制作渐变背景在Python中可以通过多种方式实现,使用PIL库、使用matplotlib库、使用numpy结合matplotlib库是常见的几种方法。接下来,我将详细介绍其中一种方法,即使用PIL库创建渐变背景。
要创建一个渐变背景,可以使用Python的PIL(Python Imaging Library)库中的Image模块。PIL是处理图像的一个强大工具,允许我们创建、修改和保存图像。通过利用PIL中的功能,我们可以轻松实现渐变效果。
一、使用PIL库实现渐变背景
PIL(现已合并为Pillow)是Python中处理图像的标准库。通过PIL,我们可以创建一个新的图像,然后逐像素地设置颜色值,从而实现渐变效果。
1. 安装Pillow库
首先,我们需要确保Pillow库已安装,可以通过以下命令安装:
pip install pillow
2. 创建渐变背景
创建渐变背景的基本步骤如下:
- 创建一个新的RGB图像。
- 使用一个循环,逐行设置每个像素的颜色值。
- 保存生成的图像。
下面是一个示例代码,演示如何使用PIL创建简单的线性渐变背景:
from PIL import Image
def create_gradient(width, height, start_color, end_color, direction='horizontal'):
# 创建一个新的图像
image = Image.new("RGB", (width, height))
# 获取起始和结束颜色的RGB分量
start_r, start_g, start_b = start_color
end_r, end_g, end_b = end_color
# 生成渐变
for i in range(width if direction == 'horizontal' else height):
# 计算渐变比例
ratio = i / (width if direction == 'horizontal' else height)
# 计算当前颜色
r = int(start_r + (end_r - start_r) * ratio)
g = int(start_g + (end_g - start_g) * ratio)
b = int(start_b + (end_b - start_b) * ratio)
# 绘制行/列
if direction == 'horizontal':
for j in range(height):
image.putpixel((i, j), (r, g, b))
else:
for j in range(width):
image.putpixel((j, i), (r, g, b))
return image
定义图像尺寸和渐变颜色
width, height = 400, 200
start_color = (255, 0, 0) # 红色
end_color = (0, 0, 255) # 蓝色
创建并保存渐变图像
gradient_image = create_gradient(width, height, start_color, end_color, 'horizontal')
gradient_image.save("gradient.png")
3. 代码解析
- 创建图像:使用
Image.new("RGB", (width, height))
创建一个新的RGB图像。 - 设置颜色值:通过计算比例
ratio
来确定每个像素的位置在渐变中的位置,并使用线性插值计算当前的颜色值。 - 绘制颜色:使用
putpixel
方法将计算出的颜色应用到图像的每个像素。
二、使用matplotlib创建渐变背景
matplotlib是Python中的一个绘图库,虽然主要用于绘制图表,但也可以用于创建渐变背景。以下是如何使用matplotlib创建渐变背景的方法。
1. 安装matplotlib库
首先,确保matplotlib已安装,可以通过以下命令安装:
pip install matplotlib
2. 创建渐变背景
使用matplotlib可以通过imshow方法创建渐变背景。
import numpy as np
import matplotlib.pyplot as plt
def create_gradient(width, height, start_color, end_color):
# 创建一个空白数组
gradient = np.zeros((height, width, 3), dtype=np.uint8)
# 生成渐变
for i in range(width):
ratio = i / width
r = int(start_color[0] + (end_color[0] - start_color[0]) * ratio)
g = int(start_color[1] + (end_color[1] - start_color[1]) * ratio)
b = int(start_color[2] + (end_color[2] - start_color[2]) * ratio)
gradient[:, i] = [r, g, b]
return gradient
定义图像尺寸和渐变颜色
width, height = 400, 200
start_color = (255, 0, 0) # 红色
end_color = (0, 0, 255) # 蓝色
创建渐变
gradient = create_gradient(width, height, start_color, end_color)
绘制渐变
plt.imshow(gradient)
plt.axis('off') # 关闭坐标轴
plt.show()
三、使用numpy结合matplotlib创建渐变背景
通过将numpy与matplotlib结合使用,可以更灵活地创建渐变背景。
1. 安装numpy库
确保numpy已安装,可以通过以下命令安装:
pip install numpy
2. 创建渐变背景
下面是使用numpy和matplotlib创建渐变背景的示例代码:
import numpy as np
import matplotlib.pyplot as plt
def create_gradient_v2(width, height, start_color, end_color):
# 创建一个线性梯度
gradient = np.linspace(0, 1, width)
# 创建一个空白数组
gradient_rgb = np.zeros((height, width, 3), dtype=np.float32)
# 将线性梯度应用到RGB通道
for i in range(3):
gradient_rgb[:, :, i] = gradient * (end_color[i] - start_color[i]) + start_color[i]
return gradient_rgb
定义图像尺寸和渐变颜色
width, height = 400, 200
start_color = np.array([255, 0, 0]) / 255 # 红色
end_color = np.array([0, 0, 255]) / 255 # 蓝色
创建渐变
gradient_rgb = create_gradient_v2(width, height, start_color, end_color)
绘制渐变
plt.imshow(gradient_rgb)
plt.axis('off') # 关闭坐标轴
plt.show()
四、总结
通过以上介绍,我们可以看到在Python中创建渐变背景的方法多种多样,可以根据具体需求选择合适的方法。PIL库适合需要精确控制像素的情况,matplotlib则提供了更高层次的绘图功能,结合numpy可以实现更加复杂的渐变效果。选择合适的工具和方法可以大大提高开发效率和代码的可读性。希望这篇文章能够帮助你在Python中创建出满意的渐变背景。
相关问答FAQs:
如何在Python中创建渐变背景的最佳库是什么?
在Python中,有几个库可以帮助您创建渐变背景。最常用的包括Matplotlib和PIL(Pillow)。Matplotlib非常适合数据可视化,并且可以轻松生成渐变色图像;而PIL则更适合图像处理和生成静态图像。您可以根据自己的需求选择合适的库。
渐变背景的颜色选取有什么技巧?
选择渐变背景的颜色时,可以考虑使用色轮工具来挑选互补色或相邻色,这样可以确保颜色之间的和谐。另一种方法是使用在线渐变生成器,帮助您快速找到合适的颜色组合。渐变的起始和结束颜色可以根据主题或情感调性进行调整,以增强视觉效果。
如何在渐变背景中添加其他图形元素?
在渐变背景中添加图形元素时,可以使用透明度设置来确保背景不干扰前景元素的可见性。可以通过调整Alpha通道来实现。使用Matplotlib时,您可以在绘制渐变背景后,利用plt.imshow()
或其他绘图函数叠加所需的图形、文本或图像。确保对前景元素进行合理的位置和大小调整,以实现最佳的视觉效果。
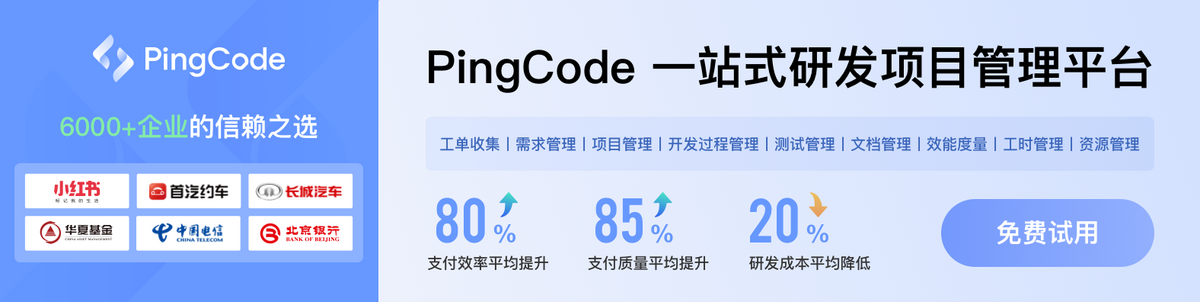