一、如何用Python删除文件
使用Python删除文件的方法主要有os.remove()、os.unlink()和pathlib.Path.unlink()。os.remove()和os.unlink()基本上是等效的,两者都可以用于删除文件;而pathlib.Path.unlink()提供了一种更面向对象的方式来进行文件删除。本文将详细介绍这三种方法及其用法,并特别着重于os.remove()的使用。
使用os.remove()时,我们首先需要导入os模块。os模块提供了操作系统接口的功能,os.remove()是用于删除文件的一种常用方法。假如我们要删除一个名为“sample.txt”的文件,只需调用os.remove('sample.txt')。这种方式简单直接,但在使用前务必确保文件存在,否则会抛出FileNotFoundError错误。
二、os模块的使用
1、os.remove()方法
os.remove()是Python中删除文件的基本方法之一。它通过调用操作系统底层的命令来实现文件的删除。
import os
def delete_file(file_path):
try:
os.remove(file_path)
print(f"File '{file_path}' has been deleted successfully.")
except FileNotFoundError:
print(f"File '{file_path}' not found.")
except PermissionError:
print(f"Permission denied to delete '{file_path}'.")
except Exception as e:
print(f"An error occurred: {e}")
示例
delete_file("sample.txt")
在此代码中,os.remove()被用来尝试删除指定路径的文件。如果文件不存在,将抛出FileNotFoundError;如果权限不足,将抛出PermissionError。通过捕获这些异常,我们可以更好地处理错误情况。
2、os.unlink()方法
os.unlink()与os.remove()在功能上是相同的,它们都用于删除文件。两者可以互换使用。
import os
def unlink_file(file_path):
try:
os.unlink(file_path)
print(f"File '{file_path}' has been deleted successfully.")
except FileNotFoundError:
print(f"File '{file_path}' not found.")
except PermissionError:
print(f"Permission denied to delete '{file_path}'.")
except Exception as e:
print(f"An error occurred: {e}")
示例
unlink_file("sample.txt")
三、pathlib模块的使用
1、pathlib.Path.unlink()方法
pathlib模块提供了一种面向对象的方法来处理文件和目录的路径。Path.unlink()是删除文件的一种方法。
from pathlib import Path
def unlink_path_file(file_path):
path = Path(file_path)
try:
path.unlink()
print(f"File '{file_path}' has been deleted successfully.")
except FileNotFoundError:
print(f"File '{file_path}' not found.")
except PermissionError:
print(f"Permission denied to delete '{file_path}'.")
except Exception as e:
print(f"An error occurred: {e}")
示例
unlink_path_file("sample.txt")
使用pathlib模块的一个优点是,它提供了更多的路径操作功能,如路径拼接、路径属性获取等。
四、删除文件前的检查
1、检查文件是否存在
在删除文件之前,最好先检查文件是否存在,这样可以避免不必要的异常。
import os
def delete_file_with_check(file_path):
if os.path.exists(file_path):
try:
os.remove(file_path)
print(f"File '{file_path}' has been deleted successfully.")
except PermissionError:
print(f"Permission denied to delete '{file_path}'.")
except Exception as e:
print(f"An error occurred: {e}")
else:
print(f"File '{file_path}' does not exist.")
示例
delete_file_with_check("sample.txt")
2、检查文件权限
确保程序具有删除文件的权限也是非常重要的。在尝试删除文件之前,可以使用os.access()检查权限。
import os
def delete_file_with_permission_check(file_path):
if os.path.exists(file_path) and os.access(file_path, os.W_OK):
try:
os.remove(file_path)
print(f"File '{file_path}' has been deleted successfully.")
except Exception as e:
print(f"An error occurred: {e}")
elif not os.path.exists(file_path):
print(f"File '{file_path}' does not exist.")
else:
print(f"Permission denied to delete '{file_path}'.")
示例
delete_file_with_permission_check("sample.txt")
五、处理多个文件
1、批量删除文件
有时需要删除多个文件,这可以通过遍历文件列表并调用删除函数来实现。
import os
def delete_multiple_files(file_list):
for file_path in file_list:
if os.path.exists(file_path):
try:
os.remove(file_path)
print(f"File '{file_path}' has been deleted successfully.")
except PermissionError:
print(f"Permission denied to delete '{file_path}'.")
except Exception as e:
print(f"An error occurred: {e}")
else:
print(f"File '{file_path}' does not exist.")
示例
delete_multiple_files(["sample1.txt", "sample2.txt", "sample3.txt"])
2、删除目录中的所有文件
如果需要删除目录中的所有文件,可以使用os.listdir()来获取目录中的文件列表,然后逐个删除。
import os
def delete_all_files_in_directory(directory_path):
if os.path.exists(directory_path) and os.path.isdir(directory_path):
for file_name in os.listdir(directory_path):
file_path = os.path.join(directory_path, file_name)
if os.path.isfile(file_path):
try:
os.remove(file_path)
print(f"File '{file_path}' has been deleted successfully.")
except PermissionError:
print(f"Permission denied to delete '{file_path}'.")
except Exception as e:
print(f"An error occurred: {e}")
else:
print(f"Directory '{directory_path}' does not exist or is not a directory.")
示例
delete_all_files_in_directory("sample_directory")
六、使用shutil模块删除文件夹
如果需要删除整个文件夹及其内容,可以使用shutil.rmtree()方法。
import shutil
def delete_directory(directory_path):
if os.path.exists(directory_path) and os.path.isdir(directory_path):
try:
shutil.rmtree(directory_path)
print(f"Directory '{directory_path}' has been deleted successfully.")
except Exception as e:
print(f"An error occurred: {e}")
else:
print(f"Directory '{directory_path}' does not exist or is not a directory.")
示例
delete_directory("sample_directory")
七、注意事项
1、谨慎操作
删除文件是一个不可逆的操作,因此在进行删除操作之前,需要确保文件的删除是必要的,避免误删重要文件。
2、处理异常
在删除文件的过程中,可能会遇到各种异常情况,如文件不存在、权限不足等。通过捕获异常并进行适当处理,可以提高程序的健壮性。
3、备份重要文件
对于重要文件,建议在删除之前进行备份,以防止数据丢失。
八、总结
本文详细介绍了如何使用Python删除文件的多种方法,包括os.remove()、os.unlink()和pathlib.Path.unlink(),并提供了多种实用的示例代码。通过结合使用这些方法和技巧,可以有效地管理文件删除操作。无论是单个文件的删除,还是批量文件的处理,Python都提供了灵活且强大的工具来满足不同的需求。在实际应用中,需根据具体的场景选择合适的方法,并始终注意数据的安全性和程序的健壮性。
相关问答FAQs:
如何在Python中检查文件是否存在?
在使用Python删除文件之前,确认文件是否存在非常重要。可以使用os.path
模块中的exists()
函数来检查文件的存在性。例如,使用以下代码可以有效地验证文件是否存在:
import os
file_path = 'your_file.txt'
if os.path.exists(file_path):
print("文件存在,可以进行删除操作。")
else:
print("文件不存在,无法删除。")
使用Python删除文件时有哪些安全措施?
在删除文件时,建议采取一些安全措施以避免意外删除重要文件。可以考虑使用try
和except
语句来捕获可能发生的异常,例如文件不存在或权限问题。此外,可以在删除之前提示用户确认删除操作。示例如下:
import os
file_path = 'your_file.txt'
if os.path.exists(file_path):
confirm = input("您确定要删除此文件吗?(yes/no): ")
if confirm.lower() == 'yes':
os.remove(file_path)
print("文件已成功删除。")
else:
print("文件未删除。")
else:
print("文件不存在,无法删除。")
Python可以删除哪些类型的文件?
使用Python的os.remove()
函数可以删除任何类型的文件,包括文本文件、图像文件、音频文件等。然而,需要注意的是,删除操作是不可逆的,删除后文件将无法恢复。因此,确保在删除之前备份重要数据或文件是个明智的选择。
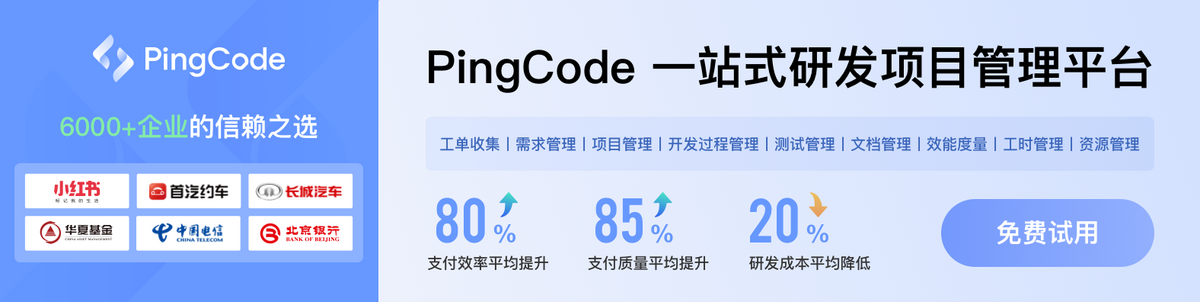