Python可以通过使用多种库和工具来翻译英文,包括Google Translate API、TextBlob、DeepL API等。通过调用这些API或使用这些库,开发者可以轻松实现语言翻译功能。以下是使用Google Translate API的详细介绍。Google Translate API是一个强大的工具,可以提供高质量的翻译服务。开发者可以使用Python编写脚本,通过API发送翻译请求并接收翻译结果。此外,TextBlob是一个简单易用的Python库,它基于Google的开源翻译API,适合处理基本的翻译任务。DeepL API则提供了高级的翻译质量,适合需要高精度翻译的应用。
一、GOOGLE TRANSLATE API
Google Translate API是一个广泛使用的翻译工具,能够支持多种语言的翻译。通过这个API,开发者可以轻松地将文本从一种语言翻译成另一种语言。要使用Google Translate API,需要先在Google Cloud Platform上启用翻译服务,并获取API密钥。
-
获取API密钥
要使用Google Translate API,首先需要在Google Cloud Platform上创建一个项目,并启用翻译服务。然后,您需要生成一个API密钥。这个密钥将用于在您的Python脚本中进行身份验证。
-
安装Google Translate API库
在Python中使用Google Translate API,需要安装相关的库。可以通过pip命令安装:
pip install google-cloud-translate
-
使用API进行翻译
安装完成后,可以编写Python脚本来进行翻译。以下是一个简单的例子:
from google.cloud import translate_v2 as translate
def translate_text(text, target='zh'):
translate_client = translate.Client()
result = translate_client.translate(text, target_language=target)
return result['translatedText']
text_to_translate = "Hello, how are you?"
translated_text = translate_text(text_to_translate)
print(f'Translated text: {translated_text}')
在这个例子中,我们定义了一个函数
translate_text
,它接受两个参数:要翻译的文本和目标语言。然后,使用Google Translate API进行翻译,并返回翻译后的文本。
二、TEXTBLOB
TextBlob是一个简单易用的Python库,适合用来处理基本的翻译任务。它基于Google的开源翻译API,提供了便捷的接口来实现翻译功能。
-
安装TextBlob
可以通过pip命令安装TextBlob:
pip install textblob
-
使用TextBlob进行翻译
使用TextBlob进行翻译非常简单。以下是一个示例:
from textblob import TextBlob
text = "Hello, how are you?"
blob = TextBlob(text)
translated_text = blob.translate(to='zh')
print(f'Translated text: {translated_text}')
在这个例子中,我们创建了一个TextBlob对象,并调用其
translate
方法来进行翻译。只需指定目标语言,即可获得翻译结果。
三、DEEPL API
DeepL API是一个提供高级翻译服务的工具,以其高翻译质量而闻名。适合需要高精度翻译的应用。
-
注册DeepL API
首先需要在DeepL网站上注册并获取API密钥。
-
安装DeepL库
使用pip命令安装DeepL库:
pip install deepl
-
使用DeepL API进行翻译
使用DeepL API进行翻译的示例代码如下:
import deepl
translator = deepl.Translator('your-api-key')
result = translator.translate_text("Hello, how are you?", target_lang="ZH")
print(f'Translated text: {result.text}')
在这个示例中,我们创建了一个Translator对象,并调用其
translate_text
方法进行翻译。只需提供待翻译文本和目标语言代码即可获得结果。
四、总结
Python为实现英文翻译提供了多种工具和库。Google Translate API、TextBlob和DeepL API都是流行且有效的选择。根据具体需求和预算,可以选择最适合的工具来实现高质量的翻译功能。在应用这些工具时,要注意API的使用限制和收费标准,以确保满足项目的需求。
相关问答FAQs:
Python可以使用哪些库来实现英文翻译?
Python提供了多个库来支持英文翻译,例如Google Translate API、DeepL API和TextBlob等。Google Translate API提供了广泛的翻译功能,并且支持多种语言。TextBlob则适合处理简单的翻译任务,易于使用且功能强大。选择合适的库可以帮助你更高效地完成翻译工作。
如何在Python中使用Google Translate进行翻译?
在Python中使用Google Translate,你可以通过安装googletrans
库来实现。首先,通过命令行安装该库:pip install googletrans==4.0.0-rc1
。接着,你可以通过简单的代码将文本翻译成英文,例如:
from googletrans import Translator
translator = Translator()
result = translator.translate('你好', dest='en')
print(result.text)
这段代码将中文“你好”翻译为英文。
使用Python翻译时需要注意哪些事项?
进行翻译时,最好考虑到翻译的上下文和语境。有些词汇在不同的上下文中可能会有不同的含义。此外,使用API时可能会有速率限制或者需要API密钥,因此在编写代码时要确保遵循相关的使用条款。同时,翻译结果可能不是完美的,建议在关键场合进行人工校对。
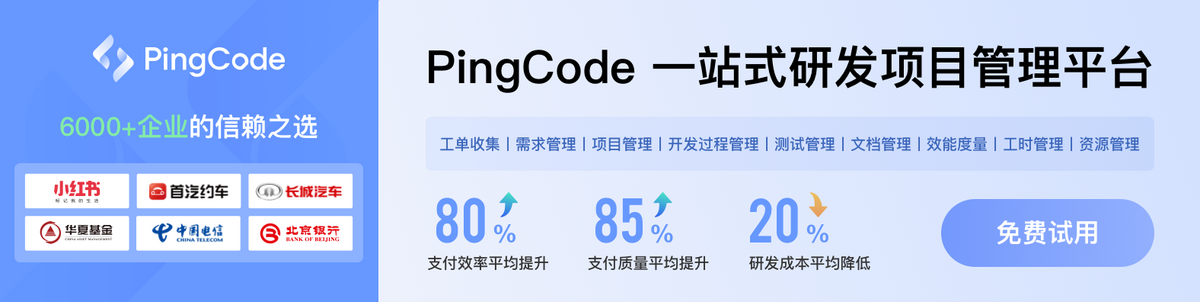