在Python中验证空值的方法包括使用None
、检查数据结构的长度、使用not
运算符、以及使用isinstance()
函数。在这些方法中,None
是Python中表示空值的特殊对象,它通常用于表示函数没有返回值或指定一个变量没有具体的值。接下来,我们将详细描述如何使用None
来验证空值。
在Python中,None
是一个单例对象,通常用于表示“没有值”或“空值”。例如,当一个函数没有显式返回值时,它默认返回None
。要验证一个变量是否为None
,可以使用is
运算符,因为None
是一个单例对象,使用is
可以精确比较对象身份,而不是值的相等性。示例如下:
x = None
if x is None:
print("x is None")
这种方式是最常见和直观的验证空值的方法,尤其是在函数返回值的检查中。
一、使用 None
检查
在Python中,None
是一个特殊的常量,通常用来表示缺失或空值。在检查变量是否为None
时,通常使用is
操作符,这是因为None
是一个单例。
1、检查变量是否为 None
当我们需要检查一个变量是否没有被赋值或明确被赋值为无时,None
是一个非常合适的工具。例如,我们可以使用以下代码来检查一个变量是否为None
:
value = None
if value is None:
print("The variable is None.")
else:
print("The variable is not None.")
这种检查方式在处理函数返回值时尤其有用,因为许多函数会返回None
来表示“无结果”或“空结果”。
2、函数返回 None
在函数中,None
可以用来表示一个特定条件下的返回值。例如,当一个查找函数没有找到目标时,可以返回None
:
def find_element(elements, target):
for element in elements:
if element == target:
return element
return None
result = find_element([1, 2, 3], 4)
if result is None:
print("Element not found.")
这种方法可以清楚地表示出函数操作的结果状态,并在必要时采取相应的操作。
二、检查数据结构的长度
对于序列(如字符串、列表、元组)和字典,检查其长度是验证是否为空的常用方法。
1、使用 len()
函数
Python的len()
函数可以用来检查序列或字典的长度。如果长度为0,则表明该数据结构为空。例如:
my_list = []
if len(my_list) == 0:
print("The list is empty.")
else:
print("The list is not empty.")
这是一个非常直观的方法,尤其在处理列表或字符串时,能够快速有效地验证其状态。
2、直接使用条件判断
Python的条件判断中,空的序列或字典会被视为False
,这使得我们可以直接在条件语句中使用它们:
my_dict = {}
if not my_dict:
print("The dictionary is empty.")
else:
print("The dictionary is not empty.")
这种方式利用了Python的内置特性,使代码更加简洁。
三、使用 not
运算符
not
运算符可以用于验证对象的逻辑否定,在验证空值时十分有效。
1、检查布尔值
在Python中,None
、空序列和空字典都被视为False
。因此,可以使用not
来检查这些对象:
empty_string = ""
if not empty_string:
print("The string is empty.")
else:
print("The string is not empty.")
这种方法简洁且语义明确,尤其适合在需要快速验证多个条件时使用。
2、结合其他条件
not
运算符还可以与其他条件结合使用,形成更复杂的判断逻辑:
value = None
if value is None or not value:
print("The value is None or empty.")
else:
print("The value is not empty.")
这种方式在需要同时验证多个条件时非常有用,保持了代码的简洁性。
四、使用 isinstance()
函数
isinstance()
函数用于检查一个对象是否是特定类型的实例。在验证空值时,可以结合None
和特定数据类型使用。
1、验证变量类型和空值
当需要同时验证变量的类型和是否为空时,isinstance()
和None
结合使用非常有效:
def check_variable(var):
if isinstance(var, str) and not var:
print("The variable is an empty string.")
elif isinstance(var, list) and not var:
print("The variable is an empty list.")
elif var is None:
print("The variable is None.")
else:
print("The variable is not empty.")
check_variable("")
check_variable([])
check_variable(None)
这种方法能够同时处理多种情况,确保变量的类型和状态都符合预期。
2、复杂数据结构的验证
在处理复杂数据结构时,isinstance()
提供了一个明确的方式来验证其组成部分:
data = {"key": []}
if isinstance(data, dict) and all(not v for v in data.values()):
print("All values in the dictionary are empty.")
else:
print("The dictionary has non-empty values.")
这种方法能够精确地检查嵌套结构的状态,确保在操作数据时不会出现意外错误。
五、使用 try-except
处理异常
在某些情况下,验证空值可能涉及到捕获异常。使用try-except
块可以有效地管理潜在的错误。
1、处理空值引发的异常
如果某种操作可能引发异常,比如访问不存在的字典键,可以使用try-except
来处理:
my_dict = {"key": "value"}
try:
value = my_dict["nonexistent_key"]
except KeyError:
print("Key does not exist, indicating an empty or missing value.")
这种方式能够优雅地处理潜在的错误,不影响程序的正常执行。
2、结合其他验证方法
try-except
块可以与其他验证方法结合使用,以提供更全面的错误处理机制:
def get_value(dictionary, key):
try:
value = dictionary[key]
if not value:
raise ValueError("Empty value found.")
return value
except KeyError:
print("Key not found.")
except ValueError as ve:
print(ve)
data = {"key": ""}
get_value(data, "key")
这种综合方法不仅验证了值的存在性,还确保其在业务逻辑中的有效性。
相关问答FAQs:
如何在Python中检查一个变量是否为空?
在Python中,可以通过多种方式检查变量是否为空。最常用的方法是直接使用if not variable:
来判断。如果变量是None
、空字符串、空列表、空字典或空元组,都会返回True
。例如:
my_var = ""
if not my_var:
print("变量为空")
在Python中,如何验证一个列表是否为空?
要检查一个列表是否为空,可以使用if not my_list:
语句。如果列表没有任何元素,将会返回True
。例如:
my_list = []
if not my_list:
print("列表为空")
如何使用Python函数验证输入是否为空?
可以定义一个函数来验证输入是否为空,这样可以方便地重复使用。例如:
def is_empty(value):
return value == "" or value is None
print(is_empty("")) # 输出: True
print(is_empty(None)) # 输出: True
这种方法允许你在不同情况下灵活使用,提升代码的可读性和可维护性。
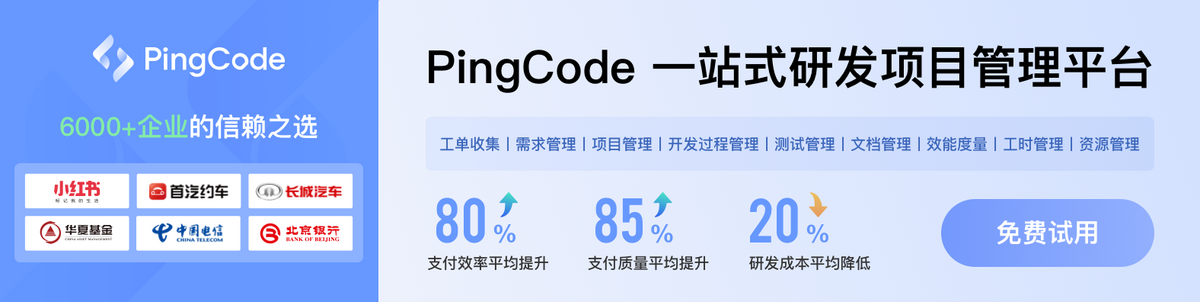