在Python中查看匹配的方法包括:使用正则表达式(regular expressions)进行字符串匹配、利用内置的字符串方法如find()和in运算符、使用外部库如re
模块来进行更复杂的模式匹配。正则表达式是处理字符串匹配的强大工具,能够匹配复杂的模式、提取特定的子串、进行替换操作等。
在Python中,正则表达式的使用是通过re
模块来实现的。re
模块提供了一系列功能强大的方法来处理字符串匹配,比如re.match()
、re.search()
、re.findall()
等。下面我将详细介绍这些方法及其应用。
一、正则表达式基础
1、什么是正则表达式
正则表达式(Regular Expression,简称regex)是一种用来描述或匹配一系列符合某个句法规则的字符串的模式。它在文本处理中被广泛应用,尤其是在查找、替换和提取字符串的场景中。
2、Python中使用正则表达式的基础
Python提供了内置的re
模块来支持正则表达式。要使用正则表达式,首先需要导入这个模块:
import re
二、常用正则表达式方法
1、re.match()
re.match()
方法用于从字符串的起始位置进行匹配。如果在起始位置匹配到模式,则返回一个匹配对象;否则返回None。
import re
pattern = r'\d+' # 匹配一个或多个数字
string = "123abc456"
match = re.match(pattern, string)
if match:
print("Match found:", match.group())
else:
print("No match")
2、re.search()
re.search()
方法用于在整个字符串中搜索模式。与re.match()
不同,它不要求从起始位置开始匹配。
import re
pattern = r'\d+'
string = "abc123"
search = re.search(pattern, string)
if search:
print("Search found:", search.group())
else:
print("No match")
3、re.findall()
re.findall()
方法用于查找字符串中所有匹配的子串,并以列表的形式返回。
import re
pattern = r'\d+'
string = "abc123def456"
matches = re.findall(pattern, string)
print("Findall matches:", matches)
4、re.finditer()
re.finditer()
方法与re.findall()
类似,但返回的是一个迭代器,能够逐个访问匹配对象。
import re
pattern = r'\d+'
string = "abc123def456"
matches = re.finditer(pattern, string)
for match in matches:
print("Finditer match:", match.group())
三、正则表达式进阶
1、捕获组
捕获组是通过圆括号()
来定义的,用于提取子模式匹配的内容。
import re
pattern = r'(\d+)-(\d+)'
string = "Phone: 123-456"
match = re.search(pattern, string)
if match:
print("Area code:", match.group(1))
print("Local number:", match.group(2))
2、非捕获组
如果不需要捕获组中的内容,可以使用(?:...)
来定义非捕获组。
import re
pattern = r'(?:\d+)-(\d+)'
string = "Phone: 123-456"
match = re.search(pattern, string)
if match:
print("Local number:", match.group(1))
3、断言
断言用于指定匹配的位置条件,包括前瞻断言(lookahead)和后瞻断言(lookbehind)。
import re
前瞻断言
pattern = r'\d+(?= dollars)' # 匹配后面跟着"dollars"的数字
string = "Price: 100 dollars"
match = re.search(pattern, string)
if match:
print("Lookahead match:", match.group())
四、字符串方法匹配
1、find()
find()
方法用于查找子串在字符串中的位置,返回第一个匹配的索引,如果未找到则返回-1。
string = "Hello, world!"
substring = "world"
index = string.find(substring)
if index != -1:
print("Substring found at index:", index)
else:
print("Substring not found")
2、in运算符
in
运算符用于检查子串是否存在于字符串中,返回布尔值。
string = "Hello, world!"
substring = "world"
if substring in string:
print("Substring is in string")
else:
print("Substring is not in string")
五、总结
Python提供了多种方法来查看字符串匹配,其中正则表达式是最强大和灵活的工具。通过re
模块中的方法,可以进行从简单到复杂的各种匹配操作。同时,Python的字符串内置方法如find()
和in
运算符提供了简单直接的匹配方式,适合于不需要复杂模式的场景。在实际应用中,根据具体需求选择合适的匹配方法,能够提升代码的效率和可读性。
相关问答FAQs:
如何在Python中检查字符串是否匹配特定模式?
在Python中,可以使用内置的re
模块来检查字符串是否匹配特定的正则表达式模式。首先,导入re
模块,并使用re.match()
或re.search()
函数来进行匹配。re.match()
会从字符串的起始位置检查匹配,而re.search()
则会搜索整个字符串。以下是一个基本示例:
import re
pattern = r'\d+' # 匹配一个或多个数字
string = "There are 123 apples"
if re.search(pattern, string):
print("匹配成功!")
else:
print("没有匹配。")
Python中如何使用正则表达式来提取匹配的内容?
使用re
模块的re.findall()
函数可以提取所有匹配的内容。这个函数返回一个列表,包含所有符合模式的子字符串。例如:
import re
pattern = r'\d+'
string = "There are 123 apples and 456 oranges"
matches = re.findall(pattern, string)
print("找到的数字:", matches) # 输出: 找到的数字: ['123', '456']
在Python中如何优化正则表达式的匹配性能?
优化正则表达式的匹配性能可以通过几个方面来实现。使用原始字符串(在字符串前加r
)可以避免转义字符的问题,从而提高可读性和性能。此外,避免过于复杂的表达式,使用非捕获组((?:...)
)以及限制匹配次数(使用量词)都可以提高匹配效率。例如:
import re
pattern = r'(?:\d{3})-\d{2}-\d{4}' # 匹配类似于 123-45-6789 的格式
string = "My number is 123-45-6789"
if re.search(pattern, string):
print("匹配成功!")
通过这些方法,可以有效提升正则表达式在Python中的匹配性能。
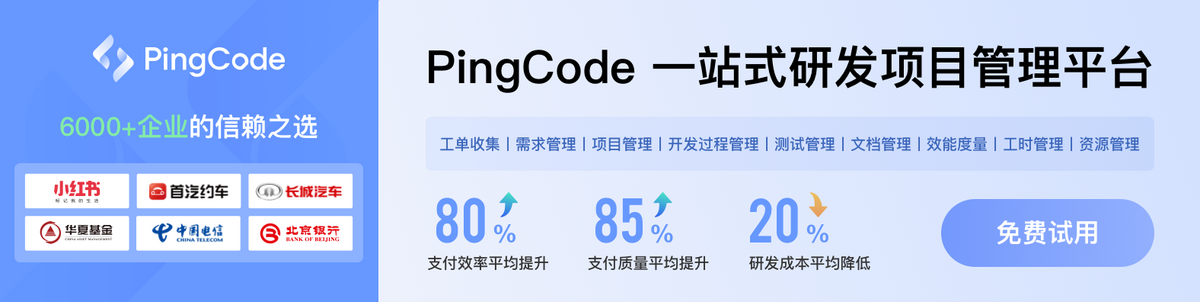