在Python中,实现文件保护可以通过设置文件权限、加密文件内容、使用密码保护、备份文件等方法来实现。其中,加密文件内容是一种常见且有效的文件保护手段。通过使用加密技术,可以确保即使文件被未经授权的人员访问,也无法读取其内容。下面将详细介绍这些方法,并提供一些实现的示例代码。
一、设置文件权限
在操作系统中,每个文件都有相应的权限设置,这些权限决定了哪些用户可以读取、写入或执行文件。在Python中,可以使用os
模块来修改文件的权限。
- 使用os.chmod设置文件权限
os.chmod()
函数可以用来修改文件的权限。权限通常以八进制数表示,如0o755
表示所有者可读、可写、可执行,组用户和其他用户可读、可执行。
import os
设置文件路径
file_path = 'example.txt'
设置文件权限为755
os.chmod(file_path, 0o755)
- 使用os.umask设置默认权限
os.umask()
函数用于设置当前进程的文件创建权限屏蔽字。通过umask,可以控制新文件的默认权限。
import os
设置默认权限屏蔽字
os.umask(0o077)
二、加密文件内容
加密是保护文件内容的重要方法。通过加密,文件内容被转换为不可读的形式,只有持有正确密钥的人才能解密读取。
- 对称加密
对称加密算法使用相同的密钥进行加密和解密。常用的对称加密算法包括AES、DES等。
from Crypto.Cipher import AES
import os
def encrypt_file(file_name, key):
with open(file_name, 'rb') as file:
plaintext = file.read()
# 使用AES对称加密
cipher = AES.new(key, AES.MODE_EAX)
ciphertext, tag = cipher.encrypt_and_digest(plaintext)
with open(file_name + '.enc', 'wb') as file_enc:
[file_enc.write(x) for x in (cipher.nonce, tag, ciphertext)]
def decrypt_file(file_name_enc, key):
with open(file_name_enc, 'rb') as file_enc:
nonce, tag, ciphertext = [file_enc.read(x) for x in (16, 16, -1)]
cipher = AES.new(key, AES.MODE_EAX, nonce=nonce)
plaintext = cipher.decrypt_and_verify(ciphertext, tag)
with open(file_name_enc[:-4], 'wb') as file_dec:
file_dec.write(plaintext)
使用示例
key = os.urandom(16) # 生成随机密钥
encrypt_file('example.txt', key)
decrypt_file('example.txt.enc', key)
- 非对称加密
非对称加密使用一对密钥:公钥和私钥。公钥用于加密,私钥用于解密。常用的非对称加密算法包括RSA等。
from Crypto.PublicKey import RSA
from Crypto.Cipher import PKCS1_OAEP
def generate_keys():
key = RSA.generate(2048)
private_key = key.export_key()
public_key = key.publickey().export_key()
return private_key, public_key
def encrypt_file_with_public_key(file_name, public_key):
with open(file_name, 'rb') as file:
plaintext = file.read()
recipient_key = RSA.import_key(public_key)
cipher_rsa = PKCS1_OAEP.new(recipient_key)
ciphertext = cipher_rsa.encrypt(plaintext)
with open(file_name + '.enc', 'wb') as file_enc:
file_enc.write(ciphertext)
def decrypt_file_with_private_key(file_name_enc, private_key):
with open(file_name_enc, 'rb') as file_enc:
ciphertext = file_enc.read()
key = RSA.import_key(private_key)
cipher_rsa = PKCS1_OAEP.new(key)
plaintext = cipher_rsa.decrypt(ciphertext)
with open(file_name_enc[:-4], 'wb') as file_dec:
file_dec.write(plaintext)
使用示例
private_key, public_key = generate_keys()
encrypt_file_with_public_key('example.txt', public_key)
decrypt_file_with_private_key('example.txt.enc', private_key)
三、使用密码保护
可以通过要求用户输入密码来访问文件,从而增加一层安全保护。密码可以用于加密密钥或直接用于文件加密。
- 基于密码的文件加密
可以使用Python的cryptography
库中的Fernet
方法来实现基于密码的加密。
from cryptography.fernet import Fernet
import base64
import os
from cryptography.hazmat.primitives import hashes
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
def generate_key_from_password(password, salt):
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32,
salt=salt,
iterations=100000,
)
return base64.urlsafe_b64encode(kdf.derive(password.encode()))
def encrypt_file_with_password(file_name, password):
salt = os.urandom(16)
key = generate_key_from_password(password, salt)
fernet = Fernet(key)
with open(file_name, 'rb') as file:
plaintext = file.read()
ciphertext = fernet.encrypt(plaintext)
with open(file_name + '.enc', 'wb') as file_enc:
file_enc.write(salt + ciphertext)
def decrypt_file_with_password(file_name_enc, password):
with open(file_name_enc, 'rb') as file_enc:
salt = file_enc.read(16)
ciphertext = file_enc.read()
key = generate_key_from_password(password, salt)
fernet = Fernet(key)
plaintext = fernet.decrypt(ciphertext)
with open(file_name_enc[:-4], 'wb') as file_dec:
file_dec.write(plaintext)
使用示例
password = 'my_secure_password'
encrypt_file_with_password('example.txt', password)
decrypt_file_with_password('example.txt.enc', password)
四、备份文件
定期备份文件是保护文件的重要手段之一。备份可以防止因意外删除、硬件故障等导致的数据丢失。
- 手动备份
可以定期手动将重要文件复制到安全的位置,如外部硬盘、云存储等。
- 自动备份
可以使用Python脚本实现文件的自动备份。
import shutil
import os
import time
def backup_file(src_file, dest_dir):
if not os.path.exists(dest_dir):
os.makedirs(dest_dir)
file_name = os.path.basename(src_file)
backup_name = f"{file_name}_{int(time.time())}.bak"
shutil.copy(src_file, os.path.join(dest_dir, backup_name))
使用示例
backup_file('example.txt', 'backup_directory')
通过以上方法,可以有效地实现文件保护,确保文件的安全性和完整性。在实际应用中,可以根据具体需求选择合适的方法,或者结合多种方法以提高文件的安全性。
相关问答FAQs:
如何在Python中加密文件以保护内容?
在Python中,可以使用库如 cryptography
来加密文件。首先,安装该库并导入所需模块。然后,使用对称加密算法(如AES)生成密钥,并利用该密钥对文件内容进行加密。加密后的文件可以安全存储,而只有持有密钥的人才能解密访问。
有哪些方法可以限制Python文件的访问权限?
可以通过操作系统的文件权限设置来限制文件的访问。在Python中,可以使用 os
模块来修改文件权限,例如使用 os.chmod()
函数。通过设置文件为只读或限制特定用户的访问,可以有效保护文件内容。
在Python中如何检测文件是否被篡改?
可以通过生成文件的哈希值来检测文件的完整性。Python中的 hashlib
模块可以计算文件的MD5或SHA-256哈希值。保存初始哈希值后,任何时候通过重新计算哈希值与原始值对比,便能判断文件是否被篡改。这样可以有效保护文件内容的安全性。
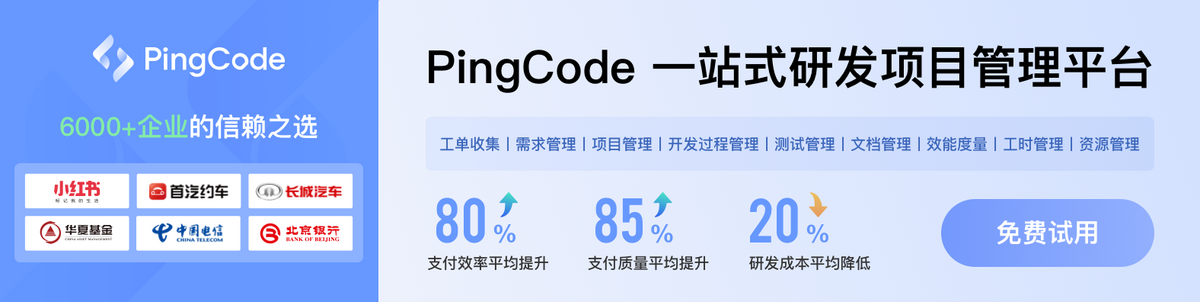