Python可以通过多种方式发送信息,包括电子邮件、短信、即时消息等。常用的方法包括使用smtplib库发送邮件、使用twilio库发送短信、使用requests库与消息API进行交互。 其中,使用smtplib库发送电子邮件是最常见的方式之一。smtplib是Python内置的一个库,用于发送电子邮件。通过配置SMTP服务器的地址和端口,以及用户的邮箱和密码,您可以使用smtplib库发送电子邮件。此外,twilio库可以用于发送短信,而requests库可以用于与各种消息API进行交互,如Slack、Telegram等。
一、使用smtplib库发送电子邮件
smtplib是Python标准库的一部分,非常适合用于发送电子邮件。以下是使用smtplib发送电子邮件的基本步骤:
1.1 配置SMTP服务器
在发送电子邮件之前,您需要配置SMTP服务器的信息。通常,电子邮件提供商(如Gmail、Yahoo等)会提供SMTP服务器的地址和端口。例如,对于Gmail,SMTP服务器地址是smtp.gmail.com
,端口是587。
1.2 编写发送邮件的代码
下面是一个简单的Python代码示例,演示如何使用smtplib发送电子邮件:
import smtplib
from email.mime.text import MIMEText
from email.mime.multipart import MIMEMultipart
def send_email(subject, body, to_email):
# SMTP服务器配置
smtp_server = 'smtp.gmail.com'
smtp_port = 587
from_email = 'your_email@gmail.com'
password = 'your_email_password'
# 创建邮件
msg = MIMEMultipart()
msg['From'] = from_email
msg['To'] = to_email
msg['Subject'] = subject
msg.attach(MIMEText(body, 'plain'))
# 连接到SMTP服务器并发送邮件
server = smtplib.SMTP(smtp_server, smtp_port)
server.starttls()
server.login(from_email, password)
server.sendmail(from_email, to_email, msg.as_string())
server.quit()
使用示例
send_email('Test Subject', 'This is a test email', 'recipient@example.com')
1.3 注意事项
- 安全性:在代码中直接使用明文密码不是一个好习惯,可以考虑使用环境变量或安全存储机制来保护您的密码。
- 授权:某些电子邮件提供商可能需要您启用应用专用密码或降低安全级别以允许第三方应用程序发送邮件。
二、使用twilio库发送短信
Twilio是一个流行的云通信平台,提供了一个简单的API来发送短信和进行电话呼叫。使用Twilio库,您可以轻松地从Python应用程序发送短信。
2.1 注册并获取API凭据
要使用Twilio,首先需要在其官方网站上注册一个帐户,并获取您的帐户SID和身份验证令牌。
2.2 安装Twilio库
在终端中运行以下命令来安装Twilio库:
pip install twilio
2.3 编写发送短信的代码
以下是一个使用Twilio发送短信的示例代码:
from twilio.rest import Client
def send_sms(to_number, message):
# Twilio API凭据
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
from_number = 'your_twilio_number'
client = Client(account_sid, auth_token)
message = client.messages.create(
body=message,
from_=from_number,
to=to_number
)
print(f"Message sent: {message.sid}")
使用示例
send_sms('+1234567890', 'Hello, this is a test SMS!')
2.4 注意事项
- 费用:Twilio的短信服务是收费的,因此在大规模使用之前,请确保了解相关费用。
- 区域限制:某些国家或地区可能对短信发送有特定的法律和合规要求。
三、使用requests库与消息API交互
requests库是一个强大的HTTP库,可以用于与各种Web服务进行交互。许多即时消息平台(如Slack、Telegram等)提供API接口,允许开发者通过HTTP请求发送消息。
3.1 使用Slack API发送消息
Slack是一个流行的团队协作工具,它的API允许开发者发送消息到Slack频道。
3.1.1 创建Slack应用并获取Webhook URL
在Slack中创建一个应用,并添加一个Incoming Webhook功能,获取Webhook URL。
3.1.2 安装requests库
在终端中运行以下命令来安装requests库:
pip install requests
3.1.3 编写发送消息的代码
下面是一个使用Slack API发送消息的示例代码:
import requests
def send_slack_message(webhook_url, message):
payload = {
'text': message
}
response = requests.post(webhook_url, json=payload)
if response.status_code == 200:
print("Message sent successfully")
else:
print(f"Failed to send message: {response.status_code}")
使用示例
send_slack_message('your_webhook_url', 'Hello, this is a message from Python!')
3.2 使用Telegram Bot API发送消息
Telegram是另一个受欢迎的即时通讯应用,它也提供了一个Bot API来发送消息。
3.2.1 创建Telegram Bot并获取API Token
在Telegram中创建一个Bot,并通过BotFather获取API Token。
3.2.2 编写发送消息的代码
以下是一个使用Telegram Bot API发送消息的示例代码:
import requests
def send_telegram_message(api_token, chat_id, message):
url = f"https://api.telegram.org/bot{api_token}/sendMessage"
payload = {
'chat_id': chat_id,
'text': message
}
response = requests.post(url, data=payload)
if response.status_code == 200:
print("Message sent successfully")
else:
print(f"Failed to send message: {response.status_code}")
使用示例
send_telegram_message('your_api_token', 'your_chat_id', 'Hello, this is a message from Python!')
3.3 注意事项
- API限制:使用API时,请注意其速率限制和使用条款。
- 安全性:避免在代码中直接暴露API Token和Webhook URL,建议使用环境变量或其他安全存储方式。
四、其他信息发送方式
除了上述介绍的几种方法,Python还可以通过其他方式发送信息,如使用第三方邮件服务、与社交媒体API交互等。
4.1 使用第三方邮件服务
一些第三方邮件服务如SendGrid、Mailgun等提供了API接口,允许开发者通过其服务发送电子邮件。这些服务通常提供更高级的功能,如批量发送、邮件跟踪等。
4.2 与社交媒体API交互
Python可以通过requests库或社交媒体平台提供的SDK与社交媒体API交互。例如,您可以使用Facebook Graph API发送消息到Facebook Messenger,或使用Twitter API发布推文。
4.3 使用消息队列
在分布式系统中,消息队列(如RabbitMQ、Kafka等)常用于实现异步消息传递。Python有许多库(如pika、kafka-python)可用于与消息队列交互。
4.4 发送推送通知
推送通知是一种直接向用户设备发送信息的方式。Python可以与推送服务(如Firebase Cloud Messaging、OneSignal等)集成,以发送推送通知到移动应用或Web应用。
综上所述,Python提供了多种灵活的方式来发送信息。根据您的具体需求和应用场景,可以选择合适的方法来实现信息发送。无论是通过电子邮件、短信、即时消息,还是其他方式,Python的丰富生态系统都能为您提供强有力的支持。
相关问答FAQs:
如何使用Python发送电子邮件?
Python提供了内置的smtplib
库,可以用来发送电子邮件。你需要设置SMTP服务器,配置发件人和收件人信息,并使用sendmail
方法来发送邮件。以下是一个简单的示例代码:
import smtplib
from email.mime.text import MIMEText
# 配置邮箱信息
smtp_server = 'smtp.example.com'
port = 587 # 或者 465
sender_email = 'your_email@example.com'
receiver_email = 'recipient@example.com'
password = 'your_password'
# 创建邮件内容
message = MIMEText('这是邮件的内容')
message['Subject'] = '邮件主题'
message['From'] = sender_email
message['To'] = receiver_email
# 发送邮件
with smtplib.SMTP(smtp_server, port) as server:
server.starttls() # 启用TLS
server.login(sender_email, password)
server.sendmail(sender_email, receiver_email, message.as_string())
Python可以通过哪些方式发送即时消息?
Python可以通过多种方式发送即时消息,比如使用聊天应用的API(如Slack、Telegram等)。这些平台通常提供RESTful API,允许开发者通过HTTP请求发送消息。你需要注册开发者账号,获取API密钥,然后使用Python的requests
库来发送请求。例如,发送Telegram消息的示例代码如下:
import requests
token = 'your_bot_token'
chat_id = 'your_chat_id'
message = '你好,这是通过Python发送的消息!'
url = f'https://api.telegram.org/bot{token}/sendMessage'
data = {'chat_id': chat_id, 'text': message}
requests.post(url, data=data)
使用Python发送SMS短信的步骤是什么?
要使用Python发送SMS短信,通常需要借助第三方服务,比如Twilio或Nexmo。这些服务提供API可以进行短信发送。你需要在相应网站上注册账号,获取API密钥,以下是使用Twilio发送SMS的基本步骤:
- 安装Twilio库:
pip install twilio
- 创建Twilio账户并获取Account SID和Auth Token。
- 使用以下代码发送短信:
from twilio.rest import Client
account_sid = 'your_account_sid'
auth_token = 'your_auth_token'
client = Client(account_sid, auth_token)
message = client.messages.create(
body='这是通过Python发送的短信',
from_='+1234567890', # Twilio提供的号码
to='+0987654321' # 收件人号码
)
print(f'消息发送成功,SID: {message.sid}')
这些方法可以帮助用户利用Python发送各种类型的信息,满足不同的需求。
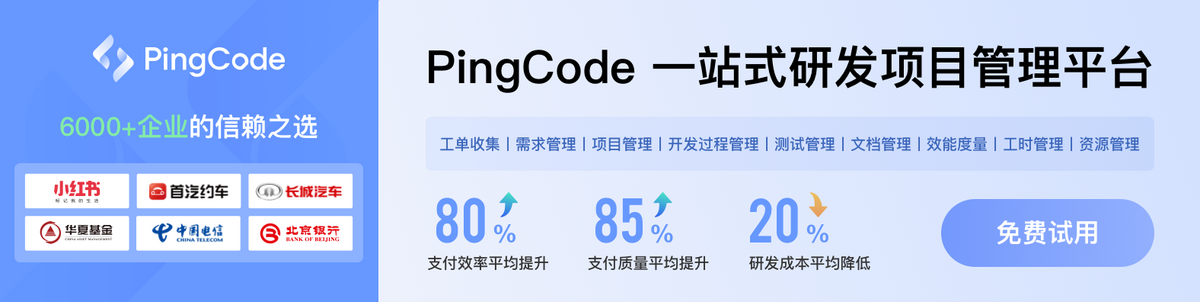