使用Python编写宠物程序可以通过定义类、创建实例、实现方法等步骤来完成。、我们需要利用面向对象编程来定义宠物的属性和行为。、通过用户交互来操控宠物的行为和状态。、可以添加更多功能使程序更具互动性和趣味性。
下面我们将详细展开这几点内容:
一、定义宠物类
在Python中,类是创建对象的蓝图。我们可以通过类来定义宠物的属性(如名字、年龄、健康状态等)和行为(如吃饭、玩耍、睡觉等)。
class Pet:
def __init__(self, name, age):
self.name = name
self.age = age
self.happiness = 50
self.hunger = 50
def eat(self, food):
self.hunger -= food
self.happiness += food
if self.hunger < 0:
self.hunger = 0
if self.happiness > 100:
self.happiness = 100
def play(self, time):
self.happiness += time
if self.happiness > 100:
self.happiness = 100
def sleep(self, hours):
self.happiness += hours
if self.happiness > 100:
self.happiness = 100
def status(self):
print(f"{self.name} is {self.age} years old. Happiness: {self.happiness}, Hunger: {self.hunger}")
二、创建宠物实例
通过定义的类,我们可以创建宠物实例,并对其进行操作。
my_pet = Pet("Buddy", 2)
my_pet.status()
my_pet.eat(20)
my_pet.status()
my_pet.play(10)
my_pet.status()
my_pet.sleep(5)
my_pet.status()
三、用户交互
为了让程序更加互动,可以通过用户输入来控制宠物的行为。我们可以使用while循环来持续运行程序,直到用户选择退出。
def main():
name = input("What is your pet's name? ")
age = int(input("How old is your pet? "))
pet = Pet(name, age)
while True:
print("\n1. Feed")
print("2. Play")
print("3. Sleep")
print("4. Check Status")
print("5. Exit")
choice = input("Choose an action: ")
if choice == '1':
food = int(input("How much food? "))
pet.eat(food)
elif choice == '2':
time = int(input("How long to play? "))
pet.play(time)
elif choice == '3':
hours = int(input("How many hours to sleep? "))
pet.sleep(hours)
elif choice == '4':
pet.status()
elif choice == '5':
break
else:
print("Invalid choice, please try again.")
if __name__ == "__main__":
main()
四、添加更多功能
为了使宠物程序更加丰富,我们可以添加更多的功能,例如:
1、健康状态管理:
通过增加健康属性,并添加生病和治疗的方法来管理宠物的健康状态。
class Pet:
def __init__(self, name, age):
self.name = name
self.age = age
self.happiness = 50
self.hunger = 50
self.health = 100
def eat(self, food):
self.hunger -= food
self.happiness += food
if self.hunger < 0:
self.hunger = 0
if self.happiness > 100:
self.happiness = 100
def play(self, time):
self.happiness += time
if self.happiness > 100:
self.happiness = 100
def sleep(self, hours):
self.happiness += hours
if self.happiness > 100:
self.happiness = 100
def status(self):
print(f"{self.name} is {self.age} years old. Happiness: {self.happiness}, Hunger: {self.hunger}, Health: {self.health}")
def get_sick(self):
self.health -= 20
if self.health < 0:
self.health = 0
def heal(self):
self.health += 30
if self.health > 100:
self.health = 100
在主函数中添加生病和治疗的选项:
def main():
name = input("What is your pet's name? ")
age = int(input("How old is your pet? "))
pet = Pet(name, age)
while True:
print("\n1. Feed")
print("2. Play")
print("3. Sleep")
print("4. Check Status")
print("5. Get Sick")
print("6. Heal")
print("7. Exit")
choice = input("Choose an action: ")
if choice == '1':
food = int(input("How much food? "))
pet.eat(food)
elif choice == '2':
time = int(input("How long to play? "))
pet.play(time)
elif choice == '3':
hours = int(input("How many hours to sleep? "))
pet.sleep(hours)
elif choice == '4':
pet.status()
elif choice == '5':
pet.get_sick()
elif choice == '6':
pet.heal()
elif choice == '7':
break
else:
print("Invalid choice, please try again.")
if __name__ == "__main__":
main()
2、增加多个宠物:
允许用户创建和管理多个宠物实例。
class PetManager:
def __init__(self):
self.pets = []
def add_pet(self, pet):
self.pets.append(pet)
def remove_pet(self, name):
self.pets = [pet for pet in self.pets if pet.name != name]
def find_pet(self, name):
for pet in self.pets:
if pet.name == name:
return pet
return None
def list_pets(self):
for pet in self.pets:
pet.status()
在主函数中管理多个宠物:
def main():
manager = PetManager()
while True:
print("\n1. Add Pet")
print("2. Remove Pet")
print("3. Find Pet")
print("4. List Pets")
print("5. Exit")
choice = input("Choose an action: ")
if choice == '1':
name = input("What is your pet's name? ")
age = int(input("How old is your pet? "))
pet = Pet(name, age)
manager.add_pet(pet)
elif choice == '2':
name = input("What is the name of the pet to remove? ")
manager.remove_pet(name)
elif choice == '3':
name = input("What is the name of the pet to find? ")
pet = manager.find_pet(name)
if pet:
pet.status()
else:
print("Pet not found.")
elif choice == '4':
manager.list_pets()
elif choice == '5':
break
else:
print("Invalid choice, please try again.")
if __name__ == "__main__":
main()
3、宠物的不同类型:
定义不同类型的宠物类,如狗、猫等,每种宠物有不同的属性和行为。
class Dog(Pet):
def __init__(self, name, age):
super().__init__(name, age)
self.loyalty = 100
def bark(self):
print(f"{self.name} is barking!")
class Cat(Pet):
def __init__(self, name, age):
super().__init__(name, age)
self.independence = 100
def meow(self):
print(f"{self.name} is meowing!")
在主函数中选择宠物类型:
def main():
manager = PetManager()
while True:
print("\n1. Add Pet")
print("2. Remove Pet")
print("3. Find Pet")
print("4. List Pets")
print("5. Exit")
choice = input("Choose an action: ")
if choice == '1':
print("1. Dog")
print("2. Cat")
pet_type = input("Choose pet type: ")
name = input("What is your pet's name? ")
age = int(input("How old is your pet? "))
if pet_type == '1':
pet = Dog(name, age)
elif pet_type == '2':
pet = Cat(name, age)
else:
print("Invalid choice.")
continue
manager.add_pet(pet)
elif choice == '2':
name = input("What is the name of the pet to remove? ")
manager.remove_pet(name)
elif choice == '3':
name = input("What is the name of the pet to find? ")
pet = manager.find_pet(name)
if pet:
pet.status()
else:
print("Pet not found.")
elif choice == '4':
manager.list_pets()
elif choice == '5':
break
else:
print("Invalid choice, please try again.")
if __name__ == "__main__":
main()
通过上述步骤,我们可以创建一个功能丰富的宠物程序,使用户可以通过命令行与宠物进行互动。通过不断扩展和添加新功能,程序可以变得更加复杂和有趣。
相关问答FAQs:
如何选择合适的Python库来开发宠物程序?
在开发宠物程序时,选择合适的Python库至关重要。常用的库包括Flask或Django用于构建Web应用,Pygame用于游戏开发,以及Pandas和NumPy用于数据处理。如果您打算添加图形用户界面,可以考虑使用Tkinter或PyQt。根据项目需求评估这些库的功能,并选择最符合您目标的工具。
我能在宠物程序中实现哪些功能?
宠物程序可以实现多种功能,具体取决于您的需求。例如,您可以创建一个宠物管理系统,记录宠物的健康信息、饮食习惯、疫苗接种记录等。也可以开发一个宠物社交平台,让宠物主人分享经验和照片。此外,可以设计一个宠物培训程序,提供训练教程和进度跟踪功能。
如何确保我的宠物程序易于使用和维护?
确保宠物程序的易用性和可维护性,可以从几个方面入手。首先,设计直观的用户界面,确保用户能够轻松找到所需功能。其次,编写清晰、简洁的代码,并附上详细的注释,以便后续维护。使用版本控制系统如Git,可以有效跟踪代码更改和更新,提升团队协作效率。定期进行用户反馈收集,及时改进程序功能和界面设计。
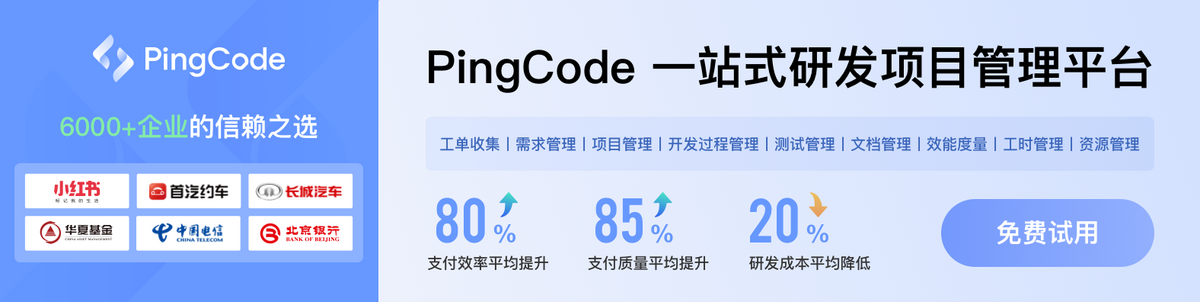