Python爬取标签内容的方法主要有:使用requests库获取网页源代码、利用BeautifulSoup解析HTML文档、通过正则表达式提取特定标签内容。其中,使用BeautifulSoup解析HTML文档是一种常用而高效的方法,因为它提供了更直观的操作方式和灵活的解析功能。下面将详细介绍如何使用BeautifulSoup来爬取网页中的标签内容。
一、安装和使用BeautifulSoup
在使用BeautifulSoup之前,需要确保已经安装了相关的库。可以通过pip命令安装BeautifulSoup和requests库:
pip install beautifulsoup4
pip install requests
安装完成后,就可以开始使用这些库来爬取网页内容。首先,通过requests库获取网页的HTML源代码,然后使用BeautifulSoup解析HTML文档。
import requests
from bs4 import BeautifulSoup
获取网页内容
url = 'http://example.com'
response = requests.get(url)
html_content = response.text
解析网页内容
soup = BeautifulSoup(html_content, 'html.parser')
二、解析HTML文档
通过BeautifulSoup,您可以轻松地解析HTML文档并提取特定标签的内容。可以使用soup.find()
或soup.find_all()
方法查找特定的标签和属性。
- 查找单个标签
soup.find()
方法用于查找第一个匹配的标签。您可以根据标签名或标签的属性进行查找。
# 查找第一个<h1>标签
h1_tag = soup.find('h1')
print(h1_tag.text)
查找具有特定class的<div>标签
div_tag = soup.find('div', class_='specific-class')
print(div_tag.text)
- 查找所有匹配标签
soup.find_all()
方法用于查找所有匹配的标签,返回一个列表。
# 查找所有<p>标签
p_tags = soup.find_all('p')
for tag in p_tags:
print(tag.text)
查找具有特定class的所有<a>标签
a_tags = soup.find_all('a', class_='link-class')
for tag in a_tags:
print(tag['href'])
三、使用正则表达式
除了使用BeautifulSoup提供的方法,还可以使用正则表达式来提取特定格式的内容。正则表达式提供了强大的字符串匹配功能,可以帮助您从HTML文档中提取特定的内容。
import re
使用正则表达式提取所有邮件地址
emails = re.findall(r'[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}', html_content)
for email in emails:
print(email)
四、处理动态网页
有些网页的内容是通过JavaScript动态加载的,使用requests和BeautifulSoup无法直接获取。这时,可以使用Selenium库来模拟浏览器操作,获取动态加载的内容。
- 安装Selenium
pip install selenium
- 配置WebDriver
根据浏览器类型下载对应的WebDriver,并将其路径添加到系统环境变量中。
- 使用Selenium获取动态内容
from selenium import webdriver
设置WebDriver
driver = webdriver.Chrome() # 如果使用Chrome浏览器
driver.get('http://example.com')
获取动态加载的内容
html_content = driver.page_source
soup = BeautifulSoup(html_content, 'html.parser')
提取特定标签内容
tags = soup.find_all('div', class_='dynamic-content')
for tag in tags:
print(tag.text)
关闭WebDriver
driver.quit()
五、处理网页反爬机制
在爬取网页内容时,可能会遇到网站的反爬机制,比如IP封禁、验证码等。以下是一些常用的反爬措施和应对策略:
- 使用代理IP
通过代理IP轮换请求来源,避免被服务器检测到过于频繁的访问请求。
proxies = {
'http': 'http://your.proxy.ip:port',
'https': 'https://your.proxy.ip:port',
}
response = requests.get(url, proxies=proxies)
- 设置请求头
模拟浏览器的请求头信息,以减少被识别为爬虫的可能性。
headers = {
'User-Agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/58.0.3029.110 Safari/537.3'
}
response = requests.get(url, headers=headers)
- 设置访问间隔
在请求之间设置合理的时间间隔,避免短时间内发送大量请求。
import time
访问多个网页
urls = ['http://example.com/page1', 'http://example.com/page2']
for url in urls:
response = requests.get(url)
# 解析和处理网页内容
time.sleep(2) # 设置2秒的访问间隔
六、存储和处理爬取的数据
爬取到的网页内容可以存储在各种格式中,如文本文件、CSV文件、数据库等,以便后续的分析和处理。
- 存储为文本文件
with open('output.txt', 'w', encoding='utf-8') as file:
file.write(html_content)
- 存储为CSV文件
import csv
data = [
['Name', 'Email'],
['John Doe', 'john@example.com'],
['Jane Smith', 'jane@example.com']
]
with open('output.csv', 'w', newline='', encoding='utf-8') as file:
writer = csv.writer(file)
writer.writerows(data)
- 存储到数据库
可以使用SQLite、MySQL、MongoDB等数据库来存储和管理大量的爬取数据。
import sqlite3
连接到SQLite数据库
conn = sqlite3.connect('data.db')
cursor = conn.cursor()
创建表
cursor.execute('''
CREATE TABLE IF NOT EXISTS users (
id INTEGER PRIMARY KEY AUTOINCREMENT,
name TEXT,
email TEXT
)
''')
插入数据
cursor.execute('INSERT INTO users (name, email) VALUES (?, ?)', ('John Doe', 'john@example.com'))
conn.commit()
查询数据
cursor.execute('SELECT * FROM users')
rows = cursor.fetchall()
for row in rows:
print(row)
关闭连接
conn.close()
七、总结
通过本文的介绍,您应该对如何使用Python爬取网页标签内容有了一个全面的了解。使用requests库获取网页源代码、利用BeautifulSoup解析HTML文档、通过正则表达式提取内容是爬取网页的基本步骤。在爬取过程中,可能会遇到动态网页和反爬机制的挑战,可以通过Selenium模拟浏览器操作和使用代理IP等方法来应对。最后,将爬取到的数据存储为文本、CSV或数据库,以便进一步分析和处理。希望这篇文章能够帮助您更好地理解和使用Python进行网页数据爬取。
相关问答FAQs:
如何使用Python提取网页中的特定标签内容?
使用Python提取网页中的特定标签内容通常可以通过库如BeautifulSoup和requests来实现。首先,使用requests库获取网页的HTML内容,然后利用BeautifulSoup解析该内容。通过查找特定的标签(例如<div>
、<span>
、<h1>
等),可以轻松提取所需的数据。以下是一个简单的示例代码:
import requests
from bs4 import BeautifulSoup
url = 'https://example.com'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
tags = soup.find_all('div', class_='example-class') # 根据标签和类名提取
for tag in tags:
print(tag.text)
这种方法可以帮助你提取所需的标签内容,并可以根据需要进行进一步处理。
在进行网页爬取时需要注意哪些法律和道德问题?
进行网页爬取时,遵循法律和道德标准至关重要。首先,确保遵守网站的robots.txt文件中的规定,了解允许爬取的内容。此外,避免对网站服务器施加过大压力,合理设置请求间隔,防止被视为恶意爬虫。还应尊重版权,确保不侵犯他人的知识产权,尤其是在提取和使用数据时。
如何处理爬取过程中遇到的反爬机制?
许多网站会实施反爬机制来防止自动化访问。应对这些机制的方法包括更改请求头部,以模拟真实用户的行为,使用代理服务器来隐藏真实IP,以及在请求之间添加随机延迟。此外,可以考虑使用selenium等工具来模拟浏览器行为,从而更有效地绕过某些反爬措施。然而,始终应遵守网站的使用条款和法律规定。
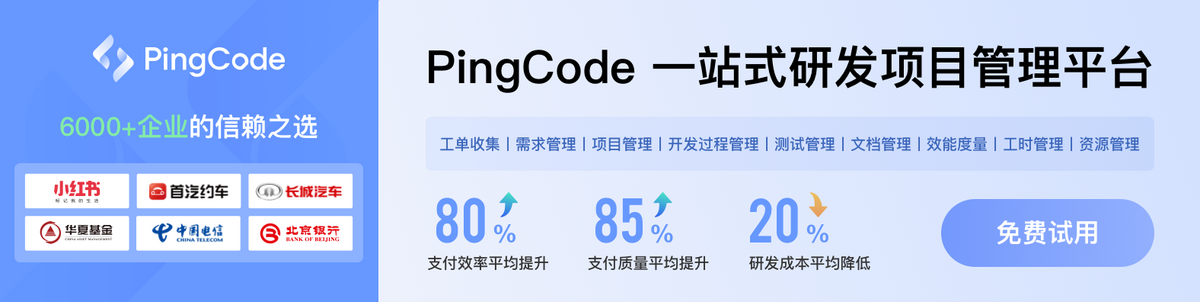