使用Python下载图片的几种方法包括:使用requests库、使用urllib库、结合BeautifulSoup抓取网页中的图片链接、使用多线程提高下载效率。其中,使用requests库是一种简单直接的方法,适合初学者。requests库可以通过发送HTTP请求来获取图片数据,并将其保存到本地。以下是详细的介绍。
一、使用requests库下载图片
requests库是Python中非常流行的HTTP请求库,它提供了简单易用的接口,使得我们可以轻松地发送HTTP请求并处理响应。使用requests库下载图片的步骤如下:
- 安装requests库
在使用requests库之前,需要先安装它。如果尚未安装,可以使用以下命令进行安装:
pip install requests
- 下载图片
要下载图片,首先需要获取图片的URL,然后使用requests.get()方法发送GET请求获取图片数据。接着,将获取到的图片数据写入文件中。以下是一个示例代码:
import requests
def download_image(url, file_name):
try:
# 发送GET请求获取图片数据
response = requests.get(url)
# 检查请求是否成功
if response.status_code == 200:
# 以二进制写入方式打开文件
with open(file_name, 'wb') as file:
file.write(response.content)
print(f"Image successfully downloaded: {file_name}")
else:
print(f"Failed to download image. Status code: {response.status_code}")
except Exception as e:
print(f"An error occurred: {e}")
示例使用
image_url = "https://example.com/image.jpg"
download_image(image_url, "downloaded_image.jpg")
在上述代码中,我们定义了一个名为download_image
的函数,该函数接受图片的URL和保存文件名作为参数。我们使用requests.get()方法获取图片数据,并将其写入本地文件中。
二、使用urllib库下载图片
urllib是Python内置的用于操作URL的库,其中的urlretrieve函数可以直接用于下载文件,包括图片。以下是使用urllib库下载图片的步骤:
- 导入urllib库
urllib库是Python的标准库,因此不需要额外安装。直接导入即可:
import urllib.request
- 下载图片
使用urllib.request.urlretrieve()方法下载图片,并指定保存路径。以下是示例代码:
import urllib.request
def download_image(url, file_name):
try:
# 使用urlretrieve下载图片
urllib.request.urlretrieve(url, file_name)
print(f"Image successfully downloaded: {file_name}")
except Exception as e:
print(f"An error occurred: {e}")
示例使用
image_url = "https://example.com/image.jpg"
download_image(image_url, "downloaded_image.jpg")
在这个示例中,我们使用urllib.request.urlretrieve()方法下载图片,并直接指定了保存路径。
三、结合BeautifulSoup抓取网页中的图片链接
BeautifulSoup是一个可以从HTML或XML文件中提取数据的Python库。它通常与requests库结合使用,用于抓取网页中的图片链接。以下是使用BeautifulSoup抓取网页中的图片链接并下载图片的步骤:
- 安装BeautifulSoup库
使用以下命令安装BeautifulSoup库:
pip install beautifulsoup4
- 抓取网页中的图片链接并下载图片
首先,使用requests库获取网页的HTML内容,然后使用BeautifulSoup解析HTML并提取图片链接。接着,下载图片。以下是示例代码:
import requests
from bs4 import BeautifulSoup
def download_images_from_webpage(url):
try:
# 获取网页内容
response = requests.get(url)
soup = BeautifulSoup(response.content, 'html.parser')
# 提取所有图片标签
img_tags = soup.find_all('img')
# 下载每个图片
for index, img in enumerate(img_tags):
img_url = img.get('src')
if img_url:
download_image(img_url, f"image_{index}.jpg")
except Exception as e:
print(f"An error occurred: {e}")
def download_image(url, file_name):
try:
response = requests.get(url)
if response.status_code == 200:
with open(file_name, 'wb') as file:
file.write(response.content)
print(f"Image successfully downloaded: {file_name}")
else:
print(f"Failed to download image. Status code: {response.status_code}")
except Exception as e:
print(f"An error occurred: {e}")
示例使用
webpage_url = "https://example.com"
download_images_from_webpage(webpage_url)
在这个示例中,我们定义了一个download_images_from_webpage
函数,该函数接受网页URL作为参数。我们使用requests库获取网页内容,并使用BeautifulSoup解析HTML。然后,我们遍历所有的标签,提取其src属性中的图片链接,并调用
download_image
函数下载图片。
四、使用多线程提高下载效率
在下载大量图片时,使用多线程可以显著提高下载效率。Python的threading模块可以帮助我们实现多线程下载。以下是使用多线程下载图片的步骤:
- 导入threading模块
import threading
- 使用多线程下载图片
我们可以为每个图片下载任务创建一个线程,并启动多个线程同时下载图片。以下是示例代码:
import requests
import threading
def download_image(url, file_name):
try:
response = requests.get(url)
if response.status_code == 200:
with open(file_name, 'wb') as file:
file.write(response.content)
print(f"Image successfully downloaded: {file_name}")
else:
print(f"Failed to download image. Status code: {response.status_code}")
except Exception as e:
print(f"An error occurred: {e}")
def download_images_concurrently(urls):
threads = []
for index, url in enumerate(urls):
file_name = f"image_{index}.jpg"
# 为每个下载任务创建线程
thread = threading.Thread(target=download_image, args=(url, file_name))
threads.append(thread)
thread.start()
# 等待所有线程完成
for thread in threads:
thread.join()
示例使用
image_urls = [
"https://example.com/image1.jpg",
"https://example.com/image2.jpg",
"https://example.com/image3.jpg"
]
download_images_concurrently(image_urls)
在这个示例中,我们定义了一个download_images_concurrently
函数,该函数接受一个图片URL列表作为参数。我们为每个下载任务创建一个线程,并启动线程进行下载。最后,我们等待所有线程完成下载任务。
通过以上几种方法,您可以使用Python轻松下载图片。根据具体需求选择合适的方法,可以帮助您高效地完成图片下载任务。无论是简单的单张图片下载,还是复杂的网页图片抓取和多线程批量下载,Python都能为您提供强大的工具和支持。
相关问答FAQs:
如何使用Python下载网络图片?
使用Python下载网络图片非常简单,您可以使用requests
库来获取图片并使用open()
函数保存到本地。首先,您需要安装requests
库,然后编写代码获取图片。例如:
import requests
url = '图片的URL'
response = requests.get(url)
with open('保存的文件名.jpg', 'wb') as file:
file.write(response.content)
确保替换url
和文件名为您需要的内容。
下载图片时,如何处理图片的异常情况?
在下载图片时,可能会遇到各种异常,例如网络问题、URL错误或文件权限问题。为了解决这些问题,可以使用try-except
语句来捕获异常并进行处理。例如:
try:
response = requests.get(url)
response.raise_for_status() # 检查请求是否成功
with open('保存的文件名.jpg', 'wb') as file:
file.write(response.content)
except requests.exceptions.RequestException as e:
print(f"下载图片时发生错误: {e}")
这样可以确保在下载过程中遇到问题时,程序不会崩溃,并能输出相关错误信息。
我可以使用哪些Python库来下载图片?
除了requests
库,您还可以使用urllib
和Pillow
等库来下载和处理图片。urllib
是Python标准库的一部分,非常适合进行简单的下载任务:
import urllib.request
url = '图片的URL'
urllib.request.urlretrieve(url, '保存的文件名.jpg')
而Pillow
库则可以帮助您下载并进行图片处理,例如调整大小、裁剪等功能,适合需要对图片进行后期处理的情况。选择适合您的需求的库即可。
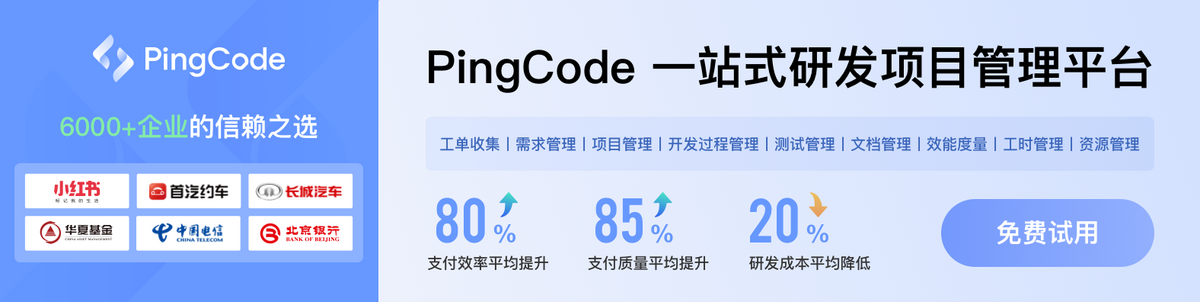