使用python-docx库在Word文档的指定位置添加表格需要先定位到相应位置,然后在该位置插入表格。通常,这可以通过在指定位置插入一个段落作为锚点,再在该段落之前或之后添加表格来实现。我们可以使用Bookmarks(书签)、Paragraphs(段落)或Search(搜索)功能来找到特定位置,或者在文档的末尾添加表格。
在Word文档中,我们通常可以利用python-docx的功能进行灵活操作,比如向现有表格中插入行或列,或者在文档的某个特定段落后面添加一个表格,以满足不同的应用场景。
一、定位并添加表格
首先,我们需要找到文档中想要插入表格的确切位置。如果文档中有特定的书签或者可辨识的段落文本,我们可以用它们作为锚点。
通过书签添加表格:
from docx import Document
打开文档
doc = Document('existing-document.docx')
设定你要添加表格的书签名
bookmark_name = "MyBookmark"
遍历文档中的所有段落
for paragraph in doc.paragraphs:
if bookmark_name in [run.text for run in paragraph.runs]:
# 在锚点段落前添加表格
table = doc.add_table(rows=2, cols=2)
# 填充表格数据
for row in table.rows:
for cell in row.cells:
cell.text = '示例文本'
保存更改后的文档
doc.save('modified-document.docx')
通过段落文本添加表格:
from docx import Document
打开文档
doc = Document('existing-document.docx')
定义你想在哪个段落后添加表格的文本内容
paragraph_text = "特定段落的文本"
定位特定段落
for paragraph in doc.paragraphs:
if paragraph_text in paragraph.text:
# 找到对应段落,我们可以创建一个新段落,以便在后面添加表格
new_paragraph = doc.add_paragraph()
# 在特定段落之后添加表格
table = new_paragraph.add_table(rows=2, cols=2)
# 填充表格数据
for row in table.rows:
for cell in row.cells:
cell.text = '示例文本'
break
保存文档
doc.save('modified-document.docx')
二、表格的创建与格式化
一旦定位到希望插入表格的位置,就可以创建一个新表格以及定义其样式。
创建表格:
# 创建一个2行2列的表格
table = doc.add_table(rows=2, cols=2)
给表格中的单元格赋值
for row in table.rows:
for cell in row.cells:
cell.text = '新的文本值'
格式化表格:
python-docx允许你对表格进行一些基本的样式设置,例如设置边框、单元格大小和表格对齐方式。
from docx.shared import Pt
from docx.enum.text import WD_ALIGN_PARAGRAPH
设置表格的样式
table.style = 'Table Grid'
遍历表中的所有单元格,并设置字体大小
for row in table.rows:
for cell in row.cells:
for paragraph in cell.paragraphs:
paragraph.alignment = WD_ALIGN_PARAGRAPH.CENTER # 对齐方式设置为居中
for run in paragraph.runs:
run.font.size= Pt(12) # 字体大小设置为12磅
保存文档
doc.save('styled-document.docx')
三、单元格的操作
在创建并格式化表格后,还可能需要对表格内的单元格进行进一步的操作,如合并单元格、拆分单元格等。
合并单元格:
# 合并第一行的单元格
a = table.cell(0,0)
b = table.cell(0,1)
A.merge(B)
分割单元格:
python-docx不直接支持拆分单元格,它通常通过先删除单元格再创建新行列来实现。
四、行与列的操作
最后,我们经常需要在表格中添加或删除行列。
插入行列:
# 添加行到表格末尾
new_row = table.add_row()
在第一列前添加一列
new_column_cells = [row.cells[0].insert_paragraph_before() for row in table.rows]
删除行列:
删除行列通常需要利用python-docx的底层操作,这可能相对复杂而且不被官方推荐。
添加表格至特定位置是通过综合运用python-docx库的多项功能实现的。通过定位、创建、格式化和操作单元格及行列,你可以在Word文档中灵活地插入和修改表格,以适应各种需要。
相关问答FAQs:
1. 如何在指定位置使用python-docx添加表格?
要在指定位置使用python-docx添加表格,可以按照以下步骤进行操作:
- 首先,导入所需的模块:
from docx import Document
- 其次,创建一个新的
Document
对象:doc = Document()
- 然后,使用
add_table()
方法在指定位置插入表格:table = doc.add_table(rows, cols)
- 最后,根据需要,可以进一步对表格进行格式设置和填充数据。
以下是一个示例代码:
from docx import Document
# 创建一个新的Document对象
doc = Document()
# 在指定位置插入表格(例如在第1段的最后一个位置)
table = doc.add_table(rows=3, cols=3)
# 对表格进行格式设置和填充数据
for i in range(3):
for j in range(3):
cell = table.cell(i, j)
cell.text = f"第{i+1}行,第{j+1}列"
# 最后保存文档
doc.save('output.docx')
这样,你就可以在指定位置成功添加表格了。
2. 如何在指定段落末尾使用python-docx添加表格?
要在指定段落的末尾添加表格,可以按照以下步骤进行操作:
- 首先,找到目标段落:
target_paragraph = doc.paragraphs[index]
(其中index
为目标段落的索引) - 其次,使用
insert_paragraph_before()
方法在目标段落之前插入一个新的段落:new_paragraph = target_paragraph.insert_paragraph_before()
- 然后,在新段落中使用
add_table()
方法添加表格:table = new_paragraph.add_table(rows, cols)
- 最后,根据需要,可以进一步对表格进行格式设置和填充数据。
以下是一个示例代码:
from docx import Document
# 打开现有的文档
doc = Document('existing_doc.docx')
# 找到目标段落的索引
index = 1
# 找到目标段落
target_paragraph = doc.paragraphs[index]
# 在目标段落之前插入一个新的段落
new_paragraph = target_paragraph.insert_paragraph_before()
# 在新段落中添加表格
table = new_paragraph.add_table(rows=3, cols=3)
# 对表格进行格式设置和填充数据
for i in range(3):
for j in range(3):
cell = table.cell(i, j)
cell.text = f"第{i+1}行,第{j+1}列"
# 最后保存文档
doc.save('output.docx')
这样,你就可以在指定段落末尾成功添加表格了。
3. 如何在指定页码处使用python-docx添加表格?
要在指定页码处添加表格,可以按照以下步骤进行操作:
- 首先,找到目标页码所在的段落:
target_paragraph = doc.paragraphs[index]
(其中index
为目标页的第一个段落的索引) - 其次,使用
insert_paragraph_before()
方法在目标段落之前插入一个新的段落:new_paragraph = target_paragraph.insert_paragraph_before()
- 然后,在新段落中使用
add_table()
方法添加表格:table = new_paragraph.add_table(rows, cols)
- 最后,根据需要,可以进一步对表格进行格式设置和填充数据。
以下是一个示例代码:
from docx import Document
# 打开现有的文档
doc = Document('existing_doc.docx')
# 找到目标页的第一个段落的索引
index = 10
# 找到目标页码所在的段落
target_paragraph = doc.paragraphs[index]
# 在目标段落之前插入一个新的段落
new_paragraph = target_paragraph.insert_paragraph_before()
# 在新段落中添加表格
table = new_paragraph.add_table(rows=3, cols=3)
# 对表格进行格式设置和填充数据
for i in range(3):
for j in range(3):
cell = table.cell(i, j)
cell.text = f"第{i+1}行,第{j+1}列"
# 最后保存文档
doc.save('output.docx')
这样,你就可以在指定页码处成功添加表格了。
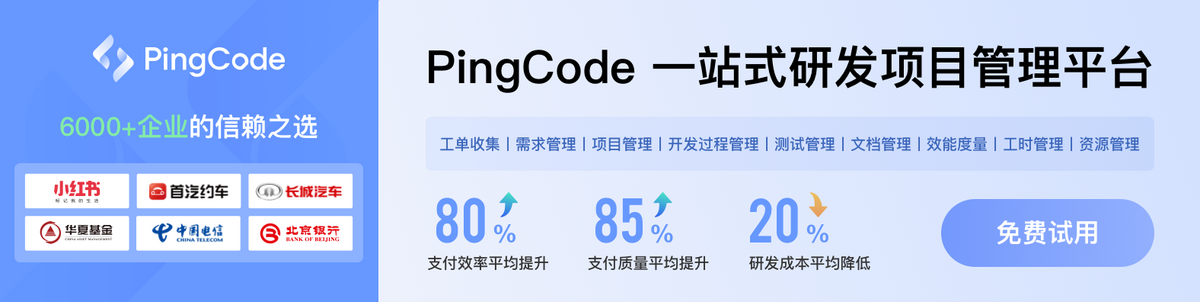