在Python中,销毁线程通常涉及通过让线程完成其任务来终止它、设置线程标志以通知其停止、使用适当的线程管理工具。直接销毁线程是不安全的,因为它可能会导致资源泄漏或数据不一致。一种常用的方法是设置一个标志,线程会定期检查这个标志,如果设置了终止标志,线程就会安全地退出。
一、线程在Python中的管理
Python的threading
模块提供了一种用于创建和管理线程的简单方式。线程是程序执行的最小单元,多线程可以提高程序的并发性和响应速度。在多线程编程中,我们需要考虑如何优雅地终止线程。直接强制终止线程是不推荐的,因为这可能会导致一些未释放的资源或中间状态。
-
线程的创建与启动
在Python中,可以通过
threading.Thread
创建一个新的线程。线程的目标函数中可以包含需要执行的任务。当我们启动线程时,它将开始并发执行目标函数中的代码。import threading
import time
def task():
while True:
print("Thread is running...")
time.sleep(1)
t = threading.Thread(target=task)
t.start()
-
线程的安全退出
在线程中,我们可以使用一个标志来控制线程的退出。通过在线程的执行循环中定期检查这个标志,可以实现线程的安全退出。这种方式避免了强制终止线程带来的潜在问题。
import threading
import time
class StoppableThread(threading.Thread):
def __init__(self):
super().__init__()
self._stop_event = threading.Event()
def run(self):
while not self._stop_event.is_set():
print("Thread is running...")
time.sleep(1)
def stop(self):
self._stop_event.set()
t = StoppableThread()
t.start()
time.sleep(5) # 让线程运行一段时间
t.stop() # 通过设置标志安全地停止线程
t.join() # 等待线程结束
二、使用标志停止线程
使用标志停止线程是一种常见的方式,这种方法简单且安全。通过在线程中定期检查标志状态,我们可以控制线程何时退出。
-
定义停止标志
可以使用Python的
threading.Event
类来作为停止标志。这个类提供了一种线程安全的方法来设置和检查标志状态。import threading
class MyThread(threading.Thread):
def __init__(self):
super().__init__()
self._stop_event = threading.Event()
def run(self):
while not self._stop_event.is_set():
# 执行任务
pass
def stop(self):
self._stop_event.set()
-
检查标志并停止线程
在线程的
run
方法中,我们可以定期检查标志状态。如果标志已设置,线程将退出执行循环,从而实现安全停止。import time
t = MyThread()
t.start()
time.sleep(5) # 线程运行5秒
t.stop() # 停止线程
t.join() # 等待线程结束
三、线程管理工具的使用
Python的concurrent.futures
模块提供了一种高级的线程管理方式,使用线程池可以更方便地管理和终止线程。
-
线程池的创建
通过
concurrent.futures.ThreadPoolExecutor
可以创建一个线程池,并提交任务到线程池中。from concurrent.futures import ThreadPoolExecutor
import time
def task():
while True:
print("Task is running...")
time.sleep(1)
with ThreadPoolExecutor(max_workers=1) as executor:
future = executor.submit(task)
-
线程池的关闭
当线程池不再需要时,可以调用其
shutdown
方法来停止线程池中的所有线程。with ThreadPoolExecutor(max_workers=1) as executor:
future = executor.submit(task)
time.sleep(5) # 让任务运行一段时间
executor.shutdown(wait=True) # 停止所有线程
四、线程的安全性与同步
在多线程编程中,线程安全性和同步是两个重要的方面。我们需要确保多个线程不会同时访问共享资源,从而避免数据不一致的问题。
-
线程锁的使用
Python的
threading.Lock
类提供了一种简单的锁机制,用于保护共享资源。在访问共享资源时,线程可以获取锁,从而确保其他线程无法同时访问。import threading
counter = 0
lock = threading.Lock()
def increment():
global counter
with lock: # 获取锁
counter += 1
t1 = threading.Thread(target=increment)
t2 = threading.Thread(target=increment)
t1.start()
t2.start()
t1.join()
t2.join()
-
条件变量的使用
条件变量允许线程在某个条件不满足时等待,并在条件满足后继续执行。这对于需要协调多个线程的场景非常有用。
condition = threading.Condition()
def wait_for_event():
with condition:
condition.wait() # 等待条件满足
print("Event occurred!")
def trigger_event():
with condition:
condition.notify() # 通知等待线程
t1 = threading.Thread(target=wait_for_event)
t2 = threading.Thread(target=trigger_event)
t1.start()
t2.start()
t1.join()
t2.join()
五、处理线程异常
在多线程编程中,处理线程异常是一个重要的方面。未处理的异常可能导致线程意外终止,从而影响整个程序的稳定性。
-
捕获线程异常
可以在线程的
run
方法中使用try-except
块来捕获异常,从而避免线程意外终止。import threading
class MyThread(threading.Thread):
def run(self):
try:
# 执行任务
pass
except Exception as e:
print(f"Exception occurred: {e}")
t = MyThread()
t.start()
t.join()
-
处理异常后的恢复
在捕获异常后,可以采取适当的恢复措施,例如重启线程或记录日志,以便在发生异常时能够迅速恢复。
import logging
logging.basicConfig(level=logging.INFO)
class MyThread(threading.Thread):
def run(self):
try:
# 执行任务
pass
except Exception as e:
logging.error(f"Exception occurred: {e}")
# 恢复措施
六、总结
Python中的线程销毁与管理是一个复杂但重要的任务。在多线程编程中,我们需要确保线程能够安全地退出,避免资源泄漏和数据不一致的问题。通过使用标志、线程锁、条件变量和异常处理等机制,我们可以有效地管理和销毁线程。此外,使用concurrent.futures
模块提供的线程池也是一种简化线程管理的有效工具。通过合理地设计和实现线程管理策略,可以提高程序的稳定性和性能。
相关问答FAQs:
如何安全地终止一个正在运行的线程?
在Python中,线程的终止并不简单,因为没有内置的方法可以直接“销毁”一个线程。为了安全地终止线程,建议使用标志位(如Event
对象)来指示线程应当结束。线程在运行时定期检查这个标志位,并在需要时自行退出。
线程终止后需要做哪些清理工作?
当一个线程完成其任务或被安全终止时,确保释放它所占用的资源是很重要的。可以使用join()
方法来等待线程完成,并在主程序中处理任何需要清理的资源,例如关闭文件或释放锁。这有助于防止资源泄露和潜在的死锁问题。
Python中是否存在强制终止线程的方法?
在Python中,强制终止线程并不是一个推荐的做法,因为这可能导致资源未释放或数据损坏。虽然可以使用一些底层的方法(如ctypes
库),但是这通常会带来不可预料的结果。更安全的方式是设计线程的工作逻辑,使其能够在适当的时候自行结束。
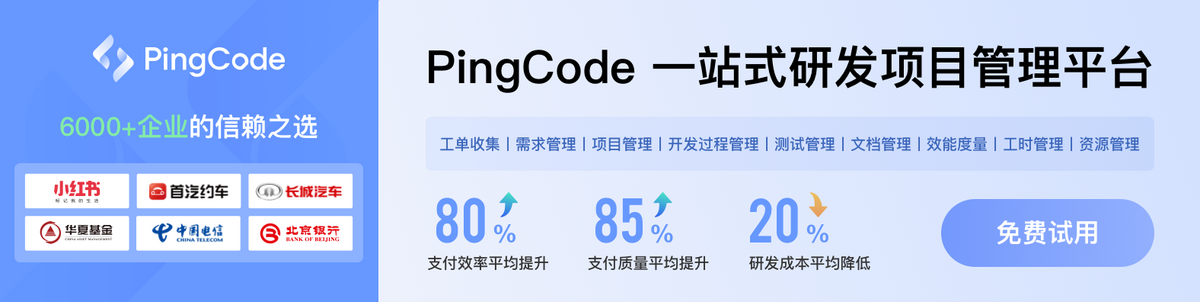