Python查看电脑是否为64位操作系统的方法包括:使用platform
模块、使用sys
模块、查看操作系统环境变量。下面将详细介绍其中一种方法,即使用platform
模块来查看电脑是否为64位操作系统。
使用platform
模块是查看电脑操作系统架构的常用方法之一。platform
模块提供了许多函数来获取操作系统的信息,其中platform.architecture()
函数可以返回操作系统的位数。通过调用这个函数,我们可以轻松地判断当前系统是32位还是64位。
import platform
def check_system_architecture():
architecture = platform.architecture()[0]
print(f"Your system is: {architecture}")
check_system_architecture()
在上述代码中,我们导入了platform
模块,然后使用platform.architecture()
函数来获取系统的位数。该函数返回一个包含两个元素的元组,第一个元素表示操作系统的位数(如'32bit'或'64bit'),第二个元素通常是空字符串或'C'。我们只需关注第一个元素即可。运行这段代码后,将会在控制台输出系统的位数信息。
接下来,我们将详细介绍其他几种方法来查看电脑是否为64位操作系统。
一、使用SYS模块
sys
模块是Python的标准库之一,提供了一些与Python解释器相关的变量和函数。通过sys
模块,我们也可以获取操作系统的架构信息。
1. 使用sys.maxsize
sys.maxsize
是一个大整数,它表示Python解释器能够处理的最大整数值。通常情况下,在32位系统上,它的值为2^31 – 1,而在64位系统上,它的值为2^63 – 1。通过这一特性,我们可以间接判断系统的位数。
import sys
def check_system_architecture_by_maxsize():
if sys.maxsize > 232:
print("Your system is 64-bit")
else:
print("Your system is 32-bit")
check_system_architecture_by_maxsize()
在这段代码中,我们通过判断sys.maxsize
的大小来确定系统的位数。如果sys.maxsize
大于2^32,则说明系统是64位的,否则是32位的。
2. 使用sys.platform
sys.platform
返回一个字符串,表示当前操作系统的名称。虽然这个方法不能直接告诉我们系统的位数,但结合其他信息可以帮助我们做出判断。例如,在Windows系统中,'win32'表示32位,而'win64'表示64位。
import sys
def check_system_architecture_by_platform():
platform = sys.platform
if "win32" in platform:
print("Your system is 32-bit Windows")
elif "win64" in platform:
print("Your system is 64-bit Windows")
else:
print(f"Your system platform is: {platform}")
check_system_architecture_by_platform()
二、使用环境变量
有时,操作系统的环境变量中也包含系统架构的信息。我们可以通过os
模块来访问这些环境变量,并从中提取相关信息。
1. 使用os.environ
os.environ
是一个字典对象,包含了系统的环境变量。我们可以通过访问相关的环境变量来判断系统的位数。
import os
def check_system_architecture_by_environ():
architecture = os.environ.get("PROCESSOR_ARCHITECTURE", "")
if architecture == "AMD64":
print("Your system is 64-bit")
elif architecture == "x86":
print("Your system is 32-bit")
else:
print(f"Processor architecture: {architecture}")
check_system_architecture_by_environ()
在Windows系统中,PROCESSOR_ARCHITECTURE
环境变量可能的值为'AMD64'(表示64位)或'x86'(表示32位)。通过读取这个变量的值,我们可以判断系统的位数。
三、使用CTYPES模块
ctypes
模块提供了与C语言库交互的功能。通过ctypes
模块,我们可以调用Windows API函数来获取系统信息。
1. 使用ctypes.windll.kernel32
在Windows系统中,我们可以使用ctypes.windll.kernel32
来调用Windows API函数GetNativeSystemInfo
,获取系统的架构信息。
import ctypes
def check_system_architecture_by_ctypes():
class SYSTEM_INFO(ctypes.Structure):
_fields_ = [("wProcessorArchitecture", ctypes.c_ushort),
("wReserved", ctypes.c_ushort),
("dwPageSize", ctypes.c_uint),
("lpMinimumApplicationAddress", ctypes.c_void_p),
("lpMaximumApplicationAddress", ctypes.c_void_p),
("dwActiveProcessorMask", ctypes.c_void_p),
("dwNumberOfProcessors", ctypes.c_uint),
("dwProcessorType", ctypes.c_uint),
("dwAllocationGranularity", ctypes.c_uint),
("wProcessorLevel", ctypes.c_ushort),
("wProcessorRevision", ctypes.c_ushort)]
system_info = SYSTEM_INFO()
ctypes.windll.kernel32.GetNativeSystemInfo(ctypes.byref(system_info))
if system_info.wProcessorArchitecture == 9:
print("Your system is 64-bit")
elif system_info.wProcessorArchitecture == 0:
print("Your system is 32-bit")
else:
print(f"Processor architecture: {system_info.wProcessorArchitecture}")
check_system_architecture_by_ctypes()
在这个例子中,我们定义了一个SYSTEM_INFO
结构体,用于存储系统信息。然后,我们通过ctypes.windll.kernel32.GetNativeSystemInfo
函数获取系统信息,并通过wProcessorArchitecture
字段判断系统的位数。值为9表示64位,0表示32位。
四、总结与最佳实践
在Python中,我们有多种方法可以判断系统的位数。根据具体需求和环境,我们可以选择最合适的方法来实现这一任务。对于大多数情况,使用platform
模块是最简单和直接的方法。而在Windows系统中,结合os.environ
和ctypes
模块的方法也非常实用。
在实际应用中,为了提高程序的兼容性和可维护性,建议尽量使用跨平台的方法(如platform
模块)来获取系统信息。此外,在开发过程中,保持代码的清晰和简洁,添加适当的注释,将有助于提高代码的可读性和易用性。
相关问答FAQs:
如何判断我的电脑是否是64位操作系统?
要确认您的电脑是否使用64位操作系统,可以通过以下步骤进行检查:在Windows系统中,右击“此电脑”或“计算机”,选择“属性”,在系统类型中查看是32位还是64位。在Mac系统中,点击苹果菜单,选择“关于本机”,然后点击“系统报告”,在“硬件”部分查看处理器的信息,64位的处理器通常会标记为“x86_64”。
Python在64位操作系统上有什么优势?
使用64位操作系统可以让Python程序处理更大的数据集和使用更多的内存。对于需要大量内存的应用,比如数据科学或机器学习项目,64位的Python版本能够更有效地利用系统资源,从而提升性能和处理速度。
如何在64位系统上安装Python?
在64位操作系统上安装Python非常简单。您可以访问Python的官方网站,选择适合64位系统的安装包下载。安装过程中,确保勾选“Add Python to PATH”选项,这样您在命令行中便能直接使用Python。安装完成后,可以通过在命令行中输入“python –version”来确认安装是否成功及其版本。
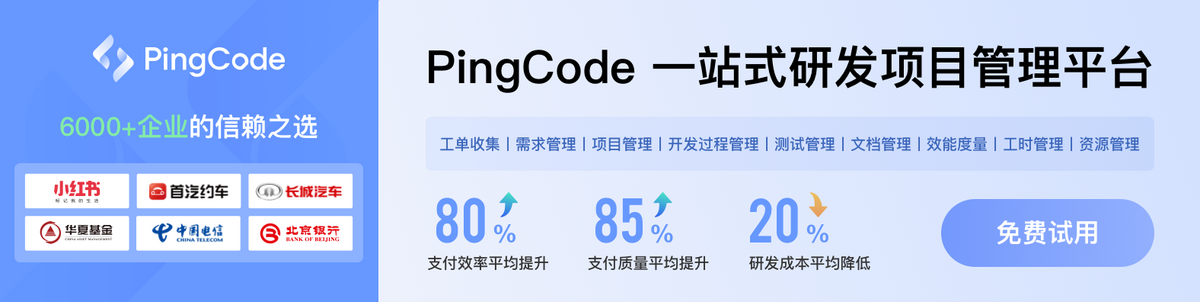