要在Python中让图案旋转,可以使用图形库如Turtle、Pygame或Matplotlib,基本方法是通过数学变换、使用旋转矩阵、修改坐标位置来实现。其中,最常用的方法是通过旋转矩阵来实现图案的旋转。旋转矩阵通过线性代数的方式,可以在二维平面中将点围绕一个中心点旋转指定的角度。使用旋转矩阵、修改坐标位置、结合Python图形库是实现图案旋转的三种主要方法。接下来,我将详细介绍如何使用旋转矩阵来实现图案的旋转。
旋转矩阵是一种将点绕原点旋转的线性变换工具。在二维平面上,给定一个点的坐标(x, y),旋转矩阵R可以将其旋转θ度,新的坐标(x', y')可以通过以下公式计算:
[
x' = x \cdot \cos(\theta) – y \cdot \sin(\theta)
]
[
y' = x \cdot \sin(\theta) + y \cdot \cos(\theta)
]
这种方式不仅适用于单个点,也适用于复杂图案的每一个组成点。通过修改这些点的坐标,可以实现图案的旋转效果。
一、使用TURTLE库旋转图案
Turtle库是Python内置的一个简单的图形库,非常适合用于学习和制作简单的图形。它提供了一种直观的方式来绘制图案,并且通过简单的命令控制“海龟”来移动和旋转。要实现图案旋转,可以通过调整Turtle的方向来实现。
- 绘制简单图案
首先,让我们使用Turtle库绘制一个简单的图案。假设我们想画一个正方形:
import turtle
t = turtle.Turtle()
for _ in range(4):
t.forward(100)
t.right(90)
turtle.done()
- 实现旋转
为了让这个正方形旋转,我们可以在每次绘制后调整Turtle的方向,使其在每次绘制后都稍微旋转一个小角度:
import turtle
t = turtle.Turtle()
for _ in range(36): # 重复36次,形成旋转效果
for _ in range(4):
t.forward(100)
t.right(90)
t.right(10) # 每次旋转10度
turtle.done()
通过这种方式,我们可以在屏幕上看到一个旋转的正方形图案。
二、使用Pygame库旋转图案
Pygame是一个功能强大的图形库,适合用于更复杂的图形和游戏开发。它提供了对图像、声音和输入设备的支持。在Pygame中,旋转图案通常通过图像旋转来实现。
- 加载和绘制图像
在Pygame中,我们可以通过加载图像并在屏幕上绘制来创建图案。首先,安装Pygame库并创建一个简单的窗口:
pip install pygame
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption('Rotate Image')
加载图像
image = pygame.image.load('your_image.png')
rect = image.get_rect(center=(200, 200))
主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
screen.blit(image, rect)
pygame.display.flip()
pygame.quit()
sys.exit()
- 实现旋转
要实现图像旋转,可以使用Pygame的transform.rotate
方法。这个方法可以创建一个新的旋转图像,并保留原始图像的质量:
import pygame
import sys
pygame.init()
screen = pygame.display.set_mode((400, 400))
pygame.display.set_caption('Rotate Image')
加载图像
image = pygame.image.load('your_image.png')
rect = image.get_rect(center=(200, 200))
angle = 0 # 初始化旋转角度
主循环
running = True
while running:
for event in pygame.event.get():
if event.type == pygame.QUIT:
running = False
screen.fill((255, 255, 255))
# 旋转图像
rotated_image = pygame.transform.rotate(image, angle)
new_rect = rotated_image.get_rect(center=rect.center)
screen.blit(rotated_image, new_rect)
pygame.display.flip()
angle += 1 # 每次循环增加角度,实现旋转效果
pygame.quit()
sys.exit()
通过这种方式,我们可以让图像在屏幕上旋转。
三、使用Matplotlib库旋转图案
Matplotlib是一个用于绘制二维图形的Python库,广泛用于数据可视化。在Matplotlib中,可以通过旋转坐标轴上的数据点来实现图案旋转。
- 绘制基本图案
首先,我们可以使用Matplotlib绘制一个简单的图案,例如一个正方形:
import matplotlib.pyplot as plt
import numpy as np
定义正方形的顶点
square = np.array([[0, 0], [1, 0], [1, 1], [0, 1], [0, 0]])
plt.plot(square[:, 0], square[:, 1])
plt.xlim(-2, 2)
plt.ylim(-2, 2)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
- 实现旋转
在Matplotlib中,可以通过旋转矩阵来旋转图案。我们可以定义一个函数来实现旋转:
import matplotlib.pyplot as plt
import numpy as np
def rotate(points, angle):
theta = np.radians(angle)
c, s = np.cos(theta), np.sin(theta)
rotation_matrix = np.array([[c, -s], [s, c]])
return np.dot(points, rotation_matrix)
定义正方形的顶点
square = np.array([[0, 0], [1, 0], [1, 1], [0, 1], [0, 0]])
旋转正方形
rotated_square = rotate(square, 45)
plt.plot(rotated_square[:, 0], rotated_square[:, 1])
plt.xlim(-2, 2)
plt.ylim(-2, 2)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
通过这种方式,我们可以在Matplotlib中实现图案的旋转。
四、使用旋转矩阵实现复杂图案的旋转
除了简单的图案,旋转矩阵还可以用于更复杂的图案。我们可以创建一个函数来旋转任意复杂的图案。
- 定义旋转函数
为了适应不同的图案,我们可以定义一个通用的旋转函数:
import numpy as np
def rotate(points, angle, center=(0, 0)):
theta = np.radians(angle)
c, s = np.cos(theta), np.sin(theta)
rotation_matrix = np.array([[c, -s], [s, c]])
# 将所有点平移到原点,旋转后再平移回去
shifted_points = points - center
rotated_points = np.dot(shifted_points, rotation_matrix)
return rotated_points + center
- 旋转任意图案
我们可以使用这个函数来旋转任意复杂的图案。假设我们有一个星形图案:
import matplotlib.pyplot as plt
import numpy as np
定义星形的顶点
star = np.array([
[0, 0],
[1, 3],
[2, 0],
[0, 2],
[2, 2],
[0, 0]
])
旋转星形
rotated_star = rotate(star, 30, center=(1, 1.5))
plt.plot(rotated_star[:, 0], rotated_star[:, 1])
plt.xlim(-3, 3)
plt.ylim(-3, 3)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
通过这种方式,我们可以实现任意复杂图案的旋转。
五、结合用户交互实现动态旋转
为了让旋转更具交互性,我们可以结合用户输入或动画来实现动态旋转。
- 使用动画实现动态旋转
我们可以使用Matplotlib的动画功能来实现动态旋转效果:
import matplotlib.pyplot as plt
import numpy as np
from matplotlib.animation import FuncAnimation
def rotate(points, angle, center=(0, 0)):
theta = np.radians(angle)
c, s = np.cos(theta), np.sin(theta)
rotation_matrix = np.array([[c, -s], [s, c]])
shifted_points = points - center
rotated_points = np.dot(shifted_points, rotation_matrix)
return rotated_points + center
定义图案
pattern = np.array([
[0, 0],
[1, 3],
[2, 0],
[0, 2],
[2, 2],
[0, 0]
])
fig, ax = plt.subplots()
ax.set_xlim(-3, 3)
ax.set_ylim(-3, 3)
ax.set_aspect('equal', adjustable='box')
line, = ax.plot([], [])
def init():
line.set_data([], [])
return line,
def update(frame):
rotated_pattern = rotate(pattern, frame, center=(1, 1.5))
line.set_data(rotated_pattern[:, 0], rotated_pattern[:, 1])
return line,
ani = FuncAnimation(fig, update, frames=np.arange(0, 360, 2), init_func=init, blit=True)
plt.show()
- 结合用户输入
通过结合用户输入,我们可以让用户指定旋转角度或速度,实现更灵活的旋转效果:
import matplotlib.pyplot as plt
import numpy as np
def rotate(points, angle, center=(0, 0)):
theta = np.radians(angle)
c, s = np.cos(theta), np.sin(theta)
rotation_matrix = np.array([[c, -s], [s, c]])
shifted_points = points - center
rotated_points = np.dot(shifted_points, rotation_matrix)
return rotated_points + center
定义图案
pattern = np.array([
[0, 0],
[1, 3],
[2, 0],
[0, 2],
[2, 2],
[0, 0]
])
angle = float(input("Enter the rotation angle: "))
rotated_pattern = rotate(pattern, angle, center=(1, 1.5))
plt.plot(rotated_pattern[:, 0], rotated_pattern[:, 1])
plt.xlim(-3, 3)
plt.ylim(-3, 3)
plt.gca().set_aspect('equal', adjustable='box')
plt.show()
通过这种方式,我们可以让用户交互地控制图案的旋转效果。
综上所述,Python提供了多种方法来实现图案的旋转。无论是使用简单的Turtle库,还是功能强大的Pygame和Matplotlib,我们都可以通过数学变换和图形库的结合,实现图案的旋转效果。在实际应用中,可以根据具体需求选择合适的实现方式。
相关问答FAQs:
如何在Python中实现图案的旋转?
要实现图案的旋转,可以使用Python的图形库,如Pygame或Matplotlib。Pygame提供了丰富的功能来处理图形和动画,而Matplotlib则适合处理数据可视化。在Pygame中,可以使用pygame.transform.rotate()
函数来旋转图像;而在Matplotlib中,可以通过imshow()
函数结合transform
参数来实现图案的旋转。
是否可以使用Python的其他库来旋转图案?
当然可以。除了Pygame和Matplotlib,Python还有其他库,例如Pillow(PIL的一个分支),可以轻松实现图像的旋转。使用Pillow时,可以调用Image.rotate()
方法,通过指定角度来旋转图像。此外,OpenCV也支持图像旋转,适合需要进行复杂图像处理的项目。
旋转图案时,如何保持图案的清晰度?
保持图案的清晰度可以通过选择适当的旋转方法和角度来实现。在使用Pygame和Matplotlib时,注意使用抗锯齿选项,确保旋转过程中图像的质量。同时,选择高分辨率的原始图像也是保证旋转后清晰度的关键,避免图像因放大或旋转而失真。
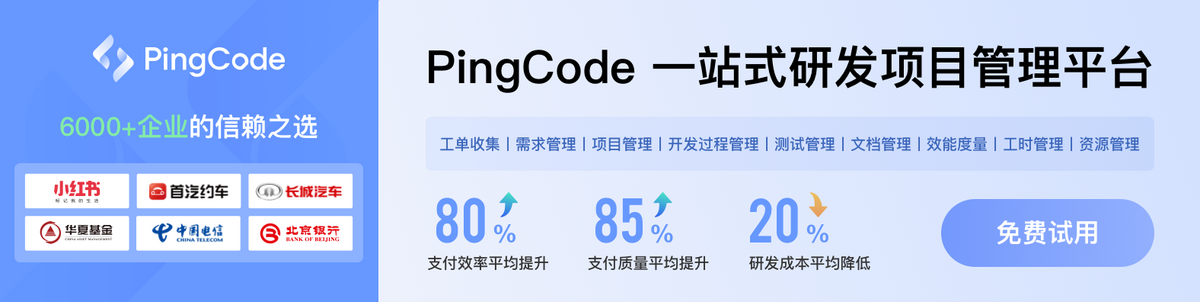