在Python中获取链接可以通过多种方式实现,如使用requests
库进行HTTP请求、BeautifulSoup
库解析HTML、re
库进行正则表达式匹配等。其中,使用requests
和BeautifulSoup
库是最常见的方法,因为它们提供了强大的功能来处理网络请求和解析HTML文档。
使用requests
库获取网页内容,然后使用BeautifulSoup
库解析HTML文档是获取链接的常用方法。requests
库提供了简单的API来发送HTTP请求并接收响应,而BeautifulSoup
库则专注于解析和遍历HTML文档。通过结合这两个库,可以轻松地从网页中提取所有的链接。BeautifulSoup
允许我们根据标签、属性等条件来查找元素,从而提取出其中的链接。
以下是详细的介绍:
一、使用requests
库获取网页内容
requests
库是一个用于发送HTTP请求的Python库,提供了非常简单的API来获取网页内容。它支持GET、POST等多种HTTP请求方法,并可以处理响应内容。
1、安装和基本用法
首先,确保你已经安装了requests
库。可以通过以下命令安装:
pip install requests
使用requests.get()
方法可以获取指定URL的内容:
import requests
url = 'http://example.com'
response = requests.get(url)
检查请求是否成功
if response.status_code == 200:
print("请求成功")
html_content = response.text
else:
print(f"请求失败,状态码:{response.status_code}")
2、处理响应内容
requests
库返回的响应对象包含了多种属性和方法,可以用于处理响应内容:
response.text
:以字符串形式返回响应内容。response.content
:以字节形式返回响应内容。response.status_code
:返回HTTP状态码。response.headers
:返回响应头信息。
通过这些属性,可以进一步处理和分析网页内容。
二、使用BeautifulSoup
解析HTML
BeautifulSoup
是一个用于解析HTML和XML文档的Python库,可以用来提取文档中的数据。
1、安装和基本用法
首先,确保你已经安装了BeautifulSoup
库及其依赖的解析器库lxml
或html5lib
。可以通过以下命令安装:
pip install beautifulsoup4 lxml
使用BeautifulSoup
解析HTML文档:
from bs4 import BeautifulSoup
html_content = '<html><body><a href="http://example.com">Example</a></body></html>'
soup = BeautifulSoup(html_content, 'lxml')
找到所有的<a>标签
links = soup.find_all('a')
for link in links:
print(link.get('href')) # 输出链接的URL
2、查找和提取链接
BeautifulSoup
提供了多种方法来查找和遍历HTML文档中的元素:
soup.find_all(name, attrs, recursive, text, kwargs)
:查找所有符合条件的标签。soup.find(name, attrs, recursive, text, kwargs)
:查找第一个符合条件的标签。soup.select(css_selector)
:通过CSS选择器查找标签。
例如,要提取所有的链接,可以使用:
# 提取所有的<a>标签中的链接
links = soup.find_all('a')
for link in links:
href = link.get('href')
print(href)
三、使用正则表达式提取链接
正则表达式提供了强大的字符串搜索和匹配功能,可以用来提取文本中的链接。
1、正则表达式基本用法
Python的re
模块提供了正则表达式功能。以下是一个简单的例子:
import re
text = 'Visit us at http://example.com or https://example.org'
pattern = r'https?://[^\s]+'
查找所有符合模式的字符串
matches = re.findall(pattern, text)
for match in matches:
print(match)
2、匹配HTML中的链接
要从HTML文档中提取链接,可以使用如下的正则表达式:
html_content = '<html><body><a href="http://example.com">Example</a></body></html>'
pattern = r'href="(http[s]?://[^"]+)"'
matches = re.findall(pattern, html_content)
for match in matches:
print(match)
四、结合使用requests
和BeautifulSoup
提取网页中的链接
通过结合requests
和BeautifulSoup
库,可以实现从网页中提取所有链接的完整流程。
import requests
from bs4 import BeautifulSoup
def get_links(url):
# 发送HTTP请求
response = requests.get(url)
# 检查请求是否成功
if response.status_code != 200:
print(f"请求失败,状态码:{response.status_code}")
return []
# 解析HTML文档
soup = BeautifulSoup(response.text, 'lxml')
# 提取所有的<a>标签中的链接
links = []
for a_tag in soup.find_all('a'):
href = a_tag.get('href')
if href:
links.append(href)
return links
使用示例
url = 'http://example.com'
links = get_links(url)
for link in links:
print(link)
五、处理相对链接和绝对链接
在实际应用中,网页中的链接可能是相对链接,需要将其转换为绝对链接。
1、相对链接和绝对链接
相对链接是相对于当前页面的链接,而绝对链接是完整的URL。为了确保链接的正确性,通常需要将相对链接转换为绝对链接。
2、使用urljoin
处理链接
Python的urllib.parse
模块提供了urljoin
函数,可以用于将相对链接转换为绝对链接:
from urllib.parse import urljoin
base_url = 'http://example.com/path/'
relative_link = 'subpage.html'
absolute_link = urljoin(base_url, relative_link)
print(absolute_link) # 输出:http://example.com/path/subpage.html
在提取链接时,可以结合使用urljoin
函数:
def get_absolute_links(base_url):
response = requests.get(base_url)
soup = BeautifulSoup(response.text, 'lxml')
links = []
for a_tag in soup.find_all('a'):
href = a_tag.get('href')
if href:
absolute_href = urljoin(base_url, href)
links.append(absolute_href)
return links
使用示例
absolute_links = get_absolute_links(url)
for link in absolute_links:
print(link)
六、处理特殊情况
在处理网页链接时,可能会遇到一些特殊情况,例如重定向、错误页面等。需要根据具体情况进行处理。
1、处理重定向
requests
库默认会自动处理HTTP重定向,可以通过response.history
属性查看重定向过程:
response = requests.get(url)
检查是否发生重定向
if response.history:
print("发生重定向")
for resp in response.history:
print(f"重定向:{resp.url} -> {response.url}")
2、处理错误页面
可以通过检查HTTP状态码来处理错误页面:
if response.status_code == 404:
print("页面未找到")
elif response.status_code == 500:
print("服务器错误")
通过上述方法,可以有效地获取网页中的链接,并处理各种可能出现的情况。这些技术在网络爬虫、数据采集等领域有着广泛的应用。
相关问答FAQs:
如何在Python中提取网页链接?
在Python中提取网页链接可以使用第三方库如Beautiful Soup和Requests。首先,使用Requests库获取网页的HTML内容,然后利用Beautiful Soup解析HTML,提取出所有的链接。示例代码如下:
import requests
from bs4 import BeautifulSoup
url = 'http://example.com' # 替换为目标网址
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
links = [a['href'] for a in soup.find_all('a', href=True)]
print(links)
这种方法可以高效地收集网页中的所有链接。
使用Python获取特定类型的链接有哪些方法?
如果需要获取特定类型的链接,例如仅限于某个域名或特定后缀的链接,可以在提取时添加条件过滤。可以在列表推导中加入if条件,以筛选满足要求的链接,例如:
filtered_links = [a['href'] for a in soup.find_all('a', href=True) if a['href'].endswith('.pdf')]
这样可以轻松获得所有PDF文件的链接。
在使用Python获取链接时,如何处理反爬虫机制?
许多网站会实施反爬虫机制,阻止自动化的请求。为了解决这个问题,可以考虑设置请求头(headers)模仿浏览器访问,或者使用时间间隔控制请求频率。此外,使用代理IP和随机用户代理(User-Agent)也是常用的技巧。示例代码如下:
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get(url, headers=headers)
通过这些措施,可以提高获取链接的成功率。
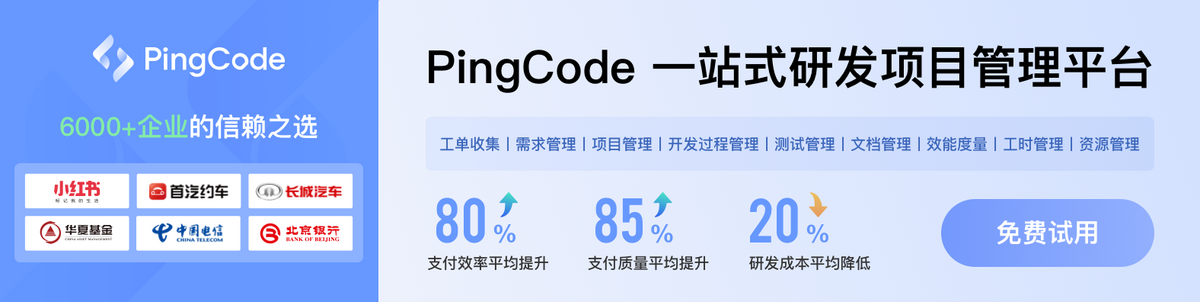