要在Python中将文件写入桌面,可以使用标准库中的os
模块和open
函数。首先,使用os.path.expanduser()
函数获取桌面路径、然后使用open()
函数打开文件并写入内容。下面是详细的步骤。
一、获取桌面路径
桌面路径因操作系统不同而有所不同。我们可以通过os.path.expanduser('~')
来获取用户的主目录,然后根据操作系统拼接出桌面的完整路径。
import os
def get_desktop_path():
home = os.path.expanduser('~')
desktop = os.path.join(home, 'Desktop')
return desktop
二、写入文件
使用open
函数打开文件并写入内容。可以使用模式'w'
来写入文件,如果文件不存在会自动创建,如果文件存在会覆盖。
def write_to_desktop_file(filename, content):
desktop = get_desktop_path()
file_path = os.path.join(desktop, filename)
with open(file_path, 'w') as file:
file.write(content)
print(f"File '{filename}' written to desktop successfully.")
Example usage:
write_to_desktop_file('example.txt', 'Hello, this is a test content!')
三、处理文件写入的异常情况
在文件写入过程中,可能会遇到一些异常情况,例如权限不足、磁盘空间不足等。我们可以通过捕获异常来处理这些情况。
def safe_write_to_desktop_file(filename, content):
try:
write_to_desktop_file(filename, content)
except PermissionError:
print("Permission denied: unable to write to the specified location.")
except IOError as e:
print(f"IO error occurred: {e}")
except Exception as e:
print(f"An unexpected error occurred: {e}")
Example usage:
safe_write_to_desktop_file('example.txt', 'Hello, this is a test content!')
四、处理不同操作系统的桌面路径
不同操作系统的桌面路径可能有所不同。为了兼容性,我们可以根据操作系统类型确定桌面路径。
import platform
def get_desktop_path():
home = os.path.expanduser('~')
if platform.system() == 'Windows':
desktop = os.path.join(home, 'Desktop')
elif platform.system() == 'Darwin': # macOS
desktop = os.path.join(home, 'Desktop')
elif platform.system() == 'Linux':
desktop = os.path.join(home, 'Desktop')
else:
raise Exception("Unsupported Operating System")
return desktop
Example usage:
print(get_desktop_path())
五、写入二进制文件
有时候我们需要写入二进制文件,例如图片、音频文件等。可以使用模式'wb'
来写入二进制文件。
def write_binary_to_desktop_file(filename, content):
desktop = get_desktop_path()
file_path = os.path.join(desktop, filename)
with open(file_path, 'wb') as file:
file.write(content)
print(f"Binary file '{filename}' written to desktop successfully.")
Example usage:
binary_content = b'\x89PNG\r\n\x1a\n\x00\x00\x00\rIHDR\x00\x00\x00\x10\x00\x00\x00\x10\x08\x06\x00\x00\x00\x1f\xf3\xff\xa5\x00\x00\x00'
write_binary_to_desktop_file('example.png', binary_content)
六、写入CSV文件
CSV文件是一种常见的数据文件格式,可以使用csv
模块来写入CSV文件。
import csv
def write_csv_to_desktop_file(filename, data):
desktop = get_desktop_path()
file_path = os.path.join(desktop, filename)
with open(file_path, 'w', newline='') as file:
writer = csv.writer(file)
writer.writerows(data)
print(f"CSV file '{filename}' written to desktop successfully.")
Example usage:
data = [
['Name', 'Age', 'City'],
['Alice', 30, 'New York'],
['Bob', 25, 'Los Angeles']
]
write_csv_to_desktop_file('example.csv', data)
七、写入JSON文件
JSON文件是一种常见的用于数据交换的文件格式,可以使用json
模块来写入JSON文件。
import json
def write_json_to_desktop_file(filename, data):
desktop = get_desktop_path()
file_path = os.path.join(desktop, filename)
with open(file_path, 'w') as file:
json.dump(data, file, indent=4)
print(f"JSON file '{filename}' written to desktop successfully.")
Example usage:
data = {
'name': 'Alice',
'age': 30,
'city': 'New York'
}
write_json_to_desktop_file('example.json', data)
八、写入文件的最佳实践
在编写文件写入代码时,有一些最佳实践可以提高代码的健壮性和可维护性:
- 使用上下文管理器:上下文管理器(
with
语句)可以确保文件在操作完成后自动关闭。 - 处理异常:捕获并处理可能的异常情况,确保程序不会因为一个错误而崩溃。
- 路径拼接:使用
os.path.join()
进行路径拼接,以确保在不同操作系统下路径格式正确。 - 编码处理:明确指定文件编码(如
utf-8
),以避免编码问题。
def write_to_desktop_file(filename, content, mode='w', encoding='utf-8'):
desktop = get_desktop_path()
file_path = os.path.join(desktop, filename)
try:
with open(file_path, mode, encoding=encoding) as file:
file.write(content)
print(f"File '{filename}' written to desktop successfully.")
except Exception as e:
print(f"An error occurred while writing the file: {e}")
Example usage:
write_to_desktop_file('example.txt', 'Hello, this is a test content!')
九、总结
通过以上步骤和示例代码,我们了解了如何在Python中写入桌面文件。无论是文本文件、二进制文件、CSV文件还是JSON文件,都可以通过合理使用Python标准库中的os
、open
、csv
和json
模块来完成。通过处理不同操作系统的桌面路径、捕获异常、使用上下文管理器和明确编码等最佳实践,我们可以编写出健壮且跨平台的文件写入代码。
在实际开发中,写入文件是一个非常常见的操作,通过掌握这些方法和技巧,可以更高效地处理文件操作任务。希望本文对您在Python编程中处理文件写入有所帮助。
相关问答FAQs:
如何使用Python将数据写入桌面文件?
使用Python写入桌面文件的步骤主要包括确定桌面的文件路径、打开文件并进行写入操作。可以使用os
模块获取桌面的路径,然后利用open()
函数创建或打开文件。确保在写入前正确处理文件模式,例如使用'w'
进行写入,或者使用'a'
进行追加。
在Windows和Mac上如何找到桌面文件路径?
在Windows上,桌面的路径通常是C:\Users\<用户名>\Desktop
。在Mac上,路径为/Users/<用户名>/Desktop
。使用Python的os.path.expanduser('~/Desktop')
可以方便地获取当前用户的桌面路径,避免手动输入。
使用Python写入文件时需要注意哪些权限问题?
在写入文件前,确保当前用户对桌面有写入权限。在某些情况下,操作系统可能会限制某些目录的访问,特别是在企业环境中。如果遇到权限问题,可以尝试以管理员身份运行Python脚本,或者选择其他可写入的目录。
如何在写入文件时处理异常情况?
在进行文件写入操作时,使用try-except
语句来捕获潜在的异常情况,例如文件无法打开或写入失败。这样可以确保程序不会因错误而崩溃,并能提供相关的错误信息,帮助用户进行调试和修复。
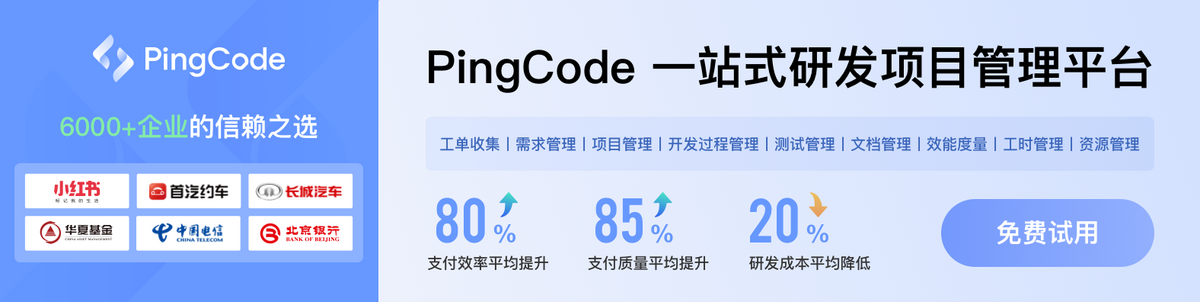