Python发请求通常通过使用requests库、http.client模块、urllib库进行。其中,requests库最为简单易用,适合大多数场景。requests库提供了get、post、put、delete等方法,帮助处理HTTP请求,简化了网络操作。下面将详细介绍requests库的使用,并简要介绍其他方式。
一、REQUESTS库
requests库是Python中最流行的HTTP请求库之一。它简单易用,功能强大,是处理HTTP请求的首选。
- 安装requests库
在使用requests库之前,需要先安装它。可以通过pip来安装:
pip install requests
安装完成后,就可以在Python脚本中使用requests库了。
- 发起GET请求
GET请求用于从服务器获取数据。使用requests库发起GET请求非常简单:
import requests
response = requests.get('https://api.example.com/data')
print(response.status_code)
print(response.text)
在上述代码中,requests.get
方法用于发起GET请求,response
对象包含了服务器的响应。可以通过response.status_code
获取状态码,通过response.text
获取响应内容。
- 发起POST请求
POST请求用于向服务器发送数据。可以通过requests.post
方法来发起POST请求:
import requests
payload = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/data', data=payload)
print(response.status_code)
print(response.text)
在上述代码中,payload
是要发送的数据,使用data
参数传递给requests.post
方法。
- 处理JSON数据
requests库可以方便地处理JSON数据。可以通过json
参数发送JSON数据,并通过response.json()
解析响应中的JSON数据:
import requests
payload = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/data', json=payload)
print(response.status_code)
print(response.json())
- 添加请求头
有时候需要在请求中添加自定义的请求头,可以通过headers
参数实现:
import requests
headers = {'Authorization': 'Bearer YOUR_ACCESS_TOKEN'}
response = requests.get('https://api.example.com/data', headers=headers)
print(response.status_code)
print(response.text)
二、HTTP.CLIENT模块
http.client模块是Python标准库的一部分,可以用于处理HTTP请求。虽然不如requests库简单易用,但在不想安装第三方库的情况下,它是一个不错的选择。
- 发起GET请求
import http.client
conn = http.client.HTTPConnection('api.example.com')
conn.request('GET', '/data')
response = conn.getresponse()
print(response.status, response.reason)
data = response.read()
print(data)
conn.close()
- 发起POST请求
import http.client
import json
conn = http.client.HTTPConnection('api.example.com')
headers = {'Content-type': 'application/json'}
payload = json.dumps({'key1': 'value1', 'key2': 'value2'})
conn.request('POST', '/data', payload, headers)
response = conn.getresponse()
print(response.status, response.reason)
data = response.read()
print(data)
conn.close()
三、URLLIB库
urllib库也是Python标准库的一部分,它提供了一些处理URL的模块。
- 发起GET请求
import urllib.request
response = urllib.request.urlopen('https://api.example.com/data')
print(response.status)
print(response.read().decode('utf-8'))
- 发起POST请求
import urllib.request
import json
url = 'https://api.example.com/data'
headers = {'Content-type': 'application/json'}
data = json.dumps({'key1': 'value1', 'key2': 'value2'}).encode('utf-8')
request = urllib.request.Request(url, data=data, headers=headers)
response = urllib.request.urlopen(request)
print(response.status)
print(response.read().decode('utf-8'))
四、异步请求
在某些情况下,可能需要发起异步请求,以避免阻塞程序的执行。这时可以使用aiohttp库。
- 安装aiohttp库
pip install aiohttp
- 使用aiohttp发起异步请求
import aiohttp
import asyncio
async def fetch(url):
async with aiohttp.ClientSession() as session:
async with session.get(url) as response:
return await response.text()
async def main():
url = 'https://api.example.com/data'
html = await fetch(url)
print(html)
loop = asyncio.get_event_loop()
loop.run_until_complete(main())
五、错误处理与重试机制
在网络请求中,错误处理和重试机制是必不可少的,尤其是在请求失败的情况下。requests库可以通过异常处理来实现这一点。
import requests
from requests.exceptions import HTTPError, Timeout, RequestException
url = 'https://api.example.com/data'
try:
response = requests.get(url, timeout=5)
response.raise_for_status()
except HTTPError as http_err:
print(f'HTTP error occurred: {http_err}')
except Timeout as timeout_err:
print(f'Timeout error occurred: {timeout_err}')
except RequestException as err:
print(f'Other error occurred: {err}')
else:
print('Success!')
以上代码演示了如何处理HTTP错误、超时错误和其他请求错误。同时,还可以使用第三方库如tenacity
来实现重试机制。
六、总结
Python提供了多种方式来发起HTTP请求,其中requests库因其简单易用而广受欢迎。对于需要异步请求的场景,可以使用aiohttp库。无论选择哪种方式,处理请求时都需要注意错误处理和重试机制,以提高程序的健壮性。通过合理地选择和使用这些工具,可以大大简化HTTP请求的处理过程。
相关问答FAQs:
如何在Python中发送GET请求?
在Python中,可以使用内置的requests
库发送GET请求。首先,确保已安装requests库,使用命令pip install requests
进行安装。发送GET请求的方法如下:
import requests
response = requests.get('https://api.example.com/data')
print(response.status_code)
print(response.json())
此代码将发送一个GET请求到指定的URL,并打印出响应的状态码和返回的JSON数据。
Python中如何处理POST请求?
使用requests
库发送POST请求同样简单。可以通过以下代码实现:
import requests
data = {'key1': 'value1', 'key2': 'value2'}
response = requests.post('https://api.example.com/submit', data=data)
print(response.status_code)
print(response.text)
在这个示例中,数据以字典形式传递,requests库会自动将其编码为表单格式。
如何在Python请求中添加自定义头信息?
在发送请求时,可以通过headers
参数添加自定义头信息。例如:
import requests
headers = {'Authorization': 'Bearer your_token', 'Content-Type': 'application/json'}
response = requests.get('https://api.example.com/protected', headers=headers)
print(response.status_code)
print(response.json())
通过这种方式,可以在请求中包含身份验证信息和其他必要的头部,以确保正确访问受保护的资源。
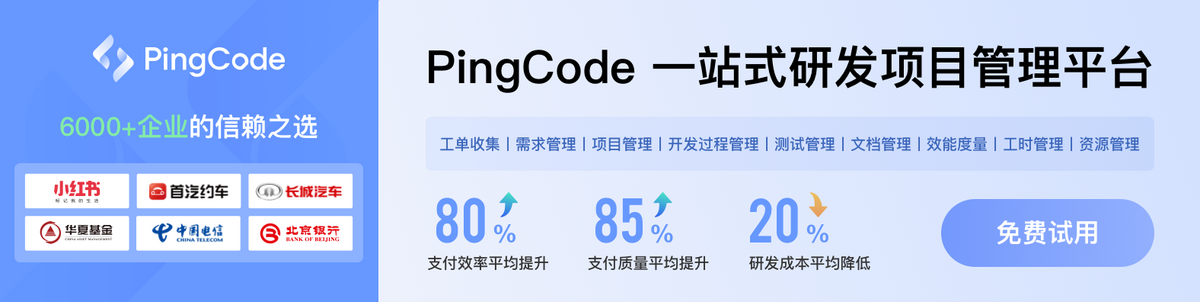