Python使用MNIST数据集的方法包括:使用Keras库加载MNIST数据集、通过TensorFlow加载MNIST数据集、从网上手动下载并加载MNIST数据集。 其中,使用Keras库加载MNIST数据集是最简单和直接的方式,因为Keras提供了内置的MNIST数据集加载功能,可以轻松地导入和使用。Keras将数据集划分为训练集和测试集,并将其格式化为适合机器学习模型输入的形式。下面我们将详细探讨如何使用Python加载和操作MNIST数据集。
一、Keras库加载MNIST数据集
Keras是一个高层神经网络API,运行在TensorFlow之上。它使得加载和使用MNIST数据集变得非常简单。以下是使用Keras加载MNIST数据集的步骤:
-
导入库和加载数据集
首先,需要导入Keras库中的
datasets
模块,然后使用mnist.load_data()
函数加载数据集。加载后,数据集被分为训练集和测试集:from keras.datasets import mnist
加载MNIST数据集
(x_train, y_train), (x_test, y_test) = mnist.load_data()
-
数据预处理
加载的数据需要进行预处理,包括归一化和形状调整。MNIST数据集中每个图像是28×28的灰度图,需要将其转换为浮点数,并将像素值缩放到0到1之间:
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
将标签转换为one-hot编码
from keras.utils import to_categorical
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
-
构建和训练模型
使用Keras可以快速构建深度学习模型。例如,使用简单的全连接网络(Dense layers)来训练模型:
from keras.models import Sequential
from keras.layers import Dense, Flatten
model = Sequential([
Flatten(input_shape=(28, 28)),
Dense(128, activation='relu'),
Dense(10, activation='softmax')
])
编译模型
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
训练模型
model.fit(x_train, y_train, epochs=5, batch_size=32, validation_data=(x_test, y_test))
-
评估模型性能
在测试集上评估模型性能,查看准确率:
test_loss, test_accuracy = model.evaluate(x_test, y_test)
print('Test accuracy:', test_accuracy)
二、通过TensorFlow加载MNIST数据集
TensorFlow也是一个非常流行的深度学习框架,直接支持MNIST数据集的加载。以下是通过TensorFlow加载MNIST的步骤:
-
导入TensorFlow和加载数据
TensorFlow提供了
tensorflow.keras.datasets
模块,可以直接加载MNIST数据集:import tensorflow as tf
使用TensorFlow加载MNIST数据集
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
此步骤与Keras加载方式类似,因为TensorFlow中的Keras模块与独立的Keras库非常相似。
-
数据预处理
同样需要对数据进行归一化和标签的one-hot编码:
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
y_train = tf.keras.utils.to_categorical(y_train, 10)
y_test = tf.keras.utils.to_categorical(y_test, 10)
-
构建和训练TensorFlow模型
使用TensorFlow构建一个简单的神经网络模型:
model = tf.keras.Sequential([
tf.keras.layers.Flatten(input_shape=(28, 28)),
tf.keras.layers.Dense(128, activation='relu'),
tf.keras.layers.Dense(10, activation='softmax')
])
model.compile(optimizer='adam',
loss='categorical_crossentropy',
metrics=['accuracy'])
model.fit(x_train, y_train, epochs=5, batch_size=32, validation_data=(x_test, y_test))
-
评估模型
使用测试数据集进行评估:
test_loss, test_accuracy = model.evaluate(x_test, y_test)
print('Test accuracy:', test_accuracy)
三、手动下载并加载MNIST数据集
如果不想使用内置函数加载数据集,您可以选择手动下载和加载MNIST数据集。这种方式适合对数据进行自定义预处理的需求。
-
下载数据集
可以从MNIST数据库官网下载四个文件:训练图像、训练标签、测试图像、测试标签。
-
读取和解析数据
使用Python读取二进制文件并解析为NumPy数组:
import numpy as np
def load_mnist_images(filename):
with open(filename, 'rb') as f:
f.read(16) # 跳过头部信息
data = np.frombuffer(f.read(), dtype=np.uint8)
return data.reshape(-1, 28, 28)
def load_mnist_labels(filename):
with open(filename, 'rb') as f:
f.read(8) # 跳过头部信息
labels = np.frombuffer(f.read(), dtype=np.uint8)
return labels
x_train = load_mnist_images('train-images-idx3-ubyte')
y_train = load_mnist_labels('train-labels-idx1-ubyte')
x_test = load_mnist_images('t10k-images-idx3-ubyte')
y_test = load_mnist_labels('t10k-labels-idx1-ubyte')
-
数据预处理
对手动加载的数据进行预处理:
x_train = x_train.astype('float32') / 255
x_test = x_test.astype('float32') / 255
from tensorflow.keras.utils import to_categorical
y_train = to_categorical(y_train, 10)
y_test = to_categorical(y_test, 10)
-
构建和训练模型
与前述Keras和TensorFlow方法相同,您可以选择任意框架来构建和训练模型。
四、MNIST数据集应用与扩展
MNIST数据集是一个入门级的数据集,虽然简单,但它在机器学习和深度学习研究中具有极大的影响力。除了基本的分类问题,您还可以使用MNIST进行其他扩展研究:
-
图像生成
使用生成对抗网络(GANs)来生成新的手写数字图像。
-
图像降噪
训练去噪自编码器来去除手写数字图像中的噪声。
-
迁移学习
使用预训练的卷积神经网络(CNN)进行迁移学习,以提高分类性能。
-
不同模型的比较
测试不同的机器学习模型(如SVM、随机森林)在MNIST数据集上的性能。
通过以上步骤,您可以在Python中成功加载和使用MNIST数据集,并进行各种机器学习和深度学习实验。无论是初学者还是经验丰富的研究人员,MNIST都是一个理想的实验数据集。
相关问答FAQs:
如何在Python中加载MNIST数据集?
在Python中加载MNIST数据集通常使用keras
库或tensorflow
库。可以通过以下代码轻松加载数据集:
from keras.datasets import mnist
(X_train, y_train), (X_test, y_test) = mnist.load_data()
这样就可以得到训练和测试数据,X_train
和X_test
包含图像数据,而y_train
和y_test
则包含对应的标签。
使用MNIST数据集进行模型训练的最佳实践是什么?
在使用MNIST数据集进行模型训练时,建议进行数据预处理,包括归一化图像数据到0到1之间,以及将标签进行独热编码。此外,采用适当的模型架构,如卷积神经网络(CNN),能够显著提高分类准确率。同时,使用交叉验证可以帮助评估模型的性能。
如何评估使用MNIST数据集训练的模型的效果?
评估模型效果的常用方法是计算分类准确率。可以使用sklearn
库中的accuracy_score
函数来评估。除了准确率,混淆矩阵、精确率、召回率等指标也是非常有用的评估标准。通过可视化混淆矩阵,可以更直观地了解模型在各类上的表现。
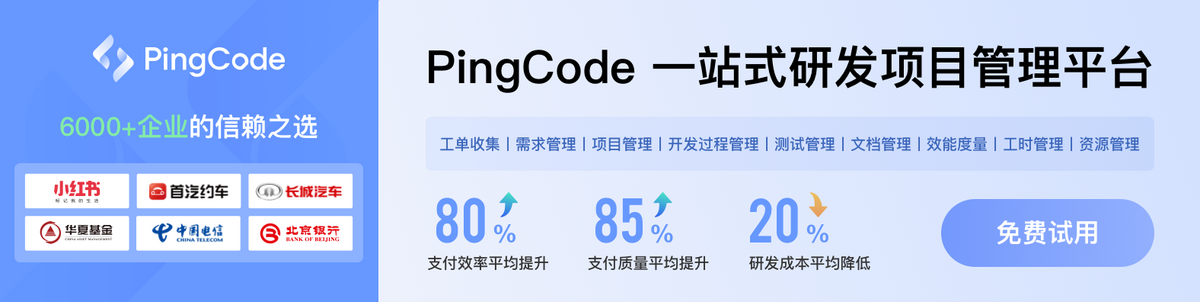