使用Python操作Active Directory (AD)可以通过多种方法实现,例如使用LDAP协议、pyAD库、或者通过PowerShell进行操作。以下将详细介绍如何使用这些方法来操作Active Directory。
要详细介绍其中的一种方式,我们可以深入探讨如何使用LDAP协议通过Python来操作Active Directory。
一、LDAP协议与Python操作AD
LDAP(Lightweight Directory Access Protocol)是一种轻量级的目录访问协议,广泛用于访问和管理目录服务,包括Active Directory。
- 安装Python LDAP库
要在Python中使用LDAP,首先需要安装相应的库。可以使用python-ldap
库来进行LDAP操作。
pip install python-ldap
- 连接到Active Directory
使用python-ldap
库连接到AD需要知道域控制器的地址和用户的凭证信息。
import ldap
连接到AD服务器
ldap_server = "ldap://your_ad_server"
conn = ldap.initialize(ldap_server)
绑定用户名和密码
username = "your_username"
password = "your_password"
try:
conn.simple_bind_s(username, password)
print("Successfully connected to AD")
except ldap.LDAPError as e:
print("Failed to connect to AD", e)
- 搜索Active Directory
一旦连接成功,就可以在AD中执行搜索操作。这通常用于查找用户、组或其他对象。
base_dn = "dc=example,dc=com"
search_filter = "(objectClass=user)"
try:
result = conn.search_s(base_dn, ldap.SCOPE_SUBTREE, search_filter)
for dn, entry in result:
print("DN:", dn)
print("Entry:", entry)
except ldap.LDAPError as e:
print("Failed to search AD", e)
- 添加、修改和删除条目
除了搜索,你还可以通过python-ldap
库添加、修改或删除AD中的条目。
- 添加条目:
dn = "cn=new_user,ou=users,dc=example,dc=com"
entry = [
('objectClass', [b'top', b'person', b'organizationalPerson', b'user']),
('cn', [b'new_user']),
('sn', [b'user']),
('userPassword', [b'password']),
]
try:
conn.add_s(dn, entry)
print("Entry added successfully")
except ldap.LDAPError as e:
print("Failed to add entry", e)
- 修改条目:
dn = "cn=existing_user,ou=users,dc=example,dc=com"
mod_attrs = [(ldap.MOD_REPLACE, 'sn', b'new_last_name')]
try:
conn.modify_s(dn, mod_attrs)
print("Entry modified successfully")
except ldap.LDAPError as e:
print("Failed to modify entry", e)
- 删除条目:
dn = "cn=old_user,ou=users,dc=example,dc=com"
try:
conn.delete_s(dn)
print("Entry deleted successfully")
except ldap.LDAPError as e:
print("Failed to delete entry", e)
二、使用pyAD库操作AD
pyAD
是另一个用于操作Active Directory的Python库,提供了更高层次的接口,简化了AD操作。
- 安装pyAD库
pip install pyad
- 连接到Active Directory
pyAD
提供了一种更高级的方式来连接和操作AD。
from pyad import aduser
查找用户
user = aduser.ADUser.from_cn("existing_user")
print(user)
- 常见操作
- 获取用户信息:
print(user.get_attribute("mail"))
- 修改用户属性:
user.update_attribute("description", "Updated description")
- 创建新用户:
from pyad import adcontainer, aduser
ou = adcontainer.ADContainer.from_dn("ou=users,dc=example,dc=com")
new_user = aduser.ADUser.create("new_user", ou, password="password")
三、使用PowerShell与Python结合操作AD
- 安装相应库
使用pywinrm
库可以在Python中远程执行PowerShell命令。
pip install pywinrm
- 通过PowerShell命令操作AD
使用PowerShell命令可以实现更多复杂的AD操作。
import winrm
创建WinRM会话
s = winrm.Session('http://your_ad_server:5985/wsman', auth=('username', 'password'))
执行PowerShell命令
r = s.run_cmd('powershell Get-ADUser -Filter *')
print(r.std_out)
以上是使用Python操作Active Directory的一些常见方法。具体选择哪种方式,取决于你所面临的需求和环境。无论是通过LDAP协议、使用pyAD库还是结合PowerShell,Python都能为Active Directory的管理提供灵活的解决方案。在实际使用中,确保遵循安全策略,妥善管理和保护连接凭证和敏感数据。
相关问答FAQs:
如何使用Python连接到Active Directory (AD)?
要连接到Active Directory,您可以使用ldap3
库。首先,您需要安装该库,可以通过命令pip install ldap3
完成。连接时,您需要提供AD服务器的地址、用户名和密码。例如:
from ldap3 import Server, Connection, ALL
server = Server('your_ad_server', get_info=ALL)
conn = Connection(server, 'user_dn', 'password', auto_bind=True)
确保替换your_ad_server
、user_dn
和password
为实际的值。成功连接后,您就可以进行查询和修改操作。
使用Python如何查询Active Directory中的用户信息?
在连接到Active Directory后,您可以使用conn.search()
方法查询用户信息。例如,查询特定用户的属性:
conn.search('ou=users,dc=example,dc=com', '(sAMAccountName=username)', attributes=['displayName', 'mail'])
print(conn.entries)
替换ou=users,dc=example,dc=com
和username
为您实际的组织单位和用户账号。查询结果将包含用户的显示名称和电子邮件地址等信息。
可以用Python实现哪些Active Directory的管理操作?
使用Python对Active Directory的管理操作非常灵活,您可以创建、更新或删除用户、组和计算机账户。例如,创建新用户可以通过以下代码实现:
from ldap3 import Server, Connection, ALL, MODIFY_ADD
server = Server('your_ad_server', get_info=ALL)
conn = Connection(server, 'user_dn', 'password', auto_bind=True)
conn.add('cn=new_user,ou=users,dc=example,dc=com', 'user', {'givenName': 'New', 'sn': 'User', 'userPrincipalName': 'new_user@example.com'})
记得根据需要调整用户的详细信息和DN。通过这种方式,您可以高效地管理Active Directory中的各类账户。
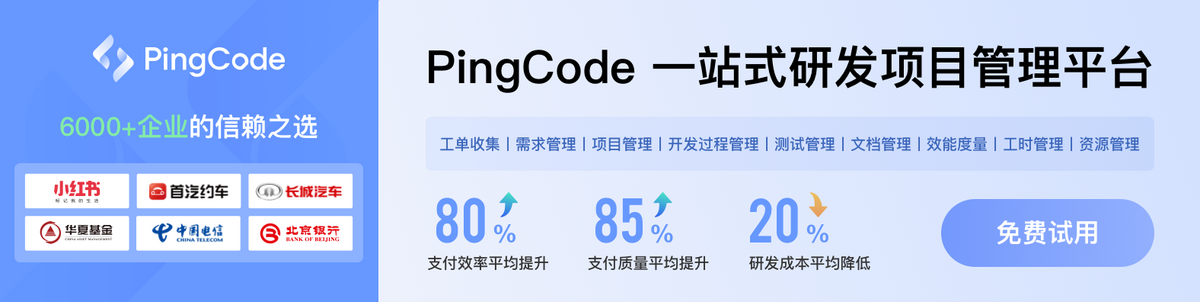