Python中设置超时时间的方法包括使用requests
库、socket
库、threading
库等。 其中最常用且简单的方法是使用requests
库的timeout
参数。例如:
import requests
try:
response = requests.get('https://example.com', timeout=5)
print(response.status_code)
except requests.Timeout:
print('The request timed out')
except requests.RequestException as e:
print(f'An error occurred: {e}')
在这段代码中,timeout=5
设置了请求的超时时间为5秒。如果请求在5秒内没有完成,会引发requests.Timeout
异常。下面将详细介绍几种设置超时时间的方法。
一、使用requests库
1、设置请求超时时间
requests
库是一个非常流行的HTTP库,它允许你发送HTTP请求。timeout
参数可以用来设置请求的超时时间。以下是一个简单的例子:
import requests
try:
response = requests.get('https://example.com', timeout=5)
print(response.status_code)
except requests.Timeout:
print('The request timed out')
except requests.RequestException as e:
print(f'An error occurred: {e}')
在这个例子中,timeout=5
设置了请求的超时时间为5秒。如果请求在5秒内没有完成,会引发requests.Timeout
异常,从而可以进行错误处理。
2、分别设置连接和读取超时时间
有时候,我们可能需要分别设置连接(connection)和读取(read)的超时时间。这可以通过传递一个元组给timeout
参数来实现:
import requests
try:
response = requests.get('https://example.com', timeout=(3.05, 27))
print(response.status_code)
except requests.Timeout:
print('The request timed out')
except requests.RequestException as e:
print(f'An error occurred: {e}')
在这个例子中,timeout=(3.05, 27)
表示连接超时时间为3.05秒,读取超时时间为27秒。这样可以更灵活地控制不同类型的超时。
二、使用socket库
1、设置默认超时时间
socket
库是Python标准库的一部分,可以用于底层网络编程。可以使用socket.setdefaulttimeout()
函数来设置默认的超时时间:
import socket
设置默认超时时间为5秒
socket.setdefaulttimeout(5)
创建一个新的socket对象
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
连接到远程服务器
try:
s.connect(('example.com', 80))
print('Connected successfully')
except socket.timeout:
print('The connection timed out')
finally:
s.close()
在这个例子中,socket.setdefaulttimeout(5)
设置了默认的超时时间为5秒。如果连接操作在5秒内没有完成,会引发socket.timeout
异常。
2、设置特定操作的超时时间
除了设置默认超时时间,还可以为特定的操作设置超时时间。通过使用settimeout()
方法,可以为某个socket对象设置特定的超时时间:
import socket
创建一个新的socket对象
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
设置连接超时时间为5秒
s.settimeout(5)
连接到远程服务器
try:
s.connect(('example.com', 80))
print('Connected successfully')
except socket.timeout:
print('The connection timed out')
finally:
s.close()
在这个例子中,s.settimeout(5)
设置了连接操作的超时时间为5秒。如果连接操作在5秒内没有完成,会引发socket.timeout
异常。
三、使用threading库
1、使用线程和事件对象
threading
库提供了强大的多线程支持,可以用来实现带有超时功能的操作。通过使用线程和事件对象,可以实现复杂的超时控制逻辑。以下是一个例子:
import threading
def long_running_task(event):
# 模拟一个长时间运行的任务
for i in range(10):
if event.is_set():
print('Task was cancelled')
return
print(f'Running task {i}')
time.sleep(1)
print('Task completed')
创建一个事件对象
event = threading.Event()
创建一个线程来执行长时间运行的任务
thread = threading.Thread(target=long_running_task, args=(event,))
thread.start()
设置超时时间
timeout = 5
等待超时时间过去或任务完成
thread.join(timeout)
检查任务是否还在运行
if thread.is_alive():
print('Task is still running, cancelling it')
event.set()
thread.join()
else:
print('Task completed within timeout')
在这个例子中,使用了threading.Event
对象来实现任务的取消。主线程等待任务线程运行指定的超时时间,如果任务线程在超时时间内没有完成,主线程会设置事件对象,从而通知任务线程取消任务。
四、使用asyncio库
1、设置异步操作的超时时间
asyncio
库是Python 3.4中引入的用于编写异步代码的标准库。asyncio.wait_for
函数可以用来设置异步操作的超时时间。以下是一个例子:
import asyncio
async def long_running_task():
for i in range(10):
print(f'Running task {i}')
await asyncio.sleep(1)
print('Task completed')
async def main():
try:
# 设置超时时间为5秒
await asyncio.wait_for(long_running_task(), timeout=5)
except asyncio.TimeoutError:
print('The task timed out')
运行主函数
asyncio.run(main())
在这个例子中,asyncio.wait_for
函数用于设置异步操作的超时时间。如果long_running_task
在5秒内没有完成,会引发asyncio.TimeoutError
异常。
2、使用asyncio.TimeoutError进行错误处理
在使用asyncio
时,超时通常表示某个操作花费的时间比预期的要长。可以通过捕获asyncio.TimeoutError
异常来处理超时情况:
import asyncio
async def long_running_task():
for i in range(10):
print(f'Running task {i}')
await asyncio.sleep(1)
print('Task completed')
async def main():
try:
# 设置超时时间为5秒
await asyncio.wait_for(long_running_task(), timeout=5)
except asyncio.TimeoutError:
print('The task timed out')
else:
print('The task completed successfully')
运行主函数
asyncio.run(main())
在这个例子中,捕获到asyncio.TimeoutError
异常后,可以执行相应的错误处理逻辑。如果任务在超时时间内完成,则会执行else
块中的代码。
五、使用concurrent.futures库
1、设置线程池执行器的超时时间
concurrent.futures
库提供了高层次的接口来管理线程和进程池。可以使用ThreadPoolExecutor
和ProcessPoolExecutor
来执行带有超时功能的任务。以下是一个例子:
from concurrent.futures import ThreadPoolExecutor, TimeoutError
def long_running_task():
import time
for i in range(10):
print(f'Running task {i}')
time.sleep(1)
return 'Task completed'
创建一个线程池执行器
with ThreadPoolExecutor() as executor:
# 提交任务并设置超时时间为5秒
future = executor.submit(long_running_task)
try:
result = future.result(timeout=5)
except TimeoutError:
print('The task timed out')
else:
print(f'The task completed successfully: {result}')
在这个例子中,使用ThreadPoolExecutor
来执行长时间运行的任务,并通过future.result(timeout=5)
设置超时时间。如果任务在5秒内没有完成,会引发TimeoutError
异常。
2、设置进程池执行器的超时时间
类似地,可以使用ProcessPoolExecutor
来执行带有超时功能的任务:
from concurrent.futures import ProcessPoolExecutor, TimeoutError
def long_running_task():
import time
for i in range(10):
print(f'Running task {i}')
time.sleep(1)
return 'Task completed'
创建一个进程池执行器
with ProcessPoolExecutor() as executor:
# 提交任务并设置超时时间为5秒
future = executor.submit(long_running_task)
try:
result = future.result(timeout=5)
except TimeoutError:
print('The task timed out')
else:
print(f'The task completed successfully: {result}')
在这个例子中,使用ProcessPoolExecutor
来执行长时间运行的任务,并通过future.result(timeout=5)
设置超时时间。如果任务在5秒内没有完成,会引发TimeoutError
异常。
六、使用signal库
1、设置信号处理器
在Unix系统上,可以使用signal
库来设置超时时间。通过设置信号处理器,可以在超时时间内中断长时间运行的操作。以下是一个例子:
import signal
def handler(signum, frame):
raise TimeoutError('The operation timed out')
设置信号处理器
signal.signal(signal.SIGALRM, handler)
设置超时时间为5秒
signal.alarm(5)
try:
# 模拟一个长时间运行的操作
import time
for i in range(10):
print(f'Running operation {i}')
time.sleep(1)
except TimeoutError as e:
print(e)
finally:
# 取消闹钟
signal.alarm(0)
在这个例子中,使用signal.signal
函数设置信号处理器,并通过signal.alarm
函数设置超时时间。如果操作在5秒内没有完成,会引发TimeoutError
异常。
2、取消闹钟
在使用signal
库时,务必要在操作完成后取消闹钟,以避免后续操作受到影响:
import signal
def handler(signum, frame):
raise TimeoutError('The operation timed out')
设置信号处理器
signal.signal(signal.SIGALRM, handler)
设置超时时间为5秒
signal.alarm(5)
try:
# 模拟一个长时间运行的操作
import time
for i in range(10):
print(f'Running operation {i}')
time.sleep(1)
except TimeoutError as e:
print(e)
finally:
# 取消闹钟
signal.alarm(0)
在这个例子中,使用signal.alarm(0)
取消闹钟,以确保后续操作不会受到超时信号的影响。
七、使用第三方库
1、使用timeout-decorator库
timeout-decorator
是一个第三方库,可以方便地为函数添加超时功能。以下是一个例子:
from timeout_decorator import timeout, TimeoutError
@timeout(5)
def long_running_task():
import time
for i in range(10):
print(f'Running task {i}')
time.sleep(1)
return 'Task completed'
try:
result = long_running_task()
except TimeoutError:
print('The task timed out')
else:
print(f'The task completed successfully: {result}')
在这个例子中,使用@timeout(5)
装饰器为long_running_task
函数添加超时功能。如果函数在5秒内没有完成,会引发TimeoutError
异常。
2、安装和使用其他第三方库
除了timeout-decorator
库外,还有许多其他第三方库可以用来实现超时功能。例如,可以使用gevent
库来实现异步超时控制:
import gevent
from gevent import Timeout
def long_running_task():
import time
for i in range(10):
print(f'Running task {i}')
time.sleep(1)
return 'Task completed'
timeout = Timeout(5)
timeout.start()
try:
result = gevent.spawn(long_running_task).get()
except Timeout:
print('The task timed out')
else:
print(f'The task completed successfully: {result}')
在这个例子中,使用gevent.Timeout
类来设置超时时间,并通过gevent.spawn
函数来执行长时间运行的任务。如果任务在5秒内没有完成,会引发Timeout
异常。
八、总结
Python中有多种方法可以设置超时时间,包括使用requests
库、socket
库、threading
库、asyncio
库、concurrent.futures
库、signal
库以及第三方库如timeout-decorator
和gevent
。每种方法都有其适用的场景和优缺点,选择适合的超时控制方法可以大大提高程序的可靠性和用户体验。
使用requests
库的timeout
参数是最常用且简单的方法,适用于大部分HTTP请求场景。而对于更复杂的异步操作,可以使用asyncio
库和concurrent.futures
库来实现灵活的超时控制。对于底层网络编程,可以使用socket
库和signal
库来实现超时控制。通过合理使用这些方法,可以有效地处理超时问题,确保程序稳定运行。
相关问答FAQs:
如何在Python中设置HTTP请求的超时时间?
在Python中,使用requests
库进行HTTP请求时,可以通过timeout
参数设置超时时间。例如,requests.get('http://example.com', timeout=5)
会在5秒内等待响应。如果在该时间内未收到响应,将引发requests.exceptions.Timeout
异常。可以根据需要调整超时时间,以确保应用程序的稳定性。
在Python的Socket编程中如何设置超时时间?
在Socket编程中,可以使用settimeout
方法设置超时时间。例如,创建一个Socket对象后,可以调用sock.settimeout(5)
,这样在5秒内没有任何操作(如发送或接收数据),将会引发socket.timeout
异常。这在网络连接不稳定的情况下尤其有用,可以避免程序无响应。
如何在Python的多线程环境中设置超时时间?
在多线程环境中,可以使用threading.Event
对象来实现超时控制。通过调用event.wait(timeout)
方法,可以设置一个超时时间。如果在指定时间内事件未被设置,方法会返回False
,从而可以在多线程中避免无限期等待。这种方式适合需要同时处理多个任务的场景。
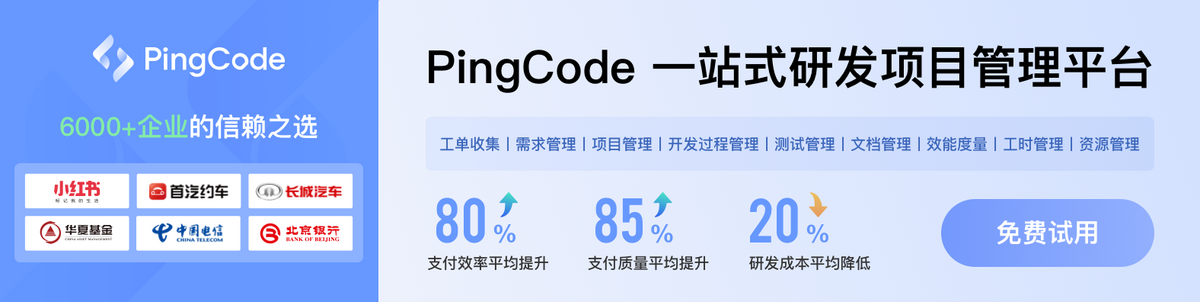