在Python中查看匹配的方法包括:使用正则表达式模块re
、检查字符串方法如find
和index
、以及使用in
关键字来检查子字符串的存在。 其中,正则表达式模块re
是最强大和灵活的工具,它可以精确地定义搜索模式以匹配复杂的字符串模式。通过使用re
模块的search
、match
、findall
等函数,我们可以查找字符串中是否存在某种模式,并返回匹配的结果。下面将详细介绍如何使用这些方法在Python中查看匹配。
一、正则表达式模块 re
正则表达式(Regular Expression)是一种强大的字符串处理工具,re
模块提供了丰富的方法来支持复杂的字符串模式匹配。
1. 使用 re.search
方法
re.search
用于在整个字符串中搜索第一次出现的匹配项。如果找到匹配项,则返回一个匹配对象,否则返回None
。
import re
pattern = r'\d+' # 匹配一个或多个数字
text = "The price is 100 dollars"
match = re.search(pattern, text)
if match:
print(f"Found match: {match.group()}")
else:
print("No match found")
2. 使用 re.match
方法
re.match
用于从字符串的开头开始匹配,如果开头没有匹配项,则返回None
。
import re
pattern = r'The'
text = "The price is 100 dollars"
match = re.match(pattern, text)
if match:
print(f"Match at the start: {match.group()}")
else:
print("No match at the start")
3. 使用 re.findall
方法
re.findall
返回字符串中所有非重叠的匹配项,作为一个列表。
import re
pattern = r'\d+'
text = "Items: 1, 2, 3, 4, 5"
matches = re.findall(pattern, text)
print(f"All matches: {matches}")
4. 使用 re.finditer
方法
re.finditer
返回一个迭代器,生成匹配对象,用于遍历所有匹配项。
import re
pattern = r'\d+'
text = "Items: 1, 2, 3, 4, 5"
matches = re.finditer(pattern, text)
for match in matches:
print(f"Match found: {match.group()}")
二、使用字符串方法
Python的字符串类提供了一些方法来检查子字符串的存在和位置。
1. 使用 str.find
方法
str.find
用于找到子字符串首次出现的位置,如果未找到,则返回-1
。
text = "Hello, world!"
position = text.find("world")
if position != -1:
print(f"Found at position: {position}")
else:
print("Not found")
2. 使用 str.index
方法
str.index
与find
类似,但如果未找到匹配项,则会引发ValueError
。
text = "Hello, world!"
try:
position = text.index("world")
print(f"Found at position: {position}")
except ValueError:
print("Not found")
三、使用 in
关键字
in
关键字用于检查一个字符串是否包含另一个字符串,返回布尔值。
text = "Hello, world!"
if "world" in text:
print("Substring found")
else:
print("Substring not found")
四、正则表达式高级使用技巧
正则表达式在Python中可以用来做更复杂的模式匹配,通过使用不同的模式和标志,可以实现更高级的搜索和替换功能。
1. 使用分组
正则表达式的分组功能可以将匹配的部分提取出来。
import re
pattern = r'(\d{4})-(\d{2})-(\d{2})'
text = "Date: 2023-10-20"
match = re.search(pattern, text)
if match:
print(f"Year: {match.group(1)}, Month: {match.group(2)}, Day: {match.group(3)}")
2. 使用替换功能
re.sub
可以用来替换匹配的字符串。
import re
pattern = r'\d+'
text = "The price is 100 dollars"
new_text = re.sub(pattern, '200', text)
print(new_text) # Output: The price is 200 dollars
3. 使用标志
正则表达式支持多种标志,比如re.IGNORECASE
用于忽略大小写。
import re
pattern = r'hello'
text = "Hello, world!"
match = re.search(pattern, text, re.IGNORECASE)
if match:
print("Match found with ignore case")
五、正则表达式的性能优化
正则表达式的匹配可能会影响性能,尤其在处理大文本时。以下是一些优化建议:
1. 合理使用非捕获组
使用(?:...)
来定义非捕获组,可以提高匹配速度。
import re
pattern = r'(?:\d{4})-(?:\d{2})-(?:\d{2})'
text = "2023-10-20"
match = re.search(pattern, text)
if match:
print("Non-capturing group match")
2. 使用原始字符串
在定义正则表达式时,使用原始字符串(以r
开头)可以避免对反斜杠进行额外的转义。
pattern = r'\d+'
六、实战应用案例
为了更好地理解Python中查看匹配的实用性,下面通过一个实战案例展示正则表达式的实际应用。
案例:提取电子邮件地址
假设我们有一段文本,其中包含多个电子邮件地址,我们需要提取出所有的电子邮件地址。
import re
text = """
Please contact us at support@example.com or sales@example.co.uk for further information.
You can also reach out to admin@company.org.
"""
email_pattern = r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}'
emails = re.findall(email_pattern, text)
print("Extracted emails:", emails)
在这个案例中,我们使用了一个正则表达式来匹配电子邮件地址的通用格式,并使用re.findall
提取出所有匹配的结果。
通过以上介绍,相信你已经对如何在Python中查看匹配有了深入的了解。无论是使用正则表达式还是字符串方法,合理选择工具可以让字符串处理变得简单而高效。
相关问答FAQs:
如何在Python中使用正则表达式进行匹配?
在Python中,可以使用re
模块来进行正则表达式匹配。首先,需要导入该模块。使用re.match()
可以从字符串的起始位置尝试匹配一个模式,而re.search()
则会搜索整个字符串中是否有匹配的模式。具体用法如下:
import re
pattern = r'\d+' # 匹配一个或多个数字
string = '我的号码是123456'
match = re.search(pattern, string)
if match:
print("匹配到的数字是:", match.group())
如何查看匹配结果中的分组信息?
在使用正则表达式进行匹配时,可以通过圆括号()
来定义分组。使用match.groups()
方法可以查看所有分组的匹配结果。示例如下:
pattern = r'(\d+)-(\d+)' # 匹配形如xx-xx的字符串
string = '我的号码是123-456'
match = re.search(pattern, string)
if match:
print("完整匹配:", match.group(0))
print("第一组匹配:", match.group(1))
print("第二组匹配:", match.group(2))
在Python中如何处理多行字符串的匹配?
对于多行字符串,可以在正则表达式中使用re.MULTILINE
标志。这个标志使得^
和$
可以匹配每一行的开头和结尾,而不仅仅是整个字符串的开头和结尾。以下是一个示例:
text = """第一行
第二行
第三行"""
pattern = r'^第二.*$' # 匹配以“第二”开头的行
matches = re.findall(pattern, text, re.MULTILINE)
print("匹配到的行:", matches)
这些方法可以帮助用户更好地理解如何在Python中进行匹配操作。
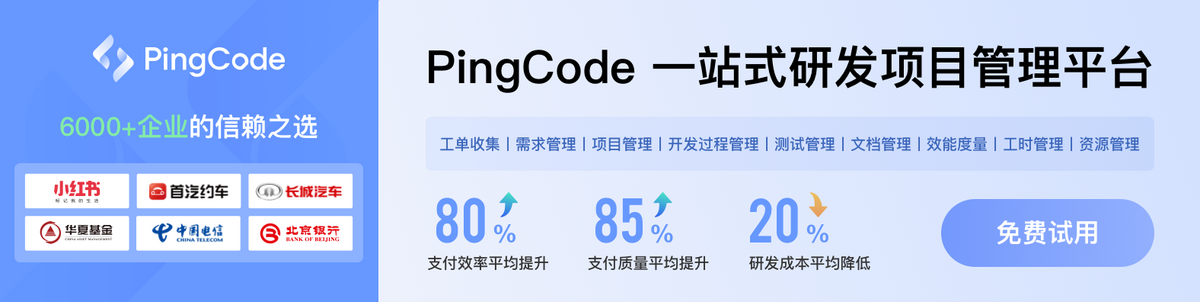