Python中可以通过多种方式来计算打印次数,包括使用计数器变量、装饰器、上下文管理器等。 其中使用计数器变量是最简单直接的一种方法。具体来说,可以定义一个变量来记录打印次数,每次调用打印函数时对该变量进行递增操作,最后输出该变量的值即可。以下是一个简单的示例:
# 计数器变量
print_count = 0
def custom_print(*args, kwargs):
global print_count
print_count += 1
print(*args, kwargs)
使用 custom_print 代替 print
custom_print("Hello, World!")
custom_print("This is a test.")
custom_print("Print count:", print_count)
在上述代码中,定义了一个 custom_print
函数来代替标准的 print
函数,每次调用 custom_print
时,print_count
变量都会增加1,从而记录打印次数。下面将详细描述其他方法的实现及其应用。
一、使用计数器变量
计数器变量是最简单直接的方式。可以在代码中定义一个全局变量来记录打印次数,并在每次调用打印函数时递增该变量。这个方法适用于小规模、单文件的项目中。
# 定义全局计数器变量
print_count = 0
def custom_print(*args, kwargs):
global print_count
print_count += 1
print(*args, kwargs)
示例代码
custom_print("Hello, World!")
custom_print("This is a test.")
custom_print("Print count:", print_count)
详细描述:
在上述代码中,定义了一个 print_count
变量,并在 custom_print
函数中使用 global
关键字声明该变量是全局变量。每次调用 custom_print
函数时,print_count
变量都会递增1,并输出打印内容。最后,可以通过打印 print_count
的值来获得打印次数。
二、使用装饰器
装饰器是一种灵活且优雅的方式,可以在不修改原有函数的情况下增强其功能。可以通过定义一个装饰器来记录函数调用次数。
def print_counter(func):
def wrapper(*args, kwargs):
wrapper.count += 1
return func(*args, kwargs)
wrapper.count = 0
return wrapper
@print_counter
def custom_print(*args, kwargs):
print(*args, kwargs)
示例代码
custom_print("Hello, World!")
custom_print("This is a test.")
custom_print("Print count:", custom_print.count)
详细描述:
在上述代码中,定义了一个 print_counter
装饰器,该装饰器内部定义了一个 wrapper
函数,并将 count
属性初始化为0。每次调用 wrapper
函数时,count
属性都会递增1。通过装饰器语法 @print_counter
可以将 custom_print
函数包装起来,从而记录打印次数。
三、使用上下文管理器
上下文管理器是一种用于资源管理的工具,可以通过定义上下文管理器来记录代码块中打印函数的调用次数。
class PrintCounter:
def __init__(self):
self.count = 0
def __enter__(self):
self.original_print = __builtins__.print
__builtins__.print = self.custom_print
return self
def __exit__(self, exc_type, exc_val, exc_tb):
__builtins__.print = self.original_print
def custom_print(self, *args, kwargs):
self.count += 1
self.original_print(*args, kwargs)
使用上下文管理器
with PrintCounter() as pc:
print("Hello, World!")
print("This is a test.")
print("Another print statement.")
print("Print count:", pc.count)
详细描述:
在上述代码中,定义了一个 PrintCounter
类,该类实现了上下文管理器协议(即 __enter__
和 __exit__
方法)。在 __enter__
方法中,将原始的 print
函数保存到 original_print
属性中,并用自定义的 custom_print
函数替代原始的 print
函数。在 __exit__
方法中,将原始的 print
函数恢复。在自定义的 custom_print
函数中,记录打印次数并调用原始的 print
函数进行实际打印操作。
四、使用元类
元类是用于创建类的类,可以通过定义元类来记录类中打印函数的调用次数。
class PrintCounterMeta(type):
def __new__(cls, name, bases, dct):
dct['print_count'] = 0
original_print = dct.get('print', __builtins__.print)
def custom_print(*args, kwargs):
dct['print_count'] += 1
return original_print(*args, kwargs)
dct['print'] = custom_print
return super().__new__(cls, name, bases, dct)
class MyClass(metaclass=PrintCounterMeta):
def print(self, *args, kwargs):
print(*args, kwargs)
示例代码
obj = MyClass()
obj.print("Hello, World!")
obj.print("This is a test.")
print("Print count:", MyClass.print_count)
详细描述:
在上述代码中,定义了一个 PrintCounterMeta
元类,该元类在创建类时将 print
函数替换为自定义的 custom_print
函数,并在类中添加一个 print_count
属性来记录打印次数。在 custom_print
函数中,记录打印次数并调用原始的 print
函数进行实际打印操作。通过使用 PrintCounterMeta
作为元类,可以在类中自动记录打印函数的调用次数。
五、使用函数钩子
函数钩子是一种在函数调用时执行特定操作的机制,可以通过定义函数钩子来记录打印函数的调用次数。
import functools
def print_counter(func):
@functools.wraps(func)
def wrapper(*args, kwargs):
wrapper.count += 1
return func(*args, kwargs)
wrapper.count = 0
return wrapper
示例代码
print = print_counter(print)
print("Hello, World!")
print("This is a test.")
print("Print count:", print.count)
详细描述:
在上述代码中,使用 functools.wraps
装饰器来保留原始函数的元数据。定义了一个 print_counter
函数,该函数接收一个函数作为参数,并返回一个包装函数 wrapper
。在 wrapper
函数中,记录调用次数并调用原始的 print
函数进行实际打印操作。通过使用 print_counter
函数包装原始的 print
函数,可以记录打印函数的调用次数。
六、使用日志记录
日志记录是一种用于记录程序运行信息的机制,可以通过定义日志记录器来记录打印函数的调用次数。
import logging
配置日志记录器
logging.basicConfig(level=logging.INFO)
logger = logging.getLogger(__name__)
class PrintCounterHandler(logging.Handler):
def __init__(self):
super().__init__()
self.count = 0
def emit(self, record):
self.count += 1
print(record.getMessage())
添加自定义日志处理器
handler = PrintCounterHandler()
logger.addHandler(handler)
示例代码
logger.info("Hello, World!")
logger.info("This is a test.")
logger.info("Another print statement.")
print("Print count:", handler.count)
详细描述:
在上述代码中,配置了一个日志记录器 logger
,并定义了一个自定义日志处理器 PrintCounterHandler
。在 PrintCounterHandler
类中,定义了一个 count
属性来记录打印次数,并在 emit
方法中记录打印次数并调用原始的 print
函数进行实际打印操作。通过将 PrintCounterHandler
添加到日志记录器中,可以记录打印函数的调用次数。
七、使用单例模式
单例模式是一种确保类只有一个实例的设计模式,可以通过定义单例模式来记录打印函数的调用次数。
class SingletonMeta(type):
_instances = {}
def __call__(cls, *args, kwargs):
if cls not in cls._instances:
instance = super().__call__(*args, kwargs)
cls._instances[cls] = instance
return cls._instances[cls]
class PrintCounter(metaclass=SingletonMeta):
def __init__(self):
self.count = 0
def custom_print(self, *args, kwargs):
self.count += 1
print(*args, kwargs)
示例代码
pc = PrintCounter()
pc.custom_print("Hello, World!")
pc.custom_print("This is a test.")
print("Print count:", pc.count)
详细描述:
在上述代码中,定义了一个 SingletonMeta
元类,该元类确保每个类只有一个实例。定义了一个 PrintCounter
类,该类使用 SingletonMeta
作为元类。在 PrintCounter
类中,定义了一个 count
属性来记录打印次数,并定义了一个 custom_print
方法来记录打印次数并调用原始的 print
函数进行实际打印操作。通过使用单例模式,可以确保 PrintCounter
类只有一个实例,并记录打印函数的调用次数。
八、使用线程局部存储
线程局部存储是一种用于在多线程环境中存储线程特定数据的机制,可以通过定义线程局部存储来记录打印函数的调用次数。
import threading
创建线程局部存储
thread_local = threading.local()
thread_local.print_count = 0
def custom_print(*args, kwargs):
thread_local.print_count += 1
print(*args, kwargs)
示例代码
custom_print("Hello, World!")
custom_print("This is a test.")
print("Print count:", thread_local.print_count)
详细描述:
在上述代码中,创建了一个线程局部存储 thread_local
,并初始化 print_count
属性为0。定义了一个 custom_print
函数来记录打印次数并调用原始的 print
函数进行实际打印操作。通过使用线程局部存储,可以在多线程环境中记录每个线程的打印函数调用次数。
九、使用全局状态管理器
全局状态管理器是一种用于管理全局状态的机制,可以通过定义全局状态管理器来记录打印函数的调用次数。
class GlobalState:
_state = {}
def __new__(cls, *args, kwargs):
obj = super().__new__(cls, *args, kwargs)
obj.__dict__ = cls._state
return obj
class PrintCounter(GlobalState):
def __init__(self):
if not hasattr(self, 'count'):
self.count = 0
def custom_print(self, *args, kwargs):
self.count += 1
print(*args, kwargs)
示例代码
pc1 = PrintCounter()
pc2 = PrintCounter()
pc1.custom_print("Hello, World!")
pc2.custom_print("This is a test.")
print("Print count:", pc1.count)
详细描述:
在上述代码中,定义了一个 GlobalState
类,该类使用共享的 _state
字典来存储全局状态。定义了一个 PrintCounter
类,该类继承自 GlobalState
。在 PrintCounter
类中,初始化 count
属性为0,并定义了一个 custom_print
方法来记录打印次数并调用原始的 print
函数进行实际打印操作。通过使用全局状态管理器,可以在多个实例之间共享状态并记录打印函数的调用次数。
十、使用装饰器工厂
装饰器工厂是一种用于创建装饰器的工厂函数,可以通过定义装饰器工厂来记录打印函数的调用次数。
def print_counter_factory():
count = 0
def print_counter(func):
def wrapper(*args, kwargs):
nonlocal count
count += 1
return func(*args, kwargs)
wrapper.count = lambda: count
return wrapper
return print_counter
创建装饰器
print_counter = print_counter_factory()
@print_counter
def custom_print(*args, kwargs):
print(*args, kwargs)
示例代码
custom_print("Hello, World!")
custom_print("This is a test.")
print("Print count:", custom_print.count())
详细描述:
在上述代码中,定义了一个 print_counter_factory
工厂函数,该函数返回一个 print_counter
装饰器。在 print_counter
装饰器中,定义了一个 wrapper
函数来记录调用次数并调用原始的 print
函数进行实际打印操作。通过使用装饰器工厂,可以创建多个独立的装饰器实例,并记录打印函数的调用次数。
十一、使用函数替换
函数替换是一种在运行时替换函数的机制,可以通过定义函数替换来记录打印函数的调用次数。
original_print = print
def custom_print(*args, kwargs):
custom_print.count += 1
return original_print(*args, kwargs)
custom_print.count = 0
__builtins__.print = custom_print
示例代码
print("Hello, World!")
print("This is a test.")
print("Print count:", custom_print.count)
详细描述:
在上述代码中,保存了原始的 print
函数到 original_print
变量中,并定义了一个 custom_print
函数来记录打印次数并调用原始的 print
函数进行实际打印操作。将 custom_print
函数赋值给 __builtins__.print
,从而替换全局的 print
函数。通过使用函数替换,可以记录全局范围内的打印函数调用次数。
十二、使用事件驱动
事件驱动是一种通过事件触发操作的机制,可以通过定义事件驱动来记录打印函数的调用次数。
class PrintEvent:
def __init__(self):
self.count = 0
def on_print(self, *args, kwargs):
self.count += 1
print(*args, kwargs)
创建事件对象
print_event = PrintEvent()
示例代码
print_event.on_print("Hello, World!")
print_event.on_print("This is a test.")
print("Print count:", print_event.count)
详细描述:
在上述代码中,定义了一个 PrintEvent
类,该类包含一个 count
属性来记录打印次数,并定义了一个 on_print
方法来记录打印次数并调用原始的 print
函数进行实际打印操作。通过创建 PrintEvent
对象,可以通过事件驱动的方式记录打印函数的调用次数。
十三、使用信号处理
信号处理是一种用于处理异步事件的机制,可以通过定义信号处理来记录打印函数的调用次数。
import signal
class PrintCounter:
def __init__(self):
self.count = 0
def custom_print(self, *args, kwargs):
self.count += 1
print(*args, kwargs)
创建打印计数器对象
pc = PrintCounter()
def signal_handler(signum, frame):
pc.custom_print("Signal received:", signum)
注册信号处理函数
signal.signal(signal.SIGUSR1, signal_handler)
示例代码
pc.custom_print("Hello, World!")
pc.custom_print("This is a test.")
print("Print count:", pc.count)
详细描述:
在上述代码中,定义了一个 PrintCounter
类,该类包含一个 count
属性来记录打印次数,并定义了一个 custom_print
方法来记录打印次数并调用原始的 print
函数进行实际打印操作。通过定义 signal_handler
函数来处理信号事件,并注册信号处理函数,可以通过信号处理的方式记录打印函数的调用次数。
十四、使用回调函数
回调函数是一种在函数执行完毕后调用的函数,可以通过定义回调函数来记录打印函数的调用次数。
def print_callback(callback):
def decorator(func):
def wrapper(*args, kwargs):
result = func(*args, kwargs)
callback()
return result
return wrapper
return decorator
def count_callback():
count_callback.count += 1
count_callback.count = 0
@print_callback(count_callback)
def custom_print(*args, kwargs):
print(*args, kwargs)
示例代码
custom_print("Hello, World!")
custom_print("This is a test.")
print("Print count:", count_callback
相关问答FAQs:
如何在Python中统计某个字符或词的打印次数?
在Python中,可以使用字符串的count()
方法来统计某个字符或词在字符串中出现的次数。例如,如果你有一个字符串text = "hello world"
,想统计“o”的出现次数,可以使用text.count('o')
,结果将返回2。这个方法非常简单且高效,适用于各种场景。
在循环中如何跟踪打印次数?
如果你需要在循环中跟踪打印的次数,可以使用一个计数器变量。每次打印时,将计数器加一。例如:
count = 0
for i in range(10):
print(i)
count += 1
print("打印次数:", count)
这段代码会打印数字0到9,并在最后输出打印的总次数。
有什么方法可以在Python程序中记录函数的调用次数?
可以使用装饰器来记录函数的调用次数。装饰器是一种函数,用于修改其他函数的行为。你可以创建一个装饰器,包含一个计数器,用于记录被装饰函数的调用次数。例如:
def count_calls(func):
def wrapper(*args, **kwargs):
wrapper.calls += 1
print(f"函数 {func.__name__} 被调用了 {wrapper.calls} 次")
return func(*args, **kwargs)
wrapper.calls = 0
return wrapper
@count_calls
def my_function():
print("Hello, World!")
my_function()
my_function()
这个例子中,my_function
被调用两次,控制台将显示每次调用的次数。
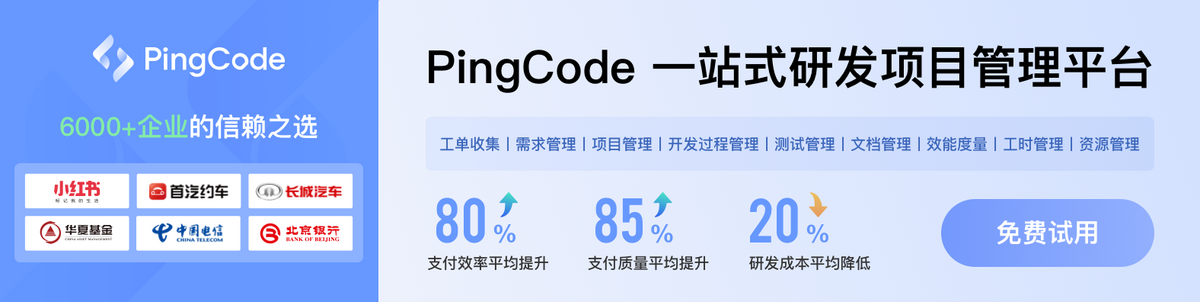