开头段落:
在Python中获取列表(list)的键(key)可以通过使用enumerate函数、循环遍历列表、以及使用字典等方法。其中,enumerate函数是最常见和推荐的方法,因为它可以在遍历列表的同时获取索引和元素。使用enumerate函数不仅简洁易懂,还能提高代码的可读性和效率。
详细描述enumerate函数的使用:
使用enumerate函数可以轻松获取列表的索引和元素。它返回一个枚举对象,该对象生成包含索引和值的元组。通过将这些元组解包,我们可以在循环中同时访问索引和值。例如:
my_list = ['a', 'b', 'c', 'd']
for index, value in enumerate(my_list):
print(f"Index: {index}, Value: {value}")
这段代码会输出:
Index: 0, Value: a
Index: 1, Value: b
Index: 2, Value: c
Index: 3, Value: d
一、使用enumerate函数获取列表的键
使用enumerate函数是获取列表索引和值的最常见方法。enumerate函数可以将一个可迭代对象(如列表)组合为一个索引序列,同时列出数据和数据下标,通常用于在for循环中获取索引和值。
my_list = ['apple', 'banana', 'cherry']
for index, value in enumerate(my_list):
print(f"Index: {index}, Value: {value}")
在这个例子中,enumerate函数将my_list中的每个元素与其索引组合成一个元组,并在for循环中解包。输出结果为:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
二、使用循环遍历列表获取键
尽管enumerate函数是最常见的方法,有时也可以使用传统的循环遍历列表来获取索引和值。我们可以使用range函数生成索引,并通过索引访问列表中的元素。
my_list = ['apple', 'banana', 'cherry']
for index in range(len(my_list)):
print(f"Index: {index}, Value: {my_list[index]}")
这段代码中,range(len(my_list))生成了一个从0到len(my_list)-1的索引序列,然后在for循环中使用这些索引访问列表中的元素。输出结果与上面的例子相同:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
三、使用字典将列表索引和值对应
有时候,我们可能需要将列表的索引和值存储在字典中,以便能够通过键快速访问值。我们可以使用字典推导式来创建这样的字典。
my_list = ['apple', 'banana', 'cherry']
index_value_dict = {index: value for index, value in enumerate(my_list)}
print(index_value_dict)
这段代码创建了一个字典,其中列表的索引作为键,列表的值作为字典的值。输出结果为:
{0: 'apple', 1: 'banana', 2: 'cherry'}
现在,我们可以通过索引快速访问列表中的元素:
print(index_value_dict[1]) # 输出:banana
四、获取嵌套列表的键
当处理嵌套列表时,我们可以使用递归的方法来获取每个子列表的索引和值。递归方法需要定义一个函数,该函数在遍历列表时检查每个元素是否为列表,如果是,则递归调用自身。
def get_nested_keys(lst, parent_key=''):
keys = []
for index, value in enumerate(lst):
current_key = f"{parent_key}.{index}" if parent_key else str(index)
if isinstance(value, list):
keys.extend(get_nested_keys(value, current_key))
else:
keys.append((current_key, value))
return keys
nested_list = [['apple', 'banana'], ['cherry', ['date', 'elderberry']]]
keys = get_nested_keys(nested_list)
for key, value in keys:
print(f"Key: {key}, Value: {value}")
这段代码定义了一个递归函数get_nested_keys来获取嵌套列表的索引和值,并将结果存储在一个列表中。输出结果为:
Key: 0.0, Value: apple
Key: 0.1, Value: banana
Key: 1.0, Value: cherry
Key: 1.1.0, Value: date
Key: 1.1.1, Value: elderberry
五、使用numpy获取列表的键
如果你在处理科学计算或需要高效地处理大规模数据,可以考虑使用numpy库。numpy提供了强大的数组对象和丰富的操作函数,可以方便地获取列表的索引和值。
import numpy as np
my_list = np.array(['apple', 'banana', 'cherry'])
indices = np.arange(len(my_list))
values = my_list[indices]
for index, value in zip(indices, values):
print(f"Index: {index}, Value: {value}")
这段代码使用numpy创建一个数组,并生成一个索引数组,然后通过索引数组访问列表中的元素。输出结果为:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
六、使用pandas获取列表的键
如果你在处理数据分析任务,可以考虑使用pandas库。pandas提供了强大的数据结构和数据分析工具,可以方便地获取列表的索引和值。
import pandas as pd
my_list = ['apple', 'banana', 'cherry']
df = pd.DataFrame(my_list, columns=['Value'])
print(df)
print(df.index)
for index, value in df.iterrows():
print(f"Index: {index}, Value: {value['Value']}")
这段代码使用pandas创建一个DataFrame,并打印其索引和值。输出结果为:
Value
0 apple
1 banana
2 cherry
Index([0, 1, 2], dtype='int64')
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
七、将列表转换为字典
有时候,我们可能需要将列表直接转换为字典,以便能够通过键快速访问值。可以使用dict和enumerate函数来实现这一点。
my_list = ['apple', 'banana', 'cherry']
index_value_dict = dict(enumerate(my_list))
print(index_value_dict)
这段代码将列表转换为字典,其中索引作为键,列表的值作为字典的值。输出结果为:
{0: 'apple', 1: 'banana', 2: 'cherry'}
现在,我们可以通过索引快速访问列表中的元素:
print(index_value_dict[1]) # 输出:banana
八、使用itertools模块获取键
itertools模块提供了许多高效的迭代器函数,适用于处理大数据集。我们可以使用itertools.islice函数来获取列表的索引和值。
import itertools
my_list = ['apple', 'banana', 'cherry']
for index, value in zip(itertools.count(), my_list):
print(f"Index: {index}, Value: {value}")
这段代码使用itertools.count生成一个无限递增的索引序列,并在for循环中与列表中的元素配对。输出结果为:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
九、使用map函数获取键
map函数可以将一个函数应用于一个可迭代对象的每个元素。我们可以使用map函数和lambda表达式来获取列表的索引和值。
my_list = ['apple', 'banana', 'cherry']
index_value_map = map(lambda index_value: (index_value[0], index_value[1]), enumerate(my_list))
for index, value in index_value_map:
print(f"Index: {index}, Value: {value}")
这段代码使用map函数将lambda表达式应用于enumerate函数生成的索引和值,并在for循环中解包。输出结果为:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
十、使用列表推导式获取键
列表推导式是一种简洁的方式,可以在一行代码中生成列表。我们可以使用列表推导式来生成包含索引和值的元组列表。
my_list = ['apple', 'banana', 'cherry']
index_value_list = [(index, value) for index, value in enumerate(my_list)]
for index, value in index_value_list:
print(f"Index: {index}, Value: {value}")
这段代码使用列表推导式生成一个包含索引和值的元组列表,并在for循环中解包。输出结果为:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
十一、使用生成器表达式获取键
生成器表达式是一种类似于列表推导式的语法,但它返回一个生成器对象,可以节省内存。我们可以使用生成器表达式来生成包含索引和值的元组。
my_list = ['apple', 'banana', 'cherry']
index_value_gen = ((index, value) for index, value in enumerate(my_list))
for index, value in index_value_gen:
print(f"Index: {index}, Value: {value}")
这段代码使用生成器表达式生成一个包含索引和值的元组生成器,并在for循环中解包。输出结果为:
Index: 0, Value: apple
Index: 1, Value: banana
Index: 2, Value: cherry
十二、获取复杂数据结构中的键
在处理复杂数据结构(如包含字典和列表的嵌套结构)时,我们可以使用递归函数来获取所有键和值。递归函数需要检查每个元素的类型,并根据类型决定如何处理。
def get_complex_keys(data, parent_key=''):
keys = []
if isinstance(data, dict):
for key, value in data.items():
current_key = f"{parent_key}.{key}" if parent_key else key
if isinstance(value, (dict, list)):
keys.extend(get_complex_keys(value, current_key))
else:
keys.append((current_key, value))
elif isinstance(data, list):
for index, value in enumerate(data):
current_key = f"{parent_key}.{index}" if parent_key else str(index)
if isinstance(value, (dict, list)):
keys.extend(get_complex_keys(value, current_key))
else:
keys.append((current_key, value))
return keys
complex_data = {'fruits': ['apple', 'banana', {'citrus': ['lemon', 'lime']}], 'vegetables': {'root': 'carrot', 'leafy': 'spinach'}}
keys = get_complex_keys(complex_data)
for key, value in keys:
print(f"Key: {key}, Value: {value}")
这段代码定义了一个递归函数get_complex_keys来获取复杂数据结构中的所有键和值,并将结果存储在一个列表中。输出结果为:
Key: fruits.0, Value: apple
Key: fruits.1, Value: banana
Key: fruits.2.citrus.0, Value: lemon
Key: fruits.2.citrus.1, Value: lime
Key: vegetables.root, Value: carrot
Key: vegetables.leafy, Value: spinach
总结:
在Python中获取列表的键有多种方法,包括使用enumerate函数、循环遍历列表、使用字典、处理嵌套列表、使用numpy、pandas、itertools、map函数、列表推导式、生成器表达式以及处理复杂数据结构。每种方法都有其优点和适用场景,可以根据具体需求选择合适的方法。enumerate函数是最常见和推荐的方法,因为它简洁易懂,适用于大多数情况。
相关问答FAQs:
如何在Python中获取列表(list)中的元素索引?
在Python中,获取列表中元素的索引可以使用list.index(value)
方法。这个方法会返回第一个匹配到的元素的索引值。如果需要获取所有匹配的元素索引,可以使用列表推导式和enumerate
函数来实现。例如:
my_list = ['apple', 'banana', 'cherry', 'banana']
indexes = [i for i, x in enumerate(my_list) if x == 'banana']
print(indexes) # 输出: [1, 3]
Python中的列表是否支持键值对的概念?
列表本身并不支持键值对的概念,它是一个有序的集合,主要通过索引来访问元素。如果需要使用键值对,可以考虑使用字典(dict)。字典允许通过键来快速访问相应的值。例如:
my_dict = {'name': 'Alice', 'age': 25}
print(my_dict['name']) # 输出: Alice
如何在Python中遍历列表并同时获取索引和元素?
使用enumerate()
函数可以轻松地遍历列表并获取索引和元素。这个函数会返回每个元素的索引和对应的值,适用于需要同时处理索引和元素的场景。示例如下:
my_list = ['apple', 'banana', 'cherry']
for index, value in enumerate(my_list):
print(f'Index: {index}, Value: {value}')
这种方式让代码变得简洁且易于理解。
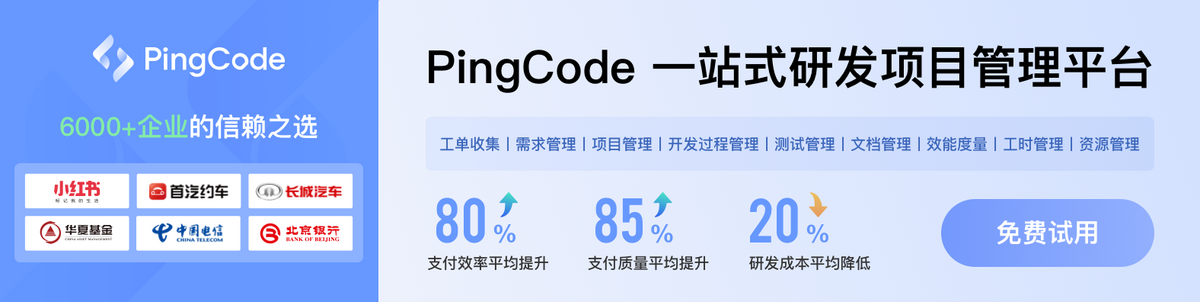