future.cancel()
在这个例子中,使用线程池提交任务,并在需要时取消任务。
总结:
在 Python 中关闭特定线程可以通过多种方法实现,包括设置线程标志、使用事件对象、守护线程、线程间通信以及使用线程池等方法。选择合适的方法取决于具体的应用场景和需求。无论使用哪种方法,都需要确保线程安全,避免资源泄露和竞争条件等问题。
相关问答FAQs:
如何通过线程名称找到并关闭指定线程?
要关闭指定名称的线程,您可以使用threading
模块中的enumerate()
方法获取当前所有活动线程的列表。随后,遍历这些线程,检查每个线程的名称是否匹配,找到后可以通过设置一个标志变量来安全地停止线程。请注意,直接强制关闭线程是不推荐的,因为这可能导致资源未被释放或数据不一致。
在Python中如何安全地停止一个线程?
在Python中,安全停止线程的一种常见方法是使用事件对象。可以通过threading.Event()
创建一个事件,当需要停止线程时,设置该事件为真,线程在循环中检查这个事件,如果发现事件被设置就退出循环,从而安全地结束线程。
如果线程没有名称,如何关闭它?
如果线程没有名称,您可以使用线程对象的引用来管理它。通过threading.active_count()
和threading.enumerate()
函数获取当前活动线程的列表,逐个检查线程对象是否是您要关闭的线程。通过对线程对象的引用,可以使用相同的方法来安全地结束线程。
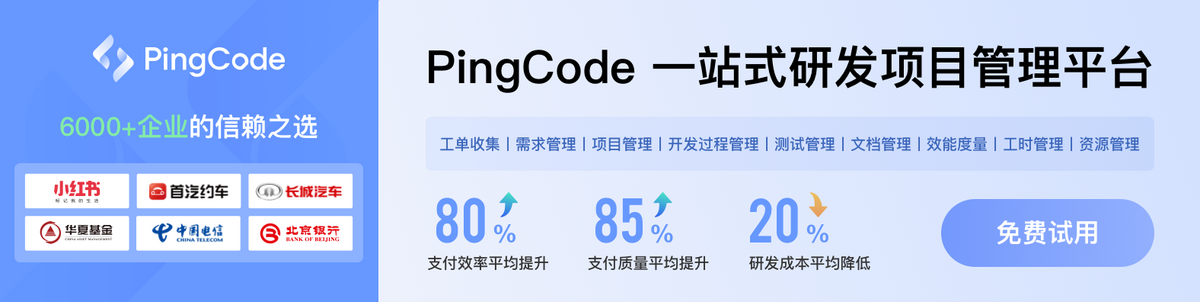