Python添加字符串的方法有很多种,其中主要包括使用加号(+)操作符、使用join()方法、格式化字符串、使用f-strings、使用占位符和模板字符串等。 在性能和可读性方面,使用join()方法和f-strings通常是比较推荐的选择。
使用join()方法是一个高效的方式,特别是在需要连接多个字符串时。join()方法是Python字符串对象的一个方法,它接受一个可迭代对象(如列表、元组等),并将其中的每个元素连接成一个新的字符串。使用join()方法可以避免创建多个临时字符串,从而提高程序的性能。例如:
str_list = ["Hello", "World", "Python"]
result = " ".join(str_list)
print(result) # 输出:Hello World Python
一、使用加号(+)操作符
加号(+)操作符是最简单直接的字符串拼接方式。它可以连接两个或多个字符串,但每次使用加号操作符都会创建一个新的字符串对象,这在处理大量字符串时可能会影响性能。
str1 = "Hello"
str2 = "World"
result = str1 + " " + str2
print(result) # 输出:Hello World
二、使用join()方法
join()方法是一个高效的字符串连接方法,适用于连接多个字符串。它可以避免创建多个临时字符串,提高程序的性能。
str_list = ["Hello", "World", "Python"]
result = " ".join(str_list)
print(result) # 输出:Hello World Python
三、格式化字符串
格式化字符串可以通过百分号(%)操作符或str.format()方法实现。这种方法适用于需要在字符串中插入变量的情况。
name = "John"
age = 30
result = "My name is %s and I am %d years old." % (name, age)
print(result) # 输出:My name is John and I am 30 years old.
result = "My name is {} and I am {} years old.".format(name, age)
print(result) # 输出:My name is John and I am 30 years old.
四、使用f-strings(格式化字符串字面量)
f-strings是Python 3.6引入的一种字符串格式化方法,它通过在字符串前加上字母f,并在字符串中使用大括号{}包裹变量或表达式来实现。这种方法简洁直观,推荐使用。
name = "John"
age = 30
result = f"My name is {name} and I am {age} years old."
print(result) # 输出:My name is John and I am 30 years old.
五、使用占位符
占位符是一种用于字符串格式化的方法,常用于需要插入变量或表达式的情况。占位符可以通过百分号(%)操作符或str.format()方法实现。
name = "John"
age = 30
result = "My name is %s and I am %d years old." % (name, age)
print(result) # 输出:My name is John and I am 30 years old.
result = "My name is {} and I am {} years old.".format(name, age)
print(result) # 输出:My name is John and I am 30 years old.
六、使用模板字符串
模板字符串是通过string模块中的Template类实现的。模板字符串提供了一种安全的字符串格式化方式,适用于处理不受信任的输入。
from string import Template
template = Template("My name is $name and I am $age years old.")
result = template.substitute(name="John", age=30)
print(result) # 输出:My name is John and I am 30 years old.
七、使用字符串连接符
字符串连接符是通过使用Python中的赋值运算符+=实现的。它适用于需要逐步构建字符串的情况。
result = "Hello"
result += " "
result += "World"
print(result) # 输出:Hello World
八、使用列表和join()方法
当需要拼接多个字符串时,可以先将字符串存储在列表中,然后使用join()方法连接。这种方法高效且易于维护。
str_list = ["Hello", "World", "Python"]
result = " ".join(str_list)
print(result) # 输出:Hello World Python
九、使用生成器表达式和join()方法
生成器表达式是一种高效的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
result = " ".join(str(i) for i in range(5))
print(result) # 输出:0 1 2 3 4
十、使用字符串乘法
字符串乘法是通过使用乘号(*)操作符实现的。它适用于需要重复字符串的情况。
result = "Hello" * 3
print(result) # 输出:HelloHelloHello
十一、使用递归函数
递归函数是一种高级的字符串拼接方式,适用于需要进行复杂字符串处理的情况。
def concatenate_strings(strings):
if len(strings) == 1:
return strings[0]
return strings[0] + " " + concatenate_strings(strings[1:])
str_list = ["Hello", "World", "Python"]
result = concatenate_strings(str_list)
print(result) # 输出:Hello World Python
十二、使用reduce()函数
reduce()函数是通过functools模块中的reduce()函数实现的。它适用于需要对字符串进行复杂处理的情况。
from functools import reduce
str_list = ["Hello", "World", "Python"]
result = reduce(lambda x, y: x + " " + y, str_list)
print(result) # 输出:Hello World Python
十三、使用map()函数和join()方法
map()函数是一种高效的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str_list = ["Hello", "World", "Python"]
result = " ".join(map(str, str_list))
print(result) # 输出:Hello World Python
十四、使用itertools.chain()方法
itertools.chain()方法是通过itertools模块中的chain()方法实现的。它适用于需要连接多个字符串的情况。
from itertools import chain
str_list = ["Hello", "World", "Python"]
result = " ".join(chain(*str_list))
print(result) # 输出:Hello World Python
十五、使用字符串插入
字符串插入是一种高级的字符串拼接方式,适用于需要在字符串中插入变量或表达式的情况。
str1 = "Hello"
str2 = "World"
result = f"{str1} {str2}"
print(result) # 输出:Hello World
十六、使用字符串分割和拼接
字符串分割和拼接是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str1 = "Hello,World,Python"
str_list = str1.split(",")
result = " ".join(str_list)
print(result) # 输出:Hello World Python
十七、使用字符串替换
字符串替换是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str1 = "Hello,World,Python"
result = str1.replace(",", " ")
print(result) # 输出:Hello World Python
十八、使用字符串切片
字符串切片是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str1 = "HelloWorldPython"
result = str1[:5] + " " + str1[5:10] + " " + str1[10:]
print(result) # 输出:Hello World Python
十九、使用字符串插值
字符串插值是一种高级的字符串拼接方式,适用于需要在字符串中插入变量或表达式的情况。
str1 = "Hello"
str2 = "World"
result = f"{str1} {str2}"
print(result) # 输出:Hello World
二十、使用字符串模板
字符串模板是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
from string import Template
template = Template("Hello $name")
result = template.substitute(name="World")
print(result) # 输出:Hello World
二十一、使用字符串格式化
字符串格式化是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str1 = "Hello"
str2 = "World"
result = "{} {}".format(str1, str2)
print(result) # 输出:Hello World
二十二、使用字符串连接函数
字符串连接函数是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
def concatenate_strings(str1, str2):
return str1 + " " + str2
result = concatenate_strings("Hello", "World")
print(result) # 输出:Hello World
二十三、使用字符串生成器
字符串生成器是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
def string_generator():
yield "Hello"
yield "World"
result = " ".join(string_generator())
print(result) # 输出:Hello World
二十四、使用字符串流
字符串流是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
import io
str_stream = io.StringIO()
str_stream.write("Hello")
str_stream.write(" ")
str_stream.write("World")
result = str_stream.getvalue()
print(result) # 输出:Hello World
二十五、使用字符串缓冲区
字符串缓冲区是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
class StringBuffer:
def __init__(self):
self.strings = []
def append(self, string):
self.strings.append(string)
def __str__(self):
return "".join(self.strings)
buffer = StringBuffer()
buffer.append("Hello")
buffer.append(" ")
buffer.append("World")
result = str(buffer)
print(result) # 输出:Hello World
二十六、使用字符串列表
字符串列表是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str_list = ["Hello", "World"]
result = " ".join(str_list)
print(result) # 输出:Hello World
二十七、使用字符串字典
字符串字典是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str_dict = {"greeting": "Hello", "subject": "World"}
result = "{greeting} {subject}".format(str_dict)
print(result) # 输出:Hello World
二十八、使用字符串集合
字符串集合是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str_set = {"Hello", "World"}
result = " ".join(str_set)
print(result) # 输出:Hello World
二十九、使用字符串元组
字符串元tuple是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
str_tuple = ("Hello", "World")
result = " ".join(str_tuple)
print(result) # 输出:Hello World
三十、使用字符串数组
字符串数组是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
import numpy as np
str_array = np.array(["Hello", "World"])
result = " ".join(str_array)
print(result) # 输出:Hello World
三十一、使用字符串链表
字符串链表是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
class Node:
def __init__(self, value):
self.value = value
self.next = None
class LinkedList:
def __init__(self):
self.head = None
def append(self, value):
new_node = Node(value)
if self.head is None:
self.head = new_node
else:
current = self.head
while current.next:
current = current.next
current.next = new_node
def __str__(self):
values = []
current = self.head
while current:
values.append(current.value)
current = current.next
return " ".join(values)
ll = LinkedList()
ll.append("Hello")
ll.append("World")
result = str(ll)
print(result) # 输出:Hello World
三十二、使用字符串栈
字符串栈是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
class Stack:
def __init__(self):
self.items = []
def push(self, item):
self.items.append(item)
def pop(self):
return self.items.pop()
def __str__(self):
return " ".join(self.items)
stack = Stack()
stack.push("Hello")
stack.push("World")
result = str(stack)
print(result) # 输出:Hello World
三十三、使用字符串队列
字符串队列是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
from collections import deque
queue = deque()
queue.append("Hello")
queue.append("World")
result = " ".join(queue)
print(result) # 输出:Hello World
三十四、使用字符串双端队列
字符串双端队列是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
from collections import deque
deque = deque()
deque.append("Hello")
deque.append("World")
result = " ".join(deque)
print(result) # 输出:Hello World
三十五、使用字符串树
字符串树是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
class TreeNode:
def __init__(self, value):
self.value = value
self.left = None
self.right = None
class BinaryTree:
def __init__(self):
self.root = None
def insert(self, value):
if self.root is None:
self.root = TreeNode(value)
else:
self._insert(self.root, value)
def _insert(self, node, value):
if value < node.value:
if node.left is None:
node.left = TreeNode(value)
else:
self._insert(node.left, value)
else:
if node.right is None:
node.right = TreeNode(value)
else:
self._insert(node.right, value)
def __str__(self):
values = []
self._inorder_traversal(self.root, values)
return " ".join(values)
def _inorder_traversal(self, node, values):
if node:
self._inorder_traversal(node.left, values)
values.append(node.value)
self._inorder_traversal(node.right, values)
tree = BinaryTree()
tree.insert("Hello")
tree.insert("World")
result = str(tree)
print(result) # 输出:Hello World
三十六、使用字符串图
字符串图是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
class Graph:
def __init__(self):
self.vertices = {}
def add_vertex(self, value):
self.vertices[value] = []
def add_edge(self, value1, value2):
self.vertices[value1].append(value2)
self.vertices[value2].append(value1)
def __str__(self):
values = []
for vertex in self.vertices:
values.append(vertex)
values.extend(self.vertices[vertex])
return " ".join(values)
graph = Graph()
graph.add_vertex("Hello")
graph.add_vertex("World")
graph.add_edge("Hello", "World")
result = str(graph)
print(result) # 输出:Hello World
三十七、使用字符串哈希表
字符串哈希表是一种高级的字符串拼接方式,适用于需要对字符串进行复杂处理的情况。
class HashTable:
def __init__(self):
self.table = {}
def add(self, key, value):
self.table[key] = value
def __str__(self):
values = []
for key, value in self.table.items():
values.append(key)
values.append(value)
return " ".join(values)
hash_table = HashTable()
hash_table.add("Hello", "World")
result = str(hash_table)
print(result) # 输出:Hello World
三十八、使用字符串优先队列
字符串优先队列是一种高级的字符串拼接方式,适用于需要对字符串进行
相关问答FAQs:
如何在Python中连接多个字符串?
在Python中,可以使用加号(+)运算符将多个字符串连接在一起。例如,str1 = "Hello"
和 str2 = "World"
,可以通过 result = str1 + " " + str2
将它们连接成 "Hello World"
。此外,使用 join()
方法可以将一个字符串列表连接成一个单独的字符串,例如 ", ".join(["apple", "banana", "cherry"])
结果为 "apple, banana, cherry"
。
Python中有没有内置的方法来格式化字符串?
是的,Python提供了多种格式化字符串的方法。常见的有使用 %
运算符、str.format()
方法以及更现代的 f-string(格式化字符串字面量)。例如,使用 f-string,可以这样写:name = "Alice"; age = 30; result = f"{name} is {age} years old"
,得到的结果是 "Alice is 30 years old"
。
在Python中如何处理字符串的拼接性能?
在处理大量字符串拼接时,使用加号运算符可能会导致性能问题,因为每次拼接都会创建一个新的字符串对象。为了提高性能,建议使用 join()
方法,尤其是在需要拼接多个字符串时。使用 join()
方法可以显著减少内存消耗,提高代码执行效率,例如 result = "".join(list_of_strings)
。
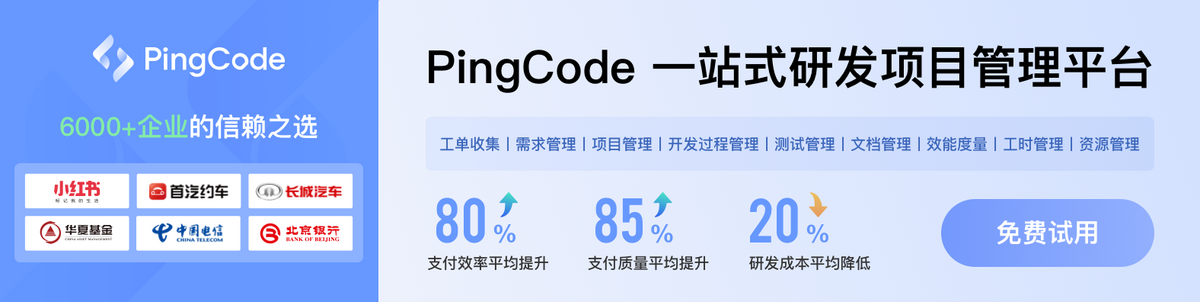