在Python中,写多行if语句可以通过使用多个if、elif和else语句来实现。 多行if语句的结构如下:
if condition1:
# Code to execute if condition1 is true
elif condition2:
# Code to execute if condition2 is true
else:
# Code to execute if none of the above conditions are true
详细描述:
在多行if语句中,可以使用if
语句检查第一个条件,如果该条件为真,则执行相应的代码块。如果第一个条件为假,则使用elif
语句检查下一个条件,依此类推。如果所有的条件都为假,则执行else
语句中的代码。通过这种方式,可以实现多条件的判断和相应的执行逻辑。
一、基础多行if语句
多行if语句是编程中常用的控制结构,用于根据不同条件执行不同的代码块。下面是一个简单的示例,展示如何在Python中使用多行if语句。
# Example of multi-line if statement
x = 10
if x < 0:
print("x is a negative number")
elif x == 0:
print("x is zero")
elif x > 0 and x < 10:
print("x is a positive single-digit number")
else:
print("x is a positive number with multiple digits")
在这个示例中,我们首先检查变量x
是否小于0,如果是,则输出"x is a negative number"。如果第一个条件不成立,则检查x
是否等于0,如果是,则输出"x is zero"。如果第二个条件也不成立,则继续检查x
是否在0和10之间,如果是,则输出"x is a positive single-digit number"。如果以上所有条件都不成立,则执行else
语句中的代码块,输出"x is a positive number with multiple digits"。
二、嵌套的if语句
有时候,我们可能需要在一个if语句内部嵌套另一个if语句,以实现更复杂的条件判断。下面是一个示例,展示如何在Python中嵌套if语句。
# Example of nested if statement
x = 15
if x > 0:
if x < 10:
print("x is a positive single-digit number")
else:
print("x is a positive number with multiple digits")
else:
print("x is zero or a negative number")
在这个示例中,我们首先检查变量x
是否大于0,如果是,则进入第一个if语句的代码块。在这个代码块中,我们再次使用if语句检查x
是否小于10,如果是,则输出"x is a positive single-digit number"。否则,输出"x is a positive number with multiple digits"。如果第一个if语句的条件不成立,则执行else
语句中的代码块,输出"x is zero or a negative number"。
三、使用逻辑运算符
在多行if语句中,我们可以使用逻辑运算符(如and
、or
和not
)来组合多个条件。下面是一个示例,展示如何在Python中使用逻辑运算符。
# Example of using logical operators
x = 7
if x > 0 and x < 10:
print("x is a positive single-digit number")
elif x >= 10 and x < 100:
print("x is a positive two-digit number")
else:
print("x is either zero, negative, or a number with more than two digits")
在这个示例中,我们使用and
运算符来组合多个条件。首先,我们检查变量x
是否大于0且小于10,如果是,则输出"x is a positive single-digit number"。如果第一个条件不成立,则检查x
是否在10到100之间,如果是,则输出"x is a positive two-digit number"。如果以上所有条件都不成立,则执行else
语句中的代码块,输出"x is either zero, negative, or a number with more than two digits"。
四、使用函数和多行if语句
在实际编程中,我们通常将多行if语句放在函数中,以提高代码的可读性和重用性。下面是一个示例,展示如何在函数中使用多行if语句。
def check_number(x):
if x < 0:
return "x is a negative number"
elif x == 0:
return "x is zero"
elif x > 0 and x < 10:
return "x is a positive single-digit number"
else:
return "x is a positive number with multiple digits"
Call the function and print the result
result = check_number(5)
print(result)
在这个示例中,我们定义了一个名为check_number
的函数,该函数接受一个参数x
并返回一个字符串。函数内部使用多行if语句来检查x
的值,并根据不同的条件返回相应的字符串。最后,我们调用check_number
函数并打印结果。
五、多行if语句中的常见错误和调试
在编写多行if语句时,容易出现一些常见的错误。了解这些错误并知道如何调试它们,可以帮助我们编写更健壮的代码。
1. 缩进错误:
Python依赖缩进来表示代码块。如果缩进不一致,可能会导致语法错误或逻辑错误。例如:
x = 10
if x > 0:
print("x is a positive number") # Incorrect indentation
解决方法: 确保所有代码块的缩进一致,通常是4个空格或1个制表符。
x = 10
if x > 0:
print("x is a positive number")
2. 忘记使用逻辑运算符:
在条件判断中,忘记使用逻辑运算符可能会导致意外的结果。例如:
x = 5
if x > 0 x < 10: # Missing 'and' operator
print("x is a positive single-digit number")
解决方法: 使用正确的逻辑运算符来组合多个条件。
x = 5
if x > 0 and x < 10:
print("x is a positive single-digit number")
3. 不必要的代码重复:
在多行if语句中,避免不必要的代码重复。例如:
x = 5
if x > 0:
if x < 10:
print("x is a positive single-digit number")
else:
print("x is a positive number with multiple digits")
else:
print("x is zero or a negative number")
解决方法: 尽量简化代码,避免多余的嵌套。
x = 5
if x > 0 and x < 10:
print("x is a positive single-digit number")
elif x >= 10:
print("x is a positive number with multiple digits")
else:
print("x is zero or a negative number")
六、多行if语句的最佳实践
为了编写高效、易读和可维护的多行if语句,遵循一些最佳实践是非常重要的。
1. 使用有意义的变量名:
确保变量名具有描述性,以便代码更易读。例如:
age = 25
if age >= 18:
print("Adult")
else:
print("Minor")
2. 避免深层嵌套:
深层嵌套的if语句会使代码难以阅读和维护。尝试使用逻辑运算符或将代码重构为函数,以简化逻辑。
def check_age(age):
if age < 0:
return "Invalid age"
elif age < 18:
return "Minor"
elif age < 65:
return "Adult"
else:
return "Senior"
result = check_age(25)
print(result)
3. 使用链式比较:
Python支持链式比较,可以使代码更简洁。例如:
x = 5
if 0 < x < 10:
print("x is a positive single-digit number")
4. 提前返回:
在函数中使用多行if语句时,提前返回可以减少嵌套层次。例如:
def check_number(x):
if x < 0:
return "x is a negative number"
if x == 0:
return "x is zero"
if x > 0 and x < 10:
return "x is a positive single-digit number"
return "x is a positive number with multiple digits"
result = check_number(5)
print(result)
七、实际应用示例
为了更好地理解多行if语句的应用,我们来看一些实际的示例。
1. 成绩分类:
根据学生的分数,将其分类为“优秀”、“良好”、“及格”或“不及格”。
def classify_grade(score):
if score < 0 or score > 100:
return "Invalid score"
elif score >= 90:
return "优秀"
elif score >= 75:
return "良好"
elif score >= 60:
return "及格"
else:
return "不及格"
grade = classify_grade(85)
print(f"学生的成绩是:{grade}")
2. 温度转换:
根据输入的温度值和单位,转换为另一种温度单位(摄氏度和华氏度)。
def convert_temperature(value, unit):
if unit == "C":
return value * 9/5 + 32 # Convert Celsius to Fahrenheit
elif unit == "F":
return (value - 32) * 5/9 # Convert Fahrenheit to Celsius
else:
return "Invalid unit"
temp_c = convert_temperature(25, "C")
temp_f = convert_temperature(77, "F")
print(f"25摄氏度等于{temp_c}华氏度")
print(f"77华氏度等于{temp_f}摄氏度")
3. 简单的计算器:
根据用户输入的操作符和两个操作数,执行相应的算术运算。
def simple_calculator(num1, num2, operator):
if operator == "+":
return num1 + num2
elif operator == "-":
return num1 - num2
elif operator == "*":
return num1 * num2
elif operator == "/":
if num2 == 0:
return "Division by zero is not allowed"
return num1 / num2
else:
return "Invalid operator"
result_add = simple_calculator(10, 5, "+")
result_div = simple_calculator(10, 0, "/")
print(f"10 + 5 = {result_add}")
print(f"10 / 0 = {result_div}")
八、总结
多行if语句是Python编程中非常重要的控制结构,用于根据不同的条件执行不同的代码块。 在使用多行if语句时,遵循一些最佳实践,如使用有意义的变量名、避免深层嵌套、使用链式比较和提前返回,可以编写出更加简洁、易读和可维护的代码。通过实际应用示例,我们可以看到多行if语句在各种场景中的应用,如成绩分类、温度转换和简单的计算器。
在编写和调试多行if语句时,注意常见的错误,并使用调试工具和方法来排查和解决问题,可以提高编程效率和代码质量。总之,掌握多行if语句的使用技巧和最佳实践,将有助于我们编写出更加高效和健壮的Python程序。
相关问答FAQs:
如何在Python中使用多行if语句?
在Python中,多行if语句通常是通过使用缩进和冒号来实现的。可以通过使用if、elif和else语句组合来处理复杂的条件。在需要的情况下,可以将多个条件放在一行中,但在条件过多或逻辑较复杂的情况下,使用多行可提高代码的可读性。例如:
if condition1:
# 执行操作1
elif condition2:
# 执行操作2
else:
# 执行默认操作
在Python中,怎样使用逻辑运算符来简化多行if?
逻辑运算符如and
和or
可以帮助您在单个if语句中组合多个条件,从而减少代码行数。然而,过于复杂的条件可能会影响代码的可读性。因此,适当使用这些运算符可以使代码更加简洁。示例:
if condition1 and condition2:
# 满足所有条件时执行的操作
如何在Python中处理嵌套的if语句?
嵌套的if语句允许在一个if语句内部再包含一个if语句。这在需要根据多个条件进行复杂判断时非常有用。虽然嵌套的if语句可以实现更复杂的逻辑,但应当注意保持代码的可读性。示例:
if condition1:
if condition2:
# 执行操作1
else:
# 执行操作2
else:
# 执行操作3
通过合理使用多行if语句,您可以有效地处理复杂的条件判断。
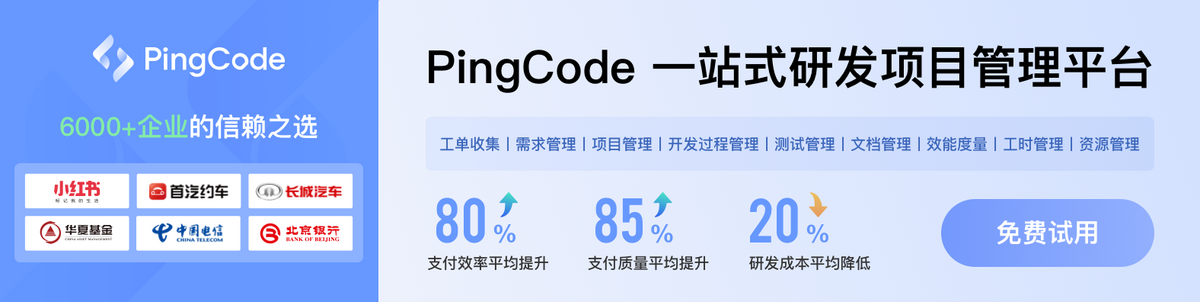