Python提供了许多方法来转换字符串,包括数据类型转换、编码转换和格式转换等。常用的字符串转换方法包括类型转换、编码转换、字符串格式化。下面我们将详细介绍这些方法中的一种——类型转换。
类型转换是将字符串转换为其他数据类型,例如整型、浮点型、列表、字典等。类型转换在数据处理和分析中非常常用,尤其是在处理用户输入和文件读取的数据时。
一、类型转换
1.1 转换为整数
Python提供了内置函数int()
来将字符串转换为整数。转换过程中,如果字符串不能被成功转换为整数,将会引发ValueError
异常。
s = "123"
num = int(s)
print(num) # 输出: 123
1.2 转换为浮点数
类似于转换为整数,Python使用内置函数float()
将字符串转换为浮点数。
s = "123.45"
num = float(s)
print(num) # 输出: 123.45
1.3 转换为列表
使用list()
函数可以将字符串转换为列表,其中每个字符作为列表的一个元素。
s = "hello"
lst = list(s)
print(lst) # 输出: ['h', 'e', 'l', 'l', 'o']
1.4 转换为字典
将字符串转换为字典相对复杂一些,通常需要使用json
模块中的loads()
函数来实现,前提是字符串的格式必须是合法的JSON格式。
import json
s = '{"name": "John", "age": 30}'
d = json.loads(s)
print(d) # 输出: {'name': 'John', 'age': 30}
二、编码转换
2.1 字符串编码
编码转换是将字符串从一种编码格式转换为另一种编码格式。Python的字符串默认是Unicode编码,可以使用encode()
方法将字符串转换为其他编码格式,如UTF-8、ASCII等。
s = "hello"
encoded_str = s.encode('utf-8')
print(encoded_str) # 输出: b'hello'
2.2 字符串解码
与编码相对应,解码是将字节序列转换为字符串,使用decode()
方法实现。
b = b'hello'
decoded_str = b.decode('utf-8')
print(decoded_str) # 输出: hello
三、字符串格式化
3.1 使用百分号格式化
百分号格式化是Python中最早的字符串格式化方法,使用%
操作符进行格式化。
name = "John"
age = 30
s = "My name is %s and I am %d years old." % (name, age)
print(s) # 输出: My name is John and I am 30 years old.
3.2 使用format()
方法
format()
方法提供了更强大的字符串格式化功能,可以通过位置参数和关键字参数来实现复杂的格式化。
name = "John"
age = 30
s = "My name is {} and I am {} years old.".format(name, age)
print(s) # 输出: My name is John and I am 30 years old.
3.3 使用f字符串
f字符串是Python 3.6引入的新特性,通过在字符串前加上f
或F
,并在字符串内部使用大括号{}
包裹表达式,实现内嵌变量的字符串格式化。
name = "John"
age = 30
s = f"My name is {name} and I am {age} years old."
print(s) # 输出: My name is John and I am 30 years old.
四、字符串操作
4.1 字符串拼接
Python中可以使用+
操作符进行字符串拼接,但在需要拼接大量字符串时,推荐使用join()
方法提高效率。
s1 = "hello"
s2 = "world"
s = s1 + " " + s2
print(s) # 输出: hello world
使用 join 方法
strings = ["hello", "world"]
s = " ".join(strings)
print(s) # 输出: hello world
4.2 字符串拆分
使用split()
方法可以将字符串拆分为列表,默认以空格为分隔符。
s = "hello world"
lst = s.split()
print(lst) # 输出: ['hello', 'world']
4.3 字符串替换
使用replace()
方法可以将字符串中的指定子字符串替换为新的子字符串。
s = "hello world"
new_s = s.replace("world", "Python")
print(new_s) # 输出: hello Python
4.4 去除空白字符
使用strip()
、lstrip()
和rstrip()
方法可以去除字符串两端、左端和右端的空白字符。
s = " hello world "
print(s.strip()) # 输出: hello world
print(s.lstrip()) # 输出: hello world
print(s.rstrip()) # 输出: hello world
4.5 字符串大小写转换
Python提供了多种方法来转换字符串的大小写,包括upper()
、lower()
、capitalize()
、title()
等。
s = "hello world"
print(s.upper()) # 输出: HELLO WORLD
print(s.lower()) # 输出: hello world
print(s.capitalize()) # 输出: Hello world
print(s.title()) # 输出: Hello World
五、正则表达式
正则表达式是一种强大的字符串处理工具,Python提供了re
模块来支持正则表达式操作。
5.1 匹配字符串
使用re.match()
方法从字符串的起始位置开始匹配,如果匹配成功,返回一个匹配对象,否则返回None
。
import re
s = "hello world"
match = re.match(r'hello', s)
if match:
print("Match found:", match.group()) # 输出: Match found: hello
else:
print("No match")
5.2 搜索字符串
使用re.search()
方法在整个字符串中搜索匹配,如果找到,返回一个匹配对象,否则返回None
。
import re
s = "hello world"
search = re.search(r'world', s)
if search:
print("Search found:", search.group()) # 输出: Search found: world
else:
print("No match")
5.3 查找所有匹配
使用re.findall()
方法返回字符串中所有非重叠匹配的列表。
import re
s = "hello world, hello Python"
matches = re.findall(r'hello', s)
print(matches) # 输出: ['hello', 'hello']
5.4 替换字符串
使用re.sub()
方法可以将字符串中所有匹配的子字符串替换为新的子字符串。
import re
s = "hello world"
new_s = re.sub(r'world', 'Python', s)
print(new_s) # 输出: hello Python
六、字符串切片
字符串切片是提取子字符串的一种方式,使用[start:stop:step]
语法,其中start
表示起始索引,stop
表示结束索引,step
表示步长。
s = "hello world"
print(s[0:5]) # 输出: hello
print(s[6:]) # 输出: world
print(s[::2]) # 输出: hlowrd
七、字符串查找
7.1 查找子字符串
使用find()
方法可以查找子字符串在字符串中第一次出现的位置,如果找不到,返回-1。
s = "hello world"
pos = s.find("world")
print(pos) # 输出: 6
7.2 统计子字符串出现次数
使用count()
方法可以统计子字符串在字符串中出现的次数。
s = "hello world, hello Python"
count = s.count("hello")
print(count) # 输出: 2
八、字符串与字节转换
8.1 字符串转字节
使用encode()
方法可以将字符串转换为字节对象。
s = "hello world"
b = s.encode('utf-8')
print(b) # 输出: b'hello world'
8.2 字节转字符串
使用decode()
方法可以将字节对象转换为字符串。
b = b'hello world'
s = b.decode('utf-8')
print(s) # 输出: hello world
以上便是关于Python如何转换字符串的详细介绍。通过这些方法和技巧,你可以轻松处理字符串的各种转换需求。在实际应用中,根据具体需求选择合适的方法和工具,将会大大提高编程效率和代码可读性。
相关问答FAQs:
如何在Python中将字符串转换为整数或浮点数?
在Python中,可以使用内置的int()
函数将字符串转换为整数,或者使用float()
函数将字符串转换为浮点数。例如,如果您有一个字符串"123"
,可以通过int("123")
将其转换为整数123,而float("123.45")
则会将字符串"123.45"
转换为浮点数123.45。确保字符串的格式是正确的,否则会引发ValueError
异常。
在Python中如何处理字符串的大小写转换?
Python提供了多种方法来处理字符串的大小写转换。您可以使用str.upper()
将字符串转换为全大写,使用str.lower()
将其转换为全小写,或者使用str.title()
将每个单词的首字母转换为大写。例如,"hello world".upper()
会返回"HELLO WORLD"
,而"hello world".title()
会返回"Hello World"
。
如何在Python中对字符串进行分割和连接?
在Python中,您可以使用str.split()
方法将字符串根据指定的分隔符分割成列表。例如,"a,b,c".split(",")
将返回["a", "b", "c"]
。要将列表中的字符串连接成一个字符串,可以使用str.join()
方法。例如,",".join(["a", "b", "c"])
将返回"a,b,c"
。这种方式非常适合处理CSV格式的数据或其他需要分隔的字符串。
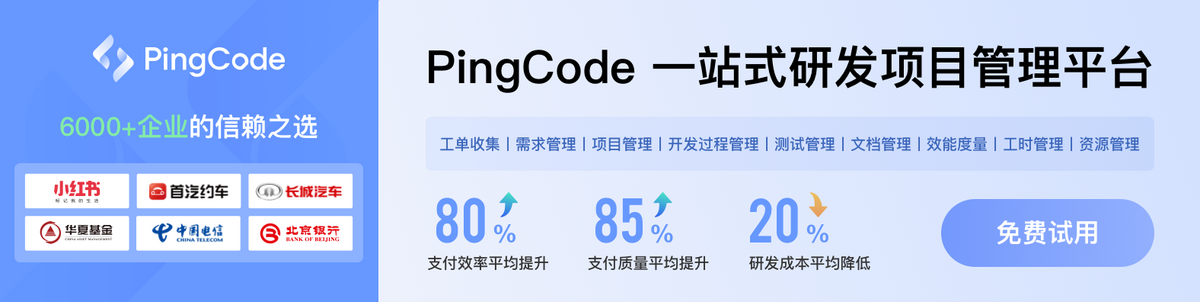