在Vue项目管理器中设置路径的方法包括:配置vue.config.js
、使用别名、配置router
和正确管理静态资源路径。其中,配置vue.config.js
文件是最常见也是最重要的步骤,通过这个文件可以对Vue项目的构建和开发环境进行全局配置。
在这篇文章中,我们将详细讨论如何在Vue项目管理器中正确设置路径。我们会从基础到高级,逐步讲解不同的路径设置方法,包括配置vue.config.js
文件、使用路径别名、配置Vue Router以及管理静态资源路径。
一、配置vue.config.js
配置vue.config.js
文件是Vue项目路径管理的重要步骤。通过这个文件,我们可以设置项目的基本路径,配置代理和其他全局设置。
1、基本路径配置
在Vue CLI 3及更高版本中,vue.config.js
文件用于配置项目的基本路径。以下是一个示例:
module.exports = {
publicPath: process.env.NODE_ENV === 'production' ? '/production-sub-path/' : '/'
}
在这个配置中,publicPath
属性用于设置项目的基础路径。当项目在生产环境中运行时,基础路径将被设置为/production-sub-path/
,在开发环境中则为根路径/
。
2、配置路径别名
使用路径别名可以让我们在项目中更方便地引用文件和模块。以下是一个示例:
const path = require('path');
module.exports = {
configureWebpack: {
resolve: {
alias: {
'@': path.resolve(__dirname, 'src'),
'@components': path.resolve(__dirname, 'src/components'),
'@assets': path.resolve(__dirname, 'src/assets')
}
}
}
}
在这个配置中,我们通过alias
属性设置了几个路径别名。现在,我们可以使用这些别名来引用文件和模块,例如:
import MyComponent from '@components/MyComponent.vue';
import MyImage from '@assets/my-image.png';
这种方法可以大大简化路径的引用,特别是当项目结构较复杂时。
二、使用别名
使用路径别名是管理Vue项目路径的另一种有效方法。它可以简化文件和模块的引用,使代码更加清晰和易于维护。
1、配置路径别名
我们可以在vue.config.js
文件中配置路径别名。以下是一个示例:
const path = require('path');
module.exports = {
configureWebpack: {
resolve: {
alias: {
'@': path.resolve(__dirname, 'src'),
'@components': path.resolve(__dirname, 'src/components'),
'@assets': path.resolve(__dirname, 'src/assets')
}
}
}
}
在这个配置中,我们通过alias
属性设置了几个路径别名。现在,我们可以使用这些别名来引用文件和模块,例如:
import MyComponent from '@components/MyComponent.vue';
import MyImage from '@assets/my-image.png';
2、在文件中使用别名
在配置好路径别名后,我们可以在项目中的任何地方使用这些别名来引用文件和模块。这不仅简化了代码,还提高了可读性和维护性。
三、配置Vue Router
配置Vue Router是管理Vue项目路径的另一个重要方面。通过Vue Router,我们可以设置项目的路由规则和导航守卫。
1、基本路由配置
以下是一个示例,展示了如何配置基本的Vue Router:
import Vue from 'vue';
import Router from 'vue-router';
import Home from '@/components/Home.vue';
import About from '@/components/About.vue';
Vue.use(Router);
export default new Router({
mode: 'history',
routes: [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/about',
name: 'About',
component: About
}
]
});
在这个配置中,我们使用了history
模式,使得URL看起来更像常规的Web路径。我们还设置了两个路由,分别对应首页和关于页。
2、嵌套路由配置
对于复杂的应用,我们可能需要配置嵌套路由。以下是一个示例:
import Vue from 'vue';
import Router from 'vue-router';
import MainLayout from '@/layouts/MainLayout.vue';
import Dashboard from '@/views/Dashboard.vue';
import Settings from '@/views/Settings.vue';
Vue.use(Router);
export default new Router({
mode: 'history',
routes: [
{
path: '/',
component: MainLayout,
children: [
{
path: '',
name: 'Dashboard',
component: Dashboard
},
{
path: 'settings',
name: 'Settings',
component: Settings
}
]
}
]
});
在这个配置中,我们使用了嵌套路由,将Dashboard
和Settings
视图嵌套在MainLayout
布局中。
四、管理静态资源路径
在Vue项目中,管理静态资源路径也是一个重要的方面。我们需要确保项目中的静态资源(如图片、字体和样式表)能够正确加载。
1、使用相对路径
在引用静态资源时,最好使用相对路径。例如:
<img src="@/assets/logo.png" alt="Logo">
这种方法可以确保静态资源在不同环境中都能正确加载。
2、配置vue.config.js
我们还可以通过vue.config.js
文件配置静态资源的加载路径。以下是一个示例:
module.exports = {
assetsDir: 'static'
}
在这个配置中,我们将静态资源目录设置为static
。这样,所有静态资源都将被放置在static
目录中。
3、使用Webpack加载器
在一些情况下,我们可能需要使用Webpack加载器来处理静态资源。例如,我们可以使用file-loader
和url-loader
来加载图片和字体:
module.exports = {
module: {
rules: [
{
test: /\.(png|jpg|gif)$/i,
use: [
{
loader: 'url-loader',
options: {
limit: 8192,
name: 'img/[name].[hash:7].[ext]'
}
}
]
},
{
test: /\.(woff|woff2|eot|ttf|otf)$/i,
use: [
{
loader: 'file-loader',
options: {
name: 'fonts/[name].[hash:7].[ext]'
}
}
]
}
]
}
}
在这个配置中,我们使用url-loader
处理图片,当图片大小小于8KB时,会被转换为Base64编码。我们还使用file-loader
处理字体文件,并将它们放置在fonts
目录中。
五、总结
在Vue项目管理器中设置路径是一个重要的任务,它直接影响到项目的可维护性和可扩展性。通过配置vue.config.js
文件、使用路径别名、配置Vue Router以及正确管理静态资源路径,我们可以确保项目在不同环境中都能正常运行。
希望这篇文章对你有所帮助。如果你有任何问题或建议,请随时留言讨论。
相关问答FAQs:
如何在Vue项目管理器中设置路径?
在Vue项目中,路径设置通常涉及到配置webpack或vue.config.js文件。为了更好地管理资源和路由,您可以在vue.config.js中设置“publicPath”属性,确保资源能够正确加载。具体操作是打开vue.config.js文件,添加如下代码:
module.exports = {
publicPath: process.env.NODE_ENV === 'production' ? '/your-path/' : '/'
}
这样可以根据不同环境动态设置路径。
如何处理Vue项目中的相对路径问题?
在开发过程中,使用相对路径可能会导致资源加载错误。建议使用绝对路径,确保无论在哪个组件中引用资源都能正确找到。可以通过设置“resolve.alias”来简化路径,例如:
const path = require('path');
module.exports = {
configureWebpack: {
resolve: {
alias: {
'@': path.resolve(__dirname, 'src')
}
}
}
}
通过这种方式,您可以使用@/assets
来引用assets文件夹中的资源,避免相对路径带来的问题。
Vue项目中如何管理路由路径?
在Vue Router中,您可以通过定义路由路径来管理应用的不同页面。路由配置通常在src/router/index.js
文件中进行。在这里,您可以为每个组件分配路径。例如:
import Vue from 'vue';
import Router from 'vue-router';
import Home from '@/views/Home.vue';
import About from '@/views/About.vue';
Vue.use(Router);
export default new Router({
routes: [
{
path: '/',
name: 'Home',
component: Home
},
{
path: '/about',
name: 'About',
component: About
}
]
});
通过这种方式,您可以轻松管理不同页面的访问路径,确保用户能够方便地导航。
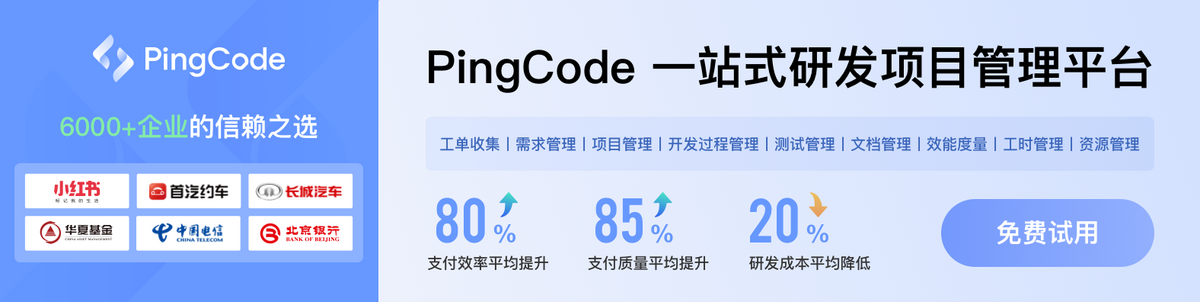