在Python3中,字符串替换可以通过以下几种方式实现:使用str.replace()方法、正则表达式re.sub()函数、字符串模板替换。其中,str.replace()
是最常用的方法,可以直接替换字符串中的某些字符或子字符串。接下来,我们将深入探讨每种方法的使用场景和具体实现。
一、STR.REPLACE()方法
str.replace()
是Python中最简单、最直接的字符串替换方法。它的基本用法是将一个字符串中的某一部分替换为另一部分。这个方法有两个必需参数:要替换的旧字符串和替换为的新字符串,还有一个可选参数是替换次数。
- 基本使用
str.replace(old, new)
会将字符串中所有的old
子字符串替换为new
。例如:
text = "Hello, World!"
new_text = text.replace("World", "Python")
print(new_text) # 输出: Hello, Python!
在这个例子中,字符串中的"World"被替换成了"Python"。
- 限定替换次数
通过设置第三个参数count
,你可以限定替换的次数:
text = "banana banana banana"
new_text = text.replace("banana", "apple", 2)
print(new_text) # 输出: apple apple banana
这里,只有前两个"banana"被替换成了"apple"。
二、RE.SUB()函数
re.sub()
是正则表达式模块中的一个函数,适用于更复杂的字符串替换,特别是当你需要使用模式匹配的时候。
- 基本用法
re.sub(pattern, repl, string)
用于将string
中所有匹配pattern
的部分替换为repl
。例如:
import re
text = "The rain in Spain"
new_text = re.sub(r"ain", "xxx", text)
print(new_text) # 输出: The rxxx in Spxxx
在这个例子中,所有匹配模式"ain"的部分都被替换成了"xxx"。
- 使用复杂的正则表达式
正则表达式提供了强大的模式匹配功能,可以用来实现更复杂的替换操作。例如,替换文本中的所有数字:
import re
text = "There are 2 apples and 5 bananas."
new_text = re.sub(r"\d+", "some", text)
print(new_text) # 输出: There are some apples and some bananas.
这个例子中,\d+
是一个正则表达式,匹配一个或多个数字。
三、字符串模板替换
Python中也可以使用模板替换,这是通过string.Template
类实现的。这种方式适合需要在字符串中进行多处替换的场景。
- 使用模板进行替换
首先,你需要导入Template
类,然后定义一个模板字符串,在字符串中使用$
进行占位:
from string import Template
template = Template("Hello, $name!")
new_text = template.substitute(name="Alice")
print(new_text) # 输出: Hello, Alice!
在这个例子中,$name
是一个占位符,使用substitute()
方法进行替换。
- 使用字典进行批量替换
Template
的substitute()
方法也可以接受一个字典参数,用于同时替换多个占位符:
from string import Template
template = Template("Hello, $name! Welcome to $place.")
data = {"name": "Alice", "place": "Wonderland"}
new_text = template.substitute(data)
print(new_text) # 输出: Hello, Alice! Welcome to Wonderland.
通过这种方法,可以很方便地进行批量替换。
四、在文件中替换
在实际应用中,字符串替换不仅限于内存中的字符串,还常常需要对文件进行操作。
- 读取文件并替换内容
可以先读取文件内容,然后进行字符串替换,最后将替换后的内容写回文件:
with open("example.txt", "r") as file:
content = file.read()
content = content.replace("old_string", "new_string")
with open("example.txt", "w") as file:
file.write(content)
这种方法适用于简单的替换操作。
- 使用正则表达式替换文件内容
如果需要进行复杂的替换,可以结合正则表达式:
import re
with open("example.txt", "r") as file:
content = file.read()
content = re.sub(r"pattern", "replacement", content)
with open("example.txt", "w") as file:
file.write(content)
这种方法适合需要用正则表达式进行模式匹配的情况。
五、替换列表中的字符串
如果你需要对列表中的每个字符串进行替换,可以使用列表推导式:
- 使用列表推导式替换
fruits = ["apple", "banana", "cherry"]
new_fruits = [fruit.replace("a", "o") for fruit in fruits]
print(new_fruits) # 输出: ['opple', 'bonono', 'cherry']
这种方法简洁明了,适合简单的替换操作。
- 结合正则表达式
同样地,如果需要使用正则表达式,可以结合re.sub()
:
import re
fruits = ["apple", "banana", "cherry"]
new_fruits = [re.sub(r"a", "o", fruit) for fruit in fruits]
print(new_fruits) # 输出: ['opple', 'bonono', 'cherry']
这种方法适用于需要更复杂的替换规则的情况。
六、替换字典中的字符串
在字典中进行字符串替换时,可以遍历字典并对每个值进行替换:
- 遍历字典并替换
data = {"name": "Alice", "place": "Wonderland"}
new_data = {key: value.replace("a", "o") for key, value in data.items()}
print(new_data) # 输出: {'name': 'Aloce', 'place': 'Wonderlond'}
这种方法适合对字典中的所有值进行统一替换。
- 使用正则表达式
同样地,正则表达式也可以用于字典中的字符串替换:
import re
data = {"name": "Alice", "place": "Wonderland"}
new_data = {key: re.sub(r"a", "o", value) for key, value in data.items()}
print(new_data) # 输出: {'name': 'Aloce', 'place': 'Wonderlond'}
这种方法适合需要使用正则表达式进行复杂替换的情况。
通过以上多种方法,Python3为字符串替换提供了强大的工具,可以满足从简单到复杂的各种替换需求。根据具体的应用场景,选择合适的方法可以提高代码的效率和可读性。
相关问答FAQs:
如何在Python3中替换字符串中的特定字符或子串?
在Python3中,您可以使用字符串的replace()
方法来替换特定字符或子串。例如,如果您想将字符串中的所有“apple”替换为“orange”,可以使用如下代码:
original_string = "I like apple and apple pie."
new_string = original_string.replace("apple", "orange")
print(new_string) # 输出: I like orange and orange pie.
此方法可以指定替换的次数,如果不传入该参数,则会替换所有匹配项。
在Python3中如何使用正则表达式进行复杂的替换?
若需要进行更复杂的替换,您可以使用re
模块中的sub()
函数。此方法支持正则表达式,允许您进行模式匹配和替换。例如,以下代码将替换所有数字为“#”:
import re
original_string = "My phone number is 123-456-7890."
new_string = re.sub(r'\d+', '#', original_string)
print(new_string) # 输出: My phone number is #-#-#.
使用正则表达式,您能够更灵活地定义需要替换的内容。
如何在Python3中替换列表中的多个元素?
如果您有一个字符串列表,并希望同时替换其中的多个元素,您可以使用列表推导式结合replace()
方法。例如,以下代码将同时替换“apple”和“banana”:
fruits = ["apple", "banana", "cherry", "apple pie", "banana split"]
new_fruits = [fruit.replace("apple", "orange").replace("banana", "grape") for fruit in fruits]
print(new_fruits) # 输出: ['orange', 'grape', 'cherry', 'orange pie', 'grape split']
这种方法能够有效地处理多个替换,使代码简洁且易于维护。
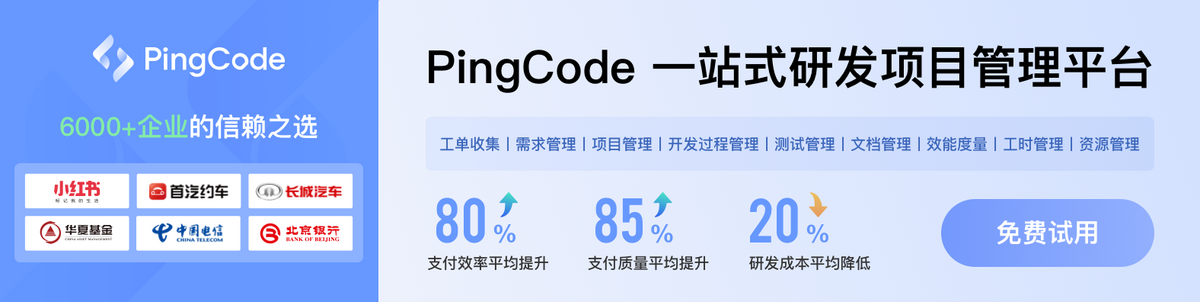