在Python中调用外部命令的常用方法包括使用subprocess模块、os.system()函数、os.popen()函数、以及sh模块等。其中,subprocess模块是最为推荐的方法,因为它功能强大且安全性高。下面将详细介绍使用subprocess模块的方法,并简要提及其他方法。
一、subprocess模块
1.1 subprocess.run()
subprocess.run()
是Python 3.5引入的新函数,用于运行外部命令并等待命令完成。
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
- capture_output=True:捕获标准输出和标准错误。
- text=True:将输出作为字符串而不是字节处理。
subprocess.run()
方法返回一个CompletedProcess
实例,其中包含命令的返回码、标准输出和标准错误。
1.2 subprocess.Popen()
subprocess.Popen()
是一个更底层的接口,提供了更大的灵活性。它不会等待命令完成,可以用于异步执行命令。
import subprocess
process = subprocess.Popen(['ls', '-l'], stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
stdout, stderr = process.communicate()
print(stdout)
- stdout=subprocess.PIPE:将标准输出重定向到管道。
- stderr=subprocess.PIPE:将标准错误重定向到管道。
- process.communicate():等待命令完成并获取输出。
二、os.system()函数
os.system()
是一个简单但不推荐的方法,因为它的功能较为有限,且不安全(容易受到shell注入攻击)。
import os
os.system('ls -l')
os.system()
的返回值是命令的退出状态码,而不是命令的输出。
三、os.popen()函数
os.popen()
用于打开一个管道连接,可以读取或写入外部命令的标准输入输出。
import os
with os.popen('ls -l') as stream:
output = stream.read()
print(output)
虽然os.popen()
比os.system()
稍微灵活一些,但仍不如subprocess
模块强大。
四、sh模块
sh
模块是一个第三方库,提供了更为Pythonic的方式来调用外部命令。需要先安装:
pip install sh
import sh
print(sh.ls('-l'))
sh
模块的API非常简洁,但是不在标准库中,需要额外安装。
五、比较与选择
在不同场景下,选择合适的方法是关键。
- subprocess.run():推荐用于同步执行命令,简单且安全。
- subprocess.Popen():用于需要异步执行或更复杂的命令操作。
- os.system():不推荐,功能有限且不安全。
- os.popen():适用于简单的输出捕获,但功能不如
subprocess
。 - sh模块:适用于需要简洁代码的情况,但需要额外安装。
六、实例分析
6.1 同步执行命令并捕获输出
使用subprocess.run()
可以方便地捕获命令的输出。
import subprocess
def run_command(command):
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
return result.stdout
else:
return result.stderr
output = run_command(['ls', '-l'])
print(output)
6.2 异步执行命令
使用subprocess.Popen()
可以实现异步执行命令。
import subprocess
def run_command_async(command):
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
return process
process = run_command_async(['ping', 'google.com', '-c', '4'])
stdout, stderr = process.communicate()
print(stdout)
6.3 处理长时间运行的命令
对于长时间运行的命令,可以使用subprocess
模块的回调机制。
import subprocess
def run_long_command(command):
process = subprocess.Popen(command, stdout=subprocess.PIPE, stderr=subprocess.PIPE, text=True)
while True:
output = process.stdout.readline()
if output == '' and process.poll() is not None:
break
if output:
print(output.strip())
run_long_command(['ping', 'google.com', '-c', '10'])
七、安全性与错误处理
使用subprocess
模块时,应注意安全性和错误处理。
7.1 避免Shell注入
避免直接传递用户输入到shell命令中,使用subprocess.run()
的args
参数传递命令列表。
import subprocess
def safe_run_command(user_input):
command = ['ls', '-l', user_input]
result = subprocess.run(command, capture_output=True, text=True)
return result.stdout
output = safe_run_command('/home/user')
print(output)
7.2 错误处理
捕获和处理命令执行中的错误,确保程序健壮性。
import subprocess
def run_command_with_error_handling(command):
try:
result = subprocess.run(command, capture_output=True, text=True, check=True)
return result.stdout
except subprocess.CalledProcessError as e:
return f"Command '{e.cmd}' returned non-zero exit status {e.returncode}."
output = run_command_with_error_handling(['ls', '-l', '/nonexistent'])
print(output)
八、实际应用场景
8.1 自动化脚本
在自动化脚本中,调用外部命令是常见需求。例如,备份数据库或部署应用程序。
import subprocess
def backup_database():
command = ['pg_dump', 'mydatabase', '-f', 'backup.sql']
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print("Backup successful.")
else:
print(f"Backup failed: {result.stderr}")
backup_database()
8.2 系统监控
调用系统命令来获取系统状态信息,例如CPU使用率、内存使用情况等。
import subprocess
def get_cpu_usage():
command = ['mpstat', '1', '1']
result = subprocess.run(command, capture_output=True, text=True)
if result.returncode == 0:
print(result.stdout)
else:
print(f"Failed to get CPU usage: {result.stderr}")
get_cpu_usage()
九、总结
Python提供了多种方法来调用外部命令,其中subprocess模块是最推荐的方法,具有功能强大、灵活性高、安全性好等优点。通过了解和掌握这些方法,可以在实际项目中更好地调用和管理外部命令,实现自动化和系统监控等任务。无论是简单的命令调用还是复杂的异步执行,subprocess模块都能够满足需求。
相关问答FAQs:
如何在Python中执行系统命令?
在Python中,可以使用subprocess
模块来执行外部命令。这个模块提供了丰富的接口,可以用于运行命令并与其进行交互。以下是一个基本的示例:
import subprocess
result = subprocess.run(['ls', '-l'], capture_output=True, text=True)
print(result.stdout)
这个代码片段将执行ls -l
命令并打印输出。
在Python中捕获外部命令的输出有什么方法?
除了使用subprocess.run()
,还可以使用subprocess.Popen()
来捕获输出。Popen
提供了更强大的控制选项,包括输入和输出流的管理。示例代码如下:
import subprocess
process = subprocess.Popen(['echo', 'Hello, World!'], stdout=subprocess.PIPE, stderr=subprocess.PIPE)
stdout, stderr = process.communicate()
print(stdout.decode())
这个示例展示了如何获取命令的标准输出和错误输出。
在执行外部命令时如何处理异常情况?
在执行外部命令时,可能会遇到多种异常情况,比如命令不存在、权限问题等。可以通过捕获subprocess.CalledProcessError
来处理这些异常。例如:
import subprocess
try:
subprocess.run(['invalid_command'], check=True)
except subprocess.CalledProcessError as e:
print(f"命令执行失败: {e}")
这段代码将尝试执行一个无效的命令,并在失败时打印错误信息。
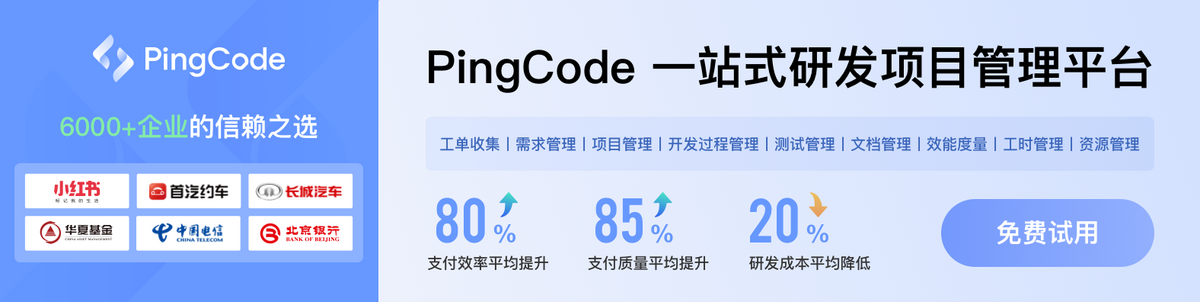