Spring管理整个项目的方法包括依赖注入、注解驱动开发、AOP(面向切面编程)、Spring Boot自动配置。 在Spring框架中,依赖注入是最核心的机制,它通过IoC容器来实现对象的创建、配置和管理。下面将详细介绍依赖注入的实现方式。
依赖注入(Dependency Injection)是一种设计模式,旨在通过外部注入依赖对象而不是在类内部创建它们。这种方法可以提高代码的可维护性和测试性。Spring通过IoC容器实现了依赖注入,主要有两种方式:构造器注入和Setter方法注入。构造器注入在对象创建时通过构造函数传递依赖对象,而Setter方法注入则通过Setter方法传递依赖对象。
一、依赖注入(DI)
依赖注入是Spring框架的核心部分,通过这种方式,Spring可以管理整个项目中的Bean对象及其依赖关系。
1、构造器注入
构造器注入是通过构造函数传递依赖对象的方式。Spring会在创建Bean实例时,通过构造函数传递依赖对象。
public class ExampleService {
private final ExampleRepository repository;
@Autowired
public ExampleService(ExampleRepository repository) {
this.repository = repository;
}
}
在配置类中,可以使用@Bean
注解来定义Bean:
@Configuration
public class AppConfig {
@Bean
public ExampleRepository exampleRepository() {
return new ExampleRepository();
}
@Bean
public ExampleService exampleService() {
return new ExampleService(exampleRepository());
}
}
2、Setter方法注入
Setter方法注入是通过Setter方法传递依赖对象的方式。Spring会在创建Bean实例后,通过Setter方法传递依赖对象。
public class ExampleService {
private ExampleRepository repository;
@Autowired
public void setRepository(ExampleRepository repository) {
this.repository = repository;
}
}
同样的,在配置类中定义Bean:
@Configuration
public class AppConfig {
@Bean
public ExampleRepository exampleRepository() {
return new ExampleRepository();
}
@Bean
public ExampleService exampleService() {
ExampleService service = new ExampleService();
service.setRepository(exampleRepository());
return service;
}
}
二、注解驱动开发
Spring提供了一系列注解,简化了配置和开发过程,使得开发者可以专注于业务逻辑。
1、@Component
和@Autowired
@Component
注解用于声明一个组件类,Spring会自动检测并注册这些组件。@Autowired
注解用于注入依赖对象。
@Component
public class ExampleRepository {
// ...
}
@Component
public class ExampleService {
@Autowired
private ExampleRepository repository;
// ...
}
2、@Service
、@Repository
和@Controller
Spring还提供了特定的组件注解,如@Service
、@Repository
和@Controller
,用于标识服务层、持久层和控制层的组件。
@Service
public class ExampleService {
// ...
}
@Repository
public class ExampleRepository {
// ...
}
@Controller
public class ExampleController {
// ...
}
三、面向切面编程(AOP)
AOP是一种编程范式,用于处理跨领域关注点,如日志记录、安全性、事务管理等。Spring AOP通过使用切面、通知和切点来实现。
1、定义切面和通知
使用@Aspect
注解定义切面,并使用@Before
、@After
、@Around
等注解定义通知。
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void logBefore(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature().getName());
}
@After("execution(* com.example.service.*.*(..))")
public void logAfter(JoinPoint joinPoint) {
System.out.println("After method: " + joinPoint.getSignature().getName());
}
}
2、配置AOP
在配置类中启用AOP:
@Configuration
@EnableAspectJAutoProxy
public class AppConfig {
// ...
}
四、Spring Boot自动配置
Spring Boot通过自动配置简化了Spring应用的开发,无需手动配置大量的Bean和依赖。
1、使用Spring Boot Starter
Spring Boot提供了一系列Starter依赖,涵盖常用的功能和技术栈。通过引入这些Starter依赖,可以快速搭建应用。
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
2、应用主类
使用@SpringBootApplication
注解标识应用主类,该注解包含了@Configuration
、@EnableAutoConfiguration
和@ComponentScan
。
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
五、Spring Data JPA
Spring Data JPA简化了数据访问层的开发,通过提供一系列注解和接口,使得开发者可以专注于业务逻辑。
1、定义实体类
使用@Entity
注解定义实体类,并使用@Id
注解标识主键。
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// getters and setters
}
2、定义仓库接口
使用@Repository
注解定义仓库接口,继承JpaRepository
接口。
@Repository
public interface UserRepository extends JpaRepository<User, Long> {
User findByEmail(String email);
}
六、Spring MVC
Spring MVC是Spring框架的一部分,用于开发Web应用,通过使用一系列注解和接口,简化了控制层的开发。
1、定义控制器
使用@Controller
注解定义控制器类,并使用@RequestMapping
注解映射请求路径。
@Controller
public class UserController {
@Autowired
private UserService userService;
@RequestMapping("/users")
public String getUsers(Model model) {
List<User> users = userService.getAllUsers();
model.addAttribute("users", users);
return "userList";
}
}
2、配置视图解析器
在配置类中定义视图解析器,指定视图文件的前缀和后缀。
@Configuration
@EnableWebMvc
public class WebConfig implements WebMvcConfigurer {
@Bean
public InternalResourceViewResolver viewResolver() {
InternalResourceViewResolver resolver = new InternalResourceViewResolver();
resolver.setPrefix("/WEB-INF/views/");
resolver.setSuffix(".jsp");
return resolver;
}
}
七、Spring Security
Spring Security是一套强大的安全框架,用于保护应用程序的安全性。它提供了一系列配置和注解,用于简化身份验证和授权。
1、配置安全性
在配置类中定义安全性配置,继承WebSecurityConfigurerAdapter
类。
@Configuration
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.authorizeRequests()
.antMatchers("/public/").permitAll()
.anyRequest().authenticated()
.and()
.formLogin().loginPage("/login").permitAll()
.and()
.logout().permitAll();
}
@Autowired
public void configureGlobal(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password("{noop}password").roles("USER");
}
}
2、定义登录页面
创建一个登录页面,并在控制器中映射登录请求。
@Controller
public class LoginController {
@RequestMapping("/login")
public String login() {
return "login";
}
}
八、Spring Cloud
Spring Cloud是一系列工具和框架的集合,用于构建分布式系统和微服务架构。它提供了服务发现、配置管理、负载均衡等功能。
1、服务发现和注册
使用Eureka作为服务发现和注册中心,在配置类中启用Eureka客户端。
@SpringBootApplication
@EnableEurekaClient
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
在配置文件中指定Eureka服务器地址:
eureka:
client:
service-url:
defaultZone: http://localhost:8761/eureka/
2、负载均衡
使用Ribbon实现客户端负载均衡,通过@LoadBalanced
注解启用负载均衡。
@Configuration
public class RibbonConfig {
@Bean
@LoadBalanced
public RestTemplate restTemplate() {
return new RestTemplate();
}
}
在服务中使用RestTemplate
进行服务调用:
@Service
public class UserService {
@Autowired
private RestTemplate restTemplate;
public User getUserById(Long id) {
return restTemplate.getForObject("http://user-service/users/" + id, User.class);
}
}
九、Spring Batch
Spring Batch是一个处理大数据批处理框架,提供了强大的批处理能力,如事务管理、并发处理和重试机制等。
1、配置批处理任务
在配置类中定义批处理任务,继承DefaultBatchConfigurer
类。
@Configuration
@EnableBatchProcessing
public class BatchConfig extends DefaultBatchConfigurer {
@Bean
public Job job(JobBuilderFactory jobBuilderFactory, StepBuilderFactory stepBuilderFactory) {
Step step = stepBuilderFactory.get("step1")
.<String, String>chunk(10)
.reader(reader())
.processor(processor())
.writer(writer())
.build();
return jobBuilderFactory.get("job1")
.start(step)
.build();
}
@Bean
public ItemReader<String> reader() {
return new ListItemReader<>(Arrays.asList("item1", "item2", "item3"));
}
@Bean
public ItemProcessor<String, String> processor() {
return item -> "processed " + item;
}
@Bean
public ItemWriter<String> writer() {
return items -> items.forEach(System.out::println);
}
}
2、运行批处理任务
在应用主类中运行批处理任务:
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
十、Spring Integration
Spring Integration是一套用于构建消息驱动系统的框架,提供了消息通道、网关和适配器等功能。
1、配置消息通道
在配置类中定义消息通道和消息处理器。
@Configuration
@EnableIntegration
public class IntegrationConfig {
@Bean
public MessageChannel inputChannel() {
return new DirectChannel();
}
@Bean
public MessageChannel outputChannel() {
return new DirectChannel();
}
@Bean
public IntegrationFlow integrationFlow() {
return IntegrationFlows.from(inputChannel())
.transform(String.class, String::toUpperCase)
.channel(outputChannel())
.get();
}
}
2、使用消息网关
定义消息网关接口,通过注解指定输入和输出通道。
@MessagingGateway
public interface MyGateway {
@Gateway(requestChannel = "inputChannel", replyChannel = "outputChannel")
String sendMessage(String message);
}
在服务中使用消息网关发送消息:
@Service
public class MyService {
@Autowired
private MyGateway myGateway;
public void processMessage(String message) {
String response = myGateway.sendMessage(message);
System.out.println("Response: " + response);
}
}
总结
Spring框架通过依赖注入、注解驱动开发、AOP、Spring Boot自动配置等机制,提供了强大的功能和灵活性,简化了项目的管理和开发。通过使用这些功能,开发者可以更加专注于业务逻辑,提高开发效率和代码质量。Spring还提供了一系列扩展框架,如Spring Data JPA、Spring MVC、Spring Security、Spring Cloud、Spring Batch和Spring Integration,进一步增强了Spring的功能和适用范围。
相关问答FAQs:
如何使用Spring框架提高项目的管理效率?
Spring框架通过提供一系列的功能,如依赖注入、面向切面编程和模块化设计,帮助开发者更高效地管理项目。通过使用Spring的IoC容器,开发者能够轻松管理对象的生命周期和依赖关系,从而减少了代码之间的耦合度,提升了项目的可维护性和扩展性。
Spring的模块化设计对项目管理有什么帮助?
Spring框架的模块化设计允许开发者根据项目需求选择不同的模块进行使用,如Spring MVC、Spring Data等。这种灵活性使得项目能够根据不同的功能需求进行扩展,同时避免了不必要的复杂性,帮助团队更专注于核心业务逻辑。
在使用Spring时,如何确保项目的可测试性?
Spring框架提供了强大的支持以提升项目的可测试性。通过使用依赖注入,开发者可以轻松地替换出需要测试的组件,进而进行单元测试和集成测试。此外,Spring还支持Mock对象的创建,帮助开发者在测试中模拟不同的场景,确保项目在上线前具备良好的稳定性。
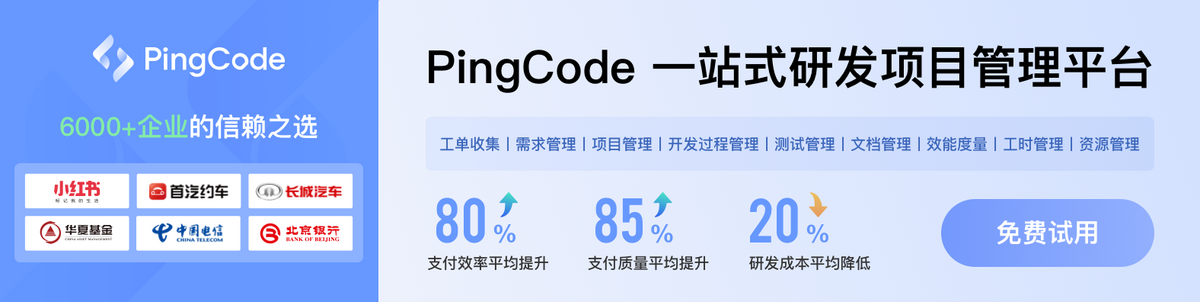