在Python中设计多线程程序时,需要使用threading模块、选择合适的同步机制、合理的任务分配、注意线程安全。多线程的主要目的是通过并行处理来提高程序的执行效率,尤其是在I/O密集型任务中。下面我将详细描述如何使用threading模块来创建和管理多线程程序。
一、THREADING模块介绍
Python的threading模块提供了一个Thread类,用于创建和管理线程。使用Thread类可以方便地启动、停止和管理线程。以下是Thread类的一些关键方法和属性:
Thread(target, args=(), kwargs={})
:用于创建新线程,target
是线程要执行的函数,args
和kwargs
是传递给该函数的参数。start()
:启动线程,使其运行target
函数。join()
:阻塞主线程,直到调用join
的线程结束。is_alive()
:检查线程是否还在运行。
二、创建和启动线程
创建和启动线程是多线程编程的第一步。可以通过继承Thread类或者直接使用Thread类创建线程。
1、继承Thread类
通过继承Thread类并重写其run方法,可以创建自定义线程类:
import threading
import time
class MyThread(threading.Thread):
def __init__(self, name):
threading.Thread.__init__(self)
self.name = name
def run(self):
print(f"Thread {self.name} is starting.")
time.sleep(2)
print(f"Thread {self.name} is ending.")
创建线程实例
thread1 = MyThread("A")
thread2 = MyThread("B")
启动线程
thread1.start()
thread2.start()
等待所有线程完成
thread1.join()
thread2.join()
2、使用Thread类
直接使用Thread类创建线程并指定目标函数:
import threading
import time
def worker(name):
print(f"Thread {name} is starting.")
time.sleep(2)
print(f"Thread {name} is ending.")
创建线程实例
thread1 = threading.Thread(target=worker, args=("A",))
thread2 = threading.Thread(target=worker, args=("B",))
启动线程
thread1.start()
thread2.start()
等待所有线程完成
thread1.join()
thread2.join()
三、线程同步机制
多线程程序中,多个线程可能需要访问共享资源,这时就需要使用同步机制来避免竞争条件和数据不一致。Python提供了多种同步机制,如Lock、RLock、Semaphore、Event和Condition。
1、Lock和RLock
Lock是最简单的同步机制,通过acquire和release方法来实现对共享资源的互斥访问。RLock是可重入锁,允许同一线程多次获得锁。
import threading
lock = threading.Lock()
shared_data = 0
def increment():
global shared_data
lock.acquire()
try:
for _ in range(100000):
shared_data += 1
finally:
lock.release()
创建并启动线程
threads = []
for _ in range(10):
thread = threading.Thread(target=increment)
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
print(f"Final shared_data: {shared_data}")
2、Semaphore
Semaphore用于控制同时访问特定资源的线程数。它维护一个计数器,只有当计数器大于0时,线程才能访问资源。
import threading
import time
semaphore = threading.Semaphore(3)
def worker(name):
semaphore.acquire()
print(f"Thread {name} is starting.")
time.sleep(2)
print(f"Thread {name} is ending.")
semaphore.release()
创建并启动线程
threads = []
for i in range(5):
thread = threading.Thread(target=worker, args=(f"Thread-{i}",))
threads.append(thread)
thread.start()
等待所有线程完成
for thread in threads:
thread.join()
四、线程池
对于大量短小任务的多线程程序,可以使用线程池来管理线程。线程池可以避免频繁创建和销毁线程的开销,提高程序性能。Python的concurrent.futures模块提供了ThreadPoolExecutor类来实现线程池。
from concurrent.futures import ThreadPoolExecutor
import time
def worker(name):
print(f"Thread {name} is starting.")
time.sleep(2)
print(f"Thread {name} is ending.")
创建线程池
with ThreadPoolExecutor(max_workers=3) as executor:
futures = [executor.submit(worker, f"Thread-{i}") for i in range(5)]
等待所有线程完成
for future in futures:
future.result()
五、线程通信
线程之间的通信是多线程编程中的一个重要方面。Python提供了多种线程间通信的方式,如Queue、Event、Condition等。
1、Queue
Queue是线程安全的队列,适用于生产者-消费者模型。Queue模块提供了FIFO队列Queue、LIFO队列LifoQueue和优先级队列PriorityQueue。
import threading
import queue
q = queue.Queue()
def producer():
for i in range(5):
item = f"item-{i}"
q.put(item)
print(f"Produced: {item}")
def consumer():
while True:
item = q.get()
if item is None:
break
print(f"Consumed: {item}")
q.task_done()
创建并启动线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
等待生产者线程完成
producer_thread.join()
向队列中放置None,表示结束
q.put(None)
等待消费者线程完成
consumer_thread.join()
2、Event
Event是线程间的信号机制,一个线程可以设置事件,另一个线程可以等待事件发生。
import threading
import time
event = threading.Event()
def worker():
print("Worker is waiting for event.")
event.wait()
print("Worker received event.")
def setter():
time.sleep(2)
print("Setter is setting event.")
event.set()
创建并启动线程
worker_thread = threading.Thread(target=worker)
setter_thread = threading.Thread(target=setter)
worker_thread.start()
setter_thread.start()
等待所有线程完成
worker_thread.join()
setter_thread.join()
3、Condition
Condition是更复杂的线程同步机制,可以实现复杂的线程间通信。
import threading
import time
condition = threading.Condition()
shared_data = []
def producer():
global shared_data
for i in range(5):
time.sleep(1)
condition.acquire()
item = f"item-{i}"
shared_data.append(item)
print(f"Produced: {item}")
condition.notify()
condition.release()
def consumer():
global shared_data
while True:
condition.acquire()
while not shared_data:
condition.wait()
item = shared_data.pop(0)
print(f"Consumed: {item}")
condition.release()
if item == "item-4":
break
创建并启动线程
producer_thread = threading.Thread(target=producer)
consumer_thread = threading.Thread(target=consumer)
producer_thread.start()
consumer_thread.start()
等待所有线程完成
producer_thread.join()
consumer_thread.join()
六、线程安全
线程安全是指多个线程访问共享资源时,能够保证数据的一致性和正确性。使用合适的同步机制可以实现线程安全,但也要注意避免死锁和性能问题。
1、避免死锁
死锁是指两个或多个线程相互等待对方释放资源,导致程序无法继续执行。可以通过避免嵌套锁和使用超时机制来避免死锁。
import threading
lock1 = threading.Lock()
lock2 = threading.Lock()
def worker1():
lock1.acquire()
print("Worker1 acquired lock1")
lock2.acquire()
print("Worker1 acquired lock2")
lock2.release()
lock1.release()
def worker2():
lock2.acquire()
print("Worker2 acquired lock2")
lock1.acquire()
print("Worker2 acquired lock1")
lock1.release()
lock2.release()
创建并启动线程
thread1 = threading.Thread(target=worker1)
thread2 = threading.Thread(target=worker2)
thread1.start()
thread2.start()
等待所有线程完成
thread1.join()
thread2.join()
2、性能优化
使用多线程时,需要注意线程的创建和销毁开销、上下文切换开销等。通过合理的任务分配和使用线程池,可以提高多线程程序的性能。
from concurrent.futures import ThreadPoolExecutor
import time
def worker(name):
print(f"Thread {name} is starting.")
time.sleep(2)
print(f"Thread {name} is ending.")
创建线程池
with ThreadPoolExecutor(max_workers=3) as executor:
futures = [executor.submit(worker, f"Thread-{i}") for i in range(5)]
等待所有线程完成
for future in futures:
future.result()
七、GIL的影响
Python的全局解释器锁(GIL)限制了多线程程序的并行执行能力。GIL使得同一时刻只有一个线程能执行Python字节码,这对于CPU密集型任务来说是一个瓶颈。可以通过以下方法来规避GIL的影响:
1、使用多进程
对于CPU密集型任务,可以使用多进程而不是多线程。multiprocessing模块提供了Process类来创建和管理进程。
import multiprocessing
import time
def worker(name):
print(f"Process {name} is starting.")
time.sleep(2)
print(f"Process {name} is ending.")
创建并启动进程
process1 = multiprocessing.Process(target=worker, args=("A",))
process2 = multiprocessing.Process(target=worker, args=("B",))
process1.start()
process2.start()
等待所有进程完成
process1.join()
process2.join()
2、使用C扩展
对于性能要求较高的部分,可以使用C扩展模块来实现。C扩展模块可以绕过GIL,实现真正的并行执行。
八、总结
设计多线程程序需要综合考虑任务的性质、同步机制、线程安全、性能优化等因素。通过合理使用threading模块和其他同步机制,可以实现高效的多线程程序。在实际应用中,需要根据具体情况选择合适的方案,以充分发挥多线程的优势。
在Python中,设计多线程程序不仅仅是创建和启动线程,还需要考虑线程间的通信、同步机制、线程安全、性能优化等方面。通过合理使用Python提供的各种工具和机制,可以实现高效、安全的多线程程序。希望本文对你在设计Python多线程程序时有所帮助。
相关问答FAQs:
在Python中,多线程程序的优势是什么?
多线程程序能够提高应用程序的性能,尤其是在处理IO密集型任务时,如网络请求和文件读取。通过并发执行多个线程,程序可以在等待某个任务完成时,继续执行其他任务,从而充分利用CPU资源。此外,多线程还可以使用户界面更加流畅,因为界面操作和后台处理可以并行进行。
如何在Python中创建和管理线程?
在Python中,可以使用threading
模块来创建和管理线程。首先,通过继承Thread
类或使用Thread
对象来定义线程。在创建线程后,可以通过start()
方法启动线程,并使用join()
方法等待线程完成。这样可以确保主线程在所有子线程执行完成后再继续进行。
如何处理多线程中的数据共享和同步问题?
在多线程环境中,数据共享可能导致竞争条件,进而影响程序的正确性。使用Lock
类可以有效地管理对共享资源的访问,确保同一时间只有一个线程可以访问该资源。此外,还可以使用RLock
、Semaphore
和Condition
等同步原语来处理更复杂的线程同步需求,确保数据的一致性和安全性。
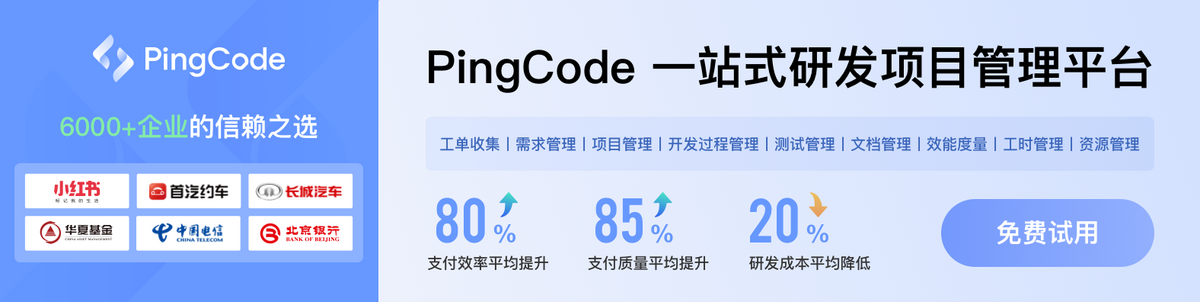