Python程序如何打开exe文件:可以使用subprocess
模块、os
模块、os.system
函数。其中,推荐使用subprocess
模块进行操作,因为它提供了更多的控制选项和更好的错误处理机制。例如,通过使用subprocess.run()
函数,可以捕获输出、处理错误、设置超时等。
详细描述:使用subprocess
模块打开exe文件时,我们需要导入subprocess
模块,然后调用subprocess.run()
或subprocess.Popen()
函数来执行exe文件。这个方法不仅仅适用于打开exe文件,还可以用于执行其他系统命令和脚本。
import subprocess
使用subprocess.run()函数打开exe文件
subprocess.run(["path_to_exe_file/executable_file.exe"])
下面将详细介绍如何使用Python程序打开exe文件,涵盖不同的方法和使用场景。
一、使用subprocess模块
1、subprocess.run()函数
subprocess.run()
函数是Python 3.5引入的一个更高级的接口。它可以捕获标准输出、标准错误,并设置超时。
import subprocess
打开exe文件并等待其完成执行
result = subprocess.run(["path_to_exe_file/executable_file.exe"], capture_output=True, text=True)
打印输出
print(result.stdout)
可以通过capture_output=True
参数捕获输出,并通过text=True
参数将输出转换为字符串。
2、subprocess.Popen()函数
subprocess.Popen()
函数提供了更底层的接口,可以对进程进行更精细的控制。
import subprocess
打开exe文件并继续执行后续代码
process = subprocess.Popen(["path_to_exe_file/executable_file.exe"], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
等待进程完成并获取输出
stdout, stderr = process.communicate()
print(stdout.decode())
subprocess.Popen()
函数允许你异步地执行exe文件,并通过stdout
和stderr
参数捕获输出和错误。
二、使用os模块
1、os.system()函数
os.system()
函数是一个简单的方式来执行系统命令,但它不提供捕获输出和处理错误的功能。
import os
使用os.system()打开exe文件
os.system("path_to_exe_file/executable_file.exe")
虽然os.system()
函数易于使用,但它在功能和灵活性方面不如subprocess
模块。
2、os.startfile()函数
os.startfile()
函数适用于Windows操作系统,可以用来打开文件或运行程序。
import os
使用os.startfile()打开exe文件
os.startfile("path_to_exe_file/executable_file.exe")
os.startfile()
函数类似于双击文件,它会使用默认关联的程序打开文件。
三、处理不同操作系统的差异
不同操作系统对文件路径和命令的处理方式有所不同,因此在编写跨平台代码时需要注意这些差异。
1、检测操作系统
可以使用os.name
或platform
模块来检测操作系统,并根据不同的系统执行相应的代码。
import os
import platform
if os.name == 'nt': # Windows
os.startfile("path_to_exe_file/executable_file.exe")
elif os.name == 'posix': # Unix/Linux/Mac
subprocess.run(["./path_to_exe_file/executable_file"])
else:
print("Unsupported operating system")
2、使用相对路径
为了使代码更具可移植性,可以使用相对路径而不是绝对路径。
import os
import subprocess
获取当前脚本的目录
script_dir = os.path.dirname(os.path.abspath(__file__))
构建相对路径
exe_path = os.path.join(script_dir, "path_to_exe_file/executable_file.exe")
使用subprocess打开exe文件
subprocess.run([exe_path])
这样可以确保exe文件在不同系统上的相对位置一致。
四、捕获输出和处理错误
在运行exe文件时,捕获输出和处理错误是非常重要的。subprocess.run()
和subprocess.Popen()
函数都提供了捕获输出和错误的功能。
1、捕获标准输出和标准错误
使用subprocess.run()
函数时,可以通过capture_output
参数捕获标准输出和标准错误。
import subprocess
打开exe文件并捕获输出和错误
result = subprocess.run(["path_to_exe_file/executable_file.exe"], capture_output=True, text=True)
打印标准输出
print("Standard Output:", result.stdout)
打印标准错误
print("Standard Error:", result.stderr)
2、处理异常和错误
在执行exe文件时,可能会遇到各种异常和错误。可以使用try-except
语句来捕获和处理这些异常。
import subprocess
try:
# 打开exe文件
result = subprocess.run(["path_to_exe_file/executable_file.exe"], capture_output=True, text=True, check=True)
print("Standard Output:", result.stdout)
except subprocess.CalledProcessError as e:
print("Error:", e)
通过设置check=True
参数,如果进程返回非零退出状态,将引发subprocess.CalledProcessError
异常。
五、使用超时和异步执行
1、设置超时
在某些情况下,可能需要为exe文件的执行设置超时。可以使用timeout
参数来设置超时。
import subprocess
try:
# 打开exe文件并设置超时
result = subprocess.run(["path_to_exe_file/executable_file.exe"], timeout=10, capture_output=True, text=True)
print("Standard Output:", result.stdout)
except subprocess.TimeoutExpired as e:
print("Process timed out:", e)
2、异步执行
subprocess.Popen()
函数允许异步执行exe文件,可以在等待进程完成的同时执行其他操作。
import subprocess
打开exe文件并异步执行
process = subprocess.Popen(["path_to_exe_file/executable_file.exe"], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
执行其他操作
print("Doing other work...")
等待进程完成并获取输出
stdout, stderr = process.communicate()
print("Standard Output:", stdout.decode())
通过subprocess.Popen()
函数,可以在进程执行期间继续执行其他代码,提高程序的并发性。
六、执行带参数的exe文件
有时候需要向exe文件传递参数,可以在subprocess.run()
或subprocess.Popen()
函数中指定参数。
1、传递命令行参数
import subprocess
打开exe文件并传递参数
subprocess.run(["path_to_exe_file/executable_file.exe", "arg1", "arg2"])
2、使用字典传递环境变量
可以使用env
参数传递环境变量。
import subprocess
import os
构建环境变量字典
env = os.environ.copy()
env["MY_VAR"] = "value"
打开exe文件并传递环境变量
subprocess.run(["path_to_exe_file/executable_file.exe"], env=env)
通过这种方式,可以在执行exe文件时设置特定的环境变量。
七、处理GUI应用程序
在使用Python打开GUI应用程序时,有一些特定的注意事项。
1、使用subprocess.Popen()函数
对于GUI应用程序,使用subprocess.Popen()
函数可以避免阻塞主线程。
import subprocess
打开GUI应用程序
process = subprocess.Popen(["path_to_exe_file/gui_application.exe"])
执行其他操作
print("GUI application is running...")
2、检测进程状态
可以使用poll()
方法检测进程的状态。
import subprocess
打开GUI应用程序
process = subprocess.Popen(["path_to_exe_file/gui_application.exe"])
检测进程状态
if process.poll() is None:
print("GUI application is still running")
else:
print("GUI application has finished")
通过检测进程状态,可以在GUI应用程序运行时执行其他操作,并在其结束后进行相应处理。
八、使用第三方库
除了标准库,还可以使用一些第三方库来打开exe文件,例如psutil
库。
1、安装psutil库
pip install psutil
2、使用psutil库打开exe文件
import psutil
import subprocess
打开exe文件
process = subprocess.Popen(["path_to_exe_file/executable_file.exe"])
获取进程信息
proc_info = psutil.Process(process.pid)
打印进程信息
print("Process name:", proc_info.name())
print("Process status:", proc_info.status())
psutil
库提供了丰富的进程管理功能,可以获取进程信息、监控进程状态等。
九、跨平台兼容性
在编写跨平台代码时,需要考虑不同操作系统的差异,并使用适当的工具和方法。
1、使用平台特定的命令
对于Windows和Unix系统,可以使用不同的命令来打开exe文件。
import os
import subprocess
if os.name == 'nt': # Windows
os.startfile("path_to_exe_file/executable_file.exe")
elif os.name == 'posix': # Unix/Linux/Mac
subprocess.run(["./path_to_exe_file/executable_file"])
else:
print("Unsupported operating system")
2、使用跨平台库
可以使用一些跨平台库,例如PyInstaller
,将Python脚本打包成可执行文件,以便在不同操作系统上运行。
pip install pyinstaller
打包Python脚本
pyinstaller --onefile script.py
通过这种方式,可以生成适用于不同操作系统的可执行文件,简化了跨平台开发。
十、总结
使用Python程序打开exe文件的方法有很多,可以根据具体需求选择合适的方法。推荐使用subprocess
模块,因为它提供了更多的控制选项和更好的错误处理机制。此外,还可以使用os
模块、第三方库等来实现相同的功能。在编写跨平台代码时,需要考虑不同操作系统的差异,并使用适当的工具和方法。通过合理使用这些方法,可以高效地在Python程序中打开和管理exe文件。
相关问答FAQs:
如何在Python中安全地打开exe文件?
在Python中打开exe文件可以使用subprocess
模块。这个模块提供了强大的功能,可以执行外部命令,并能够处理输入和输出流。使用subprocess.run()
或subprocess.Popen()
方法,可以方便地启动exe文件,确保安全性和稳定性。例如:
import subprocess
subprocess.run(['path_to_your_exe_file.exe'])
是否可以通过Python传递参数给exe文件?
是的,使用subprocess
模块时,您可以轻松地传递参数给exe文件。只需在subprocess.run()
或subprocess.Popen()
的参数列表中添加所需的参数。例如:
subprocess.run(['path_to_your_exe_file.exe', 'arg1', 'arg2'])
这样,exe文件就可以接收并处理这些参数了。
在打开exe文件时,如何处理可能的错误?
在调用exe文件时,处理潜在的错误是非常重要的。可以使用try-except
块来捕获并处理异常,确保程序的健壮性。例如:
import subprocess
try:
subprocess.run(['path_to_your_exe_file.exe'], check=True)
except subprocess.CalledProcessError as e:
print(f"An error occurred: {e}")
通过这种方式,您可以捕获执行exe文件时的错误并进行相应的处理。
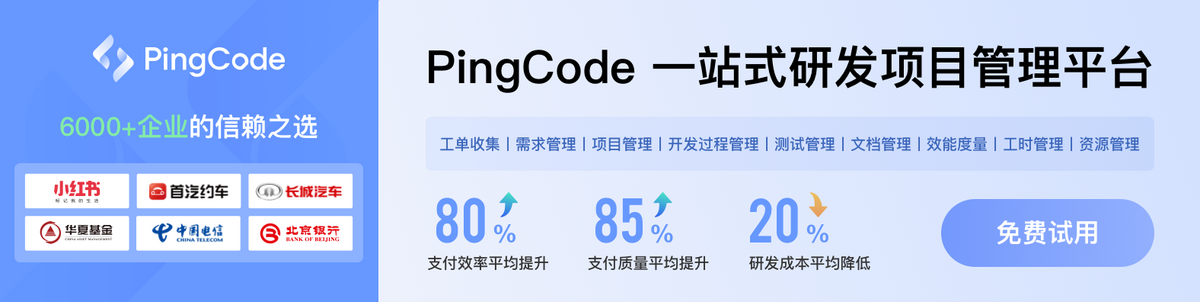