一、去掉字符串的方法
使用strip()方法、使用lstrip()和rstrip()方法、使用replace()方法、使用正则表达式、使用切片。其中,使用strip()方法是最常用和简便的方式之一。strip()方法可以删除字符串开头和结尾的指定字符(默认为空格)。例如:string.strip()
将会去掉字符串两端的空格。
strip()方法不仅适用于空格,还可以去除指定的字符。通过传递参数,可以去除字符串开头和结尾的任意字符。例如:string.strip('abc')
会去掉字符串两端的a、b、c字符。
接下来,我们将详细探讨上述每种方法的使用和适用场景。
二、使用strip()方法
1. 去除空格
strip()方法是用来去除字符串开头和结尾的空格的。示例如下:
string = " Hello, World! "
new_string = string.strip()
print(new_string) # 输出: "Hello, World!"
在上面的例子中,使用strip()方法后,字符串开头和结尾的空格被去掉了。
2. 去除指定字符
strip()方法还可以去除指定的字符。示例如下:
string = "aaaHello, World!aaa"
new_string = string.strip('a')
print(new_string) # 输出: "Hello, World!"
在上面的例子中,使用strip('a')方法后,字符串开头和结尾的a字符被去掉了。
三、使用lstrip()和rstrip()方法
1. 去除左侧字符
lstrip()方法是用来去除字符串左侧的指定字符(默认为空格)的。示例如下:
string = " Hello, World! "
new_string = string.lstrip()
print(new_string) # 输出: "Hello, World! "
在上面的例子中,使用lstrip()方法后,字符串左侧的空格被去掉了。
2. 去除右侧字符
rstrip()方法是用来去除字符串右侧的指定字符(默认为空格)的。示例如下:
string = " Hello, World! "
new_string = string.rstrip()
print(new_string) # 输出: " Hello, World!"
在上面的例子中,使用rstrip()方法后,字符串右侧的空格被去掉了。
四、使用replace()方法
1. 替换特定字符
replace()方法可以用于替换字符串中的特定字符。示例如下:
string = "Hello, World!"
new_string = string.replace('o', '')
print(new_string) # 输出: "Hell, Wrld!"
在上面的例子中,使用replace('o', '')方法后,字符串中的所有o字符被去掉了。
2. 替换多个字符
replace()方法还可以用于替换字符串中的多个字符。示例如下:
string = "Hello, World!"
new_string = string.replace('o', '').replace('l', '')
print(new_string) # 输出: "He, Wr!"
在上面的例子中,使用replace('o', '').replace('l', '')方法后,字符串中的所有o和l字符被去掉了。
五、使用正则表达式
1. 替换特定字符
可以使用正则表达式来匹配并替换字符串中的特定字符。示例如下:
import re
string = "Hello, World!"
new_string = re.sub('o', '', string)
print(new_string) # 输出: "Hell, Wrld!"
在上面的例子中,使用re.sub('o', '', string)方法后,字符串中的所有o字符被去掉了。
2. 替换模式匹配的字符
可以使用正则表达式来匹配并替换模式匹配的字符。示例如下:
import re
string = "Hello, World!"
new_string = re.sub('[aeiou]', '', string)
print(new_string) # 输出: "Hll, Wrld!"
在上面的例子中,使用re.sub('[aeiou]', '', string)方法后,字符串中的所有元音字母被去掉了。
六、使用切片
1. 去除前n个字符
可以使用切片来去除字符串的前n个字符。示例如下:
string = "Hello, World!"
new_string = string[2:]
print(new_string) # 输出: "llo, World!"
在上面的例子中,使用切片string[2:]方法后,字符串的前2个字符被去掉了。
2. 去除后n个字符
可以使用切片来去除字符串的后n个字符。示例如下:
string = "Hello, World!"
new_string = string[:-2]
print(new_string) # 输出: "Hello, Worl"
在上面的例子中,使用切片string[:-2]方法后,字符串的后2个字符被去掉了。
七、总结
在Python中,有多种方法可以去掉字符串中的特定字符或空格。strip()方法、lstrip()和rstrip()方法、replace()方法、正则表达式、切片都是常用的方法。根据具体需求选择合适的方法,可以高效地去除字符串中的不需要的字符。
相关问答FAQs:
如何在Python中移除字符串中的空格?
在Python中,可以使用str.replace()
方法或者str.strip()
方法来去掉字符串中的空格。str.strip()
会去掉字符串两端的空格,而str.replace(" ", "")
则可以移除字符串中所有的空格。例如:
original_str = " Hello World "
stripped_str = original_str.strip() # 去掉两端空格
no_space_str = original_str.replace(" ", "") # 去掉所有空格
Python中有没有内置方法可以移除特定字符?
Python的str.replace()
方法可以用来替换特定字符或子字符串。如果你想要移除某个字符,可以将其替换为空字符串。例如,如果要去掉字符'a':
original_str = "banana"
result_str = original_str.replace("a", "") # 移除所有'a'
如何使用正则表达式去掉字符串中的特定模式?
使用Python的re
模块可以通过正则表达式来移除字符串中的特定模式。re.sub()
方法非常有效。例如,以下代码可以去掉字符串中的所有数字:
import re
original_str = "Hello123 World456"
result_str = re.sub(r'\d+', '', original_str) # 移除所有数字
这样可以轻松处理更复杂的字符串清理任务。
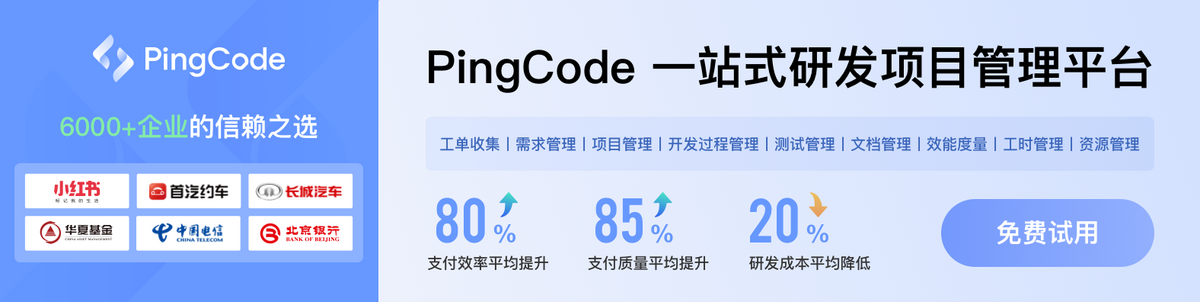