Python中保证线程安全的方法包括:使用锁机制、线程局部存储、使用队列、避免共享状态。其中,使用锁机制是最常用的方法之一,它可以有效防止多个线程同时访问共享资源,导致数据不一致的情况发生。
在多线程编程中,线程之间共享数据会引发数据竞争问题,从而导致数据不一致。Python通过提供线程锁(如Lock、RLock)来解决这个问题。锁机制可以保证同一时间只有一个线程能够访问共享资源,其他线程必须等待。这种机制虽然会影响程序的并发性,但在需要保证数据一致性的场景下是必要的。
接下来将详细介绍Python中保证线程安全的几种方法。
一、使用锁机制
锁机制是解决线程安全问题最常用的方法之一。Python的threading
模块提供了多种锁机制,包括简单的锁(Lock)、可重入锁(RLock)、条件变量(Condition)、信号量(Semaphore)等。
-
锁(Lock)
Lock是最基本的锁机制,它用于保护共享资源。一个Lock对象有两个状态:锁定(locked)和非锁定(unlocked)。线程在访问共享资源前需要获取锁,访问完成后释放锁。以下是一个使用Lock的示例:
import threading
balance = 0
lock = threading.Lock()
def update_balance(amount):
global balance
with lock:
balance += amount
threads = []
for i in range(5):
t = threading.Thread(target=update_balance, args=(100,))
threads.append(t)
t.start()
for t in threads:
t.join()
print(f"Final balance: {balance}")
在这个例子中,多个线程尝试更新共享变量
balance
。通过使用Lock,保证了同一时间只有一个线程能够修改balance
。 -
可重入锁(RLock)
RLock(Reentrant Lock)允许同一线程多次获得锁,这是Lock所不具备的特性。RLock在递归调用或需要多次加锁的场景中很有用。用法与Lock类似:
import threading
rlock = threading.RLock()
def recursive_function(n):
with rlock:
if n > 0:
print(f"Level: {n}")
recursive_function(n - 1)
recursive_function(3)
-
条件变量(Condition)
Condition变量用于线程间的通信和协作。它允许一个或多个线程等待某个条件变为真时被唤醒。
import threading
condition = threading.Condition()
products = []
def producer():
with condition:
products.append("product")
print("Produced a product")
condition.notify()
def consumer():
with condition:
while not products:
condition.wait()
print(f"Consumed a {products.pop()}")
threading.Thread(target=consumer).start()
threading.Thread(target=producer).start()
在这个例子中,生产者线程生产一个产品并通知消费者线程。消费者线程在产品列表为空时等待,直到被生产者线程唤醒。
-
信号量(Semaphore)
Semaphore用于控制对共享资源的访问线程数。它维护一个计数器,计数器大于0时,线程可以访问资源并减少计数器,释放时增加计数器。
import threading
semaphore = threading.Semaphore(2)
def access_resource():
with semaphore:
print("Accessing resource")
# 模拟对资源的访问
threading.Event().wait(1)
threads = [threading.Thread(target=access_resource) for _ in range(5)]
for t in threads:
t.start()
for t in threads:
t.join()
在这个例子中,信号量限制了同时访问资源的线程数为2。
二、线程局部存储
线程局部存储(Thread-Local Storage)允许每个线程维护自己的数据副本,而不与其他线程共享。这在需要线程独立的数据处理时非常有用。Python的threading.local()
提供了线程局部存储的支持。
import threading
local_data = threading.local()
def process_data(data):
local_data.value = data
print(f"Thread {threading.current_thread().name} has value {local_data.value}")
threads = []
for i in range(5):
t = threading.Thread(target=process_data, args=(i,))
threads.append(t)
t.start()
for t in threads:
t.join()
在这个例子中,每个线程有自己的local_data.value
,互不干扰。
三、使用队列
使用线程安全的队列(Queue)是避免数据竞争的另一种方法。Python的queue
模块提供了线程安全的队列实现,如Queue、LifoQueue、PriorityQueue等。
import threading
import queue
q = queue.Queue()
def producer():
for i in range(5):
q.put(i)
print(f"Produced {i}")
def consumer():
while not q.empty():
item = q.get()
print(f"Consumed {item}")
q.task_done()
threading.Thread(target=producer).start()
threading.Thread(target=consumer).start()
在这个例子中,生产者将数据放入队列,消费者从队列中取数据,队列保证了线程安全。
四、避免共享状态
在某些情况下,避免共享状态可以减少线程安全问题的复杂性。可以通过以下方式实现:
-
使用不可变对象:不可变对象(如tuple、str)在多线程环境中是安全的,因为它们无法被修改。
-
使用进程而非线程:Python的
multiprocessing
模块提供了与线程类似的接口,但使用进程而非线程,避免了GIL(全局解释器锁)带来的性能限制。 -
设计无状态的函数:函数尽量不依赖于外部状态,减少共享数据,可以通过传递参数或返回值来传递数据。
通过上述方法,可以有效解决Python中的线程安全问题。在实际开发中,应根据具体场景选择合适的方法来保证线程安全。
相关问答FAQs:
如何在Python中实现线程安全的机制?
在Python中,实现线程安全的机制主要依赖于使用锁(Lock)、条件变量(Condition)、信号量(Semaphore)等同步原语。通过使用这些工具,多个线程可以安全地访问共享资源,从而避免数据竞争和不一致性问题。例如,使用threading.Lock()
可以在进入临界区时锁定资源,确保同一时间只有一个线程能够访问该资源。
Python中有哪些常用的线程安全数据结构?
Python的标准库提供了一些线程安全的数据结构,例如queue.Queue
、collections.deque
和threading.Event
等。queue.Queue
是一个线程安全的FIFO队列,非常适合用于生产者-消费者模型。collections.deque
也可以安全地在多线程环境下使用,特别适合需要快速插入和删除操作的场景。
如何检测并解决Python程序中的线程安全问题?
识别线程安全问题通常需要通过仔细的代码审查和调试工具来进行。可以使用日志记录、调试器和性能分析工具来监测共享资源的访问情况。如果发现数据竞争或不一致性,可以通过引入锁、重构代码或使用线程安全的数据结构来解决这些问题。此外,使用测试框架编写多线程的单元测试也是一个有效的策略。
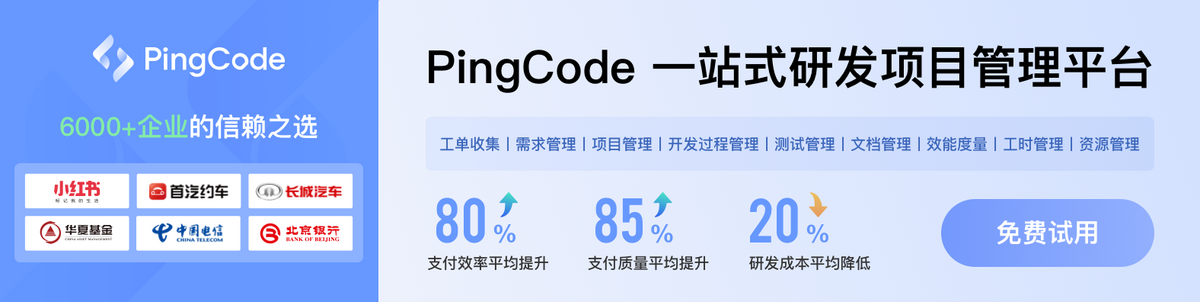