在Python中,统计最大值的方法有多种,主要包括使用内置函数max()
、列表的max()
方法、以及通过循环遍历来手动查找最大值等。 其中,使用内置函数max()
是最简单和直接的方法。
例如,假设有一个列表numbers
,可以通过max(numbers)
来获取列表中的最大值。下面将详细描述使用内置函数max()
的方法。
numbers = [3, 5, 7, 2, 8, 1]
max_value = max(numbers)
print("The maximum value in the list is:", max_value)
在这个示例中,max(numbers)
会返回列表中的最大值,这里是8。
一、使用内置函数max()
Python提供了一个内置函数max()
,它可以直接用于找到可迭代对象中的最大值。
1、基本用法
max()
函数可以用于列表、元组、字符串等可迭代对象。它的基本语法如下:
max(iterable, *[, key, default])
其中,iterable
是一个可迭代对象,key
是一个可选参数,用于指定一个函数,该函数会在比较过程中应用于每个元素,default
参数用于指定在可迭代对象为空时返回的默认值。
numbers = [10, 20, 30, 40, 50]
max_value = max(numbers)
print(max_value) # 输出:50
2、使用key
参数
有时候,我们需要根据特定的条件来查找最大值,这时候可以使用key
参数。
students = [
{'name': 'Alice', 'age': 25},
{'name': 'Bob', 'age': 30},
{'name': 'Charlie', 'age': 28}
]
oldest_student = max(students, key=lambda student: student['age'])
print(oldest_student) # 输出:{'name': 'Bob', 'age': 30}
在这个例子中,我们通过key
参数指定了一个匿名函数lambda student: student['age']
,max()
函数会根据学生的年龄来查找最大值。
二、使用列表的max()
方法
在Python中,列表没有单独的max()
方法,但可以通过max()
函数找到列表中的最大值。
numbers = [3, 5, 7, 2, 8, 1]
max_value = max(numbers)
print("The maximum value in the list is:", max_value)
三、通过循环遍历手动查找最大值
有时候为了更灵活的处理逻辑,可能需要手动实现查找最大值的方法。通过循环遍历来找到最大值也是一种常见的方法。
numbers = [3, 5, 7, 2, 8, 1]
max_value = numbers[0]
for number in numbers:
if number > max_value:
max_value = number
print("The maximum value in the list is:", max_value)
在这个例子中,我们首先假设列表中的第一个元素是最大值,然后通过遍历列表中的每一个元素,依次比较并更新最大值。
四、使用NumPy库
对于数值计算,NumPy库提供了更多的功能和更高的性能。NumPy的amax()
函数可以用来查找数组中的最大值。
import numpy as np
numbers = np.array([3, 5, 7, 2, 8, 1])
max_value = np.amax(numbers)
print("The maximum value in the array is:", max_value)
在这个示例中,np.amax(numbers)
会返回数组中的最大值。
五、在多维数组中查找最大值
在处理多维数组时,可以通过指定轴来查找最大值。轴的概念在多维数组中非常重要,它决定了操作是沿着哪个维度进行的。
import numpy as np
matrix = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
max_value = np.amax(matrix, axis=0)
print("The maximum values along the columns are:", max_value)
max_value = np.amax(matrix, axis=1)
print("The maximum values along the rows are:", max_value)
在这个示例中,np.amax(matrix, axis=0)
会返回每一列的最大值,而np.amax(matrix, axis=1)
会返回每一行的最大值。
六、在字典中查找最大值
有时候,我们需要在字典中查找最大值,可以通过max()
函数结合key
参数来实现。
scores = {'Alice': 85, 'Bob': 90, 'Charlie': 78}
max_score = max(scores, key=scores.get)
print("The person with the highest score is:", max_score)
在这个示例中,max(scores, key=scores.get)
会返回字典中得分最高的键,这里是'Bob'。
七、在集合中查找最大值
集合也是一种可迭代对象,我们可以使用max()
函数在集合中查找最大值。
numbers = {3, 5, 7, 2, 8, 1}
max_value = max(numbers)
print("The maximum value in the set is:", max_value)
在这个示例中,max(numbers)
会返回集合中的最大值,这里是8。
八、在字符串中查找最大值
字符串也是一种可迭代对象,可以使用max()
函数在字符串中查找最大字符。
text = "python"
max_char = max(text)
print("The maximum character in the string is:", max_char)
在这个示例中,max(text)
会返回字符串中的最大字符,这里是'y'。
九、在二维列表中查找最大值
在处理二维列表时,可以通过嵌套的max()
函数来查找最大值。
matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]]
max_value = max(max(row) for row in matrix)
print("The maximum value in the matrix is:", max_value)
在这个示例中,我们首先通过max(row)
找到每一行的最大值,然后通过外层的max()
函数找到这些最大值中的最大值。
十、在Pandas DataFrame中查找最大值
Pandas是一个非常强大的数据分析库,可以轻松处理和分析数据。在Pandas DataFrame中,可以使用max()
方法来查找最大值。
import pandas as pd
data = {'A': [1, 2, 3], 'B': [4, 5, 6], 'C': [7, 8, 9]}
df = pd.DataFrame(data)
max_value = df.max()
print("The maximum values in each column are:\n", max_value)
max_value = df.max(axis=1)
print("The maximum values in each row are:\n", max_value)
在这个示例中,df.max()
会返回每一列的最大值,而df.max(axis=1)
会返回每一行的最大值。
十一、在多线程环境中查找最大值
在多线程环境中查找最大值,可以利用ThreadPoolExecutor
来并行计算。
import concurrent.futures
numbers = [3, 5, 7, 2, 8, 1]
def find_max(numbers):
return max(numbers)
with concurrent.futures.ThreadPoolExecutor() as executor:
future = executor.submit(find_max, numbers)
max_value = future.result()
print("The maximum value in the list is:", max_value)
在这个示例中,我们通过ThreadPoolExecutor
提交了一个任务来查找最大值,并通过future.result()
获取结果。
十二、在分布式环境中查找最大值
在分布式环境中查找最大值,可以利用Dask
库来并行计算。
import dask.array as da
numbers = da.array([3, 5, 7, 2, 8, 1])
max_value = numbers.max().compute()
print("The maximum value in the array is:", max_value)
在这个示例中,我们使用Dask
库将数组分布式处理,并通过compute()
方法获取结果。
十三、在大数据集上查找最大值
在处理大数据集时,可以利用PySpark
来查找最大值。
from pyspark.sql import SparkSession
spark = SparkSession.builder.appName("MaxValue").getOrCreate()
data = [(1,), (2,), (3,), (4,), (5,)]
df = spark.createDataFrame(data, ["value"])
max_value = df.agg({"value": "max"}).collect()[0][0]
print("The maximum value in the DataFrame is:", max_value)
在这个示例中,我们使用PySpark
来创建一个DataFrame,并通过agg()
方法查找最大值。
十四、在数据库中查找最大值
在数据库中查找最大值,可以通过SQL查询语句来实现。
import sqlite3
conn = sqlite3.connect(':memory:')
cursor = conn.cursor()
cursor.execute("CREATE TABLE numbers (value INTEGER)")
cursor.executemany("INSERT INTO numbers (value) VALUES (?)", [(1,), (2,), (3,), (4,), (5,)])
cursor.execute("SELECT MAX(value) FROM numbers")
max_value = cursor.fetchone()[0]
print("The maximum value in the table is:", max_value)
conn.close()
在这个示例中,我们使用SQLite数据库创建一个表,并通过SQL查询语句查找最大值。
十五、在JSON数据中查找最大值
在处理JSON数据时,可以通过解析JSON并使用max()
函数来查找最大值。
import json
data = '{"numbers": [1, 2, 3, 4, 5]}'
parsed_data = json.loads(data)
max_value = max(parsed_data["numbers"])
print("The maximum value in the JSON data is:", max_value)
在这个示例中,我们首先解析JSON数据,然后通过max()
函数查找最大值。
十六、在XML数据中查找最大值
在处理XML数据时,可以通过解析XML并使用max()
函数来查找最大值。
import xml.etree.ElementTree as ET
data = """
<numbers>
<number>1</number>
<number>2</number>
<number>3</number>
<number>4</number>
<number>5</number>
</numbers>
"""
root = ET.fromstring(data)
numbers = [int(number.text) for number in root.findall('number')]
max_value = max(numbers)
print("The maximum value in the XML data is:", max_value)
在这个示例中,我们首先解析XML数据,然后通过max()
函数查找最大值。
十七、在CSV文件中查找最大值
在处理CSV文件时,可以通过读取CSV文件并使用max()
函数来查找最大值。
import csv
with open('numbers.csv', newline='') as csvfile:
reader = csv.reader(csvfile)
numbers = [int(row[0]) for row in reader]
max_value = max(numbers)
print("The maximum value in the CSV file is:", max_value)
在这个示例中,我们首先读取CSV文件,然后通过max()
函数查找最大值。
十八、在文本文件中查找最大值
在处理文本文件时,可以通过读取文件并使用max()
函数来查找最大值。
with open('numbers.txt') as file:
numbers = [int(line.strip()) for line in file]
max_value = max(numbers)
print("The maximum value in the text file is:", max_value)
在这个示例中,我们首先读取文本文件,然后通过max()
函数查找最大值。
十九、在混合数据类型中查找最大值
在处理混合数据类型时,可以通过自定义比较函数来查找最大值。
data = [1, '2', 3, '4', 5]
def custom_max(a, b):
return a if int(a) > int(b) else b
max_value = data[0]
for item in data:
max_value = custom_max(max_value, item)
print("The maximum value in the mixed data is:", max_value)
在这个示例中,我们通过自定义比较函数custom_max
来处理混合数据类型,并通过循环查找最大值。
二十、在复杂数据结构中查找最大值
在处理复杂数据结构时,可以通过递归函数来查找最大值。
data = [1, [2, 3], [4, [5, 6]], 7]
def find_max(data):
max_value = float('-inf')
for item in data:
if isinstance(item, list):
max_value = max(max_value, find_max(item))
else:
max_value = max(max_value, item)
return max_value
max_value = find_max(data)
print("The maximum value in the complex data is:", max_value)
在这个示例中,我们通过递归函数find_max
来处理复杂数据结构,并查找最大值。
结论
在Python中,统计最大值的方法有很多,选择合适的方法取决于具体的场景和数据类型。无论是使用内置函数max()
、列表的max()
方法,还是通过循环遍历来手动查找最大值,都可以有效地找到数据中的最大值。对于大数据集或分布式计算,可以利用NumPy、Pandas、Dask、PySpark等库来提高性能和效率。通过灵活运用这些方法,可以在各种复杂的数据结构和场景中查找最大值,满足不同的需求。
相关问答FAQs:
如何在Python中找到列表的最大值?
在Python中,可以使用内置的max()
函数轻松找到列表中的最大值。例如,如果你有一个包含数字的列表numbers = [1, 2, 3, 4, 5]
,可以通过max_value = max(numbers)
来获取最大值,结果将是5。此外,max()
函数也可以接受多个参数,从而比较多个数字并返回最大的一个。
是否可以自定义比较规则来统计最大值?
是的,Python的max()
函数允许你通过key
参数自定义比较规则。例如,如果你有一个包含字典的列表,并希望根据某个字段的值来找出最大值,可以使用一个lambda函数作为key
参数。假设有一个字典列表data = [{'value': 10}, {'value': 20}, {'value': 15}]
,可以通过max(data, key=lambda x: x['value'])
找到{'value': 20}
,也就是最大值的字典。
如何统计多个最大值而不仅仅是一个?
如果你想要获取多个最大值,可以先对列表进行排序,或者使用heapq
模块中的nlargest
函数。nlargest(n, iterable)
可以返回前n个最大值。例如,如果你想找出列表numbers = [3, 1, 4, 1, 5, 9, 2, 6]
中的三个最大值,可以使用import heapq
后,执行top_three = heapq.nlargest(3, numbers)
,结果将是[9, 6, 5]
。这样可以有效地获取最大的多个数值。
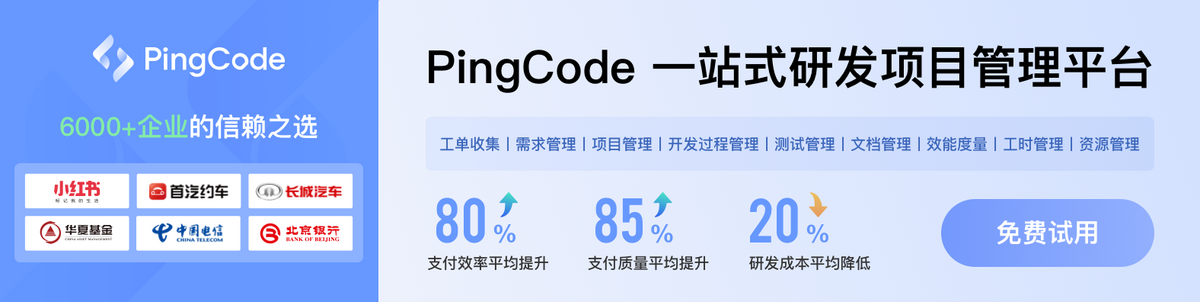