使用Python生成文件可以通过多种方式实现,常见的方法包括使用内置的open()
函数、os
模块、pathlib
模块和第三方库(如pandas
、numpy
等)来创建和写入文件。 其中,最常用的方式是使用open()
函数来打开或创建文件,然后通过文件对象的方法进行读写操作。下面将对其中一种方法进行详细描述。
使用open()
函数生成文件:
open()
函数是Python内置的文件操作函数,可以用来打开一个文件。如果文件不存在,它可以创建文件。使用open()
函数的基本语法如下:
file = open('filename', 'mode')
其中,filename
是文件的名称,mode
是文件的打开模式,如读取('r')、写入('w')、追加('a')等。常用的模式包括:
- 'r':读取模式(默认模式),如果文件不存在会报错。
- 'w':写入模式,如果文件不存在会创建文件,如果文件存在会覆盖文件内容。
- 'a':追加模式,如果文件不存在会创建文件,如果文件存在会在文件末尾追加内容。
- 'b':二进制模式,用于处理二进制文件,如图片、视频等。
以下是一个示例,展示如何使用open()
函数生成并写入文件:
# 打开或创建文件
file = open('example.txt', 'w')
写入内容
file.write('Hello, World!\n')
file.write('This is a new file created by Python.\n')
关闭文件
file.close()
在使用open()
函数时,建议使用with
语句来确保文件被正确关闭,即使在写入过程中发生异常:
with open('example.txt', 'w') as file:
file.write('Hello, World!\n')
file.write('This is a new file created by Python.\n')
这样可以自动管理文件的打开和关闭,避免资源泄漏的问题。
一、使用open()
函数生成文件
使用open()
函数生成文件是Python中最常见的方法之一。它提供了灵活的文件操作方式,适用于各种简单文件操作场景。
1、基本操作
open()
函数是Python内置的文件操作函数,可以用来打开、读取、写入和追加文件。基本操作步骤如下:
# 打开或创建文件
file = open('example.txt', 'w')
写入内容
file.write('Hello, World!\n')
file.write('This is a new file created by Python.\n')
关闭文件
file.close()
在上述示例中,open()
函数以写入模式('w')打开或创建名为example.txt
的文件,然后使用write()
方法写入内容,最后关闭文件。
2、使用with
语句
使用with
语句可以确保文件在操作完成后被正确关闭,即使在写入过程中发生异常:
with open('example.txt', 'w') as file:
file.write('Hello, World!\n')
file.write('This is a new file created by Python.\n')
with
语句会在代码块执行完毕后自动调用file.close()
方法,避免资源泄漏问题。
3、读取文件内容
除了写入文件,open()
函数还可以用于读取文件内容。以下示例展示如何读取文件内容并打印到控制台:
with open('example.txt', 'r') as file:
content = file.read()
print(content)
在上述示例中,open()
函数以读取模式('r')打开文件,然后使用read()
方法读取文件内容,并打印到控制台。
4、追加文件内容
如果需要在文件末尾追加内容,可以使用追加模式('a'):
with open('example.txt', 'a') as file:
file.write('Appending new content to the file.\n')
在上述示例中,open()
函数以追加模式('a')打开文件,然后使用write()
方法在文件末尾追加内容。
二、使用os
模块生成文件
os
模块提供了与操作系统进行交互的功能,可以用于文件和目录的创建、删除、移动等操作。使用os
模块生成文件的方法如下:
1、创建文件
使用os
模块创建文件可以通过调用open()
函数实现,但通常用于更复杂的文件操作场景:
import os
创建文件
with open('example_os.txt', 'w') as file:
file.write('This file is created using os module.\n')
2、检查文件是否存在
在创建文件之前,可以使用os.path.exists()
方法检查文件是否存在:
import os
filename = 'example_os.txt'
if not os.path.exists(filename):
with open(filename, 'w') as file:
file.write('This file is created using os module.\n')
else:
print(f'File {filename} already exists.')
在上述示例中,使用os.path.exists()
方法检查文件是否存在,如果不存在则创建文件。
3、删除文件
可以使用os.remove()
方法删除文件:
import os
filename = 'example_os.txt'
if os.path.exists(filename):
os.remove(filename)
print(f'File {filename} has been deleted.')
else:
print(f'File {filename} does not exist.')
在上述示例中,使用os.remove()
方法删除文件,并检查文件是否存在。
4、创建目录
os
模块还可以用于创建目录:
import os
directory = 'example_directory'
if not os.path.exists(directory):
os.makedirs(directory)
print(f'Directory {directory} has been created.')
else:
print(f'Directory {directory} already exists.')
在上述示例中,使用os.makedirs()
方法创建目录,并检查目录是否存在。
三、使用pathlib
模块生成文件
pathlib
模块提供了面向对象的文件和目录操作方法,是Python 3.4引入的标准库。使用pathlib
模块生成文件的方法如下:
1、创建文件
使用pathlib
模块创建文件可以通过调用write_text()
方法实现:
from pathlib import Path
创建文件
file_path = Path('example_pathlib.txt')
file_path.write_text('This file is created using pathlib module.\n')
在上述示例中,使用Path
对象的write_text()
方法创建文件并写入内容。
2、读取文件内容
可以使用read_text()
方法读取文件内容:
from pathlib import Path
读取文件内容
file_path = Path('example_pathlib.txt')
content = file_path.read_text()
print(content)
在上述示例中,使用Path
对象的read_text()
方法读取文件内容,并打印到控制台。
3、检查文件是否存在
可以使用exists()
方法检查文件是否存在:
from pathlib import Path
检查文件是否存在
file_path = Path('example_pathlib.txt')
if file_path.exists():
print(f'File {file_path} exists.')
else:
print(f'File {file_path} does not exist.')
在上述示例中,使用Path
对象的exists()
方法检查文件是否存在。
4、删除文件
可以使用unlink()
方法删除文件:
from pathlib import Path
删除文件
file_path = Path('example_pathlib.txt')
if file_path.exists():
file_path.unlink()
print(f'File {file_path} has been deleted.')
else:
print(f'File {file_path} does not exist.')
在上述示例中,使用Path
对象的unlink()
方法删除文件,并检查文件是否存在。
5、创建目录
pathlib
模块还可以用于创建目录:
from pathlib import Path
创建目录
directory_path = Path('example_pathlib_directory')
if not directory_path.exists():
directory_path.mkdir()
print(f'Directory {directory_path} has been created.')
else:
print(f'Directory {directory_path} already exists.')
在上述示例中,使用Path
对象的mkdir()
方法创建目录,并检查目录是否存在。
四、使用第三方库生成文件
除了Python内置模块,许多第三方库也提供了生成文件的功能,如pandas
、numpy
等。使用第三方库生成文件的方法如下:
1、使用pandas
生成CSV文件
pandas
是一个强大的数据分析库,可以方便地生成和处理CSV文件:
import pandas as pd
创建数据
data = {'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35],
'City': ['New York', 'Los Angeles', 'Chicago']}
创建DataFrame
df = pd.DataFrame(data)
生成CSV文件
df.to_csv('example_pandas.csv', index=False)
在上述示例中,使用pandas
库创建一个DataFrame,然后使用to_csv()
方法生成CSV文件。
2、使用numpy
生成文本文件
numpy
是一个强大的科学计算库,可以方便地生成和处理文本文件:
import numpy as np
创建数据
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
生成文本文件
np.savetxt('example_numpy.txt', data, fmt='%d')
在上述示例中,使用numpy
库创建一个数组,然后使用savetxt()
方法生成文本文件。
3、使用json
生成JSON文件
json
模块是Python内置的库,用于处理JSON数据,可以方便地生成和读取JSON文件:
import json
创建数据
data = {'Name': 'Alice', 'Age': 25, 'City': 'New York'}
生成JSON文件
with open('example.json', 'w') as file:
json.dump(data, file, indent=4)
在上述示例中,使用json
模块创建一个字典,然后使用dump()
方法生成JSON文件。
五、文件读写的高级操作
除了基本的文件读写操作,Python还支持一些高级的文件操作,如文件定位、批量处理等。
1、文件定位
可以使用文件对象的seek()
方法定位文件指针的位置:
with open('example.txt', 'r') as file:
# 定位到文件开头
file.seek(0)
# 读取文件内容
content = file.read()
print(content)
在上述示例中,使用seek()
方法将文件指针定位到文件开头,然后读取文件内容。
2、批量处理文件
可以使用os
模块遍历目录中的所有文件,并进行批量处理:
import os
directory = 'example_directory'
for filename in os.listdir(directory):
file_path = os.path.join(directory, filename)
if os.path.isfile(file_path):
with open(file_path, 'r') as file:
content = file.read()
print(f'Content of {filename}:\n{content}\n')
在上述示例中,使用os.listdir()
方法遍历目录中的所有文件,并读取每个文件的内容。
六、文件操作的最佳实践
在进行文件操作时,遵循以下最佳实践可以提高代码的健壮性和可维护性:
1、使用with
语句
使用with
语句可以确保文件在操作完成后被正确关闭,避免资源泄漏问题:
with open('example.txt', 'w') as file:
file.write('Hello, World!\n')
2、检查文件是否存在
在进行文件操作之前,检查文件是否存在可以避免不必要的错误:
import os
filename = 'example.txt'
if os.path.exists(filename):
with open(filename, 'r') as file:
content = file.read()
else:
print(f'File {filename} does not exist.')
3、处理文件操作异常
在进行文件操作时,处理可能发生的异常可以提高代码的健壮性:
try:
with open('example.txt', 'r') as file:
content = file.read()
except FileNotFoundError:
print('File not found.')
except IOError:
print('An error occurred while reading the file.')
通过遵循这些最佳实践,可以编写出更加健壮和可维护的文件操作代码。
相关问答FAQs:
1. 使用Python生成文件的基本步骤是什么?
生成文件的基本步骤包括:导入必要的模块(如os
),使用open()
函数创建或打开一个文件,选择写入模式(如'w'
或'a'
),然后使用write()
方法将内容写入文件。最后,使用close()
方法关闭文件以确保数据被保存。
2. Python中可以生成哪些类型的文件?
Python可以生成多种类型的文件,包括文本文件(.txt)、CSV文件(.csv)、JSON文件(.json)、Excel文件(.xlsx)、甚至是PDF文件(.pdf)。用户可以根据需要选择合适的库和格式来生成所需的文件类型。
3. 如何在Python中处理文件生成时的异常情况?
在生成文件时,可以使用try-except
语句来处理可能出现的异常情况。例如,可以捕获文件无法打开、写入权限不足或路径错误等异常,并提供相应的错误提示或处理措施。这可以确保程序的健壮性和用户体验的流畅性。
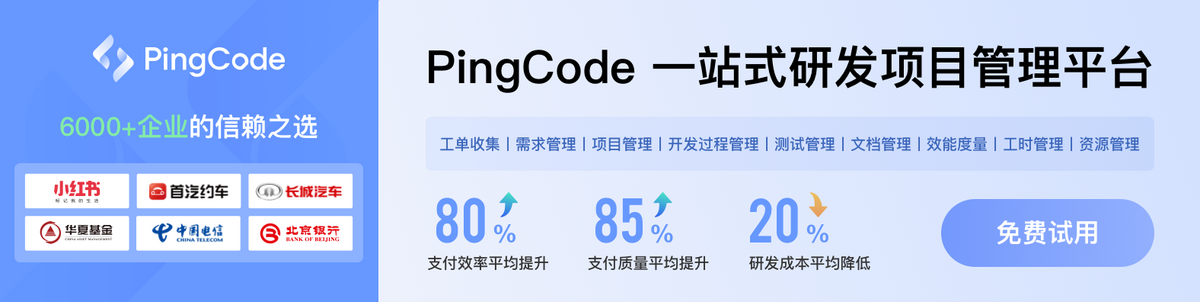